Claim Your Offer
Unlock an amazing offer at www.programminghomeworkhelp.com with our latest promotion. Get an incredible 10% off on your all programming assignment, ensuring top-quality assistance at an affordable price. Our team of expert programmers is here to help you, making your academic journey smoother and more cost-effective. Don't miss this chance to improve your skills and save on your studies. Take advantage of our offer now and secure exceptional help for your programming assignments.
We Accept
- Understanding the Assignment Requirements
- Breaking Down the Assignment
- Identifying Key Functionalities
- Planning Your Approach
- Implementing the Solution
- User Input
- Name Generation
- Statistics Generation
- Graphic Confirmation
- Code Optimization
- Adding Comments
- Testing and Debugging
- Testing the Program
- Debugging Common Issues
- Additional Tips for Success
Programming assignments can often seem overwhelming, particularly when they involve complex and multifaceted requirements. However, by breaking down the problem into manageable tasks and following a systematic approach, you can tackle any programming assignment effectively This guide will provide you with a comprehensive framework for solving Python assignments and other programming tasks, using a generic Python assignment as an example. The steps outlined here can be applied to similar tasks, regardless of their complexity. The steps outlined here can be applied to similar tasks, regardless of their complexity.
Understanding the Assignment Requirements
The first step in solving any programming assignment is to thoroughly understand the requirements. Misinterpreting the task can lead to incorrect or incomplete solutions. Let's break down the example assignment to understand what is expected.
Breaking Down the Assignment
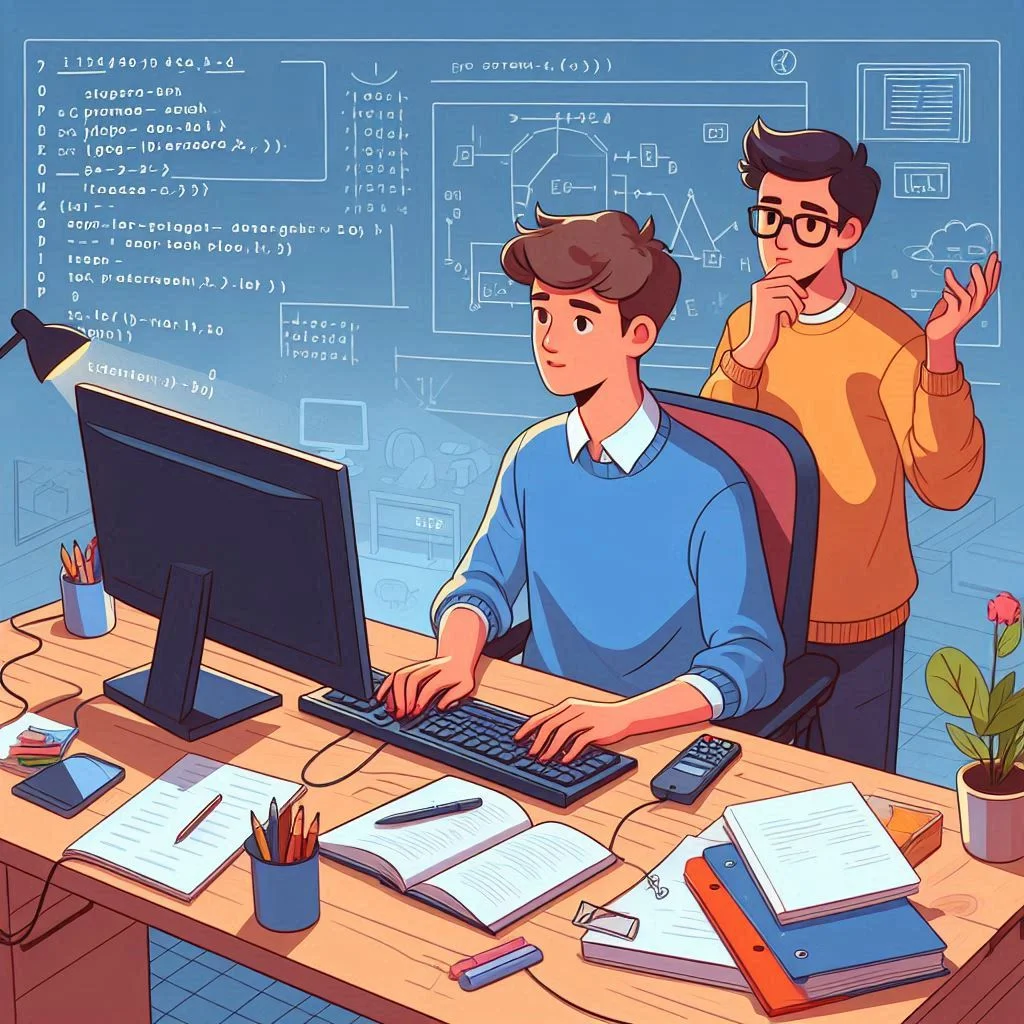
The given assignment is to create a Python program that generates a new hybrid creature based on user input. Here are the key requirements:
- User Input: The program should prompt the user to provide two creatures.
- Name Generation: Combine the names of the two creatures to generate a new hybrid creature name.
- Statistics Generation: Produce random statistics about the new creature, such as weight and diet.
- Graphic Confirmation: Display a graphic confirmation of the creature, which can be in ASCII art if GUI programming is not feasible.
- Code Optimization: Ensure that the program runs efficiently and effectively.
- Code Comments: Provide comments explaining how different sections of the code work.
Identifying Key Functionalities
To solve the assignment, the program needs to:
- Prompt for user input
- Generate a hybrid name
- Generate random statistics
- Display a graphic
- Optimize the code
- Comment the code appropriately
By identifying these key functionalities, you can create a structured plan to tackle the assignment.
Planning Your Approach
Before diving into coding, it's essential to outline a plan or algorithm for each task. This helps in understanding the flow of the program and identifying any potential challenges beforehand.
User Input
- Use input() function to get names of the two creatures from the user.
- Validate the input to ensure non-empty strings.
Name Generation
- Combine parts of the two input names to create a new hybrid name.
- Ensure the new name is unique and meaningful.
Statistics Generation
- Use the random module to generate random weight and diet type.
- Ensure the statistics are within a reasonable range.
Graphic Confirmation
- Use ASCII art to display a graphical representation of the hybrid creature.
- Alternatively, use a GUI library like tkinter for a more sophisticated graphic.
Code Optimization
- Write efficient and optimized code to ensure smooth execution.
- Avoid unnecessary computations and use appropriate data structures.
Code Comments
- Add comments to explain the logic and functionality of each section.
- Ensure comments are clear and concise.
Implementing the Solution
With a clear plan in place, you can start implementing the solution. Let's go through each part of the assignment step-by-step.
User Input
The first step is to prompt the user for input and validate it.
Prompting for User Input
def get_creatures():
creature1 = input("Enter the first creature: ")
creature2 = input("Enter the second creature: ")
return creature1, creature2
Validating User Input
def get_creatures():
while True:
creature1 = input("Enter the first creature: ").strip()
creature2 = input("Enter the second creature: ").strip()
if creature1 and creature2:
return creature1, creature2
print("Invalid input. Please enter non-empty creature names.")
Name Generation
Next, we need to generate a hybrid name from the two input names.
Combining Names
def generate_name(creature1, creature2):
name1 = creature1[:len(creature1)//2]
name2 = creature2[len(creature2)//2:]
return name1 + name2
Ensuring Unique and Meaningful Name
def generate_name(creature1, creature2):
name1 = creature1[:len(creature1)//2]
name2 = creature2[len(creature2)//2:]
hybrid_name = name1 + name2
if len(hybrid_name) < 3:
hybrid_name = name1 + "_" + name2
return hybrid_name
Statistics Generation
We now need to generate random statistics for the new creature.
Generating Random Weight and Diet
import random
def generate_statistics():
weight = random.randint(1, 2000) # Weight in pounds
diet = random.choice(["carnivorous", "herbivorous", "omnivorous"])
return weight, diet
Ensuring Reasonable Statistics
def generate_statistics():
weight = random.randint(50, 1500) # More reasonable weight range
diet = random.choice(["carnivorous", "herbivorous", "omnivorous"])
return weight, diet
Graphic Confirmation
To confirm the creation of the new creature, we can display a simple ASCII art.
Displaying ASCII Art
def display_ascii_art(creature_name):
art = f"""
, ,
(\\____/)
(_oo_)
(O)
__||__ \\)
[]/______\\[] /
/ \\______/ \\/
/ /__\\
(\\ /____\\
"""
print(f"{creature_name} has been generated!")
print(art)
Using a GUI Library
For a more sophisticated graphic, you can use tkinter.
import tkinter as tk
def display_gui(creature_name):
root = tk.Tk()
root.title(f"{creature_name} Generated")
label = tk.Label(root, text=f"{creature_name} has been generated!", font=("Arial", 20))
label.pack()
art = """
, ,
(\\____/)
(_oo_)
(O)
__||__ \\)
[]/______\\[] /
/ \\______/ \\/
/ /__\\
(\\ /____\\
"""
art_label = tk.Label(root, text=art, font=("Courier", 12))
art_label.pack()
root.mainloop()
Code Optimization
It's crucial to ensure that the code is optimized for better performance.
Writing Efficient Code
- Avoid redundant calculations.
- Use efficient data structures.
- Ensure the program runs in a reasonable time frame.
Adding Comments
Comments are essential for explaining the code logic.
Adding Clear and Concise Comments
def get_creatures():
# Prompt the user to input the names of two creatures
while True:
creature1 = input("Enter the first creature: ").strip()
creature2 = input("Enter the second creature: ").strip()
if creature1 and creature2:
return creature1, creature2
print("Invalid input. Please enter non-empty creature names.")
def generate_name(creature1, creature2):
# Combine parts of the two creature names to create a hybrid name
name1 = creature1[:len(creature1)//2]
name2 = creature2[len(creature2)//2:]
hybrid_name = name1 + name2
# Ensure the hybrid name is unique and meaningful
if len(hybrid_name) < 3:
hybrid_name = name1 + "_" + name2
return hybrid_name
def generate_statistics():
# Generate random statistics for the new creature
weight = random.randint(50, 1500) # More reasonable weight range
diet = random.choice(["carnivorous", "herbivorous", "omnivorous"])
return weight, diet
def display_ascii_art(creature_name):
# Display ASCII art to confirm the creation of the new creature
art = f"""
, ,
(\\____/)
(_oo_)
(O)
__||__ \\)
[]/______\\[] /
/ \\______/ \\/
/ /__\\
(\\ /____\\
"""
print(f"{creature_name} has been generated!")
print(art)
def main():
# Main function to execute the program
creature1, creature2 = get_creatures()
hybrid_name = generate_name(creature1, creature2)
weight, diet = generate_statistics()
print(f"Your new creature is: {hybrid_name}")
print(f"It weighs {weight} lbs and is {diet}.")
display_ascii_art(hybrid_name)
if __name__ == "__main__":
main()
Testing and Debugging
After implementing the solution, it's essential to test and debug the program to ensure it works correctly.
Testing the Program
- Run the program with various inputs to check for correctness.
- Test edge cases and unexpected inputs.
Debugging Common Issues
- Fix any syntax or runtime errors.
- Ensure the program handles invalid inputs gracefully.
Additional Tips for Success
- Practice Regularly: The more you practice, the better you will become at solving programming assignments.
- Seek Help When Needed: Don't hesitate to seek help from online forums, classmates, or professional assignment help services like ProgrammingHomeworkHelp.com.
- Stay Organized: Keep your code and files organized for easier management and debugging.
By applying these principles and techniques, you can approach your programming assignments with confidence and successfully complete them. By following a structured approach, you can tackle any programming assignment effectively.