Claim Your Discount Today
Kick off the fall semester with a 20% discount on all programming assignments at www.programminghomeworkhelp.com! Our experts are here to support your coding journey with top-quality assistance. Seize this seasonal offer to enhance your programming skills and achieve academic success. Act now and save!
We Accept
- Understanding the Problem Statement
- Breaking Down the Problem
- Planning Your Solution
- Keyword-Based Search Function
- Price-Based Search Function
- Location-Based Search Function
- Implementing the Solution
- Keyword-Based Search Implementation
- Price-Based Search Implementation
- Location-Based Search Implementation
- Testing Your Solution
- Keyword-Based Search
- Price-Based Search
- Location-Based Search
- Conclusion
Navigating complex coding tasks can often seem daunting, especially when assignments involve multiple functionalities and intricate logic. However, breaking down the problem and approaching it systematically can make the process much more manageable. This guide provides a detailed walkthrough for solving your Python assignment effectively, illustrated with examples of keyword-based, price-based, and location-based searches. By following these steps, you'll build a strong foundation for handling similar challenges in your programming journey.
Understanding the Problem Statement
The first step in tackling any programming assignment is to thoroughly understand the problem statement. Before you start coding, make sure you have a clear grasp of what the assignment is asking you to do. Let's consider a typical assignment that involves writing three functions to search for food stalls based on keywords, prices, and locations. Here are the key tasks:
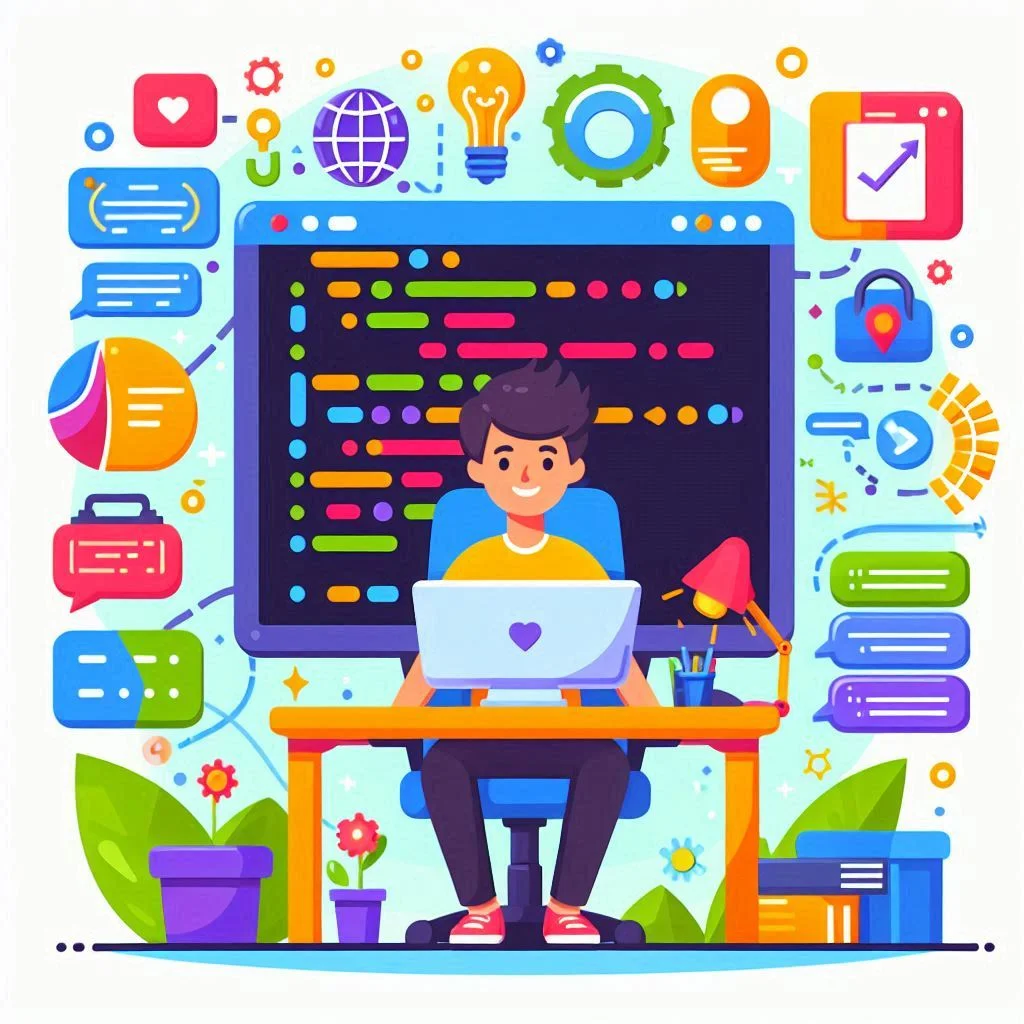
- Keyword-Based Search: Write a function that searches for food stalls based on given keywords.
- Price-Based Search: Write a function that searches for food stalls based on food prices.
- Location-Based Search: Write a function that finds the nearest canteens based on user locations.
Breaking Down the Problem
Breaking down the problem into smaller, manageable parts can make it easier to solve. Let's break down each of these tasks.
1. Keyword-Based Search
Objective: Write a function search_by_keyword(keywords) that returns food stalls serving food specified by the given keywords.
Steps:
- Load Data: Load the canteen data from an Excel file (canteens.xlsx).
- Input Parsing: Accept multiple keywords separated by spaces and make the search case-insensitive.
- Search Logic: Iterate through the food stalls and check if they contain all provided keywords.
- Output: Print the number of matches and the corresponding canteen and food stall names.
- Error Handling: Handle cases where no input is provided or no matches are found.
2. Price-Based Search
Objective: Write a function search_by_price(keywords) to find food stalls offering food that matches given keywords and sort them by price.
Steps:
- Load Data: Load the canteen data from an Excel file (canteens.xlsx).
- Input Parsing: Accept multiple keywords separated by spaces and make the search case-insensitive.
- Search Logic: Iterate through the food stalls to find matches and store them along with their prices.
- Sorting: Sort the results by price in ascending order.
- Output: Print the number of matches and the corresponding canteen, food stall names, and prices.
- Error Handling: Handle cases where no input is provided or no matches are found.
3. Location-Based Search
Objective: Write a function search_nearest_canteens(user_locations, k) to find the k nearest canteens to two user locations.
Steps:
- Input Parsing: Accept two user locations and the number of nearest canteens to find (default k=1).
- Load Data: Load the canteen data from an Excel file (canteens.xlsx).
- Distance Calculation: Use the Euclidean distance formula to calculate the distance between user locations and canteens.
- Sorting: Sort the canteens by their distance from the users.
- Output: Print the k nearest canteens and their distances.
- Error Handling: Handle cases where k is negative or no canteens are found.
Planning Your Solution
Planning your solution involves deciding on the data structures, algorithms, and functions you will use. For each task, define the function prototypes and outline the logic.
Keyword-Based Search Function
def search_by_keyword(keywords):
# Load the data from canteens.xlsx
# Parse the keywords
# Search for matching food stalls
# Handle errors and edge cases
# Print the results
Price-Based Search Function
def search_by_price(keywords):
# Load the data from canteens.xlsx
# Parse the keywords
# Search for matching food stalls
# Sort the results by price
# Handle errors and edge cases
# Print the results
Location-Based Search Function
def search_nearest_canteens(user_locations, k=1):
# Get user locations using get_user_location_interface()
# Calculate Euclidean distance
# Sort canteens by distance
# Handle errors and edge cases
# Print the results
Implementing the Solution
Now that you have a plan, start implementing your solution step by step. Focus on one function at a time and test it thoroughly before moving on to the next.
Keyword-Based Search Implementation
Let's start with the keyword-based search function. This function will load the data from an Excel file, parse the keywords, search for matching food stalls, and print the results.
import pandas as pd
def search_by_keyword(keywords):
# Load the data
try:
data = pd.read_excel('canteens.xlsx')
except FileNotFoundError:
print("Error: Data file not found.")
return
if not keywords.strip():
print("Error: No input found. Please try again.")
return
keyword_list = keywords.lower().split()
results = []
for index, row in data.iterrows():
food_stall = row['Food Stall'].lower()
if all(keyword in food_stall for keyword in keyword_list):
results.append((row['Canteen'], row['Food Stall']))
if results:
print(f"Food Stalls found: {len(results)}")
for canteen, stall in results:
print(f"{canteen} – {stall}")
else:
print("Food Stalls found: No food stall found with input keyword.")
Price-Based Search Implementation
Next, we'll implement the price-based search function. This function will load the data, parse the keywords, search for matching food stalls, sort them by price, and print the results.
def search_by_price(keywords):
# Load the data
try:
data = pd.read_excel('canteens.xlsx')
except FileNotFoundError:
print("Error: Data file not found.")
return
if not keywords.strip():
print("Error: No input found. Please try again.")
return
keyword_list = keywords.lower().split()
results = []
for index, row in data.iterrows():
food_stall = row['Food Stall'].lower()
if all(keyword in food_stall for keyword in keyword_list):
results.append((row['Canteen'], row['Food Stall'], row['Price']))
if results:
results.sort(key=lambda x: x[2]) # Sort by price
print(f"Food Stalls found: {len(results)}")
for canteen, stall, price in results:
print(f"{canteen} – {stall} – ${price:.2f}")
else:
print("Food Stalls found: No food stall found with input keyword.")
Location-Based Search Implementation
Finally, let's implement the location-based search function. This function will calculate the distance between user locations and canteens, sort the canteens by distance, and print the nearest canteens.
import math
def search_nearest_canteens(user_locations, k=1):
def euclidean_distance(loc1, loc2):
return math.sqrt((loc1[0] - loc2[0])**2 + (loc1[1] - loc2[1])**2)
try:
data = pd.read_excel('canteens.xlsx')
except FileNotFoundError:
print("Error: Data file not found.")
return
if k < 1:
k = 1
user1, user2 = user_locations
results = []
for index, row in data.iterrows():
canteen_loc = (row['Location X'], row['Location Y'])
dist = (euclidean_distance(user1, canteen_loc) + euclidean_distance(user2, canteen_loc)) / 2
results.append((row['Canteen'], dist))
results.sort(key=lambda x: x[1]) # Sort by distance
print(f"Nearest {k} canteens:")
for i in range(min(k, len(results))):
print(f"{results[i][0]} – {results[i][1]:.2f} units")
Testing Your Solution
Testing is a crucial part of the development process. Test each function with various inputs, including edge cases, to ensure they work as expected. Here are some example test cases:
Keyword-Based Search
Test Case 1: keywords = "Western"
- Expected Output: List of food stalls serving Western food.
Test Case 2: keywords = ""
- Expected Output: Error message for no input.
Test Case 3: keywords = "Nonexistent"
- Expected Output: No matches found.
Price-Based Search
Test Case 1: keywords = "Chicken"
- Expected Output: List of food stalls serving chicken dishes, sorted by price.
Test Case 2: keywords = ""
- Expected Output: Error message for no input.
Test Case 3: keywords = "Nonexistent"
- Expected Output: No matches found.
Location-Based Search
Test Case 1: user_locations = [(0,0), (10,10)], k = 3
- Expected Output: Nearest 3 canteens and their distances.
Test Case 2: user_locations = [(0,0), (10,10)], k = -1
- Expected Output: Nearest canteen (default k=1).
Test Case 3: user_locations = [(0,0), (0,0)], k = 3
- Expected Output: Nearest 3 canteens.
Conclusion
Solving programming assignments effectively requires a clear understanding of the problem, breaking it down into manageable parts, planning your solution, implementing it step by step, and thoroughly testing your code. By following this structured approach, you can tackle even the most complex assignments with confidence.
In this guide, we walked through the process of solving three typical programming tasks: keyword-based search, price-based search, and location-based search. By applying these principles to similar assignments, you'll be able to develop robust solutions and enhance your programming skills.
Remember, practice is key to mastering programming. Keep challenging yourself with new assignments, experiment with different approaches, and don't hesitate to seek help when needed.