Claim Your Offer
Unlock an amazing offer at www.programminghomeworkhelp.com with our latest promotion. Get an incredible 10% off on your all programming assignment, ensuring top-quality assistance at an affordable price. Our team of expert programmers is here to help you, making your academic journey smoother and more cost-effective. Don't miss this chance to improve your skills and save on your studies. Take advantage of our offer now and secure exceptional help for your programming assignments.
We Accept
- Understand the Problem Requirements
- Plan the Structure of Your Program
- Create the Encryption Dictionary
- Write the Function for Encryption
- Testing and User Interaction
- Additional Tips for Solving Similar Assignments
- Conclusion
When tackling programming assignments that involve creating encryption programs using Python dictionaries, it’s important to approach the problem with a structured and methodical mindset. These types of tasks not only test your ability to write functional code but also evaluate your understanding of key programming concepts and problem-solving skills. Successfully completing such assignments requires a solid grasp of Python dictionaries, as they play a crucial role in mapping data efficiently. Understanding how to define, populate, and access elements in dictionaries is essential when creating encryption schemes, especially when each character or symbol needs to be uniquely paired.
Additionally, proficiency in string manipulation techniques is crucial for handling and transforming user input into the desired encrypted format. You’ll need to know how to iterate through strings, access individual characters, and apply substitutions based on the dictionary mappings. Moreover, basic input/output operations are fundamental for interacting with the user, whether you’re prompting them for a sentence to encrypt or displaying the final output. Understanding error handling and ensuring user input is validated correctly can further enhance the program's reliability.
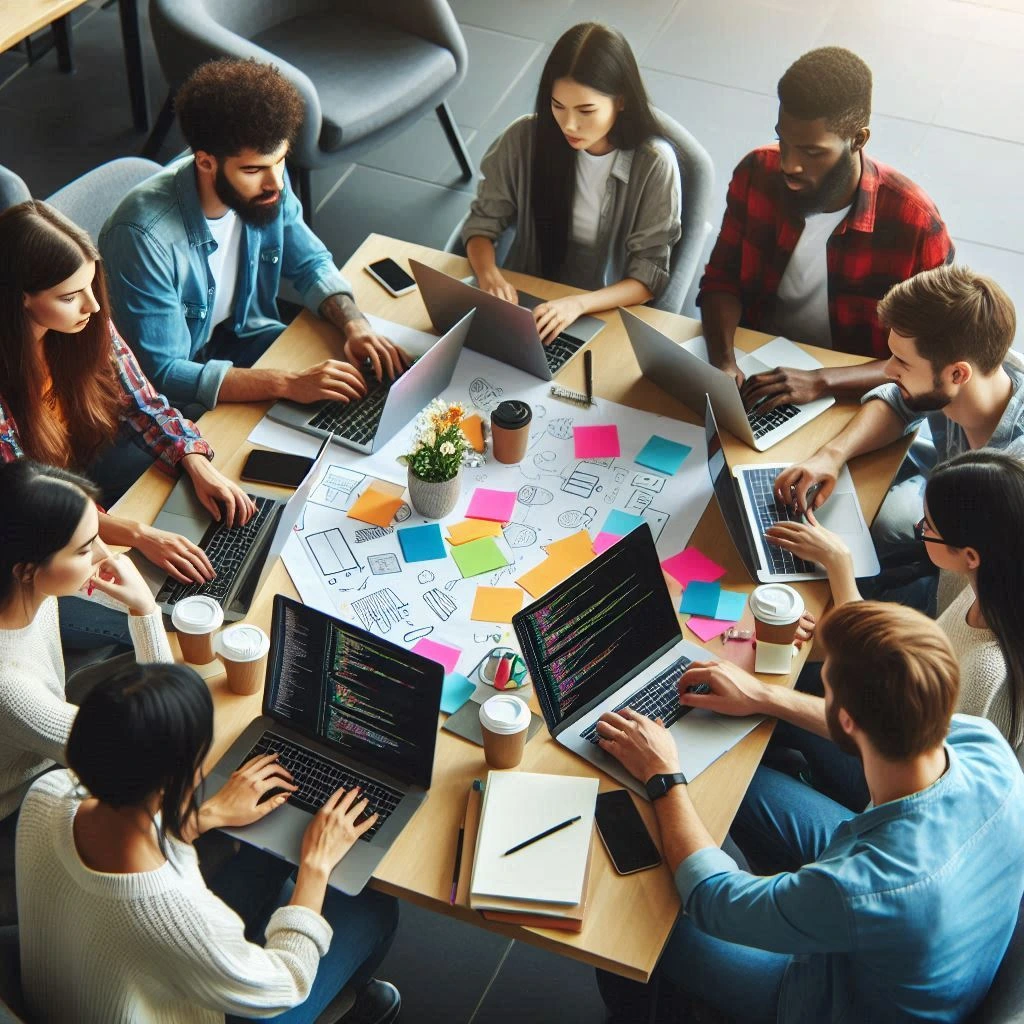
Beyond the technical coding aspects, assignments like this also test your ability to break down complex problems into manageable components. Analyzing the problem requirements, designing an efficient algorithm, and organizing the code into logical sections will help in creating a clear and maintainable program. By focusing on these areas—dictionaries, string manipulation, input/output operations, and problem decomposition—you’ll be well-prepared to tackle any encryption problem in Python or similar programming tasks with confidence and precision. If you find yourself struggling, don’t hesitate to seek assistance from a Python assignment helper to guide you through the process and enhance your understanding.
Understand the Problem Requirements
Before diving into coding, it’s crucial to ensure that you thoroughly understand the assignment and all its specifications. This particular task requires you to create a Python program that takes a user-provided sentence as input and encrypts it by replacing each letter with a predefined "code" from a dictionary. The primary goal is to transform the sentence by substituting characters based on a mapping of letters to symbols or numbers, which is predefined in the form of a Python dictionary. If you need additional support, consider taking programming assignment help which can provide guidance and clarify any confusing aspects of the assignment. This will not only help you complete your task but also deepen your understanding of the concepts involved.
Key elements to focus on include the following:
- Mapping characters with a dictionary: You’ll need to create a comprehensive dictionary that assigns specific characters to every letter in the alphabet. It’s important to account for both uppercase and lowercase letters, ensuring the dictionary distinguishes between them. This means that 'A' and 'a' should have different corresponding codes in the dictionary to ensure the encryption process is case-sensitive.
- User interaction: The program must request input from the user, prompting them to enter a sentence that will undergo the encryption process. This step should involve proper validation, making sure the input is appropriate for the encryption task.
- Processing and encryption: After receiving the user input, the program needs to process the sentence by iterating through each character. For every character in the sentence, the program will reference the predefined dictionary and replace it with its corresponding "code". If the character is not found in the dictionary (such as punctuation or spaces), you’ll need to decide whether to keep it unchanged or handle it in a different way.
- Return the encrypted sentence: The program must return the fully encrypted version of the user's sentence, reflecting the transformation dictated by the dictionary. Additionally, the encrypted output should be formatted and displayed in a clear and user-friendly manner, ensuring that the end result is easy to read and understand.
Plan the Structure of Your Program
Before you begin coding, it’s essential to carefully plan and design the structure of your program. This helps ensure that each part of the task is logically organized and easy to follow. In this encryption assignment, the program can be divided into a few core steps that guide its flow from start to finish.
Here’s how you can structure your program:
- Creating the dictionary: The first step is to set up a dictionary that maps each letter of the alphabet to a unique symbol, number, or special character. This dictionary serves as the core encryption mechanism, as it defines how each character in the input sentence will be substituted. Ensure that the dictionary includes both uppercase and lowercase letters, as Python dictionaries are case-sensitive. This way, you’ll have unique mappings for letters like 'A' and 'a'. If needed, the dictionary can be extended to include numbers or additional symbols for more complex encryption.
- User input: Once the dictionary is ready, the program should prompt the user to input a sentence that will be encrypted. This step should include clear instructions to the user, letting them know what kind of input is expected. It’s also a good idea to handle edge cases like empty input or invalid characters (depending on the rules of the encryption). Validating the input ensures the program behaves predictably and avoids potential errors.
- Processing the input: After receiving the user input, the next step is processing the sentence to apply the encryption. This can be done by writing a dedicated function that takes the input sentence and the dictionary as arguments. The function will loop through each character in the sentence and replace it with the corresponding value from the dictionary. If a character isn’t found in the dictionary (e.g., spaces or punctuation marks), it should remain unchanged. This ensures that only the letters are encrypted, while other parts of the sentence are preserved.
- Output: Finally, the program should output the encrypted sentence to the user. Once the encryption function has processed the input, the resulting sentence should be displayed in a clear and readable format. You can also include additional feedback to the user, such as printing the original sentence alongside the encrypted version for comparison. If you want to extend the program further, you could even allow the user to input multiple sentences or implement a decryption function as an optional feature.
By following this structured approach, you'll ensure that your program is organized, easy to understand, and effectively solves the task at hand. Planning ahead like this helps to minimize errors and streamline the coding process.
Create the Encryption Dictionary
In Python, a dictionary is a powerful data structure used to store key-value pairs, making it ideal for encryption tasks where each letter in the alphabet is mapped to a corresponding symbol, number, or special character. For encryption, you will need to create a dictionary where each letter of the alphabet (both uppercase and lowercase) is assigned a unique "code." For example:
codes = {'A': ')', 'a': '0', 'B': '(', 'b': '9', ...}
This dictionary should cover both uppercase and lowercase letters, as many encryption schemes are case-sensitive and the assignment specifically requires distinguishing between the two. While creating the dictionary manually is straightforward for smaller encryption schemes, if your encryption rules are more complex or involve a large dataset, you can write a function to automate the generation of these key-value pairs. This approach can save time and minimize errors, especially when working with a more dynamic or customized encryption system. Additionally, depending on the complexity of your encryption scheme, you could also include special handling for numbers or other non-alphabetic characters, making the encryption more versatile.
Write the Function for Encryption
The function responsible for processing the input and returning the encrypted string is the core of this assignment. This function takes in a sentence provided by the user, iterates through each character in that sentence, and substitutes each letter with its corresponding "code" from the dictionary. The result is an encrypted version of the sentence. It is important that the function not only handle the mapping for alphabetic characters but also correctly deal with spaces, punctuation, and other non-alphabetic characters to ensure they remain unchanged.
Here’s a simple approach for writing this function:
def encrypt_sentence(sentence, codes):
encrypted_sentence = ""
for char in sentence:
if char in codes:
encrypted_sentence += codes[char]
else:
encrypted_sentence += char # Non-alphabet characters (e.g., spaces, punctuation) remain unchanged
return encrypted_sentence
This function loops through each character in the input sentence. If the character exists in the codes dictionary, it replaces the character with its corresponding encrypted value. If the character is not found (such as spaces, punctuation, or numbers), it remains unchanged. This ensures that the encryption is applied only to the alphabetic characters, while other parts of the sentence are preserved as-is. By keeping the function flexible, you can easily modify or expand it to handle different encryption schemes or additional data types in future assignments.
Testing and User Interaction
Once you’ve written the encryption function, the next step is to integrate it into the main program, where the user can interact with it. This involves prompting the user to input a sentence, passing that sentence to the encryption function, and then displaying the encrypted result to the user. The process should be smooth and user-friendly, allowing for multiple inputs or even providing error messages if necessary.
Here's an example of how this interaction might look:
# Create the encryption dictionary
codes = {'A': ')', 'a': '0', 'B': '(', 'b': '9', ...}
# Get user input
sentence = input("Enter a sentence to encrypt: ")
# Encrypt the sentence
encrypted_sentence = encrypt_sentence(sentence, codes)
# Output the result
print(f"Encrypted Sentence: {encrypted_sentence}")
This simple interaction ensures that the program asks for user input, processes it using the encryption function, and then outputs the encrypted sentence. You can further improve user interaction by adding additional features like input validation or options to decrypt the sentence.
Additional Tips for Solving Similar Assignments
- Pay attention to edge cases: It's essential to consider edge cases when solving programming assignments. For instance, think about how your program should handle input that includes numbers, special characters, or even empty strings. While these characters may not be part of the encryption, it’s important to handle them gracefully to ensure the program runs without errors. In some cases, you may want to implement specific handling for these cases to improve the program's functionality.
- Modular code: Keeping your code modular by breaking it down into distinct functions—like dictionary creation, encryption, and input/output handling—makes your code easier to maintain, debug, and extend. If you need to modify or expand your encryption scheme later on, having a modular structure allows you to do so without affecting the entire program. This is a good practice not only for small assignments but also for larger projects.
- Testing: Thoroughly test your program with a variety of input cases to ensure it behaves as expected. Use different types of sentences that include both uppercase and lowercase letters, as well as non-alphabetic characters like numbers and punctuation marks. This will help you confirm that the encryption works correctly across different scenarios and that the program handles all possible inputs efficiently. Proper testing also ensures that the program is robust and reliable.
By following these steps, you can confidently approach and solve similar programming assignments. Whether it's encryption, data manipulation, or another type of problem, applying a structured approach will help ensure that you thoroughly understand the requirements, plan an effective solution, and execute it successfully.
Conclusion
Successfully tackling programming assignments, such as creating encryption programs in Python, requires a systematic and methodical approach. By starting with a clear understanding of the assignment’s requirements, you can ensure that you are addressing the problem correctly. Planning the structure of your program, from creating a dictionary for encryption to writing a function that handles input processing, is key to solving such tasks efficiently.
In addition to solving the current problem, focusing on best practices like modular coding ensures your solution is adaptable for future modifications or more complex assignments. Testing your code with a variety of inputs and edge cases is essential to confirm the program’s robustness and ability to handle different types of data, including numbers, punctuation, and special characters.
Moreover, approaching these assignments with a focus on clarity and simplicity not only makes the code easier to read but also reduces the chances of bugs or logic errors. This structured problem-solving approach is not limited to encryption tasks but can be applied to a broad range of programming challenges. By developing these problem-solving habits, you’ll gain the confidence to handle more advanced assignments and enhance your overall programming skills, leading to success in future coding projects.