Claim Your Discount Today
Ring in Christmas and New Year with a special treat from www.programminghomeworkhelp.com! Get 15% off on all programming assignments when you use the code PHHCNY15 for expert assistance. Don’t miss this festive offer—available for a limited time. Start your New Year with academic success and savings. Act now and save!
We Accept
- 2. Haskell
- 1. Data Types
- 2. Type Inference
- 3. Lazy Evaluation
- 4. Pattern Matching
- 3. Understanding the Problem
- 1. Breaking Down the Problem
- 4. Defining Data Structures
- 1. Data Types in Haskell
- 2. Creating Sample Data
- 5. Implementing Core Functionalities
- 1. Listing All Place Names
- 2. Calculating Average Rainfall
- 3. Formatting Data for Output
- 4. Updating Rainfall Data
- 5. Replacing a Place
- 6. Finding the Closest Dry Place
- 6. Handling Input/Output Operations
- 1. Reading and Writing Files
- 2. Creating a User Interface
- 7. Ensuring Code Quality
- 1. Writing Clear and Concise Code
- 2. Testing and Debugging
- 3. Refactoring
- Conclusion
Functional programming is a paradigm that treats computation as the evaluation of mathematical functions and avoids changing state and mutable data. It is built on the principles of lambda calculus and emphasizes the use of pure functions, immutability, and higher-order functions. The use of pure functions, immutability, and higher-order functions to create software that is both robust and maintainable. Unlike imperative programming, which relies on changing state and mutable data, functional programming treats computation as the evaluation of mathematical functions, ensuring that each function produces the same result given the same inputs, without side effects.
Haskell is a prominent example of a pure functional programming language. It offers a strong type system, lazy evaluation, and an elegant syntax that aligns well with functional principles. In this data structure assignment, we will harness Haskell's capabilities to manage and analyze rainfall data for various locations.
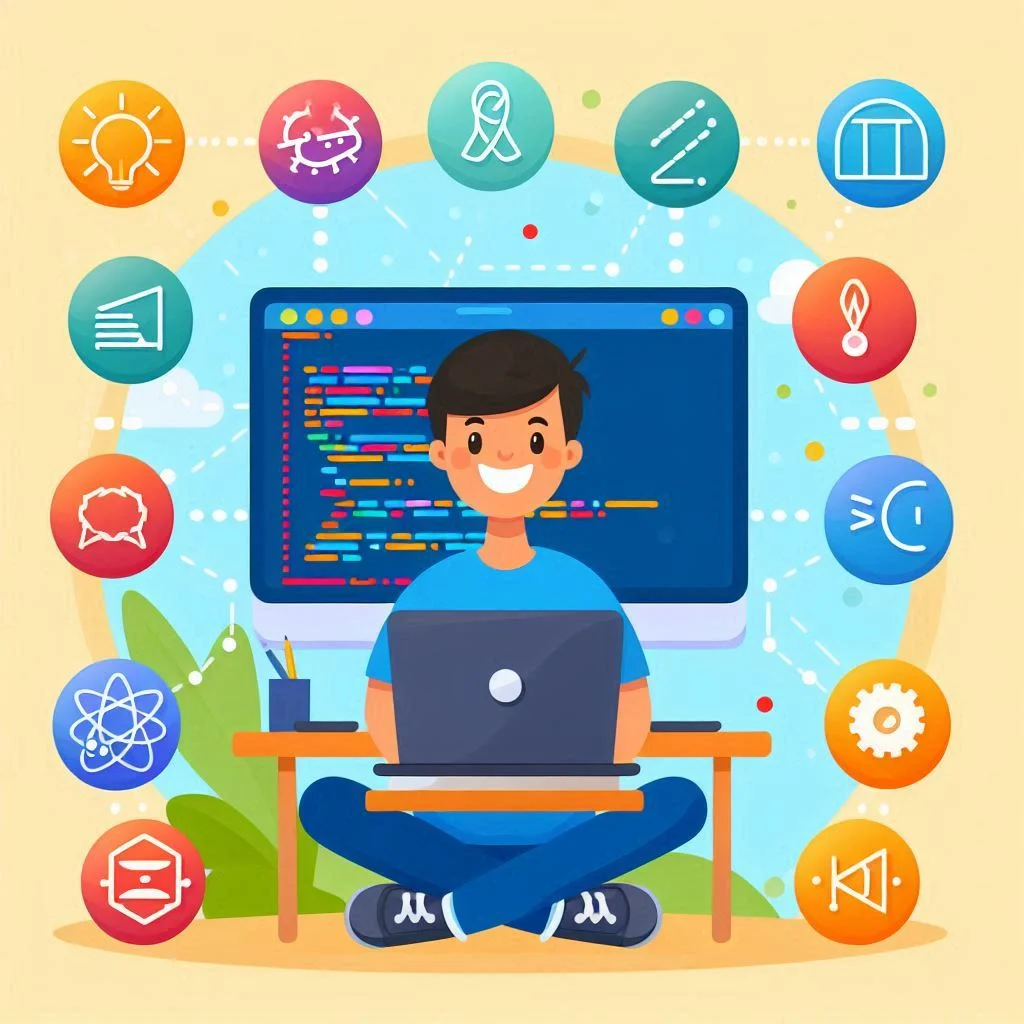
In this programming assignment, we will apply functional programming principles using Haskell to create a system for managing and analyzing rainfall data for various locations. The assignment is designed to help you practice and deepen your understanding of functional programming concepts such as immutability, pure functions, and higher-order functions. Here's a closer look at the key aspects of the assignment:
- Defining Data Structures: Crafting a suitable data model to represent places and their associated rainfall data.
- Implementing Core Functionalities: Developing functions to calculate averages, update records, and more, all while adhering to functional programming principles.
- Handling Input and Output: Managing data through file operations and user interactions.
- Creating a User Interface: Designing a text-based menu to facilitate user interaction with the program.
By delving into this assignment, you'll gain practical experience in applying functional programming concepts to a real-world problem, leveraging Haskell's powerful features to build a comprehensive solution for managing rainfall data.
Here’s a detailed look at the core concepts of functional programming:
1. Pure Functions
Pure functions are functions where the output value is determined only by the input values, without observable side effects. This means:
- Deterministic: The same input always produces the same output.
- No Side Effects: The function does not modify any external state or variables.
For example, a function that computes the square of a number is pure:
square :: Int -> Int
square x = x * x
2. Immutability
In functional programming, data is immutable, meaning it cannot be changed once created. Instead of modifying existing data, you create new data. This avoids issues related to shared state and side effects.
For instance, if you want to update a list, you create a new list with the desired changes rather than modifying the existing list:
originalList = [1, 2, 3]
newList = 0 : originalList -- New list with 0 prepended
3. Higher-Order Functions
Higher-order functions are functions that can take other functions as arguments or return functions as results. They enable powerful and flexible operations on data.
Common higher-order functions include:
- map: Applies a function to each element of a list.
map (*2) [1, 2, 3] -- Result: [2, 4, 6]
- filter: Selects elements from a list that satisfy a predicate.
filter even [1, 2, 3, 4] -- Result: [2, 4]
- foldr: Reduces a list to a single value using a binary function.
foldr (+) 0 [1, 2, 3] -- Result: 6
4. Recursion
Functional programming relies heavily on recursion as the primary mechanism for iteration. Recursion occurs when a function calls itself in order to solve a problem.
Example of a recursive function to compute the factorial of a number:
factorial :: Int -> Int
factorial 0 = 1
factorial n = n * factorial (n - 1)
2. Haskell
Haskell is a pure functional programming language known for its strong type system, laziness, and emphasis on immutability and pure functions. Here are some core concepts:
1. Data Types
Haskell uses a strong and static type system to enforce correctness and improve safety. Key types include:
- Primitive Types: Int, Float, Char, Bool.
- Lists: Ordered collections of elements of the same type.
numbers :: [Int]
numbers = [1, 2, 3, 4]
- Tuples: Fixed-size collections of elements of potentially different types.
person :: (String, Int) person = ("Alice", 30)
- Custom Data Types: Defined using the data keyword to create complex structures.
data Person = Person {
name :: String,
age :: Int
}
2. Type Inference
Haskell has a powerful type inference system, which can automatically deduce the types of expressions. You usually don't need to explicitly specify types, but you can for clarity.
For example, Haskell can infer the type of the square function:
square :: Int -> Int
square x = x * x
3. Lazy Evaluation
Haskell uses lazy evaluation, meaning expressions are not evaluated until their values are needed. This allows for efficient computation and the creation of infinite data structures.
Example of lazy evaluation with an infinite list:
<code ignore--minify class="code-view">square :: Int -> Int
square x = x * x
</code>
4. Pattern Matching
Pattern matching allows you to decompose and match data structures directly in function definitions and case expressions.
Example with a list:
headOfList :: [a] -> a
headOfList [] = error "Empty list"
headOfList (x:_) = x
3. Understanding the Problem
Functional programming assignments often require you to solve problems using principles unique to functional programming, such as immutability and higher-order functions. For example, in the given assignment on rainfall data, you'll need to implement a Haskell program to manage and query rainfall data for various locations.
1. Breaking Down the Problem
The assignment involves several tasks:
- Data Management: You need to handle a list of places, each with associated rainfall data.
- Data Querying: Implement functions to retrieve specific information, such as average rainfall or locations with zero rainfall on a given day.
- Data Updating: You must be able to update rainfall records and replace existing places.
- User Interface: Finally, your program should provide a user interface for interaction.
To tackle these tasks, you should:
- Define Data Structures: Represent places and their rainfall data in a way that supports the required operations.
- Implement Core Functionalities: Write pure functions to handle data querying and updating.
- Handle I/O Operations: Implement functions for reading and writing data to files and interacting with users.
- Create a User Interface: Design a text-based menu to allow users to interact with the program.
4. Defining Data Structures
In Haskell, data structures are crucial for organizing and manipulating data. For the rainfall data assignment, you need a data structure to represent each place and its associated information.
1. Data Types in Haskell
Define a Place type to encapsulate all necessary information:
data Place = Place { name :: String, -- Name of the place latitude :: Float, -- Latitude in degrees longitude :: Float, -- Longitude in degrees rainfall :: [Float] -- List of daily rainfall figures for the past week } deriving (Show)
In this definition:
- name is a String representing the name of the place.
- latitude and longitude are Float values representing the geographical location.
- rainfall is a list of Float values representing daily rainfall for the past 7 days.
2. Creating Sample Data
For testing purposes, you might need sample data. Create a list of Place values to use during development:
testData :: [Place]
testData = [
Place "London" 51.5074 (-0.1278) [0.1, 0.2, 0.0, 0.0, 0.3, 0.4, 0.1],
Place "Edinburgh" 55.9533 (-3.1883) [0.0, 0.0, 0.0, 0.2, 0.1, 0.0, 0.0],
-- Add more test places as needed
]
5. Implementing Core Functionalities
Once the data structures are defined, you can start implementing core functionalities. Each functionality should be implemented as a pure function where possible.
1. Listing All Place Names
To list all place names, you can use the map function:
listPlaceNames :: [Place] -> [String]
listPlaceNames places = map name places
This function takes a list of Place and returns a list of their names.
2. Calculating Average Rainfall
To compute the average rainfall for a specific place:
averageRainfall :: [Float] -> Float
averageRainfall rainfalls = sum rainfalls / fromIntegral (length rainfalls)
averageRainfallForPlace :: String -> [Place] -> Maybe Float
averageRainfallForPlace placeName places =
case filter ((== placeName) . name) places of
[place] -> Just (averageRainfall (rainfall place))
_ -> Nothing
The averageRainfall function computes the average from a list of rainfall values. The averageRainfallForPlace function finds a place by name and calculates its average rainfall.
3. Formatting Data for Output
To format the data for display, you might need to convert the data into a string representation:
import Text.Printf (printf)
placesToString :: [Place] -> String
placesToString places = unlines $ map placeToString places
where
placeToString (Place name lat long rainfalls) =
printf "%-15s %6.2f %7.2f %s" name lat long (show rainfalls)
This function formats each Place into a string with specified width and alignment.
4. Updating Rainfall Data
To update the rainfall data by removing the oldest figure and adding a new one:
updateRainfall :: [Place] -> [Float] -> [Place]
updateRainfall places newRainfalls =
zipWith updatePlace places newRainfalls
where
updatePlace place newRainfall =
place { rainfall = init (rainfall place) ++ [newRainfall] }
Here, updatePlace replaces the old rainfall data with the new data.
5. Replacing a Place
To replace an existing place with a new one:
replacePlace :: [Place] -> Place -> [Place]
replacePlace places newPlace =
newPlace : filter ((/= name newPlace) . name) places
This function replaces the place with the given name with a new place.
6. Finding the Closest Dry Place
To find the closest place with zero rainfall on a given day, you need to calculate distances:
import Data.List (minimumBy)
import Data.Ord (comparing)
distance :: Place -> Place -> Float
distance p1 p2 = sqrt ((lat1 - lat2)^2 + (long1 - long2)^2)
where
lat1 = latitude p1
long1 = longitude p1
lat2 = latitude p2
long2 = longitude p2
closestDryPlace :: Float -> Float -> Int -> [Place] -> Maybe Place
closestDryPlace lat long day places =
case filter (\p -> rainfall p !! (7 - day) == 0) places of
[] -> Nothing
dryPlaces -> Just (minimumBy (comparing (distance (Place "" lat long []))) dryPlaces)
This function finds the closest place with zero rainfall on a specified day.
6. Handling Input/Output Operations
In Haskell, I/O operations are handled separately from pure functions. You need to implement functions for reading from and writing to files and for interacting with users.
1. Reading and Writing Files
To read a list of places from a file:
import System.IO (readFile)
import Text.Read (readMaybe)
readPlacesFromFile :: FilePath -> IO [Place]
readPlacesFromFile filePath = do
contents <- readFile filePath
let places = readMaybe contents :: Maybe [Place]
return (fromMaybe [] places)
To write a list of places to a file:
import System.IO (writeFile)
writePlacesToFile :: FilePath -> [Place] -> IO ()
writePlacesToFile filePath places = writeFile filePath (show places)
2. Creating a User Interface
A simple text-based menu can be implemented using Haskell’s IO functions:
main :: IO ()
main = do
putStrLn "Welcome to the Rainfall Program!"
putStrLn "1. List all places"
putStrLn "2. Get average rainfall"
putStrLn "3. Update rainfall data"
putStrLn "4. Exit"
choice <- getLine
case choice of
"1" -> listAllPlaces
"2" -> getAverageRainfall
"3" -> updateRainfallData
"4" -> return ()
_ -> putStrLn "Invalid choice" >> main
Each menu option corresponds to a different function or set of functions. Implement these functions to handle user input and display results.
7. Ensuring Code Quality
Code quality is essential for maintainability and readability. In functional programming, this involves:
1. Writing Clear and Concise Code
Use Haskell’s powerful functional constructs to write concise and readable code. Higher-order functions, list comprehensions, and pattern matching can help make your code more elegant.
2. Testing and Debugging
Test your functions thoroughly to ensure they work as expected. Use sample data and edge cases to verify that your functions handle all scenarios correctly. For instance, test the averageRainfallForPlace function with various place names and rainfall data.
3. Refactoring
Review your code to identify opportunities for refactoring. For example, if you find repeated patterns, consider abstracting them into helper functions. Refactoring improves code readability and can enhance performance.
Conclusion
In summary, solving Haskell programming assignments involves:
- Understanding the Problem: Break down the assignment into manageable tasks and understand the requirements.
- Defining Data Structures: Create appropriate data types to represent the problem domain.
- Implementing Core Functionalities: Write pure functions to handle data querying, updating, and formatting.
- Handling Input/Output: Implement functions for file operations and user interaction.
- Ensuring Code Quality: Write clear, concise code and test it thoroughly.
By following these steps, you’ll be well-equipped to solve programming assignments effectively. Whether you’re working with Haskell or another functional language, the principles of immutability, pure functions, and higher-order functions will guide you in writing robust and elegant solutions.