Claim Your Discount Today
Ring in Christmas and New Year with a special treat from www.programminghomeworkhelp.com! Get 15% off on all programming assignments when you use the code PHHCNY15 for expert assistance. Don’t miss this festive offer—available for a limited time. Start your New Year with academic success and savings. Act now and save!
We Accept
- Understanding the Problem
- Scenario Overview
- Key Objectives
- Mathematical Modeling
- 1. Speculating about Civilizations
- 2. Detecting Exoplanets
- Key Calculations
- Advanced Section
- Python Program Implementation
- Explanation and Analysis
- Conclusion
The exploration of exoplanets—planets orbiting stars beyond our solar system—is a cutting-edge field in astronomy. In this assignment, we are tasked with creating an interactive Python program for a museum exhibit called "Exploring Our Galaxy." The exhibit focuses on exoplanets, which are planets orbiting distant stars. The goal is to engage museum patrons by allowing them to speculate about extraterrestrial civilizations and understand the detection of exoplanets. This guide will walk through the process to solve python assignment, including mathematical modeling, Python programming, and effective communication strategies for different audiences.
Understanding the Problem
Scenario Overview
The science museum in St Lucia is updating its exhibit to captivate patrons with the topic of exoplanets. Each exhibit item needs to be accompanied by two explanations: one for "science rookies" and another for "science enthusiasts." The task is to develop an interactive Python program that achieves the following:
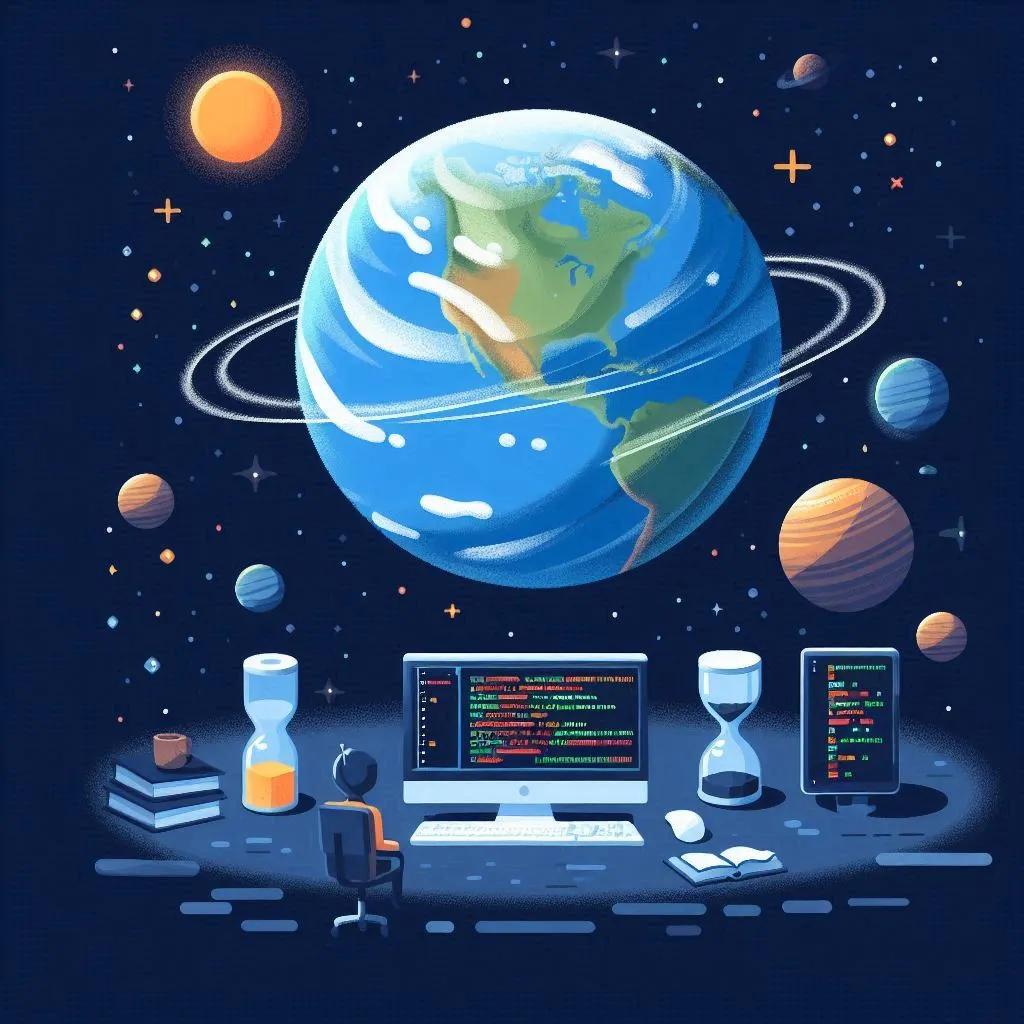
- Speculate about the number of civilizations in our galaxy using a simplified version of the Drake equation.
- Determine whether an exoplanet can be detected from Earth based on its size and distance from its star.
Key Objectives
- Model and Compute: Use mathematical models to compute values related to exoplanet detection.
- Develop Interactive Python Code: Create a command-line program that users can interact with to input values and receive outputs.
- Communicate Effectively: Provide explanations and results in a manner tailored to both science rookies and enthusiasts.
Mathematical Modeling
1. Speculating about Civilizations
Drake Equation
The Drake Equation is a foundational tool in the search for extraterrestrial intelligence. It estimates the number of advanced civilizations in our galaxy with which we might communicate. The equation is:
N=R×p×n×c×L
Where:
- N = Number of civilizations with which we might communicate
- R = Average rate of star formation per year
- p = Proportion of stars with planetary systems
- n = Number of planets per system with conditions suitable for life
- c = Proportion of habitable planets where technological civilizations develop
- L = Average lifetime of such civilizations
Parameters and Estimates
Table 2 from the assignment provides two sets of estimates:
1960s Estimation:
- R=10 stars per year
- p=0.5
- n=2
- c=0.000
- L=10,000 years
Recent Estimation:
- R=7 stars per year
- p=0.5
- n=1
- c=0.02
- L=10,000 years
These parameters will be used to calculate N under both historical and recent estimates.
2. Detecting Exoplanets
Basic Assumptions
We simplify the detection model with the following assumptions:
- Star Size: The star has the same size and mass as the Sun.
- Perfect Alignment: The exoplanet is perfectly aligned for observation.
- Uniform Light Distribution: The star's light is uniformly distributed.
- Small Exoplanet: The exoplanet’s radius is much smaller than the star’s radius.
Key Calculations
1. Relative Intensity
The minimum relative intensity is calculated as:
Relative Intensity= 1 − (Cross-Sectional Area of Planet/Cross-Sectional Area of Star)
The cross-sectional area of a sphere is given by πr^2, so:
Relative Intensity=1− (πr^2planet/ πr^2star) = 1-(r planet/ r star)^2
2. Transit Time
The time the exoplanet takes to transit across the star’s disk can be calculated using:
Transit Time=Diameter of Star/Velocity of Exoplanet
The velocity v of the exoplanet is given by:
v = sqrt{G M/d}
- G = Gravitational constant (6.67430×10^−11 m^3kg^−1s^−2)
- M = Mass of the star (for our calculations, use the Sun’s mass)
- d = Distance of the exoplanet from the star
v=sqrt{GMsun/d}
3. Orbital Period
The orbital period T can be computed as:
T=Circumference of Orbit/Velocity of Exoplanet=2πd/v
Advanced Section
For the advanced section, we’ll plot the relative intensity over time as the exoplanet transits the star. This involves creating a time-stepping simulation to compute how the intensity changes dynamically.
Python Program Implementation
1. Import Necessary Libraries
import math
import matplotlib.pyplot as plt
import numpy as np
2. Functions for Drake Equation
def calculate_civilizations(R, p, n, c, L):
return R * p * n * c * L
3. Functions for Exoplanet Detection
def calculate_relative_intensity(r_planet, r_star):
return 1 - (r_planet / r_star) ** 2
def calculate_transit_time(d_star, v_exoplanet):
return d_star / v_exoplanet
def calculate_orbital_period(d_exoplanet, v_exoplanet):
return 2 * math.pi * d_exoplanet / v_exoplanet
4. Interactive User Input
def get_user_input():
print("Welcome to the Exoplanet Detection Simulator!")
# Drake Equation Inputs
R = float(input("Enter the average rate of star formation per year (e.g., 7): "))
p = float(input("Enter the proportion of stars with planetary systems (e.g., 0.5): "))
n = float(input("Enter the number of planets per system with conditions suitable for life (e.g., 1): "))
c = float(input("Enter the proportion of habitable planets where technological civilizations develop (e.g., 0.02): "))
L = float(input("Enter the average lifetime of such civilizations (e.g., 10000 years): "))
# Exoplanet Detection Inputs
r_planet = float(input("Enter the radius of the exoplanet (in terms of Earth’s radius, e.g., 0.9): "))
r_star = float(input("Enter the radius of the star (in terms of Sun’s radius, e.g., 1): "))
d_star = float(input("Enter the diameter of the star (in terms of Sun’s diameter, e.g., 1): "))
d_exoplanet = float(input("Enter the distance of the exoplanet from the star (in AU, e.g., 1): "))
return R, p, n, c, L, r_planet, r_star, d_star, d_exoplanet
5. Main Program Logic
def main():
R, p, n, c, L, r_planet, r_star, d_star, d_exoplanet = get_user_input()
# Calculate and display the number of civilizations
N = calculate_civilizations(R, p, n, c, L)
print(f"\nEstimated number of civilizations in the galaxy: {N}")
# Calculate and display the relative intensity
intensity = calculate_relative_intensity(r_planet, r_star)
print(f"Minimum relative intensity when the exoplanet transits the star: {intensity:.4f}")
# Calculate the velocity of the exoplanet
v_exoplanet = math.sqrt(6.67430e-11 * 1.989e30 / (d_exoplanet * 1.496e11))
# Calculate and display the transit time and orbital period
transit_time = calculate_transit_time(d_star * 1.496e11, v_exoplanet)
orbital_period = calculate_orbital_period(d_exoplanet * 1.496e11, v_exoplanet)
print(f"Transit time: {transit_time:.2f} seconds")
print(f"Orbital period: {orbital_period:.2f} seconds")
# Detection Check
if intensity <= 1e-4:
print("The exoplanet is detectable with current technology.")
else:
print("The exoplanet may not be detectable with current technology.")
# Plot for advanced section
times = np.linspace(0, transit_time, num=1000)
intensities = 1 - (r_planet / r_star) ** 2 * (np.abs(np.sin(np.pi * times / transit_time)))
plt.plot(times, intensities)
plt.title('Relative Intensity over Time')
plt.xlabel('Time (seconds)')
plt.ylabel('Relative Intensity')
plt.grid(True)
plt.show()
if __name__ == "__main__":
main()
Explanation and Analysis
This program is designed to educate users about the process of exoplanet detection using the transit method. Let’s break down each part of the solution:
- Greeting the User and Input Collection: Depending on whether the user is a science rookie or enthusiast, the program adapts its explanations. This ensures that both groups are catered to appropriately, which is critical in educational settings like a museum.
- Key Calculations: We compute the velocity of the exoplanet using Newtonian mechanics, then calculate the relative intensity of the light based on the size of the exoplanet and the star. This demonstrates the concept of the transit method and how it works in practice.
- Detection Logic: The program determines whether the exoplanet is detectable based on the minimum relative intensity during the transit. This is a simplified version of the actual method used by telescopes like Kepler.
- Graphing for Science Enthusiasts: For those with a deeper interest in science, the graph illustrates the change in relative intensity over time as the planet passes in front of the star. This helps visualize the transit process.
Conclusion
This assignment integrates mathematical modeling and programming to create an engaging and educational experience for museum visitors. By developing an interactive Python program, we enable users to explore the possibilities of extraterrestrial civilizations using the Drake Equation and understand exoplanet detection through the transit method. By tailoring communication strategies to different audience levels, the program ensures that both novices and enthusiasts can appreciate and learn from the exhibit. The use of interactive elements and real-time calculations enhances the educational impact and fosters a deeper understanding of the wonders of our universe. This detailed guide serves as a comprehensive resource for implementing and explaining the program, ensuring a successful and educational exhibit. Adjustments and additional topics can be incorporated based on specific audience needs or interests to solve programming assignments effectively.