Claim Your Offer
Unlock an amazing offer at www.programminghomeworkhelp.com with our latest promotion. Get an incredible 10% off on your all programming assignment, ensuring top-quality assistance at an affordable price. Our team of expert programmers is here to help you, making your academic journey smoother and more cost-effective. Don't miss this chance to improve your skills and save on your studies. Take advantage of our offer now and secure exceptional help for your programming assignments.
We Accept
Algorithmic problems often involve intricate challenges that require a deep understanding of both theoretical concepts and practical implementation. Whether you are dealing with advanced algorithms in cryptography, graph theory, or secure multiparty computation, the core principles of problem-solving remain consistent. This blog post aims to provide a structured approach to tackling complex algorithmic assignments, offering insights into how to break down, understand, and solve your cryptography assignment or any other challenging problems effectively.
To illustrate these principles, we will use two example problems that exemplify the kind of assignments commonly encountered in advanced programming courses. The first problem involves Online Bipartite Matching, a critical concept in graph theory with applications in scheduling and resource allocation. The second problem focuses on Secure Multiparty Computation, a crucial area in cryptography that deals with how multiple parties can jointly compute a function over their inputs while keeping those inputs private. To fully understand these concepts and complete your data structures assignment, it's essential to explore these problems in detail and practice their implementations.
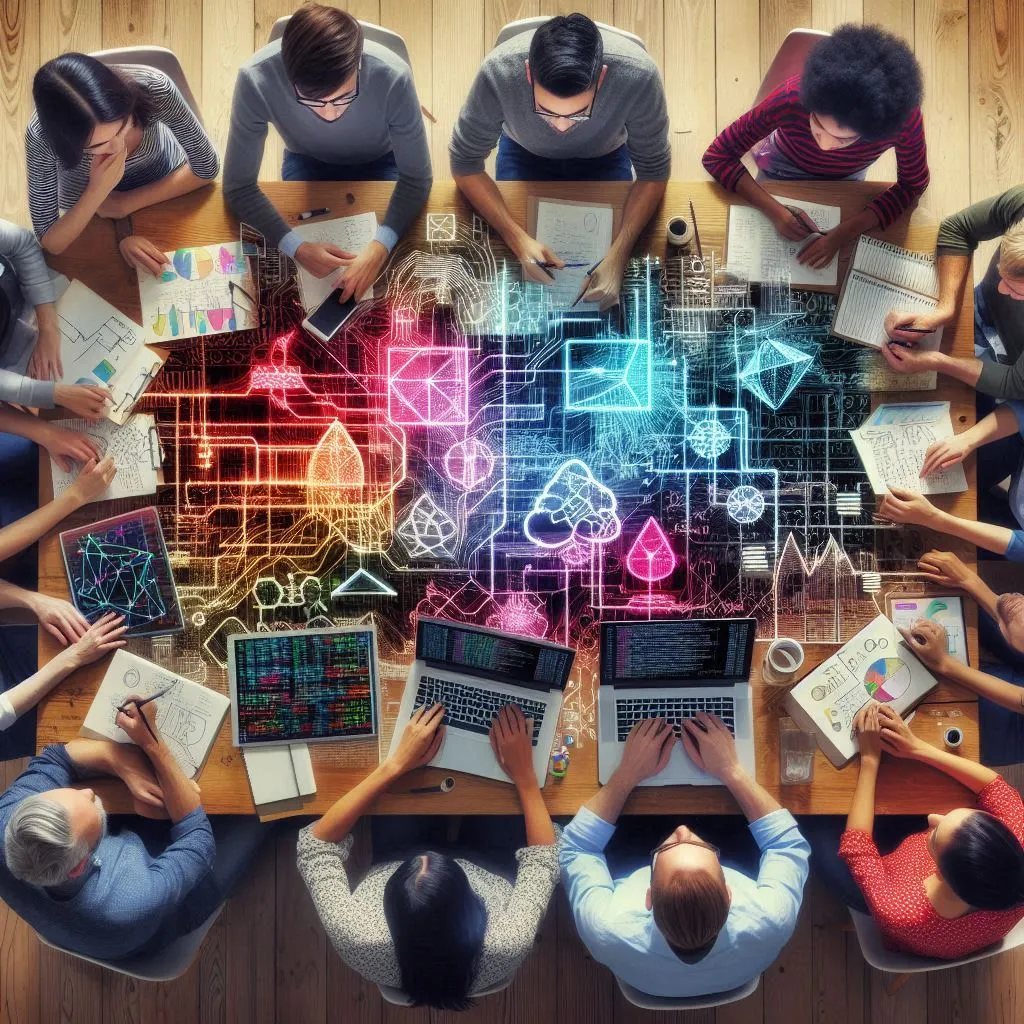
These problems not only require a solid grasp of algorithms but also demand a rigorous approach to proving correctness and analyzing runtime efficiency. By exploring these examples in detail, we will uncover the steps necessary to approach and solve similar assignments, equipping you with the skills to solve your programming assignment and tackle any complex algorithmic challenge.
Problem 1: Online Bipartite Matching
Online Bipartite Matching is a classic problem in computer science and combinatorial optimization, particularly relevant in scenarios where decisions must be made without complete information about future inputs. It involves a bipartite graph, which is a graph divided into two disjoint sets of vertices, U and V, such that every edge connects a vertex in U to a vertex in V.
In the online variant of this problem, the vertices of set V arrive one by one, and we must decide immediately how to match them to vertices in U without knowledge of future vertices in V. The goal is to maximize the size of the matching, which is a set of edges that do not share any vertices.
Steps to Solve Online Bipartite Matching
1. Problem Description:
- Given two sets U and V, with edges only between U and V.
- Vertices in V appear one at a time.
- Match each incoming vertex in V to an unmatched vertex in U or leave it unmatched.
2. Algorithm Design:
- A common strategy is the Greedy Algorithm: As each vertex v∈Vv arrives, match it to any unmatched vertex u∈Uu it is connected to, if possible.
3. Algorithm in Pseudo Code:
for each vertex v in V arriving online:
for each vertex u in U:
if (u, v) is an edge and u is not matched:
match u and v
break
4. Correctness and Runtime Analysis:
- Correctness: This algorithm guarantees a valid matching because it only matches unmatched vertices.
- Runtime: The worst-case runtime is O(∣U∣×∣V∣), as each vertex in V may be checked against all vertices in U.
5. Python Code Implementation:
def online_bipartite_matching(U, V, edges):
matched = {u: False for u in U}
matching = []
for v in V:
for u in U:
if (u, v) in edges and not matched[u]:
matched[u] = True
matching.append((u, v))
break
return matching
# Example Usage
U = ['u1', 'u2', 'u3']
V = ['v1', 'v2', 'v3']
edges = [('u1', 'v1'), ('u2', 'v2'), ('u1', 'v3')]
print(online_bipartite_matching(U, V, edges)) # Output could be [('u1', 'v1'), ('u2', 'v2')]
In this implementation, we use a dictionary matched to keep track of whether a vertex in U is already matched, ensuring that each vertex in U is matched at most once.
Example and Explanation
Consider a bipartite graph where:
- U={u1,u2,u3}
- V={v1,v2,v3}
- Edges are: (u1,v1),(u2,v2),(u1,v3
As v1 arrives, it matches with u1. When v2 arrives, it matches with u2. When v3 arrives, u1u1u1 is already matched, so v3 remains unmatched.
Problem 2: Secure Multiparty Computation
Secure multiparty computation (MPC) is a cryptographic protocol that enables multiple parties to jointly compute a function over their inputs while keeping those inputs private.
Understanding the Example
The problem discusses a scenario where multiple parties compute a function securely. The example involves polynomial computations and secure sharing among parties. We’ll break this down step by step.
1. Problem Description:
- Multiple parties have secret inputs.
- They want to compute a function (like a sum or product) over their inputs without revealing them to each other.
- Use of polynomials and secure sharing to maintain privacy.
2. Detailed Example Explanation:
- Each party picks a random polynomial and evaluates it at different points.
- These evaluations are shared securely among the parties.
3. Algorithm Steps:
- Each party chooses a random polynomial of a specific degree.
- Evaluate this polynomial at several points.
- Securely share these evaluations with all parties.
4. Polynomial Sharing and Reconstruction:
- For example, if we use a polynomial f(x)=a+bx+cx^2, each party computes f(1),f(2),…,f(n) where n is the number of parties.
- After sharing, the original polynomial can be reconstructed using Lagrange interpolation, ensuring the computation remains private.
5. Python Code Implementation:
Here, we'll simulate a simple secure sharing and reconstruction with polynomials.
import random
def generate_random_polynomial(degree):
return [random.randint(0, 100) for _ in range(degree + 1)]
def evaluate_polynomial(coeffs, x):
result = 0
for power, coeff in enumerate(coeffs):
result += coeff * (x ** power)
return result
def share_polynomial(coeffs, num_parties):
shares = []
for i in range(1, num_parties + 1):
shares.append((i, evaluate_polynomial(coeffs, i)))
return shares
def reconstruct_polynomial(shares):
# Using Lagrange interpolation for simplicity
# Note: This code assumes the existence of a library or function to perform the interpolation
from sympy import interpolate
return interpolate(shares)
# Example Usage
degree = 2
num_parties = 6
coeffs = generate_random_polynomial(degree)
print("Polynomial Coefficients:", coeffs)
shares = share_polynomial(coeffs, num_parties)
print("Shares:", shares)
reconstructed = reconstruct_polynomial(shares)
print("Reconstructed Polynomial:", reconstructed)
6. Correctness Proof:
- Correctness: The use of random polynomials ensures that individual shares do not reveal any information about the secret. Reconstruction via Lagrange interpolation ensures correctness.
- Security: The polynomial sharing is secure because knowing fewer than t+1 shares (where t is the polynomial degree) reveals nothing about the polynomial.
7. Runtime Analysis:
- Polynomial Evaluation: Evaluating a polynomial of degree d takes O(d) time.
- Sharing: For n parties, this operation is O(n×d).
- Reconstruction: Assuming an efficient interpolation algorithm, this step is O(n^2).
Detailed Example Walkthrough
Given parties P1,P2,…,P6 and a polynomial f(x) = 7 + 41x + 44x^2:
- Each party computes evaluations like f(1),f(2),…,f(6).
- Shares are distributed.
- Using shares, reconstruct the original polynomial without revealing individual inputs.
Conclusion
Both Online Bipartite Matching and Secure Multiparty Computation illustrate the importance of a methodical approach to complex algorithmic challenges. By carefully dissecting the problem, designing efficient algorithms, and rigorously analyzing their correctness and performance, you can develop a deeper understanding of advanced computational concepts. These examples also underscore the value of applying theoretical knowledge in practical scenarios, fostering a more intuitive grasp of how to approach and navigate similar algorithmic problems. Developing these skills not only enhances your problem-solving capabilities but also prepares you to tackle a broad spectrum of challenges in the field of computer science. If you need assistance with Python assignment related to these topics, it's essential to apply these concepts methodically to effectively solve the problems at hand.