Claim Your Discount Today
Take your coding game to new heights with expert help at unbeatable prices. Got a tricky project or a tight deadline? We’ve got you covered! Use code PHHBF10 at checkout and save BIG. Don’t wait—this exclusive Black Friday deal won’t last long. Secure your academic success today!
We Accept
- The Importance of Visualization
- Objective
- Why JavaFX?
- Understanding Binary Search Trees (BSTs)
- Basic Operations on a BST:
- Setting Up Your JavaFX Environment
- 1. Install JavaFX SDK:
- 2. Configure Your IDE:
- 3. Set Up a New JavaFX Project:
- Creating the JavaFX Application
- Basic JavaFX Application Structure:
- Implementing the Binary Search Tree
- Binary Search Tree Implementation:
- Visualizing the BST with JavaFX
- TreePane Implementation:
- Adding Interactivity
- Adding Drag Support:
- Binding and Layout
- Final Touches and Enhancements
- Conclusion:
In computer science assignments visualizing data structures is a critical aspect, especially when it comes to understanding and optimizing data manipulation operations. One such fundamental structure is the Binary Search Tree (BST), which provides efficient data insertion, deletion, and retrieval through its hierarchical organization. Each node in a BST follows a strict ordering property: the left child’s value is less than the parent’s, and the right child’s value is greater.
This project involves creating a JavaFX application to visualize a Binary Search Tree. JavaFX, a powerful framework for building interactive graphical user interfaces in Java, is well-suited for this task. It allows us to create dynamic and visually appealing applications that can display complex data structures interactively.
The Importance of Visualization
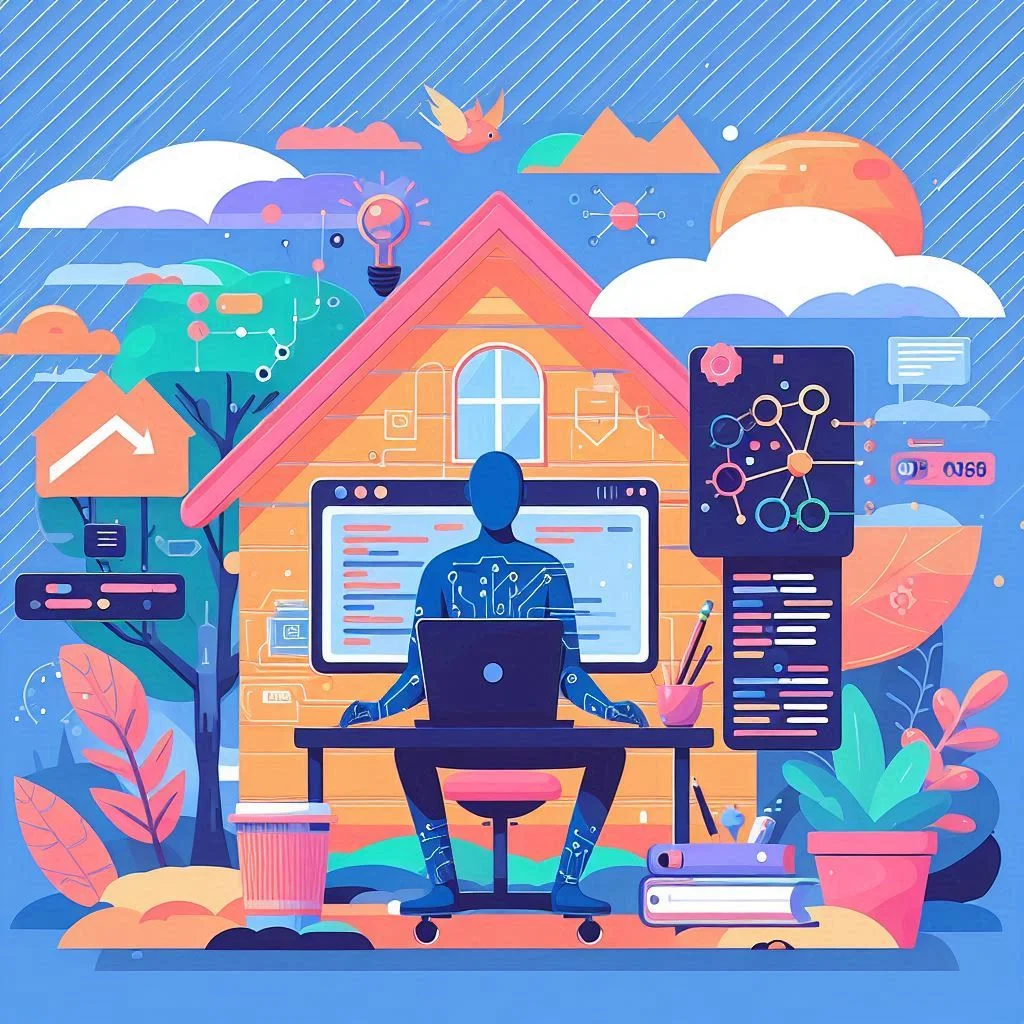
Visualizing a BST helps in understanding its structure and operations. By seeing how nodes are added, removed, and organized, you gain insights into the tree’s behavior and performance characteristics. This visualization is not only valuable for educational purposes but also for debugging and optimizing algorithms that manipulate trees.
Objective
The goal of this assignment is to create a JavaFX application that visualizes a Binary Search Tree. This project will involve:
- Setting up a JavaFX Environment: Preparing your development environment to work with JavaFX.
- Implementing the Binary Search Tree: Creating the data structure and its core operations.
- Visualizing the Tree: Using JavaFX to graphically represent the tree, including nodes and edges.
- Adding Interactivity: Allowing users to interact with the tree by adding nodes and dragging them.
- Enhancing Functionality: Implementing additional features to improve the visualization and user experience.
Why JavaFX?
JavaFX is a powerful framework for building rich graphical user interfaces in Java. It provides a range of features for creating interactive and visually appealing applications. By using JavaFX, you can create a dynamic visualization of the BST that not only shows the tree structure but also allows users to interact with it in real-time.
Understanding Binary Search Trees (BSTs)
A Binary Search Tree (BST) is a type of binary tree where each node follows a specific order. In a BST:
- Each node has up to two children: a left child and a right child.
- The left child’s value is less than the parent node’s value.
- The right child’s value is greater than the parent node’s value.
This ordering property ensures that searching for a value in a BST is efficient, typically O(log n) for balanced trees, because at each step you halve the number of nodes you need to consider.
Basic Operations on a BST:
- Insertion: Add a new node to the tree while maintaining the BST property.
- Search: Find a node with a given value.
- Deletion: Remove a node and rearrange the tree to maintain the BST property.
In this JavaFX assignment, we’ll focus on how to visualize these operations using JavaFX, particularly insertion, as it will give you a foundational understanding of how BSTs are represented and manipulated graphically.
Setting Up Your JavaFX Environment
Before starting with the JavaFX application, ensure that your development environment is correctly set up:
1. Install JavaFX SDK:
- Download the JavaFX SDK from the official website.
- Follow the installation instructions provided for your operating system.
2. Configure Your IDE:
- If you’re using an IDE like IntelliJ IDEA or Eclipse, ensure you’ve added JavaFX libraries to your project.
- In IntelliJ IDEA, you can do this by going to File -> Project Structure -> Libraries and adding the JavaFX SDK.
3. Set Up a New JavaFX Project:
- Create a new Java project in your IDE.
- Ensure you have the necessary JavaFX dependencies included in your build path.
Creating the JavaFX Application
Let’s start by creating a simple JavaFX application that will serve as the basis for our BST visualization. This application will set up the primary stage and scene for displaying our tree.
Basic JavaFX Application Structure:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.Pane;
import javafx.stage.Stage;
public class TreeVisualizer extends Application {
@Override
public void start(Stage primaryStage) {
Pane pane = new Pane();
TreePane treePane = new TreePane();
pane.getChildren().add(treePane);
Scene scene = new Scene(pane, 1024, 640);
primaryStage.setScene(scene);
primaryStage.setTitle("Binary Search Tree Visualizer");
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Explanation:
- start Method: This method is called when the application starts. It sets up the primary stage (the main window) and creates a Pane which serves as the root container for our visual components.
- TreePane Class: This class, which we'll define next, will handle the visualization of our BST. It extends Pane to allow us to add graphical components like circles and lines.
- Scene and Stage: The Scene represents the content of the window, while the Stage is the actual window itself. We set the scene on the stage and display it.
Implementing the Binary Search Tree
To visualize the BST, we need to first implement the BST data structure itself. This class will handle the tree operations such as insertion.
Binary Search Tree Implementation:
import java.util.Random;
public class BinarySearchTree<T extends Comparable<T>> {
private Node<T> root;
private class Node<T> {
T value;
Node<T> left, right;
// Add properties for visual representation
Circle circle;
Line edge;
Node(T value) {
this.value = value;
this.left = null;
this.right = null;
}
}
public boolean add(T value) {
if (root == null) {
root = new Node<>(value);
return true;
}
Node<T> current = root;
Node<T> parent = null;
while (true) {
parent = current;
int cmp = value.compareTo(current.value);
if (cmp < 0) {
current = current.left;
if (current == null) {
parent.left = new Node<>(value);
return true;
}
} else if (cmp > 0) {
current = current.right;
if (current == null) {
parent.right = new Node<>(value);
return true;
}
} else {
// Value already exists
return false;
}
}
}
public Node<T> getRoot() {
return root;
}
}
Explanation:
1. Node Class: This inner class represents each node in the BST. It includes:
- value: The data stored in the node.
- left and right: Pointers to the left and right children.
- circle and edge: JavaFX components for visualization.
2. add Method: This method inserts a new value into the BST. It handles both empty trees (by setting the root) and non-empty trees (by finding the correct insertion point).
Visualizing the BST with JavaFX
To visualize the BST, extend the Pane class to create a custom pane that will draw the tree structure. This pane will handle drawing nodes and edges, as well as updating their positions.
TreePane Implementation:
import javafx.scene.layout.Pane;
import javafx.scene.shape.Circle;
import javafx.scene.shape.Line;
import javafx.scene.text.Text;
import javafx.scene.input.MouseButton;
import javafx.scene.input.MouseEvent;
import javafx.scene.paint.Color;
import java.util.Random;
public class TreePane extends Pane {
private BinarySearchTree<Integer> tree = new BinarySearchTree<>();
private Random rand = new Random();
public TreePane() {
this.setOnMouseClicked(this::handleMouseClick);
}
private void handleMouseClick(MouseEvent event) {
if (event.getButton() == MouseButton.SECONDARY) {
int value = rand.nextInt(Integer.MAX_VALUE);
if (tree.add(value)) {
visualizeNode(tree.getRoot());
}
}
}
private void visualizeNode(BinarySearchTree.Node<Integer> node) {
if (node == null) return;
Circle circle = new Circle(20);
circle.setFill(Color.WHITE);
circle.setStroke(Color.BLACK);
Text text = new Text(node.value.toString());
text.setFont(new javafx.scene.text.Font(16));
text.xProperty().bind(circle.centerXProperty().add(10));
text.yProperty().bind(circle.centerYProperty().subtract(10));
this.getChildren().addAll(circle, text);
if (node.left != null) {
drawEdge(node, node.left, circle);
visualizeNode(node.left);
}
if (node.right != null) {
drawEdge(node, node.right, circle);
visualizeNode(node.right);
}
}
private void drawEdge(BinarySearchTree.Node<Integer> parent, BinarySearchTree.Node<Integer> child, Circle parentCircle) {
Line edge = new Line();
edge.startXProperty().bind(parentCircle.centerXProperty());
edge.startYProperty().bind(parentCircle.centerYProperty());
// Position edge end based on child position
// Placeholder values
edge.setEndX(parentCircle.getCenterX() + 50);
edge.setEndY(parentCircle.getCenterY() + 50);
edge.setStroke(Color.BLACK);
this.getChildren().add(edge);
}
}
Explanation:
- handleMouseClick Method: This method handles mouse clicks. If the user right-clicks, a new random value is added to the BST, and the tree is visualized.
- visualizeNode Method: This method recursively visualizes each node in the BST. It creates a Circle and Text for each node, and updates their positions. It also recursively visualizes child nodes.
- drawEdge Method: This method draws a Line connecting a parent node to a child node. The positions of the start and end of the line are bound to the positions of the circles representing the nodes.
Adding Interactivity
To make the visualization interactive, you need to allow users to drag nodes around. This involves handling mouse drag events and updating the positions of nodes and edges accordingly.
Adding Drag Support:
private void addDragSupport(Circle circle) {
circle.setOnMousePressed(event -> {
circle.setUserData(new double[] {event.getX(), event.getY()});
});
circle.setOnMouseDragged(event -> {
double[] startPosition = (double[]) circle.getUserData();
double deltaX = event.getX() - startPosition[0];
double deltaY = event.getY() - startPosition[1];
circle.setCenterX(circle.getCenterX() + deltaX);
circle.setCenterY(circle.getCenterY() + deltaY);
// Update edge positions if necessary
updateEdges(circle);
});
}
private void updateEdges(Circle circle) {
// Implement logic to update edge positions based on the new position of the circle
}
Explanation:
- addDragSupport Method: This method adds mouse event handlers to support dragging of nodes. It updates the position of the node (circle) based on the mouse drag events.
- updateEdges Method: This method (to be implemented) updates the positions of edges when a node is dragged. It ensures that the edges follow the nodes as they are moved.
Binding and Layout
JavaFX provides property bindings that simplify aligning and positioning elements relative to each other. Use these bindings to ensure that text labels remain centered around their respective circles.
Binding Example:
text.xProperty().bind(circle.centerXProperty().subtract(text.getLayoutBounds().getWidth() / 2));
text.yProperty().bind(circle.centerYProperty().add(text.getLayoutBounds().getHeight() / 4));
Explanation:
- Binding Text to Circle: The text position is bound to the circle’s position so that the text stays centered around the circle. Adjust the offsets as needed to ensure proper alignment.
Final Touches and Enhancements
After implementing the core functionality, consider these additional enhancements:
- Edge Adjustment: Refine edge drawing and updating logic to ensure lines accurately connect nodes and adjust when nodes are dragged.
- User Interface Improvements: Add features like zooming or panning to handle larger trees, and include controls for node operations (e.g., deletion, searching).
- Error Handling: Implement error handling and edge cases, such as dealing with nodes outside the visible area or invalid inputs.
- Performance Optimization: Optimize the rendering and updating logic for better performance, especially with larger trees or complex visualizations.
Conclusion:
Building a JavaFX application to visualize a Binary Search Tree (BST) offers a valuable and interactive approach to both understanding data structures and honing GUI programming skills. This JavaFX assignment not only enhances your grasp of tree structures but also provides practical experience in developing dynamic, user-friendly applications.
By completing this programming assignment, you'll gain hands-on experience in setting up a JavaFX environment, visualizing nodes, enabling user interactions, and dynamically updating the tree. Testing your application thoroughly and iterating based on feedback will further refine your project and demonstrate your ability to handle real-world programming challenges.
This project is beneficial not just for academic purposes but also for real-world applications. Feel free to experiment with different styles, colors, and functionalities to enhance your JavaFX application. Visualizing data through such data structure assignments makes learning both effective and engaging.