Claim Your Offer
New semester, new challenges—but don’t stress, we’ve got your back! Get expert programming assignment help and breeze through Python, Java, C++, and more with ease. For a limited time, enjoy 10% OFF on all programming assignments with the Spring Special Discount! Just use code SPRING10OFF at checkout! Why stress over deadlines when you can score high effortlessly? Grab this exclusive offer now and make this semester your best one yet!
We Accept
- Understanding Order Statistics and the Problem Scope
- Order Statistics
- Efficiently Finding Order Statistics: The Quickselect Algorithm
- Overview of Quickselect
- Quickselect Algorithm Implementation in Java
- Step 1: The Main Class and Method
- Explanation:
- Iterative Version of Quickselect
- Testing and Debugging
- Key Points to Test:
- Practical Considerations and Optimization
- Conclusion
Order statistics are a fundamental concept in computer science and statistics, involving the identification of specific elements within a dataset based on their position when the data is sorted. For example, finding the median, the minimum, or the k-th smallest element in a dataset are common problems in this domain. These operations are essential for various applications, including data analysis, machine learning, and more. Traditional approaches might involve sorting the entire dataset, but this can be computationally expensive, especially for large datasets. Instead, advanced algorithms like Quickselect can find these elements efficiently without fully sorting the data.
In this blog, we'll delve into advanced java programming assignments, such as finding order statistics like the median or kth smallest element in an array, it's essential to understand the underlying algorithms and strategies. This guide will help you approach similar programming assignments with confidence, using efficient techniques without the need to sort the entire array.
Understanding Order Statistics and the Problem Scope
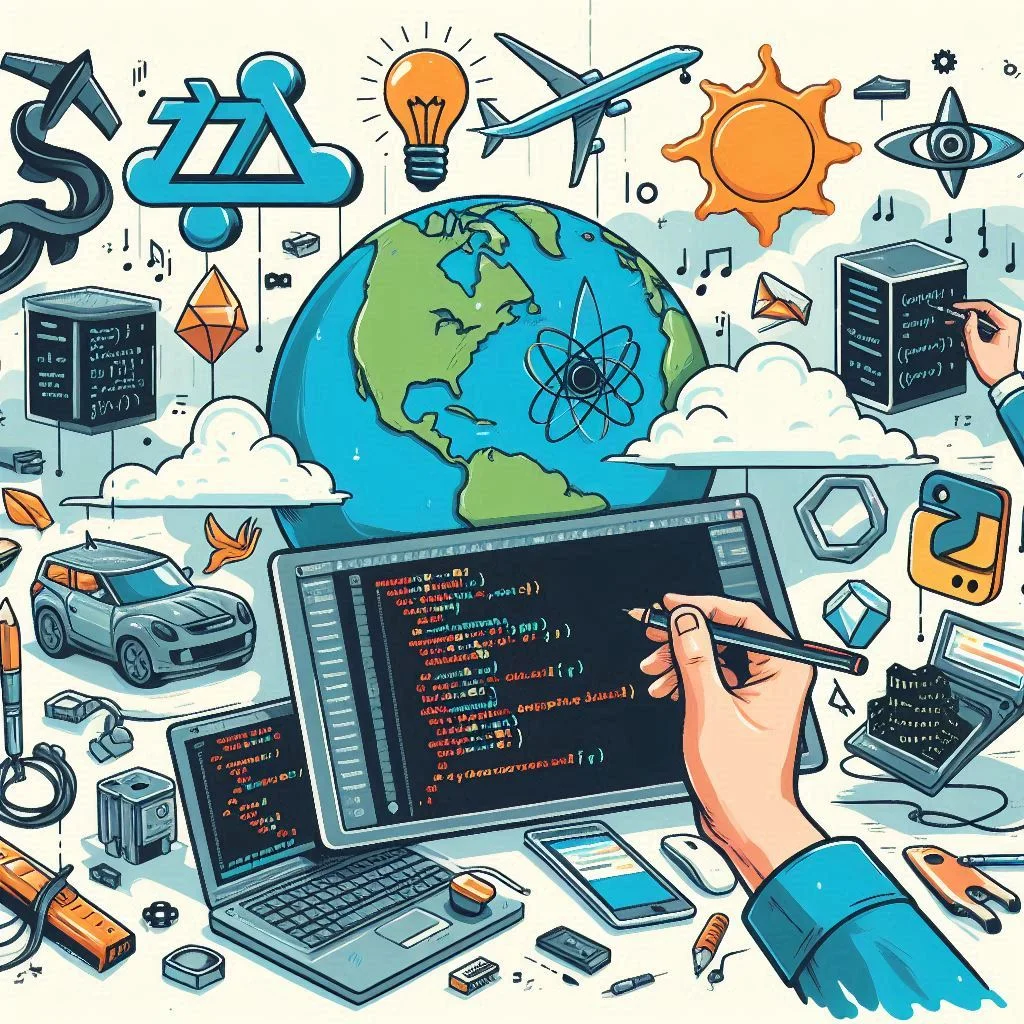
Order statistics refer to the elements of a dataset arranged in ascending or descending order. Specifically, the k-th order statistic is the k-th smallest element in a set. For example, in the array [3, 1, 4, 1, 5, 9, 2], the 3rd order statistic is 2, as it is the third smallest element when the array is sorted.
Finding the k-th smallest or largest element is often necessary in various applications:
- Data Analysis: Median calculation, percentile extraction, and outlier detection.
- Algorithm Design: In algorithms like Median of Medians, which selects a good pivot for Quick Sort, and in selection problems.
- Competitive Programming: Problems that involve finding specific elements under constraints.
The naive approach to find the k-th order statistic involves sorting the array and then accessing the element at the k-th index. However, this approach has a time complexity of O(n log n), where n is the number of elements in the array. For large datasets, this is inefficient, leading to the exploration of more advanced algorithms like Quickselect.
Order Statistics
Order statistics refer to the elements of a dataset sorted by their values. For a dataset with n elements, the k-th order statistic is the k-th smallest element in the sorted order. Key order statistics include:
- Minimum (1st order statistic): The smallest element in the dataset.
- Maximum (n-th order statistic): The largest element in the dataset.
- Median: The middle element(s) in the dataset. For an odd number of elements, it is the (n/2+1)(n/2 + 1)(n/2+1)-th smallest element; for even, it is the average of the n/2n/2n/2-th and (n/2+1)(n/2 + 1)(n/2+1)-th elements.
Order statistics are crucial in various applications, such as:
- Data analysis: Summarizing datasets, such as finding the median in a dataset to understand central tendencies.
- Decision-making systems: In real-time systems where quick decisions are needed based on certain quantiles of data.
- Statistical algorithms: Many algorithms require the identification of specific percentiles, such as the 95th percentile in statistical quality control.
Efficiently Finding Order Statistics: The Quickselect Algorithm
Sorting an entire dataset to find an order statistic can be inefficient, with a time complexity of O(nlogn)O(n \log n)O(nlogn). However, it is often unnecessary to sort the whole array to find a specific element's position. The Quickselect algorithm, an adaptation of the QuickSort algorithm, provides a more efficient solution.
Overview of Quickselect
Quickselect finds the k-th smallest element in an unordered list in O(n)O(n)O(n) expected time, using a divide-and-conquer approach. Here's a high-level breakdown:
- Partitioning: Like QuickSort, Quickselect uses a pivot to partition the array into two parts: elements less than the pivot and elements greater than the pivot.
- Selection: Depending on the pivot's position, the algorithm either finds the desired element in the left or right partition or concludes if the pivot itself is the k-th smallest element.
This method avoids the complete sorting of the array and focuses only on the necessary portion, reducing the average case time complexity.
Quickselect Algorithm Implementation in Java
Let's implement the Quickselect algorithm in Java, focusing on finding the k-th smallest element.
Step 1: The Main Class and Method
We begin by creating a class, SortArray, containing methods for our sorting and selection tasks.
public class SortArray {
public int kthItem(int[] arr, int k) {
return quickSelect(arr, 0, arr.length - 1, k - 1); // k-1 for zero-based index
}
private int quickSelect(int[] arr, int low, int high, int k) {
if (low == high) {
return arr[low];
}
int pivotIndex = partition(arr, low, high);
if (k == pivotIndex) {
return arr[k];
} else if (k < pivotIndex) {
return quickSelect(arr, low, pivotIndex - 1, k);
} else {
return quickSelect(arr, pivotIndex + 1, high, k);
}
}
private int partition(int[] arr, int low, int high) {
int pivot = arr[high];
int i = low;
for (int j = low; j < high; j++) {
if (arr[j] <= pivot) {
swap(arr, i, j);
i++;
}
}
swap(arr, i, high);
return i;
}
private void swap(int[] arr, int i, int j) {
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
Explanation:
- kthItem(int[] arr, int k): This public method is the entry point for finding the k-th smallest element. It adjusts for zero-based indexing and calls the quickSelect method.
- quickSelect(int[] arr, int low, int high, int k): This is the core recursive method. It handles the base case (when the list has one element) and the recursive partitioning logic.
- partition(int[] arr, int low, int high): This method partitions the array using the last element as the pivot. It rearranges the array so that all elements less than the pivot are on the left, and all greater elements are on the right.
- swap(int[] arr, int i, int j): A utility method to swap two elements in the array.
This implementation finds the k-th smallest element in the array with an expected time complexity of O(n)O(n)O(n).
Iterative Version of Quickselect
In some cases, recursion might not be ideal, especially with large datasets that could lead to stack overflow. An iterative version of the Quickselect algorithm can mitigate this issue.
public class SortArray {
public int kthItemIterative(int[] arr, int k) {
int low = 0, high = arr.length - 1;
k = k - 1; // Convert to zero-based index
while (low <= high) {
int pivotIndex = partition(arr, low, high);
if (pivotIndex == k) {
return arr[pivotIndex];
} else if (pivotIndex < k) {
low = pivotIndex + 1;
} else {
high = pivotIndex - 1;
}
}
return -1; // This line should not be reached
}
private int partition(int[] arr, int low, int high) {
int pivot = arr[high];
int i = low;
for (int j = low; j < high; j++) {
if (arr[j] <= pivot) {
swap(arr, i, j);
i++;
}
}
swap(arr, i, high);
return i;
}
private void swap(int[] arr, int i, int j) {
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
This iterative approach replaces the recursive calls with a loop, continuously partitioning the array until the k-th smallest element is found.
Testing and Debugging
To ensure the correctness of the Quickselect implementation, thorough testing is necessary. This involves testing with various array sizes and different values of k, as well as considering edge cases.
Test Example:
Java code
public class CheckKth {
public static void main(String[] args) {
SortArray sorter = new SortArray();
int[] array = {12, 3, 5, 7, 4, 19, 26};
int k = 3;
System.out.println("Kth smallest element is " + sorter.kthItem(array, k));
System.out.println("Kth smallest element (iterative) is " + sorter.kthItemIterative(array, k));
}
}
Key Points to Test:
- Small Arrays: Start with small arrays to verify basic functionality.
- Large Arrays: Test with large datasets to ensure the algorithm's efficiency and stability.
- Edge Cases: Include edge cases like arrays with duplicate values, single-element arrays, and when k equals the length of the array.
Practical Considerations and Optimization
While Quickselect is efficient, certain considerations and optimizations can further enhance its performance and reliability:
- Pivot Selection: The choice of pivot can significantly affect performance. Using random pivot selection or median-of-medians can improve worst-case scenarios.
- Handling Duplicates: Special care is needed when handling arrays with many duplicate values, as this can skew the partitioning process.
- Memory Usage: The iterative version of Quickselect can help manage memory usage by avoiding deep recursive calls.
- Stability and Precision: Ensure the implementation can handle various data types and maintains precision, especially with floating-point numbers.
Conclusion
Understanding and implementing algorithms for finding order statistics, such as Quickselect, is a valuable skill in both academic and professional settings. These techniques provide efficient solutions to problems that would otherwise require more resource-intensive approaches, such as full sorting. By focusing on the core principles and understanding the nuances of the algorithms, you can efficiently tackle a wide range of problems involving large datasets and complex data analysis tasks.
For students and professionals alike, mastering these algorithms for finding order statistics is a valuable skill in both academic and professional settings. By understanding the principles behind these algorithms and practicing their implementation, you'll be well-equipped to handle similar data structure assignments. Remember, the key is to focus on understanding the problem deeply, plan your approach, and rigorously test your solution.