Claim Your Discount Today
Kick off the fall semester with a 20% discount on all programming assignments at www.programminghomeworkhelp.com! Our experts are here to support your coding journey with top-quality assistance. Seize this seasonal offer to enhance your programming skills and achieve academic success. Act now and save!
We Accept
- Key Concepts of Multithreading
- Languages Supporting Multithreading
- Role of Compilers in Multithreading
- Approaching Multithreaded Programming Problems
- Problem: Numerical Integration Using Simpson's Rule
- Haskell Implementation
- Conclusion
Multithreaded parallelism is a powerful technique in modern programming that allows multiple threads to execute concurrently, improving the performance and efficiency of software applications. This approach is particularly valuable when dealing with computationally intensive tasks or applications that require real-time responsiveness. Understanding the concepts of multithreading, the languages that support it, and the role of compilers can help students tackle Multithreaded Parallelism assignments involving parallel programming.
Key Concepts of Multithreading
- Threads: A thread is the smallest unit of execution within a process. Multiple threads within the same process share the same memory space but can execute independently, allowing for concurrent operations.
- Parallelism vs. Concurrency: While concurrency involves multiple tasks making progress, parallelism involves multiple tasks running simultaneously. Multithreading primarily deals with parallelism.
- Synchronization: Managing access to shared resources is crucial in multithreaded applications to prevent race conditions and ensure data consistency. Techniques like mutexes, semaphores, and locks are used for synchronization.
- Deadlock: A situation where two or more threads are blocked forever, waiting for each other. Avoiding deadlock is an essential aspect of multithreaded programming.
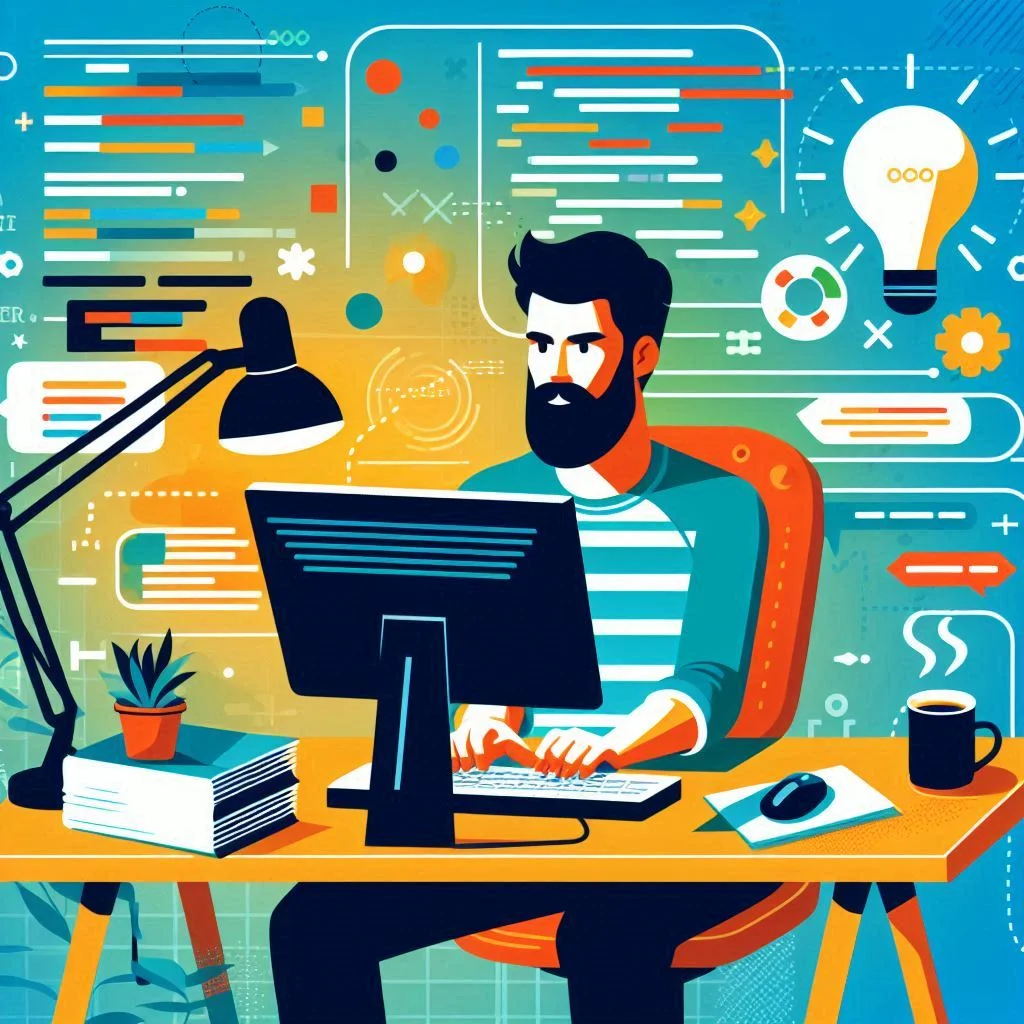
Languages Supporting Multithreading
Several programming languages offer robust support for multithreading. Here are a few notable ones:
- Java: Java provides built-in support for multithreading through the `java.lang.Thread` class and the `java.util.concurrent` package, which offers higher-level concurrency utilities.
- C++: The C++ Standard Library includes the `<thread>` header, which provides thread management capabilities. Additionally, the Boost library offers advanced multithreading features.
- Python: Python's `threading` module allows for multithreaded programming, though the Global Interpreter Lock (GIL) can limit true parallelism in CPU-bound tasks. The `multiprocessing` module is often used as an alternative.
- Haskell: As a functional programming language, Haskell supports parallelism and concurrency through libraries like `Control.Concurrent` and parallel strategies provided by the Glasgow Haskell Compiler (GHC).
Role of Compilers in Multithreading
Compilers play a crucial role in optimizing and managing multithreaded programs. They are responsible for translating high-level code into machine instructions that efficiently utilize multiple cores and processors. Some key aspects of how compilers assist in multithreading include:
- Optimization: Compilers optimize code to minimize overhead and maximize parallel execution. This includes loop unrolling, inlining functions, and optimizing memory access patterns.
- Thread Management: Compilers generate code that efficiently manages thread creation, synchronization, and communication. This involves reducing context-switching overhead and ensuring proper cache usage.
- Parallel Libraries and APIs: Many compilers provide libraries and APIs that simplify multithreaded programming. For instance, GHC for Haskell includes support for Software Transactional Memory (STM) and parallel strategies.
Approaching Multithreaded Programming Problems
When tackling problems that involve multithreaded programming, consider the following steps:
- Understand the Problem: Carefully read the problem statement to identify the tasks that can be parallelized. Determine the dependencies and shared resources.
- Choose the Right Language and Tools: Select a programming language that offers good support for multithreading and is well-suited for the problem at hand. Use appropriate libraries and tools to simplify development.
- Design the Solution: Break down the problem into smaller tasks that can be executed concurrently. Design the thread management and synchronization mechanisms to avoid race conditions and deadlocks.
- Implement and Test: Write the code, focusing on thread creation, synchronization, and efficient use of resources. Thoroughly test the program to ensure correctness and performance.
- Analyze and Optimize: Use profiling tools to identify bottlenecks and optimize the code for better performance. Ensure that the solution scales well with increasing numbers of threads.
Example: Numerical Integration in Haskell
Let's consider a simplified example related to numerical integration in Haskell, highlighting how multithreaded parallelism can be applied.
Problem: Numerical Integration Using Simpson's Rule
Given a function \( f \) and an interval \([a, b]\), Simpson's rule approximates the integral as follows:
\[ I = \frac{h}{3} [f(a) + 4f(a + h) + f(a + 2h)] \]
where \( h = \frac{b - a}{2} \).
To improve accuracy, the interval \([a, b]\) is divided into smaller subintervals, and Simpson's rule is applied to each subinterval. This can be parallelized to take advantage of multithreading.
Haskell Implementation
Here is a basic Haskell implementation using parallel strategies:
```haskell
import Control.Parallel.Strategies
Simpson's rule for a single interval
simpson :: (Double -> Double) -> Double -> Double -> Double
simpson f a b = (h / 3) * (f a + 4 * f (a + h) + f (a + 2 * h))
where h = (b - a) / 2
Composite Simpson's rule for multiple intervals
compositeSimpson :: (Double -> Double) -> Double -> Double -> Int -> Double
compositeSimpson f a b n = sum $ parMap rdeepseq (uncurry (simpson f)) intervals
where
h = (b - a) / fromIntegral n
intervals = [(a + i * h, a + (i + 1) * h) | i <- [0..n-1]]
main :: IO ()
main = do
let result = compositeSimpson sin 0 pi 1000000
print result
```
In this example, the `parMap rdeepseq` function from the `Control.Parallel.Strategies` library is used to parallelize the computation of Simpson's rule over multiple intervals. This allows the program to utilize multiple CPU cores, improving performance.
Conclusion
Multithreaded parallelism is a vital concept in modern programming, offering significant performance benefits for various applications. By understanding the basics of multithreading, choosing appropriate languages and tools, and effectively utilizing compilers, students can efficiently tackle assignments involving parallel programming. The example of numerical integration in Haskell demonstrates how parallelism can be applied to a computational problem, providing a foundation for solving similar assignments. When you need to solve your programming assignment, understanding these concepts can greatly enhance your ability to deliver efficient and effective solutions.