Claim Your Offer
New semester, new challenges—but don’t stress, we’ve got your back! Get expert programming assignment help and breeze through Python, Java, C++, and more with ease. For a limited time, enjoy 10% OFF on all programming assignments with the Spring Special Discount! Just use code SPRING10OFF at checkout! Why stress over deadlines when you can score high effortlessly? Grab this exclusive offer now and make this semester your best one yet!
We Accept
- Understanding the Requirements
- Contact Service Requirements
- Task Service Requirements
- Appointment Service Requirements
- Designing Data Models
- Contact Model
- Task Model
- Appointment Model
- Developing Service Classes
- ContactService
- TaskService
- AppointmentService
- Implementing Business Logic
- Contact Business Logic
- Task Business Logic
- Appointment Business Logic
- Writing Unit Tests
- ContactService Unit Tests
- TaskService Unit Tests
- AppointmentService Unit Tests
- Ensuring Code Quality
- Code Reviews
- Continuous Integration and Deployment
- Benefits of CI/CD
- Documentation
- Conclusion
When tasked with developing a mobile application that includes services for managing contacts, tasks, and appointments, it is essential to follow a systematic approach. Below is a guide to help you develop such an application effectively, focusing on core principles and methodologies that can be applied to similar assignments. Whether you need help with a specific programming challenge or general help with Java assignment tasks, this guide will provide valuable insights to streamline your development process.
Understanding the Requirements
Before diving into the development process, it's crucial to thoroughly understand the requirements. The application involves three main services: Contact Service, Task Service, and Appointment Service. Each service has specific requirements for its respective data objects and functionalities.
Contact Service Requirements
Contact Class Requirements
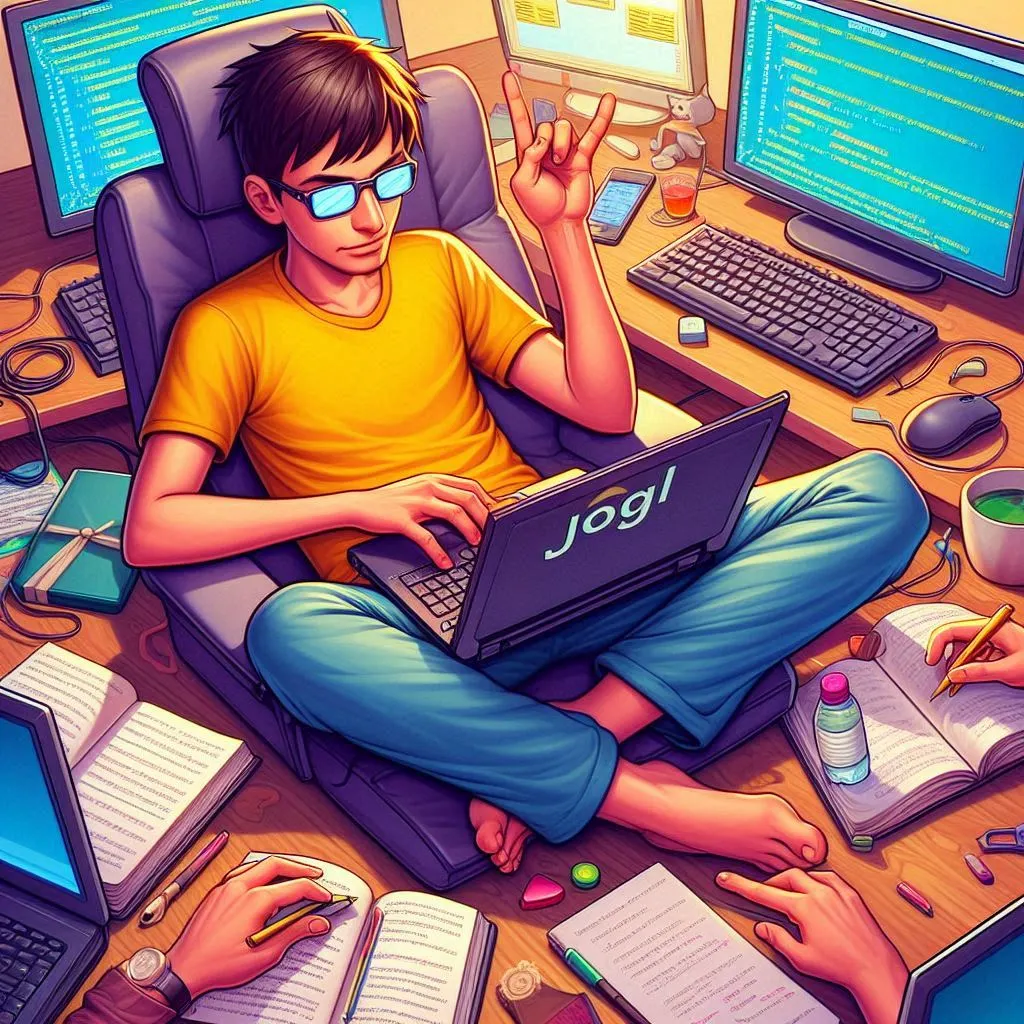
- Unique Contact ID: A required unique contact ID string that cannot be longer than 10 characters. The contact ID must not be null and must not be updatable.
- First Name: A required firstName string field that cannot be longer than 10 characters. The firstName field must not be null.
- Last Name: A required lastName string field that cannot be longer than 10 characters. The lastName field must not be null.
- Phone Number: A required phone string field that must be exactly 10 digits. The phone field must not be null.
- Address: A required address field that must be no longer than 30 characters. The address field must not be null.
Contact Service Functionalities
- Add Contacts: The service must be able to add contacts with a unique ID.
- Delete Contacts: The service must be able to delete contacts by their contactId.
- Update Contacts: The service must be able to update contact fields by their contactId, specifically the firstName, lastName, phone number, and address.
Task Service Requirements
Task Class Requirements
- Unique Task ID: A required unique task ID string that cannot be longer than 10 characters. The task ID must not be null and must not be updatable.
- Task Name: A required name string field that cannot be longer than 20 characters. The name field must not be null.
- Task Description: A required description string field that cannot be longer than 50 characters. The description field must not be null.
Task Service Functionalities
- Add Tasks: The service must be able to add tasks with a unique ID.
- Delete Tasks: The service must be able to delete tasks by their taskId.
- Update Tasks: The service must be able to update task fields by their taskId, specifically the name and description.
Appointment Service Requirements
Appointment Class Requirements
- Unique Appointment ID: A required unique appointment ID string that cannot be longer than 10 characters. The appointment ID must not be null and must not be updatable.
- Appointment Date: A required appointment date field that cannot be in the past. The appointmentDate field must not be null.
- Appointment Description: A required description string field that cannot be longer than 50 characters. The description field must not be null.
Appointment Service Functionalities
- Add Appointments: The service must be able to add appointments with a unique appointmentId.
- Delete Appointments: The service must be able to delete appointments by their appointmentId.
Designing Data Models
The data models form the backbone of your application. Each service—Contact, Task, and Appointment—requires its own model with specific attributes and constraints.
Contact Model
Attributes
- contactId: Unique identifier, max 10 characters, immutable.
- firstName: Max 10 characters, cannot be null.
- lastName: Max 10 characters, cannot be null.
- phone: Exactly 10 digits, cannot be null.
- address: Max 30 characters, cannot be null.
Example Code
public class Contact {
private final String contactId;
private String firstName;
private String lastName;
private String phone;
private String address;
public Contact(String contactId, String firstName, String lastName, String phone, String address) {
if (contactId == null || contactId.length() > 10) throw new IllegalArgumentException("Invalid ID");
if (firstName == null || firstName.length() > 10) throw new IllegalArgumentException("Invalid first name");
if (lastName == null || lastName.length() > 10) throw new IllegalArgumentException("Invalid last name");
if (phone == null || phone.length() != 10) throw new IllegalArgumentException("Invalid phone number");
if (address == null || address.length() > 30) throw new IllegalArgumentException("Invalid address");
this.contactId = contactId;
this.firstName = firstName;
this.lastName = lastName;
this.phone = phone;
this.address = address;
}
// Getters and setters...
}
Task Model
Attributes
- taskId: Unique identifier, max 10 characters, immutable.
- name: Max 20 characters, cannot be null.
- description: Max 50 characters, cannot be null.
Example Code
public class Task {
private final String taskId;
private String name;
private String description;
public Task(String taskId, String name, String description) {
if (taskId == null || taskId.length() > 10) throw new IllegalArgumentException("Invalid ID");
if (name == null || name.length() > 20) throw new IllegalArgumentException("Invalid name");
if (description == null || description.length() > 50) throw new IllegalArgumentException("Invalid description");
this.taskId = taskId;
this.name = name;
this.description = description;
}
// Getters and setters...
}
Appointment Model
Attributes
- appointmentId: Unique identifier, max 10 characters, immutable.
- appointmentDate: Cannot be in the past, cannot be null.
- description: Max 50 characters, cannot be null.
Example Code
import java.util.Date;
public class Appointment {
private final String appointmentId;
private Date appointmentDate;
private String description;
public Appointment(String appointmentId, Date appointmentDate, String description) {
if (appointmentId == null || appointmentId.length() > 10) throw new IllegalArgumentException("Invalid ID");
if (appointmentDate == null || appointmentDate.before(new Date())) throw new IllegalArgumentException("Invalid date");
if (description == null || description.length() > 50) throw new IllegalArgumentException("Invalid description");
this.appointmentId = appointmentId;
this.appointmentDate = appointmentDate;
this.description = description;
}
// Getters and setters...
}
Developing Service Classes
Service classes handle CRUD (Create, Read, Update, Delete) operations for the respective data models. Here’s how to structure each service.
ContactService
Methods
- addContact(Contact contact): Adds a new contact with a unique ID.
- deleteContact(String contactId): Deletes a contact by ID.
- updateContact(String contactId, String firstName, String lastName, String phone, String address): Updates contact details by ID.
Example Code
import java.util.HashMap;
import java.util.Map;
public class ContactService {
private Map<String, Contact> contacts = new HashMap<>();
public void addContact(Contact contact) {
if (contacts.containsKey(contact.getContactId())) throw new IllegalArgumentException("Contact ID already exists");
contacts.put(contact.getContactId(), contact);
}
public void deleteContact(String contactId) {
if (!contacts.containsKey(contactId)) throw new IllegalArgumentException("Contact ID not found");
contacts.remove(contactId);
}
public void updateContact(String contactId, String firstName, String lastName, String phone, String address) {
Contact contact = contacts.get(contactId);
if (contact == null) throw new IllegalArgumentException("Contact ID not found");
contact.setFirstName(firstName);
contact.setLastName(lastName);
contact.setPhone(phone);
contact.setAddress(address);
}
}
TaskService
Methods
- addTask(Task task): Adds a new task with a unique ID.
- deleteTask(String taskId): Deletes a task by ID.
- updateTask(String taskId, String name, String description): Updates task details by ID.
Example Code
import java.util.HashMap;
import java.util.Map;
public class TaskService {
private Map<String, Task> tasks = new HashMap<>();
public void addTask(Task task) {
if (tasks.containsKey(task.getTaskId())) throw new IllegalArgumentException("Task ID already exists");
tasks.put(task.getTaskId(), task);
}
public void deleteTask(String taskId) {
if (!tasks.containsKey(taskId)) throw new IllegalArgumentException("Task ID not found");
tasks.remove(taskId);
}
public void updateTask(String taskId, String name, String description) {
Task task = tasks.get(taskId);
if (task == null) throw new IllegalArgumentException("Task ID not found");
task.setName(name);
task.setDescription(description);
}
}
AppointmentService
Methods
- addAppointment(Appointment appointment): Adds a new appointment with a unique ID.
- deleteAppointment(String appointmentId): Deletes an appointment by ID.
Example Code
import java.util.HashMap; import java.util.Map; public class AppointmentService { private Map String, Appointment appointments = new HashMap(); public void addAppointment(Appointment appointment) { if (appointments.containsKey(appointment.getAppointmentId())) throw new IllegalArgumentException("Appointment ID already exists"); appointments.put(appointment.getAppointmentId(), appointment); } public void deleteAppointment(String appointmentId) { if (!appointments.containsKey(appointmentId)) throw new IllegalArgumentException("Appointment ID not found"); appointments.remove(appointmentId); } }
Implementing Business Logic
The business logic ensures that data adheres to the requirements and constraints. Proper validation and error handling are crucial for a robust application.
Contact Business Logic
Validation
- Ensure contactId is unique and has a maximum length of 10 characters.
- Ensure firstName and lastName are not null and have a maximum length of 10 characters.
- Ensure phone is exactly 10 digits long.
- Ensure address is not null and has a maximum length of 30 characters.
Example Validation Code
public class Contact {
private final String contactId;
private String firstName;
private String lastName;
private String phone;
private String address;
public Contact(String contactId, String firstName, String lastName, String phone, String address) {
if (contactId == null || contactId.length() > 10) throw new IllegalArgumentException("Invalid ID");
if (firstName == null || firstName.length() > 10) throw new IllegalArgumentException("Invalid first name");
if (lastName == null || lastName.length() > 10) throw new IllegalArgumentException("Invalid last name");
if (phone == null || phone.length() != 10) throw new IllegalArgumentException("Invalid phone number");
if (address == null || address.length() > 30) throw new IllegalArgumentException("Invalid address");
this.contactId = contactId;
this.firstName = firstName;
this.lastName = lastName;
this.phone = phone;
this.address = address;
}
}
Task Business Logic
Validation
- Ensure taskId is unique and has a maximum length of 10 characters.
- Ensure name is not null and has a maximum length of 20 characters.
- Ensure description is not null and has a maximum length of 50 characters.
Example Validation Code
public class Task {
private final String taskId;
private String name;
private String description;
public Task(String taskId, String name, String description) {
if (taskId == null || taskId.length() > 10) throw new IllegalArgumentException("Invalid ID");
if (name == null || name.length() > 20) throw new IllegalArgumentException("Invalid name");
if (description == null || description.length() > 50) throw new IllegalArgumentException("Invalid description");
this.taskId = taskId;
this.name = name;
this.description = description;
}
}
Appointment Business Logic
Validation
- Ensure appointmentId is unique and has a maximum length of 10 characters.
- Ensure appointmentDate is not null and is not in the past.
- Ensure description is not null
Example Validation Code
import java.util.Date;
public class Appointment {
private final String appointmentId;
private Date appointmentDate;
private String description;
public Appointment(String appointmentId, Date appointmentDate, String description) {
if (appointmentId == null || appointmentId.length() > 10) throw new IllegalArgumentException("Invalid ID");
if (appointmentDate == null || appointmentDate.before(new Date())) throw new IllegalArgumentException("Invalid date");
if (description == null || description.length() > 50) throw new IllegalArgumentException("Invalid description");
this.appointmentId = appointmentId;
this.appointmentDate = appointmentDate;
this.description = description;
}
}
Writing Unit Tests
Unit tests are essential for ensuring the reliability and correctness of your application. They help catch bugs early in the development process and provide a safety net for future code changes.
ContactService Unit Tests
Example Test Cases
- Test Adding a Contact: Ensure a new contact can be added successfully.
- Test Deleting a Contact: Ensure a contact can be deleted by ID.
- Test Updating a Contact: Ensure contact details can be updated by ID.
Example Unit Test Code
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
public class ContactServiceTest {
@Test
public void testAddContact() {
ContactService service = new ContactService();
Contact contact = new Contact("1", "John", "Doe", "1234567890", "123 Main St");
service.addContact(contact);
assertEquals(contact, service.getContact("1"));
}
@Test
public void testDeleteContact() {
ContactService service = new ContactService();
Contact contact = new Contact("1", "John", "Doe", "1234567890", "123 Main St");
service.addContact(contact);
service.deleteContact("1");
assertNull(service.getContact("1"));
}
@Test
public void testUpdateContact() {
ContactService service = new ContactService();
Contact contact = new Contact("1", "John", "Doe", "1234567890", "123 Main St");
service.addContact(contact);
service.updateContact("1", "Jane", "Doe", "0987654321", "456 Elm St");
Contact updatedContact = service.getContact("1");
assertEquals("Jane", updatedContact.getFirstName());
assertEquals("Doe", updatedContact.getLastName());
assertEquals("0987654321", updatedContact.getPhone());
assertEquals("456 Elm St", updatedContact.getAddress());
}
}
TaskService Unit Tests
Example Test Cases
- Test Adding a Task: Ensure a new task can be added successfully.
- Test Deleting a Task: Ensure a task can be deleted by ID.
- Test Updating a Task: Ensure task details can be updated by ID.
Example Unit Test Code
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
public class TaskServiceTest {
@Test
public void testAddTask() {
TaskService service = new TaskService();
Task task = new Task("1", "Homework", "Complete math homework");
service.addTask(task);
assertEquals(task, service.getTask("1"));
}
@Test
public void testDeleteTask() {
TaskService service = new TaskService();
Task task = new Task("1", "Homework", "Complete math homework");
service.addTask(task);
service.deleteTask("1");
assertNull(service.getTask("1"));
}
@Test
public void testUpdateTask() {
TaskService service = new TaskService();
Task task = new Task("1", "Homework", "Complete math homework");
service.addTask(task);
service.updateTask("1", "Chores", "Clean the house");
Task updatedTask = service.getTask("1");
assertEquals("Chores", updatedTask.getName());
assertEquals("Clean the house", updatedTask.getDescription());
}
}
AppointmentService Unit Tests
Example Test Cases
- Test Adding an Appointment: Ensure a new appointment can be added successfully.
- Test Deleting an Appointment: Ensure an appointment can be deleted by ID.
Example Unit Test Code
import org.junit.jupiter.api.Test;
import java.util.Date;
import static org.junit.jupiter.api.Assertions.*;
public class AppointmentServiceTest {
@Test
public void testAddAppointment() {
AppointmentService service = new AppointmentService();
Appointment appointment = new Appointment("1", new Date(), "Doctor's appointment");
service.addAppointment(appointment);
assertEquals(appointment, service.getAppointment("1"));
}
@Test
public void testDeleteAppointment() {
AppointmentService service = new AppointmentService();
Appointment appointment = new Appointment("1", new Date(), "Doctor's appointment");
service.addAppointment(appointment);
service.deleteAppointment("1");
assertNull(service.getAppointment("1"));
}
}
Ensuring Code Quality
Maintaining high code quality is critical for the long-term success and maintainability of your application. Here are some best practices to follow:
Code Reviews
Regular code reviews help ensure that code adheres to the project’s coding standards and is free of defects. They also promote knowledge sharing among team members.
Benefits of Code Reviews
- Error Detection: Catch potential bugs and issues early.
- Consistency: Maintain consistent coding standards across the project.
- Learning: Share knowledge and best practices among team members.
Continuous Integration and Deployment
Implementing continuous integration (CI) and continuous deployment (CD) ensures that code changes are automatically tested and deployed, reducing the risk of bugs in production.
Benefits of CI/CD
- Automated Testing: Run tests automatically on every code change to catch issues early.
- Faster Deployment: Deploy changes to production quickly and reliably.
- Feedback Loop: Receive immediate feedback on code changes.
Documentation
Good documentation is essential for the maintainability of your code. Ensure that your code is well-documented, including inline comments and comprehensive README files.
Benefits of Documentation
- Clarity: Make the code easier to understand for new developers.
- Maintenance: Simplify future maintenance and updates.
- Onboarding: Help new team members get up to speed quickly.
Conclusion
By following a structured approach, you can tackle complex programming assignments more effectively. Understand the requirements, plan your approach, implement each component step-by-step, and test thoroughly. With practice, you'll become more proficient at solving your programming assignment and similar tasks. Remember, the key to success lies in breaking down the problem, managing your time wisely, and continuously refining your coding skills. As you gain more experience, you'll find that even the most challenging programming assignments become manageable and rewarding.