Claim Your Discount Today
Ring in Christmas and New Year with a special treat from www.programminghomeworkhelp.com! Get 15% off on all programming assignments when you use the code PHHCNY15 for expert assistance. Don’t miss this festive offer—available for a limited time. Start your New Year with academic success and savings. Act now and save!
We Accept
- Introduction to Binary Numbers and Bitwise Operations
- Understanding Binary Numbers
- Common Bitwise Operations
- Practical Applications of Bitwise Operations
- Solving Binary Manipulation Assignments
- Setting Up the Environment
- Implementing Bitwise Operations
- Converting Binary to Decimal
- Performing Bit Shifting
- Handling File Input and Output
- Reading Input from a File
- Writing Output to a File
- Example Program Structure
- Advanced Techniques and Best Practices
- Error Handling and Validation
- Optimizing Performance
- Handling Edge Cases
- Practical Tips for Success
- Conclusion
Binary manipulation assignments are an essential part of programming education, especially for students pursuing computer science and engineering. In order to complete your programming assignments often involve operations on binary numbers, which are the foundation of digital computers and modern technology. Understanding and mastering binary manipulation can enhance your programming skills and provide a deeper insight into how computers process data. This comprehensive guide will help you tackle binary manipulation assignments, such as bitwise operations, binary-to-decimal conversion, and bit shifting. This approach will give you confidence to solve your C++ assignment effectively.
Introduction to Binary Numbers and Bitwise Operations
Binary numbers and bitwise operations are fundamental concepts in computer science. They are used extensively in digital systems, data processing, and various programming applications. Before diving into the specifics of binary manipulation assignments, it's crucial to have a solid understanding of these concepts.
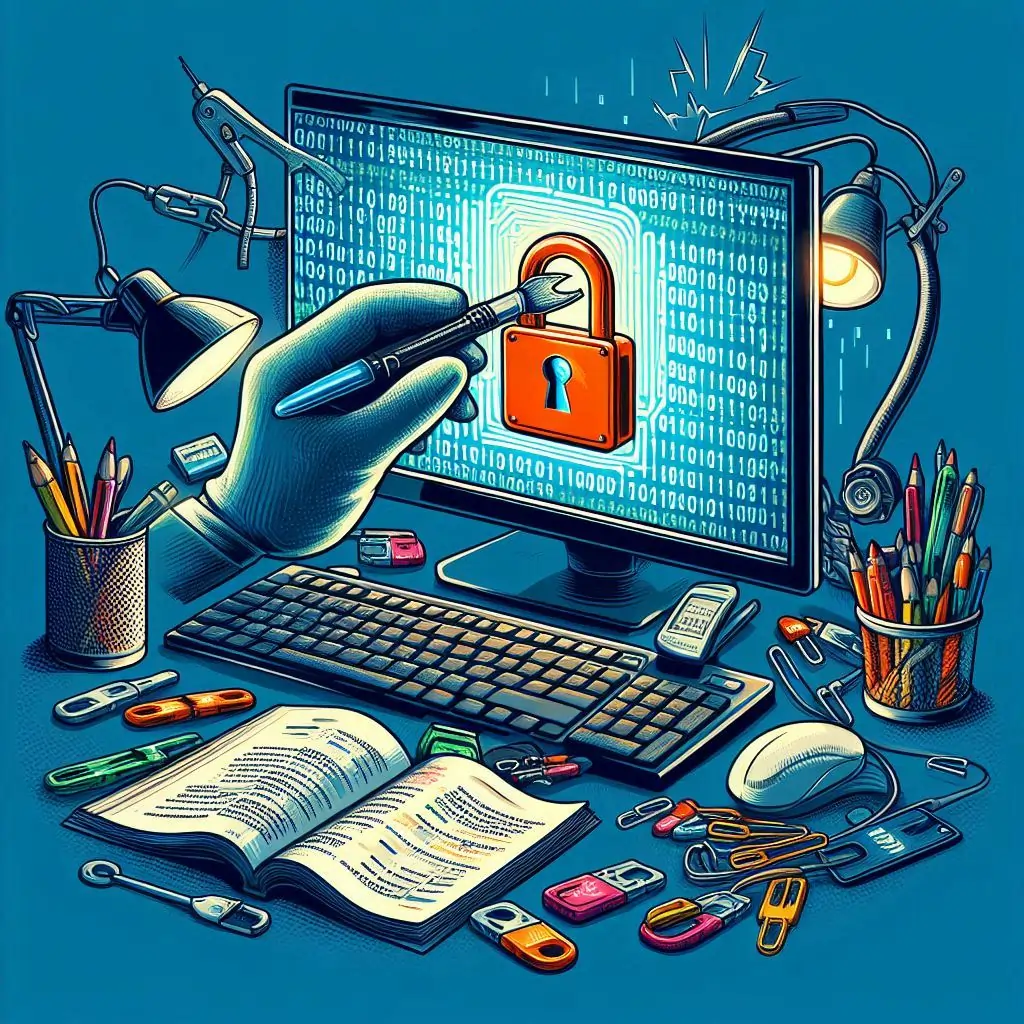
Understanding Binary Numbers
Binary numbers, also known as base-2 numbers, consist of only two digits: 0 and 1. Each digit in a binary number is called a bit. Binary numbers are the basis of all digital systems, including computers, smartphones, and other electronic devices. An 8-bit binary number (or byte) can represent values ranging from 0 to 255.
In a binary number, each bit represents a power of 2. For example, the binary number 10010110 can be converted to a decimal (base-10) number as follows:
- 1 * 2^7 + 0 * 2^6 + 0 * 2^5 + 1 * 2^4 + 0 * 2^3 + 1 * 2^2 + 1 * 2^1 + 0 * 2^0
- 128 + 0 + 0 + 16 + 0 + 4 + 2 + 0 = 150
Understanding how to convert binary numbers to decimal and vice versa is fundamental for performing binary manipulations.
Common Bitwise Operations
Bitwise operations are operations that directly manipulate bits in binary numbers. These operations are used in various programming tasks, including data processing, encryption, and graphics rendering. The most common bitwise operations are NOT, AND, and OR.
Bitwise NOT
The bitwise NOT operation inverts each bit in a binary number. If a bit is 0, it becomes 1, and if it is 1, it becomes 0. For example:
- Operand: 11010011
- Result: 00101100
The bitwise NOT operation is useful for flipping bits and creating complementary binary patterns.
Bitwise AND
The bitwise AND operation compares each bit of two binary numbers. If both bits are 1, the result is 1; otherwise, it is 0. For example:
- Operand 1: 10010010
- Operand 2:11001110
- Result:10000010
The bitwise AND operation is used for masking and extracting specific bits from binary numbers.
Bitwise OR
The bitwise OR operation compares each bit of two binary numbers. If at least one of the bits is 1, the result is 1; otherwise, it is 0. For example:
- Operand 1: 10011001
- Operand 2: 11100101
- Result: 11111101
The bitwise OR operation is used for combining bits and setting specific bits in binary numbers.
Practical Applications of Bitwise Operations
Bitwise operations have numerous practical applications in programming and digital systems. They are used in tasks such as setting and clearing flags, manipulating data for graphics rendering, and optimizing performance in low-level programming.
Understanding how to perform bitwise operations manually is crucial for solving binary manipulation assignments and gaining a deeper insight into how computers process data at the binary level.
Solving Binary Manipulation Assignments
Solving binary manipulation assignments requires a systematic approach and a solid understanding of the fundamental concepts. This section will guide you through the process of solving typical binary manipulation assignments, including bitwise operations, binary-to-decimal conversion, and bit shifting.
Setting Up the Environment
Before you start solving binary manipulation assignments, it's essential to set up your programming environment correctly. This involves choosing the right programming language, setting up your development environment, and preparing your input and output files.
Choosing the Right Programming Language
Binary manipulation assignments can be solved using various programming languages, including C, C++, Python, and Java. Each language has its syntax and functions for performing bitwise operations. Choose a language you are comfortable with and that supports efficient binary manipulation.
Setting Up Your Development Environment
Setting up your development environment involves installing the necessary software and configuring your IDE (Integrated Development Environment). Ensure you have a text editor or IDE that supports the programming language you have chosen. Additionally, make sure you have access to a compiler or interpreter for running your code.
Preparing Input and Output Files
Binary manipulation assignments often involve reading input from a file and writing output to a file. Prepare your input file with the necessary commands and operands, and ensure your program can read from the file and process the data correctly. Similarly, configure your program to write the results to an output file.
Implementing Bitwise Operations
Implementing bitwise operations involves writing functions for each operation and ensuring they handle the binary operands correctly. This section will provide example functions for bitwise NOT, AND, and OR operations.
Implementing Bitwise NOT
The bitwise NOT operation inverts each bit in a binary number. Here is an example function in C++ for performing the bitwise NOT operation:
void bitwiseNOT(int binary[8], int result[8]) { for (int i = 0; i < 8; i++) { result[i] = binary[i] == 0 ? 1 : 0; } }
This function takes an array representing an 8-bit binary number and produces the inverted result.
Implementing Bitwise AND
The bitwise AND operation compares each bit of two binary numbers and produces the result. Here is an example function in C++ for performing the bitwise AND operation:
void bitwiseAND(int binary1[8], int binary2[8], int result[8]) { for (int i = 0; i < 8; i++) { result[i] = binary1[i] & binary2[i]; } }
This function takes two arrays representing 8-bit binary numbers and produces the AND result.
Implementing Bitwise OR
The bitwise OR operation compares each bit of two binary numbers and produces the result. Here is an example function in C++ for performing the bitwise OR operation:
void bitwiseAND(int binary1[8], int binary2[8], int result[8]) {
for (int i = 0; i < 8; i++) {
result[i] = binary1[i] & binary2[i];
}
}
This function takes two arrays representing 8-bit binary numbers and produces the OR result.
Converting Binary to Decimal
Converting an 8-bit binary number to a decimal (base-10) number involves calculating the sum of each bit multiplied by 2 raised to the power of its position. Here is an example function in C++ for converting a binary number to decimal:
void bitwiseOR(int binary1[8], int binary2[8], int result[8]) {
for (int i = 0; i < 8; i++) {
result[i] = binary1[i] | binary2[i];
}
}
This function takes an array representing an 8-bit binary number and returns the decimal equivalent.
Performing Bit Shifting
Bit shifting involves moving bits to the left or right in a binary number. For left shifting, each bit is moved to the left by N positions, and bits "pushed off" the left end are discarded, with 0s filling the vacated positions on the right. Here is an example function in C++ for performing a left shift:
int convertToDecimal(int binary[8]) {
int decimal = 0;
for (int i = 0; i < 8; i++) {
decimal += binary[i] * (1 << (7 - i)); // 1 << (7 - i) is equivalent to 2^(7 - i)
}
return decimal;
}
This function takes an array representing an 8-bit binary number, a shift amount, and produces the left-shifted result.
Handling File Input and Output
Handling file input and output is a critical part of solving binary manipulation assignments. This section will guide you through reading commands and operands from a file and writing the results to an output file.
Reading Input from a File
To read input from a file, open the file and read each line to extract the command and operands. Here is an example function in C++ for reading input from a file:
#include <fstream>
#include <iostream>
#include <string>
void readInput(const std::string& filename) {
std::ifstream inputFile(filename);
if (!inputFile.is_open()) {
std::cerr << "Error: Could not open the file!" << std::endl;
exit(EXIT_FAILURE);
}
std::string command;
std::string operand1;
std::string operand2;
int shiftAmount;
while (inputFile >> command >> operand1 >> operand2 >> shiftAmount) {
// Process the command and operands
// Call the appropriate function based on the command
}
inputFile.close();
}
This function opens the input file, reads each line, and extracts the command and operands for further processing.
Writing Output to a File
To write the results to an output file, open the file and write the results in a clear format. Here is an example function in C++ for writing output to a file:
#include <fstream>
#include <iostream>
#include <string>
void writeOutput(const std::string& filename, const std::string& result) {
std::ofstream outputFile(filename);
if (!outputFile.is_open()) {
std::cerr << "Error: Could not open the file!" << std::endl;
exit(EXIT_FAILURE);
}
outputFile << result << std::endl;
outputFile.close();
}
This function opens the output file and writes the result to the file.
Example Program Structure
Here is an example structure of a C++ program that reads input from a file, performs binary manipulations, and writes the results to an output file:
#include <iostream>
#include <fstream>
#include <string>
void bitwiseNOT(int binary[8], int result[8]);
void bitwiseAND(int binary1[8], int binary2[8], int result[8]);
void bitwiseOR(int binary1[8], int binary2[8], int result[8]);
int convertToDecimal(int binary[8]);
void leftShift(int binary[8], int shiftAmount, int result[8]);
void readInput(const std::string& filename);
void writeOutput(const std::string& filename, const std::string& result);
int main() {
// Example usage
readInput("input.txt");
// Perform operations
writeOutput("output.txt", "Result of binary operations");
return 0;
}
This program provides a framework for reading input, performing binary manipulations, and writing the results.
Advanced Techniques and Best Practices
To excel in binary manipulation assignments, it's essential to adopt advanced techniques and best practices. This section will provide tips and strategies for mastering binary manipulations and handling common challenges.
Error Handling and Validation
Error handling and validation are crucial for ensuring your program handles invalid inputs and unexpected situations gracefully. Implement checks for valid commands, operands, and file operations. For example:
if (!inputFile.is_open()) {
std::cerr << "Error: Could not open the file!" << std::endl;
exit(EXIT_FAILURE);
}
This check ensures the file opens successfully, and if not, the program terminates with an appropriate error message.
Optimizing Performance
Optimizing performance involves writing efficient functions and minimizing unnecessary computations. For example, use bitwise operations instead of arithmetic operations for faster processing. Additionally, avoid redundant operations and use efficient data structures.
Handling Edge Cases
Consider edge cases when writing your functions. For example, when performing a left shift, handle cases where the shift amount is 0 or exceeds the number of bits. Similarly, handle cases where binary operands are of different lengths or contain invalid characters.
Practical Tips for Success
- Practice Regularly: Regular practice is essential for mastering binary manipulations. Solve various binary manipulation problems and experiment with different approaches.
- Understand the Concepts:Ensure you have a solid understanding of the underlying concepts, such as binary numbers, bitwise operations, and conversions.
- Use Debugging Tools: Use debugging tools to identify and fix issues in your code. Debugging helps you understand how your code executes and identify logical errors.
- Seek Help and Resources:Don't hesitate to seek help from professors, classmates, or online resources. Participate in programming communities and forums to exchange knowledge and ideas.
Conclusion
Mastering binary manipulation assignments requires a solid understanding of binary numbers, bitwise operations, and efficient programming techniques. This comprehensive guide provides the necessary knowledge and strategies to tackle binary manipulation assignments with confidence. By following the structured approach outlined in this guide, you can enhance your programming skills and gain a deeper insight into how digital systems process data at the binary level.