Claim Your Offer
Unlock an amazing offer at www.programminghomeworkhelp.com with our latest promotion. Get an incredible 10% off on your all programming assignment, ensuring top-quality assistance at an affordable price. Our team of expert programmers is here to help you, making your academic journey smoother and more cost-effective. Don't miss this chance to improve your skills and save on your studies. Take advantage of our offer now and secure exceptional help for your programming assignments.
We Accept
- Understanding List Indexing and Slicing
- Accessing List Elements
- Slicing Lists
- Common Errors in List Indexing and Slicing
- Practical Examples
- Python Problems related to List Manipulation
- Understanding Tuples and Slicing
- What Are Tuples?
- Accessing Tuple Elements
- Slicing Tuples
- Common Errors in Tuple Indexing and Slicing
- Practical Examples
- Python Problems Related to Tuples
- Positional Parameters in Functions
- Definition and Role
- Practical Example
- Python Problems Related to Function Definitions and Parameters
- Defining Functions with Positional Parameters
- Example Task
- Keyword Parameters in Functions
- Definition and Role
- Practical Example
- Defining Functions with Positional and Keyword Parameters
- Example Task
- Function Output and Tuple Indexing
- Understanding and Predicting Outputs
- Common Errors
- Defining Functions with Conditional Logic
- Example Task
- Defining Functions with Complex Logic and Tuples
- Example Task
- Computing Averages from Nested Dictionaries
- Example Task
- Understanding and Debugging Function Outputs with Dictionaries
- Example Task
- Modifying Dictionary Entries
- Example Task
- Python Problems Related to Dictionary Operations
- Conclusion
Python's simplicity and power make it a popular choice for programmers across the globe. At the heart of Python programming are lists, tuples, functions, and dictionaries—each offering unique capabilities and serving specific roles. This blog delves deeply into these fundamental constructs, explaining key concepts, common practices, and advanced techniques to help you successfully complete your programming assignments, similar to those encountered in courses or take-home exams.
Understanding List Indexing and Slicing
Accessing List Elements
Lists in Python are mutable, ordered collections of items. Each item in a list is accessed via an index, starting from 0. This indexing allows you to retrieve or manipulate elements efficiently.
Consider the following list:
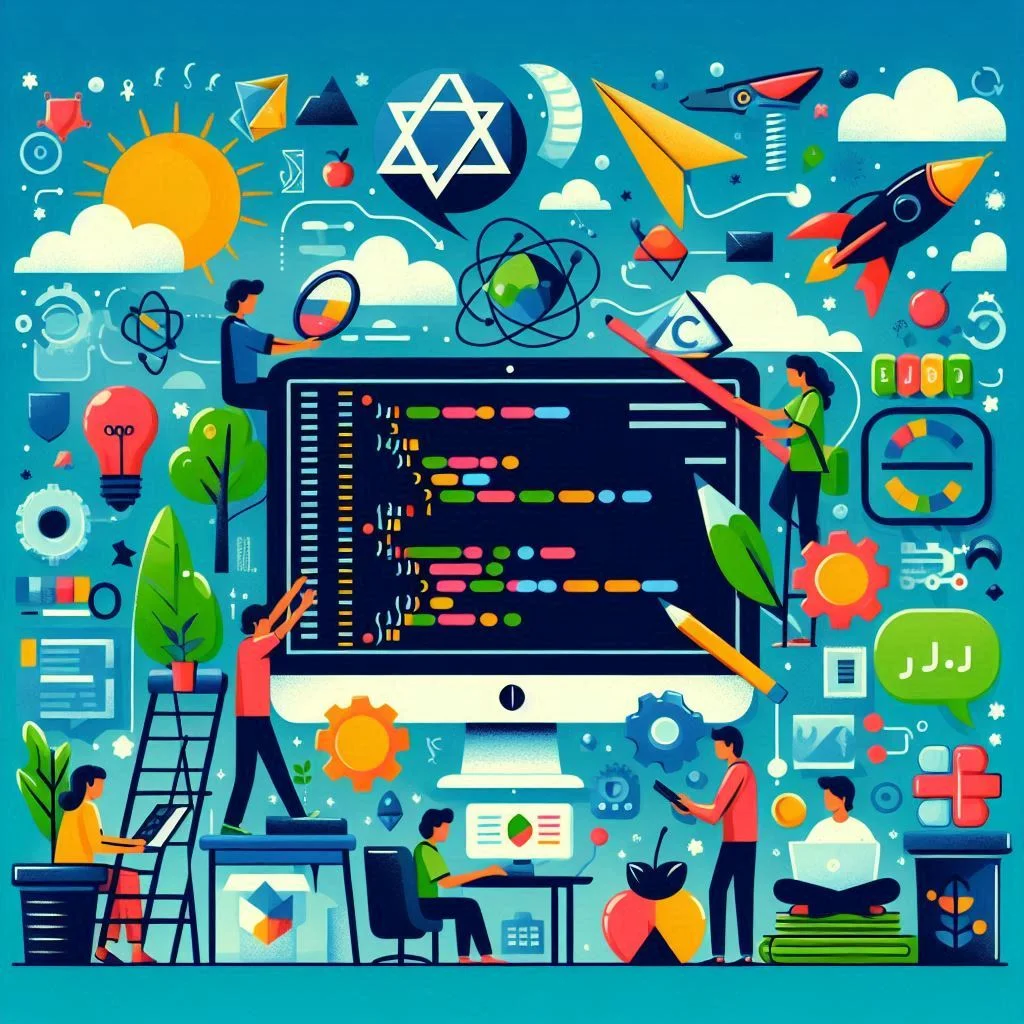
fruits = ['apple', 'banana', 'cherry', 'date', 'elderberry']
To access the first element ('apple'), you use:
print(fruits[0]) # Output: apple
To access the last element ('elderberry'), you can use negative indexing:
print(fruits[-1]) # Output: elderberry
Slicing Lists
Slicing allows you to create sublists from an existing list. The syntax for slicing is list[start:stop:step], where start is the index to begin slicing, stop is the index to end (excluding), and step defines the stride between elements.
# Slice from index 1 to 3
sublist = fruits[1:4]
print(sublist) # Output: ['banana', 'cherry', 'date']
# Slice with a step of 2
sublist_step = fruits[::2]
print(sublist_step) # Output: ['apple', 'cherry', 'elderberry']
Slicing is particularly useful for manipulating and analyzing parts of a list without altering the original list.
Common Errors in List Indexing and Slicing
- IndexError: This occurs when you try to access an index that is out of the range of the list.
print(fruits[10]) # IndexError: list index out of range
- TypeError: This happens if you use a non-integer value for an index or slice.
print(fruits['one']) # TypeError: list indices must be integers or slices, not str
Practical Examples
Let's consider a practical example where slicing is used to manage a list of employee records:
employees = ['John Doe', 'Jane Smith', 'Emily Davis', 'Michael Brown', 'Sarah Wilson']
# Get the first three employees
first_three = employees[:3]
print(first_three) # Output: ['John Doe', 'Jane Smith', 'Emily Davis']
# Get every second employee from the list
every_second = employees[::2]
print(every_second) # Output: ['John Doe', 'Emily Davis', 'Sarah Wilson']
In this example, slicing helps extract specific subsets of data, which is useful for reporting and analysis.
Python Problems related to List Manipulation
Assignments might require you to:
- Create and modify lists: Write functions to add, remove, or modify elements in a list.
- Sort and filter lists: Implement sorting algorithms or use built-in methods to sort and filter lists based on conditions.
- Slicing and indexing: Extract sublists or specific elements based on indexing and slicing techniques.
Example Task: Write a function that takes a list of numbers and returns a list of only the even numbers, sorted in ascending order.
def filter_and_sort_even_numbers(numbers):
even_numbers = [num for num in numbers if num % 2 == 0]
return sorted(even_numbers)
Understanding Tuples and Slicing
What Are Tuples?
Tuples are immutable sequences, meaning once created, their contents cannot be changed. They are defined using parentheses ().
coordinates = (10.0, 20.0, 30.0)
Tuples are useful when you need to ensure that the data remains constant and unmodified. They can be used to represent fixed collections of items, such as geographical coordinates or RGB values.
Accessing Tuple Elements
Similar to lists, tuples are accessed using indices:
print(coordinates[1]) # Output: 20.0
Slicing Tuples
# Slice from index 1 to 2
subset = coordinates[1:3]
print(subset) # Output: (20.0, 30.0)
Common Errors in Tuple Indexing and Slicing
Since tuples are immutable, attempting to modify an element will result in an error:
coordinates[1] = 25.0 # TypeError: 'tuple' object does not support item assignment
Practical Examples
Let's consider a scenario where tuples are used to store fixed data about locations:
locations = (
('Paris', 48.8566, 2.3522),
('New York', 40.7128, -74.0060),
('Tokyo', 35.6895, 139.6917)
)
# Get the coordinates of New York
ny_coordinates = locations[1][1:]
print(ny_coordinates) # Output: (40.7128, -74.0060)
Tuples are ideal for grouping related data together without risking modification.
Python Problems Related to Tuples
Assignments may involve:
- Creating and accessing tuples: Define tuples and access their elements.
- Tuple unpacking: Use tuples to return multiple values from functions and unpack them in a meaningful way.
- Slicing tuples: Extract parts of tuples using slicing techniques.
Example Task: Create a function that returns the first and last elements of a tuple.
def first_and_last(tuple_data):
return (tuple_data[0], tuple_data[-1])
Positional Parameters in Functions
Definition and Role
Positional parameters are the most common type of parameters used in functions. They are defined by their position in the function call. When calling the function, the order of the arguments must match the order of the parameters.
def greet(name, age):
print(f"Hello, {name}. You are {age} years old.")
When you call this function:
greet('Alice', 30) # Output: Hello, Alice. You are 30 years old.
The value 'Alice' is assigned to name and 30 is assigned to age.
Practical Example
Let's create a function that calculates the area of a rectangle using positional parameters:
def rectangle_area(length, width):
return length * width
# Example usage
area = rectangle_area(5, 10)
print(area) # Output: 50
In this example, the function uses positional parameters to compute the area based on the provided length and width.
Python Problems Related to Function Definitions and Parameters
Assignments often include:
- Defining functions: Write functions with various parameters, including positional, keyword, and default parameters.
- Handling function outputs: Return values from functions and use them effectively in other parts of the program.
- Complex logic: Implement functions with conditional logic and nested structures.
Example Task: Define a function that calculates the total price of items with optional discount and tax rate.
Python code
def calculate_total_price(price, discount=0, tax_rate=0):
discounted_price = price * (1 - discount / 100)
total_price = discounted_price * (1 + tax_rate / 100)
return total_price
Defining Functions with Positional Parameters
Example Task
Define a function to compute the area of a rectangle with length and width as positional parameters. The function should return the area and handle cases where either dimension might be zero or negative.
def rectangle_area(length, width):
if length <= 0 or width <= 0:
return "Invalid dimensions"
return length * width
# Example usage
print(rectangle_area(5, 10)) # Output: 50
print(rectangle_area(5, 0)) # Output: Invalid dimensions
In this function, we add conditional logic to handle invalid input, ensuring that only positive dimensions are accepted.
Keyword Parameters in Functions
Definition and Role
Keyword parameters allow you to specify arguments by name, which can make function calls more readable and flexible. They also allow you to provide default values for parameters.
def describe_person(name, age=30, city='Unknown'):
print(f"{name} is {age} years old and lives in {city}.")
You can call this function with or without specifying the keyword arguments:
describe_person('Alice') # Output: Alice is 30 years old and lives in Unknown.
describe_person('Bob', city='New York') # Output: Bob is 30 years old and lives in New York.
Practical Example
Consider a function that calculates the price of an item with optional discount and tax rate:
def calculate_total(item_name, price, discount=0, tax_rate=0):
discounted_price = price - (price * discount / 100)
total_price = discounted_price + (discounted_price * tax_rate / 100)
return f"Total cost for {item_name} is ${total_price:.2f}"
# Example usage
print(calculate_total('Laptop', 1000, discount=10, tax_rate=5))
# Output: Total cost for Laptop is $945.00
In this function, keyword parameters discount and tax_rate provide default values, allowing for flexible function calls.
Defining Functions with Positional and Keyword Parameters
Example Task
Define a function that calculates the total cost of an order. The function should use positional parameters for the item name and price, and keyword parameters for discount and tax rate. The total cost should be calculated based on these parameters.
def calculate_order_total(item_name, price, discount=0, tax_rate=0):
discounted_price = price - (price * discount / 100)
total_price = discounted_price + (discounted_price * tax_rate / 100)
return f"Total cost for {item_name} is ${total_price:.2f}"
# Example usage
print(calculate_order_total('Smartphone', 800, discount=15, tax_rate=7))
# Output: Total cost for Smartphone is $690.40
This function uses both positional and keyword parameters to compute and return the total cost of an order, providing flexibility and clarity in function usage.
Function Output and Tuple Indexing
Understanding and Predicting Outputs
Functions can return tuples to provide multiple results from a single function call. To access these results, you use tuple indexing.
Consider a function that returns statistics about a list of numbers:
def compute_stats(numbers):
total = sum(numbers)
count = len(numbers)
average = total / count if count > 0 else 0
return (total, count, average)
result = compute_stats([10, 20, 30])
print(result[2]) # Output: 20.0
In this function, result[2] retrieves the average value from the returned tuple.
Common Errors
Ensure that tuple indices are within range to avoid IndexError:
print(result[3]) # IndexError: tuple index out of range
Defining Functions with Conditional Logic
Example Task
Define a function that processes a list of numbers based on a condition parameter. The function should return a new list where even numbers are doubled and odd numbers are halved.
def process_numbers(numbers, condition='even'):
processed = []
for number in numbers:
if condition == 'even' and number % 2 == 0:
processed.append(number * 2)
elif condition == 'odd' and number % 2 != 0:
processed.append(number / 2)
return processed
# Example usage
print(process_numbers([1, 2, 3, 4, 5], condition='even')) # Output: [4, 8]
In this function, the condition parameter determines how the numbers are processed, showcasing how conditional logic can be used to customize function behavior.
Defining Functions with Complex Logic and Tuples
Example Task
Define a function with multiple parameters that processes input values and returns a tuple with computed results based on conditions.
def analyze_data(values, factor=1):
total = sum(values)
average = total / len(values) if values else 0
adjusted_values = [x * factor for x in values]
return (total, average, adjusted_values)
# Example usage
print(analyze_data([10, 20, 30], factor=2))
# Output: (60, 20.0, [20, 40, 60])
This function computes various metrics and adjustments, returning a tuple with the total, average, and adjusted values, illustrating how complex logic can be implemented and returned.
Computing Averages from Nested Dictionaries
Example Task
Define a function to compute averages from nested dictionaries where each dictionary contains lists of numbers. The function should calculate the average of all numbers for each key.
def compute_averages(data):
averages = {}
for key, values in data.items():
total_sum = 0
count = 0
for sublist in values:
total_sum += sum(sublist)
count += len(sublist)
averages[key] = total_sum / count if count > 0 else 0
return averages
# Example usage
data = {
'group1': [[10, 20], [30]],
'group2': [[40, 50, 60], [70]],
}
print(compute_averages(data)) # Output: {'group1': 20.0, 'group2': 55.0}
In this example, the function calculates the average for each group in the nested dictionary, demonstrating how to handle complex data structures and perform calculations.
Understanding and Debugging Function Outputs with Dictionaries
Example Task
Debug a function that compares values from two nested dictionaries to identify matching or differing values.
def compare_values(dict1, dict2):
results = {}
for key in dict1:
if key in dict2:
results[key] = dict1[key] == dict2[key]
else:
results[key] = False
return results
# Example usage
dict1 = {'a': 10, 'b': 20}
dict2 = {'a': 10, 'b': 25}
print(compare_values(dict1, dict2)) # Output: {'a': True, 'b': False}
This function compares values from two dictionaries, returning a new dictionary indicating whether the values for each key are equal, helping in debugging and validation tasks.
Modifying Dictionary Entries
Example Task
Modify specific entries in a dictionary, including adding, updating, and deleting items.
def modify_dictionary(d):
d['new_key'] = 'new_value' # Adding an entry
if 'old_key' in d:
del d['old_key'] # Deleting an entry
d.update({'updated_key': 'updated_value'}) # Updating an entry
return d
# Example usage
my_dict = {'old_key': 'old_value'}
print(modify_dictionary(my_dict)) # Output: {'new_key': 'new_value', 'updated_key': 'updated_value'}
This function demonstrates basic dictionary operations—adding, updating, and deleting entries—illustrating how dictionaries can be dynamically manipulated.
Python Problems Related to Dictionary Operations
Assignments might involve:
- Creating and updating dictionaries: Add, remove, or modify key-value pairs.
- Nested dictionaries: Work with dictionaries inside dictionaries to manage complex data structures.
- Dictionary comprehensions: Use comprehensions to create and manipulate dictionaries.
Example Task: Write a function that merges two dictionaries, with values from the second dictionary overwriting values from the first where keys match.
def merge_dicts(dict1, dict2):
result = dict1.copy()
result.update(dict2)
return result
Conclusion
Python programming assignments related to lists, tuples, functions, and dictionaries are designed to help you grasp these fundamental concepts and apply them effectively. Whether you’re working on basic tasks or more complex projects, understanding these constructs is crucial. By exploring these concepts in detail, we have covered the basics of indexing, slicing, parameter types, and complex data manipulation. These skills form the foundation of effective Python programming, enabling you to handle and process data with precision and flexibility. Continue practicing and applying these techniques to deepen your understanding and enhance your data structure programming capabilities. If you encounter difficulties, leveraging online resources, educational platforms, and community support can provide valuable assistance and enhance your learning experience.