Claim Your Discount Today
Kick off the fall semester with a 20% discount on all programming assignments at www.programminghomeworkhelp.com! Our experts are here to support your coding journey with top-quality assistance. Seize this seasonal offer to enhance your programming skills and achieve academic success. Act now and save!
We Accept
- What is a Memory Pool?
- Advantages of Memory Pools
- Core Components of a Memory Pool
- Designing a Memory Pool
- Memory Pool Structure
- Creating the Memory Pool
- Allocating and Freeing Blocks
- Accessing Block Data
- Destroying the Memory Pool
- Practical Example: Using the Memory Pool
- Detailed Explanation of the Sample Application
- Conclusion
Efficient memory management is a cornerstone of high-performance and real-time systems, where every microsecond counts. One of the most effective techniques for managing memory in such environments is the implementation of a memory pool. This method allows developers to optimize memory allocation and deallocation, significantly improving both speed and predictability. This guide delves into the details of designing, implementing, and using memory pools, providing a solid foundation to solve programming assignments and real-world applications.
What is a Memory Pool?
A memory pool is a pre-allocated block of memory managed manually by a software system to handle frequent and predictable allocation and deallocation requests. Instead of relying on the system's heap for each allocation request, a memory pool allows you to allocate a large block of memory upfront and then manage this memory in a controlled manner.
Advantages of Memory Pools
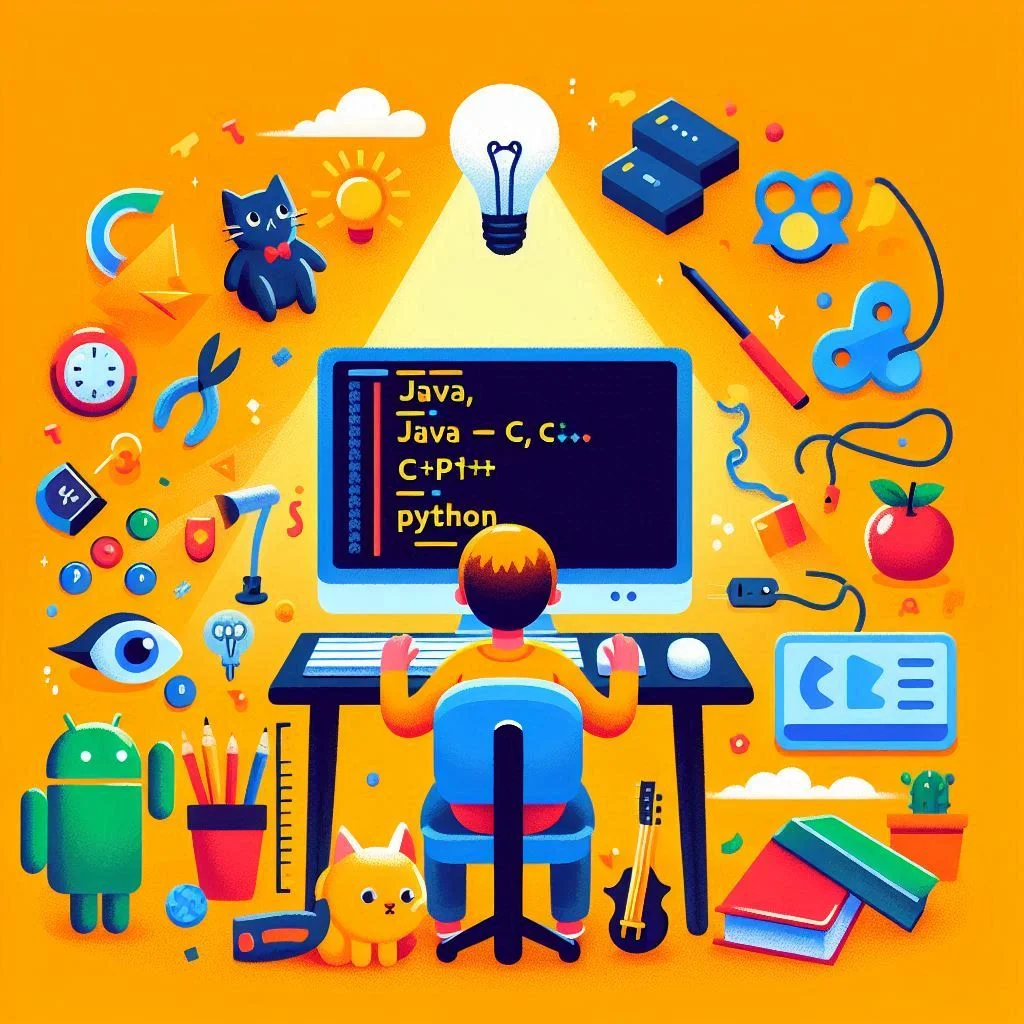
- Performance Efficiency: By reducing the overhead associated with frequent allocation and deallocation operations, memory pools can improve performance, especially in systems with high allocation rates.
- Reduced Fragmentation: Memory pools help mitigate fragmentation issues that can arise from dynamic memory allocation, providing a more stable memory usage pattern.
- Predictable Behavior: The allocation and deallocation operations are more predictable in terms of time complexity, which is crucial for real-time and embedded systems where consistent response times are required.
Core Components of a Memory Pool
To build an effective memory pool, you need to understand its core components:
- Memory Pool Structure: The data structure that holds the pool’s metadata and manages the allocation and deallocation of memory blocks.
- Block Size: The size of each memory block within the pool, which should be optimized based on the application's needs.
- Number of Blocks: The total number of blocks in the pool, determining the maximum number of concurrent allocations that can be handled.
Designing a Memory Pool
Designing a memory pool involves creating a data structure that efficiently manages the allocation and deallocation of memory blocks. Below, we explore the design and implementation details step-by-step.
Memory Pool Structure
The memory pool structure must track both the memory blocks and their allocation status. Here is a typical design:
typedef struct {
unsigned char *pool; // Pointer to the start of the memory pool
int blocksize; // Size of each block
int num_blocks; // Total number of blocks
int *free_blocks; // Array to track free blocks
int free_count; // Number of free blocks
} mp_t;
- pool: Points to the start of the large block of memory allocated for the pool.
- blocksize: Defines the size of each individual block within the pool.
- num_blocks: Specifies the total number of blocks available.
- free_blocks: An array that keeps track of which blocks are free.
- free_count: The count of currently available free blocks.
Creating the Memory Pool
The function for creating a memory pool initializes the pool structure and allocates the required memory:
mp_t *MP_Create(int blocksize, int num_blocks) {
if (blocksize <= 0 || num_blocks <= 0 || blocksize > 1024 || num_blocks > 100) {
return NULL; // Invalid parameters
}
// Allocate memory for the pool structure
mp_t *mp = malloc(sizeof(mp_t));
if (!mp) return NULL; // Memory allocation failure
// Allocate memory for the pool
mp->pool = malloc(blocksize * num_blocks);
if (!mp->pool) {
free(mp);
return NULL; // Memory allocation failure
}
mp->blocksize = blocksize;
mp->num_blocks = num_blocks;
// Allocate memory for the free blocks tracking array
mp->free_blocks = malloc(num_blocks * sizeof(int));
if (!mp->free_blocks) {
free(mp->pool);
free(mp);
return NULL; // Memory allocation failure
}
// Initialize free blocks
for (int i = 0; i < num_blocks; ++i) {
mp->free_blocks[i] = i;
}
mp->free_count = num_blocks;
return mp;
}
Allocating and Freeing Blocks
Two essential functions for managing memory blocks are allocation and deallocation.
Allocating a Block:
int MP_AllocBlock(mp_t *mp) {
if (mp->free_count == 0) {
return -1; // No free blocks available
}
// Get the index of the next free block
int block_idx = mp->free_blocks[--mp->free_count];
return block_idx;
}
- Check Availability: Ensure that there are free blocks available.
- Allocate Block: Reduce the count of free blocks and return the index of the allocated block.
Freeing a Block:
C code
int MP_FreeBlock(mp_t *mp, int block_idx) {
if (block_idx < 0 || block_idx >= mp->num_blocks) {
return 0; // Invalid block index
}
// Clear the block contents
memset(mp->pool + block_idx * mp->blocksize, 0, mp->blocksize);
// Mark the block as free
mp->free_blocks[mp->free_count++] = block_idx;
return 1;
}
- Check Validity: Validate the block index.
- Clear Block: Set the contents of the block to zero to avoid residual data.
- Mark Free: Add the block index to the list of free blocks and increment the free count.
Accessing Block Data
To access the data stored in a block, use the following function:
void *MP_GetBlockData(mp_t *mp, int block_idx) {
if (block_idx < 0 || block_idx >= mp->num_blocks || mp->free_count == mp->num_blocks) {
return NULL; // Block is free or invalid index
}
return mp->pool + block_idx * mp->blocksize;
}
- Check Status: Ensure the block is valid and not free.
- Return Data: Calculate and return the address of the block’s data.
Destroying the Memory Pool
When the memory pool is no longer needed, it must be properly cleaned up:
int MP_Destroy(mp_t **mp) {
if (!mp || !*mp) {
return 0; // Null pointer check
}
free((*mp)->pool);
free((*mp)->free_blocks);
free(*mp);
*mp = NULL;
return 1;
}
- Check Validity: Ensure the memory pool is valid and not already destroyed.
- Free Memory: Release all allocated memory and set the pointer to NULL.
Practical Example: Using the Memory Pool
To illustrate the usage of a memory pool, consider a sample application that performs the following:
- Create the Pool: Initialize a memory pool with specific parameters.
- Allocate and Write: Allocate blocks and write data to them.
- Verify Data: Check if the data in the blocks is correct.
- Handle Errors: Attempt to allocate more blocks than available and handle the error.
- Clean Up: Destroy the memory pool.
Here’s a complete example in C:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// Definitions of the memory pool functions go here
int main() {
// Create a memory pool with block size 2 bytes and 100 blocks
mp_t *mp = MP_Create(2, 100);
if (!mp) {
printf("Failed to create memory pool\n");
return 1;
}
// Allocate some blocks and write data
for (int i = 0; i < 100; ++i) {
int idx = MP_AllocBlock(mp);
if (idx != -1) {
unsigned short *data = (unsigned short *)MP_GetBlockData(mp, idx);
*data = (unsigned short)idx;
}
}
// Verify block data
for (int i = 0; i < 100; ++i) {
unsigned short *data = (unsigned short *)MP_GetBlockData(mp, i);
if (data && *data == i) {
printf("Block %d correctly set to %04x\n", i, *data);
} else {
printf("Block %d not set correctly\n", i);
}
}
// Attempt to allocate more blocks than available
int extra_block = MP_AllocBlock(mp);
if (extra_block == -1) {
printf("Failed to allocate block beyond pool size, as expected\n");
}
// Free the memory pool
if (MP_Destroy(&mp)) {
printf("Memory pool successfully destroyed\n");
}
return 0;
}
Detailed Explanation of the Sample Application
- Creating the Pool: The MP_Create function initializes a memory pool with 100 blocks of 2 bytes each. It returns a pointer to the pool if successful, or NULL if there is a failure in memory allocation.
- Allocating and Writing: Blocks are allocated in a loop, and each block’s data is set to its index value. The MP_AllocBlock function is used to get an available block index, and MP_GetBlockData provides a pointer to the block’s data area.
- Verifying Data: After writing data, each block is checked to ensure it contains the correct value. This is done using a loop that verifies each block’s content.
- Handling Allocation Failure: An attempt is made to allocate an additional block beyond the pool’s capacity to verify that the failure handling works as expected.
- Destroying the Pool: The MP_Destroy function cleans up all allocated memory, ensuring there are no memory leaks.
Conclusion
Memory pools are a powerful technique for managing memory in performance-critical applications. By pre-allocating a block of memory and managing allocations within that block, you can achieve significant performance improvements and reduced fragmentation. This guide has provided a detailed look at designing, implementing, and using a memory pool, offering practical insights and examples to help you tackle similar C programming assignments and real-world scenarios.
With a solid understanding of memory pool mechanics, you are well-prepared to leverage this technique in your own projects, ensuring efficient and reliable memory management.