Claim Your Offer
New semester, new challenges—but don’t stress, we’ve got your back! Get expert programming assignment help and breeze through Python, Java, C++, and more with ease. For a limited time, enjoy 10% OFF on all programming assignments with the Spring Special Discount! Just use code SPRING10OFF at checkout! Why stress over deadlines when you can score high effortlessly? Grab this exclusive offer now and make this semester your best one yet!
We Accept
In the world of programming, pseudocode serves as a bridge between the problem statement and the actual code implementation. Writing pseudocode is a highly effective technique that simplifies the problem-solving process, especially for complex assignments. This blog will delve into the significance of pseudocode, how it aids students in breaking down challenging assignments, and the key steps to writing effective pseudocode.
Whether you're working with a large data set, solving a complex algorithmic problem, or simply trying to make your programming journey easier, pseudocode provides an organized and straightforward framework. Let's explore how it can enhance your efficiency and accuracy in solving programming assignments.
Why Pseudocode is Essential for Programming Assignments
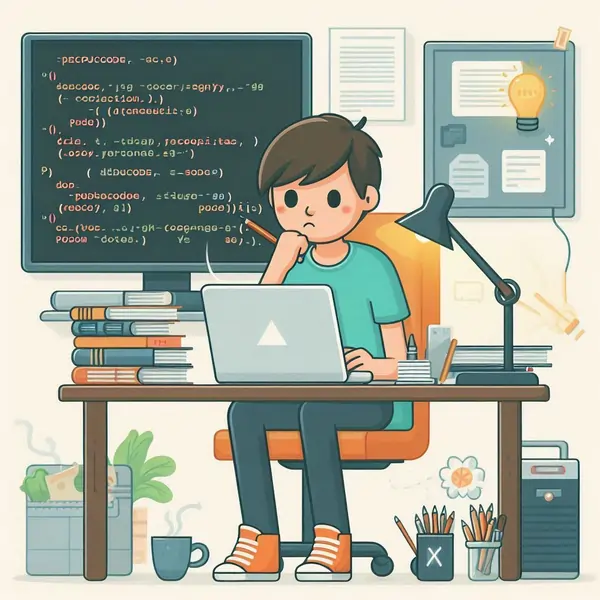
- Clarifies Complex Logic: Pseudocode helps to lay out the logic in a simplified manner without diving into syntax specifics. This makes it easier for students to grasp complex concepts and algorithms.
- Reduces Errors in Code Implementation: Writing pseudocode allows you to map out a clear plan, reducing the likelihood of errors in the code. Since you’ve already thought through the steps, you’re less likely to make logical errors.
- Improves Collaboration and Communication: Especially in academic settings, pseudocode is easier to understand for peers, tutors, or collaborators. This makes teamwork smoother, as pseudocode provides a shared, language-agnostic approach to problem-solving.
- Boosts Confidence for Beginners: For beginners, pseudocode is a confidence-booster. By practicing this approach, students can focus on the problem-solving aspect without feeling overwhelmed by language syntax.
Key Steps to Writing Effective Pseudocode
- Understand the Problem: Before diving into pseudocode, ensure you have a solid grasp of the assignment requirements. Break down the problem statement to pinpoint the inputs, outputs, and overall goal.
- Identify Major Steps: Outline the primary actions needed to solve the problem. In this phase, you’re breaking down the task into manageable pieces.
- Use Plain Language: Write each step in simple, plain language. Avoid technical jargon; pseudocode should read more like structured instructions than programming language syntax.
- Review and Refine: After completing your pseudocode, review it. Does it solve the problem efficiently? Are there any redundancies? This is where you can refine your logic.
- Translate to Code: Once you’re confident in your pseudocode, it’s time to convert it to the actual programming language. This should be a relatively smooth process if the pseudocode is well-written.
Examples of Pseudocode for Popular Problems
To provide a clearer picture, let's look at pseudocode examples for some commonly encountered programming problems.
Example 1: Sorting an Array Using Bubble SortProblem Statement: Write a pseudocode for sorting an array using the Bubble Sort algorithm.
Pseudocode:
BEGIN
INPUT array
SET n to the length of array
FOR each i from 0 to n-1
FOR each j from 0 to n-i-1
IF array[j] > array[j+1] THEN
SWAP array[j] and array[j+1]
END IF
END FOR
END FOR
OUTPUT sorted array
END
Explanation: In this example, the pseudocode defines a simple process where we iterate through the array multiple times, comparing adjacent elements and swapping them if they’re out of order. This process continues until the entire array is sorted. By following this structured approach, a student can translate it into any programming language.
Example 2: Searching an Element Using Binary SearchProblem Statement: Write pseudocode to search for a specific value in a sorted array using the Binary Search algorithm.
Pseudocode:
BEGIN
INPUT sorted array and target value
SET left to 0
SET right to length of array - 1
WHILE left <= right
SET mid to (left + right) / 2
IF array[mid] equals target THEN
RETURN "Target found at index" mid
ELSE IF array[mid] < target THEN
SET left to mid + 1
ELSE
SET right to mid - 1
END IF
END WHILE
RETURN "Target not found"
END
Explanation: Binary search is an efficient algorithm for finding a target value in a sorted array by repeatedly dividing the search interval in half. This pseudocode provides a clear and concise roadmap, making the logic easier to translate into programming language syntax.
Example 3: Calculating Factorial of a Number (Recursive Approach)Problem Statement: Write pseudocode to calculate the factorial of a number using recursion.
Pseudocode:
FUNCTION factorial(n)
IF n == 0 THEN
RETURN 1
ELSE
RETURN n * factorial(n - 1)
END IF
END FUNCTION
Explanation: The pseudocode above leverages recursion to calculate the factorial of a given number. It includes a base case to stop the recursion when nnn is 0, and a recursive case where the function calls itself with a decremented value of nnn. By breaking down this logic in pseudocode, students can better understand how recursion operates without getting bogged down in syntax.
Example 4: Checking if a String is a PalindromeProblem Statement: Write pseudocode to check if a given string is a palindrome.
Pseudocode:
BEGIN
INPUT string
SET left to 0
SET right to length of string - 1
WHILE left < right
IF string[left] does not equal string[right] THEN
RETURN "Not a palindrome"
END IF
INCREMENT left by 1
DECREMENT right by 1
END WHILE
RETURN "Is a palindrome"
END
Explanation: This pseudocode illustrates a straightforward algorithm to check if a string is a palindrome by comparing characters from both ends. It’s an example of a problem where pseudocode can clarify how we approach the comparison step-by-step. This approach is straightforward to implement in various programming languages.
Example 5: Merge Sort AlgorithmProblem: Sort an array using the Merge Sort algorithm.
Solution: Merge Sort is a recursive algorithm that divides the array into two halves, sorts each half, and then merges them.
Pseudocode:
START
Function MergeSort(array)
If length(array) <= 1
Return array
EndIf
Set mid to length(array) / 2
Set left to MergeSort(array from 0 to mid)
Set right to MergeSort(array from mid to length(array))
Return Merge(left, right)
EndFunction
Function Merge(left, right)
Set result to empty array
While left and right are not empty
If left[0] <= right[0]
Append left[0] to result
Remove left[0]
Else
Append right[0] to result
Remove right[0]
EndIf
EndWhile
Append any remaining elements in left to result
Append any remaining elements in right to result
Return result
EndFunction
END
This pseudocode for Merge Sort is a powerful tool for students who want to approach recursive problems methodically. It clarifies how recursive calls and merging work, laying the groundwork for successful coding.
Benefits of Writing Pseudocode Before Coding
- Increased Accuracy in Code Implementation
By planning out each step with pseudocode, students can reduce their chances of logical errors, ensuring the final code aligns with the intended solution.
- Better Time Management
When you use pseudocode as a roadmap, you save time debugging and rewriting sections of code. This methodical approach can lead to faster, more accurate programming assignment completion.
- Enhanced Problem-Solving Skills
Pseudocode strengthens students' problem-solving abilities, as it encourages logical thinking and algorithmic planning. Over time, students become more adept at visualizing complex programming tasks.
- Applicable Across All Languages
Pseudocode isn’t language-specific, which means students can apply it across different programming languages, making it a valuable skill for programmers of all levels.
Conclusion
Incorporating pseudocode into your problem-solving routine can make a world of difference in handling complex programming assignments. By breaking down the problem into simpler, digestible steps, pseudocode acts as a valuable guide that simplifies coding and enhances understanding. For students seeking programming assignment help or online programming assignment help, mastering pseudocode can lead to better grades and a more efficient coding process.
If you’re looking to elevate your programming assignments and take a step toward writing better code, start using pseudocode today. It’s a small change that yields significant benefits, especially as programming tasks become more challenging.