Claim Your Discount Today
Ring in Christmas and New Year with a special treat from www.programminghomeworkhelp.com! Get 15% off on all programming assignments when you use the code PHHCNY15 for expert assistance. Don’t miss this festive offer—available for a limited time. Start your New Year with academic success and savings. Act now and save!
We Accept
- Core Concepts of Multi-threaded Programming
- 1. Semaphores
- 2. Semaphore Operations
- 3. Implementing Semaphores Using Mutexes and Condition Variables
- 4. Practical Applications of Semaphores
- Implementing a Producer-Consumer Queue
- 1. What is a Producer-Consumer Queue?
- 2. Key Concepts in Producer-Consumer Queue
- 3. Implementing a Producer-Consumer Queue
- Handling Edge Cases
- 1. Resource Management
- 2. Deadlocks
- 3. Race Conditions
- 4. Condition Variables
- Testing and Debugging
- 1. Unit Testing
- 2. Integration Testing
- 3. Tools
- 4. Logging
- Advanced Topics
- 1. Deadlock Avoidance Algorithms
- 2. Lock-Free Data Structures
- 3. Real-Time Systems
- 4. Performance Optimization
- Conclusion
Multithreaded programming introduces complex challenges when it comes to managing shared resources and ensuring proper communication between threads. To handle these challenges effectively, synchronization mechanisms such as semaphores and producer-consumer queues become crucial. Multi-threaded C programming assignments can be some of the most challenging tasks in a computer science curriculum. They often involve implementing synchronization primitives, like semaphores, and complex data structures, such as producer-consumer queues. This guide will provide an in-depth explanation of these mechanisms, including their implementation using POSIX threads (Pthreads), and offer insights into solving related data structure programming assignments.
Core Concepts of Multi-threaded Programming
Before diving into implementation details, it's crucial to understand the core concepts of multi-threaded programming, especially synchronization primitives and the producer-consumer problem.
1. Semaphores
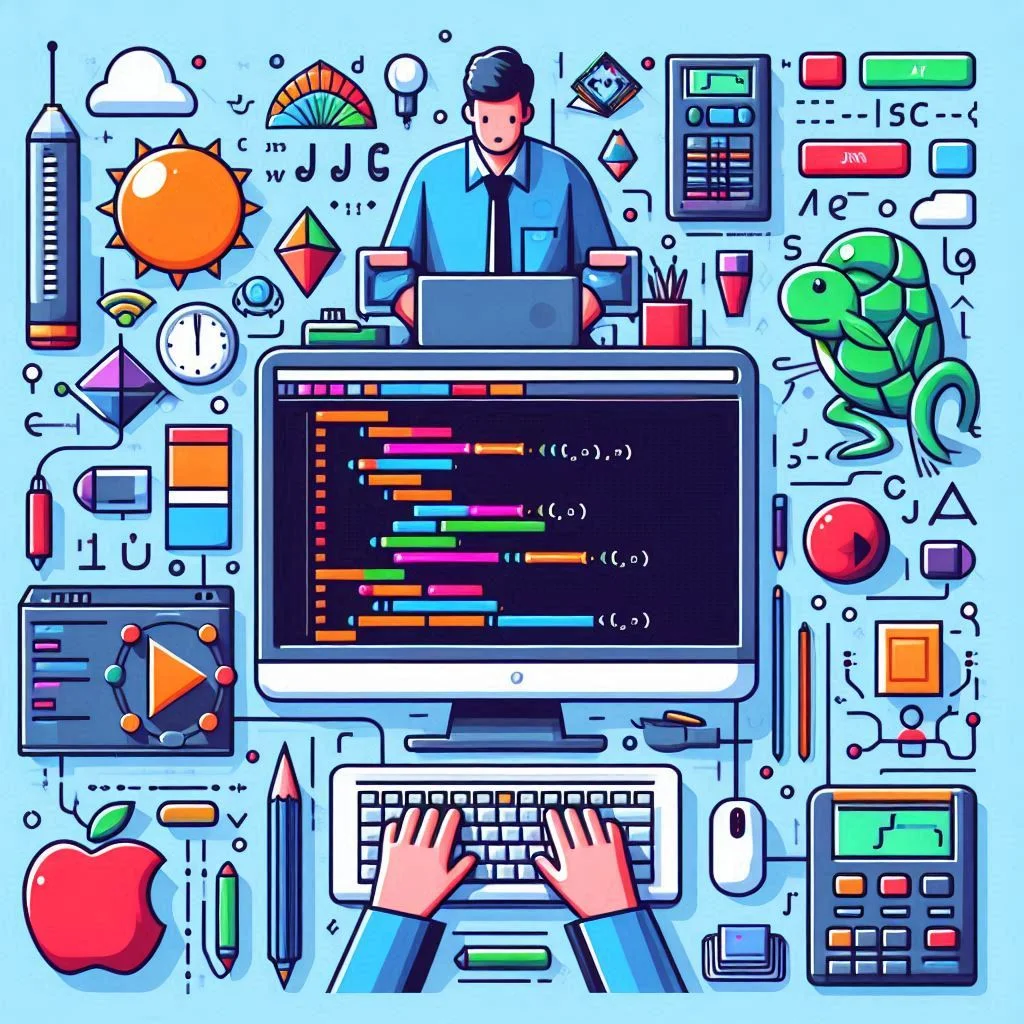
A semaphore is a synchronization primitive that controls access to shared resources by multiple threads. It maintains a count, which represents the number of threads that can access the resource simultaneously. Semaphores are often used to manage resource pools, such as limiting the number of threads accessing a critical section or a limited number of resources like database connections.
There are two main types of semaphores:
- Counting Semaphores: These have a count that can range from zero to a maximum value. They are used to manage access to a pool of resources.
- Binary Semaphores: These are a special case of counting semaphores with a count of either zero or one. They are used as a simpler form of mutex.
2. Semaphore Operations
Semaphores support two fundamental operations:
- Wait (P Operation): Decrements the semaphore’s count. If the count is negative, the calling thread blocks until the count becomes non-negative.
- Signal (V Operation): Increments the semaphore’s count. If there are any threads blocked in the wait operation, one of them is unblocked.
These operations ensure mutual exclusion and coordination among threads. The semaphore's value determines whether a thread can proceed or must wait.
3. Implementing Semaphores Using Mutexes and Condition Variables
In POSIX threads (Pthreads), semaphores are not provided directly. Instead, you can implement semaphores using mutexes and condition variables. Here’s a step-by-step guide to implementing a semaphore:
- Initialize the Semaphore
You need to initialize a mutex and a condition variable. The mutex will protect access to the semaphore’s internal state, and the condition variable will manage the waiting and signaling of threads.
pthread_mutex_t mutex;
pthread_cond_t cond;
int count;
void semaphore_init(int initial_count) {
pthread_mutex_init(&mutex, NULL);
pthread_cond_init(&cond, NULL);
count = initial_count;
}
- Wait Operation
The wait operation decreases the semaphore count. If the count is less than zero, the thread must wait until the count becomes non-negative.
void semaphore_wait() {
pthread_mutex_lock(&mutex);
while (count <= 0) {
pthread_cond_wait(&cond, &mutex);
}
count--;
pthread_mutex_unlock(&mutex);
}
- Signal Operation
The signal operation increases the semaphore count. If there are any threads waiting, one of them will be awakened.
void semaphore_signal() {
pthread_mutex_lock(&mutex);
count++;
if (count > 0) {
pthread_cond_signal(&cond);
}
pthread_mutex_unlock(&mutex);
}
- Destroy the Semaphore
Clean up the resources used by the semaphore, including the mutex and condition variable.
void semaphore_destroy() {
pthread_mutex_destroy(&mutex);
pthread_cond_destroy(&cond);
}
4. Practical Applications of Semaphores
Semaphores are used in various scenarios, including:
- Resource Management: Limiting the number of threads that can access a resource, such as a connection pool.
- Synchronization: Coordinating threads to ensure that they proceed in a specific order.
- Preventing Race Conditions: Ensuring that critical sections of code are executed atomically.
Implementing a Producer-Consumer Queue
1. What is a Producer-Consumer Queue?
The producer-consumer problem is a classic synchronization issue where producers generate data and place it into a shared queue, while consumers retrieve and process the data. This requires coordination between producers and consumers to handle the queue's capacity and ensure FIFO (first-in, first-out) order.
2. Key Concepts in Producer-Consumer Queue
- Queue Structure
The queue can be implemented as a circular buffer or a linked list. A circular buffer is efficient for fixed-size queues, while a linked list can handle dynamically sized queues.
- Producer Operations
Producers need to:
- Check if there is space available in the queue.
- Block if the queue is full until space becomes available.
- Insert the data at the end of the queue.
Consumer Operations
Consumers need to:
- Check if there is data in the queue.
- Block if the queue is empty until data becomes available.
- Remove data from the front of the queue.
3. Implementing a Producer-Consumer Queue
Here’s a detailed guide to implementing a producer-consumer queue using semaphores and mutexes:
- Queue Structure
Define a structure for the queue, including a buffer, head and tail indices, and semaphores for managing the buffer's state.
#define BUFFER_SIZE 10
typedef struct {
void *buffer[BUFFER_SIZE];
int head;
int tail;
int count;
pthread_mutex_t mutex;
pthread_cond_t not_full;
pthread_cond_t not_empty;
} PCQueue;
PCQueue* pcq_create() {
PCQueue *pcq = (PCQueue*) malloc(sizeof(PCQueue));
pcq->head = 0;
pcq->tail = 0;
pcq->count = 0;
pthread_mutex_init(&pcq->mutex, NULL);
pthread_cond_init(&pcq->not_full, NULL);
pthread_cond_init(&pcq->not_empty, NULL);
return pcq;
}
- Insert Operation
The producer inserts an item into the queue:
void pcq_insert(PCQueue *pcq, void *data) {
pthread_mutex_lock(&pcq->mutex);
while (pcq->count == BUFFER_SIZE) {
pthread_cond_wait(&pcq->not_full, &pcq->mutex);
}
pcq->buffer[pcq->tail] = data;
pcq->tail = (pcq->tail + 1) % BUFFER_SIZE;
pcq->count++;
pthread_cond_signal(&pcq->not_empty);
pthread_mutex_unlock(&pcq->mutex);
}
- Retrieve Operation
The consumer retrieves an item from the queue:
void* pcq_retrieve(PCQueue *pcq) {
pthread_mutex_lock(&pcq->mutex);
while (pcq->count == 0) {
pthread_cond_wait(&pcq->not_empty, &pcq->mutex);
}
void *data = pcq->buffer[pcq->head];
pcq->head = (pcq->head + 1) % BUFFER_SIZE;
pcq->count--;
pthread_cond_signal(&pcq->not_full);
pthread_mutex_unlock(&pcq->mutex);
return data;
}
- Destroy the Queue
Clean up the resources used by the queue:
void pcq_destroy(PCQueue *pcq) {
pthread_mutex_destroy(&pcq->mutex);
pthread_cond_destroy(&pcq->not_full);
pthread_cond_destroy(&pcq->not_empty);
free(pcq);
}
Handling Edge Cases
When working with multi-threaded programs, it’s important to handle edge cases to ensure robustness and avoid deadlocks or resource leaks.
1. Resource Management
Ensure that all resources (memory, mutexes, condition variables) are properly managed. For instance, always free allocated memory and destroy synchronization primitives when they are no longer needed.
2. Deadlocks
Be cautious of deadlocks, which occur when two or more threads are waiting indefinitely for each other to release resources. Avoid holding multiple locks simultaneously, and ensure that locks are always acquired and released in a consistent order.
3. Race Conditions
Race conditions can occur when multiple threads access shared data concurrently, leading to unpredictable results. Properly synchronize access to shared resources using mutexes and ensure that critical sections are minimal.
4. Condition Variables
When using condition variables, ensure that you handle spurious wakeups and always check the condition in a loop to avoid unexpected behavior.
Testing and Debugging
Testing multi-threaded code can be complex due to the non-deterministic nature of thread execution. Here are some strategies to effectively test and debug your implementations:
1. Unit Testing
Write unit tests for individual functions to ensure they behave correctly in isolation. Use assertions to verify that your semaphores and queues work as expected.
2. Integration Testing
Test your entire system by simulating scenarios with multiple producers and consumers. Ensure that the queue handles concurrent access correctly and maintains FIFO order.
3. Tools
Use debugging tools like GDB to step through your code and track thread execution. Tools like Valgrind can help detect memory leaks and thread-related issues.
4. Logging
Implement logging to trace thread activity and diagnose issues. Logging can help you understand the sequence of events and identify problems in synchronization.
Advanced Topics
For those interested in diving deeper into multi-threaded programming, consider exploring the following advanced topics:
1. Deadlock Avoidance Algorithms
Learn about algorithms and techniques for avoiding deadlocks, such as the Banker's Algorithm and resource allocation graphs.
2. Lock-Free Data Structures
Explore lock-free data structures that avoid the use of traditional locking mechanisms, potentially improving performance in highly concurrent environments.
3. Real-Time Systems
Study real-time systems where timing constraints are crucial, and ensure that synchronization mechanisms meet real-time requirements.
4. Performance Optimization
Investigate performance optimization techniques, including reducing lock contention, optimizing memory access patterns, and using hardware-specific features.
Conclusion
Multi-threaded programming assignments can be challenging, but understanding the core concepts, implementing synchronization primitives, and handling edge cases effectively will set you on the right path. By following best practices, testing thoroughly, and continuously improving your code, you can develop robust and efficient multi-threaded applications. With practice and attention to detail, you'll become proficient in managing concurrent tasks. Additionally if you are in search for help with your programming assignments then applying these methods would be beneficial for you.