Claim Your Discount Today
Kick off the fall semester with a 20% discount on all programming assignments at www.programminghomeworkhelp.com! Our experts are here to support your coding journey with top-quality assistance. Seize this seasonal offer to enhance your programming skills and achieve academic success. Act now and save!
We Accept
- Understanding the Problem Statement
- Reading the Matrix from a File
- Finding the Path
- Printing the Path
- Breaking Down the Requirements
- Reading the Matrix from a File
- Implementing the Pathfinding Algorithm
- Printing the Path
- Step-by-Step Implementation
- Reading the Matrix from a File
- Implementing the Pathfinding Algorithm
- Printing the Path
- Testing and Debugging
- Test with Different Cases
- Debugging
- Conclusion
Matrix pathfinding assignments are a staple in programming education, often appearing in coursework and technical interviews. These problems require navigating through a matrix to find a path that meets specific criteria. Whether you're seeking help with algorithm assignment tasks or simply looking to improve your problem-solving skills, the principles discussed here will be valuable. We will use a specific example to illustrate the concepts, but the principles can be applied to any similar assignment. In this blog, we will delve into the strategies and techniques needed to solve such assignments effectively. We will use a specific example to illustrate the concepts, but the principles can be applied to any similar assignment.
Understanding the Problem Statement
The first step in tackling any programming assignment is to thoroughly understand the problem statement. Let's dissect the example problem provided:
Reading the Matrix from a File
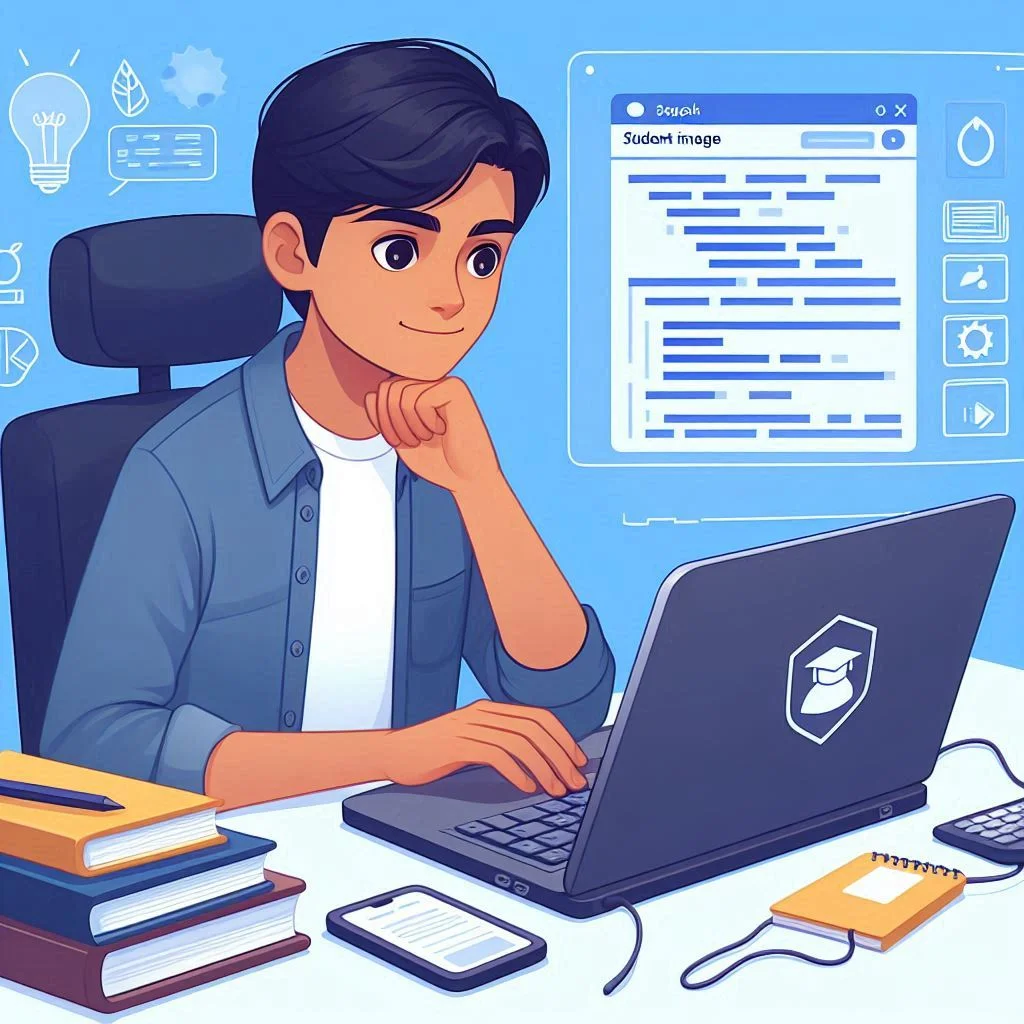
Matrix pathfinding assignments often involve reading data from a file. Here, the file contains a matrix of numbers with the first two lines specifying the number of rows and columns. Our goal is to store these values in a data structure that allows efficient manipulation and pathfinding.
Finding the Path
The core of the assignment is to find a continuous path through the matrix. The path must start and end at a zero, the sum of the path must be greater than zero, and the path can only move in four directions: up, down, left, and right. Additionally, the path cannot revisit any position.
Printing the Path
Once a valid path is found, it needs to be printed in a specified format. This involves using characters to represent the direction of movement and marking the start and end points clearly.
Breaking Down the Requirements
Breaking down the problem into manageable tasks is crucial for solving it efficiently. We can categorize the requirements into four main tasks: reading the matrix, storing the matrix, finding the path, and printing the path. Each of these tasks can be further divided into sub-tasks, which we will explore in detail.
Reading the Matrix from a File
Parsing the File
The first step is to read the matrix dimensions and values from the file. This involves opening the file, reading the first two lines to get the dimensions, and then reading the subsequent lines to populate the matrix.
Storing the Matrix
Once the matrix values are read, they need to be stored in a data structure. A 2D vector (or a list of lists in Python) is a suitable choice for this task, as it allows easy access and manipulation of the matrix elements.
Implementing the Pathfinding Algorithm
Recursive Function for Pathfinding
The heart of the assignment is the pathfinding algorithm. A recursive function is a natural choice for this task, as it can explore all possible paths from the starting zero to the ending zero. The function needs to keep track of the visited positions to avoid cycles and ensure that the path's sum is greater than zero.
Backtracking to Ensure Constraints
Backtracking is essential to ensure that the path adheres to the constraints. If a path does not meet the criteria, the algorithm should backtrack and explore other possibilities.
Printing the Path
Marking the Path
Once a valid path is found, the matrix should be updated to mark the path. Specific characters are used to represent the direction of movement, and the start and end points are clearly marked.
Displaying the Matrix
Finally, the matrix with the marked path is printed. This involves iterating through the matrix and printing the appropriate characters for each position.
Step-by-Step Implementation
Let's implement the solution step-by-step, starting with reading the matrix from the file and ending with printing the marked path.
Reading the Matrix from a File
Parsing the File
def read_matrix(filename):
with open(filename, 'r') as file:
rows = int(file.readline().strip())
cols = int(file.readline().strip())
matrix = []
for _ in range(rows):
matrix.append(list(map(int, file.readline().strip().split())))
return matrix, rows, cols
In this code snippet, we open the file, read the number of rows and columns, and then read the matrix values into a list of lists.
Storing the Matrix
We store the matrix in a 2D list, which allows us to easily access and manipulate the elements. This structure is ideal for implementing the pathfinding algorithm.
Implementing the Pathfinding Algorithm
Recursive Function for Pathfinding
def find_path(matrix, start, end, path=[], visited=set()):
if start == end:
return path + [end]
x, y = start
rows, cols = len(matrix), len(matrix[0])
directions = [(-1, 0), (1, 0), (0, -1), (0, 1)]
for dx, dy in directions:
nx, ny = x + dx, y + dy
if 0 <= nx < rows and 0 <= ny < cols and (nx, ny) not in visited:
if matrix[nx][ny] != 0 or (nx, ny) == end:
new_path = find_path(matrix, (nx, ny), end, path + [start], visited | {(nx, ny)})
if new_path:
return new_path
return None
In this recursive function, we explore all possible paths from the start position to the end position. The function checks if the current position is valid and has not been visited before. If a valid path is found, it returns the path; otherwise, it backtracks and explores other possibilities.
Backtracking to Ensure Constraints
Backtracking is achieved by marking the current position as visited and removing it from the visited set if the path does not meet the criteria. This ensures that the algorithm explores all possible paths while adhering to the constraints.
Printing the Path
Marking the Path
def print_path(matrix, path):
path_set = set(path)
for i in range(len(matrix)):
for j in range(len(matrix[0])):
if (i, j) in path_set:
if (i, j) == path[0]:
print(determine_start_direction(path), end=' ')
elif (i, j) == path[-1]:
print('X', end=' ')
else:
print(get_direction_symbol(path, i, j), end=' ')
else:
print('-', end=' ')
print()
In this function, we iterate through the matrix and print the appropriate characters for each position. The start and end points are clearly marked, and the direction of movement is indicated using specific symbols.
Displaying the Matrix
The final step is to print the matrix with the marked path. This involves iterating through the matrix and printing each row with the appropriate characters.
Testing and Debugging
Test with Different Cases
To ensure the program works correctly, it is essential to test it with various matrix sizes and values. This helps to identify any edge cases and verify that the pathfinding algorithm handles different scenarios.
Debugging
If the program does not work as expected,def print_path(matrix, path):
path_set = set(path)
for i in range(len(matrix)):
for j in range(len(matrix[0])):
if (i, j) in path_set:
if (i, j) == path[0]:
print(determine_start_direction(path), end=' ')
elif (i, j) == path[-1]:
print('X', end=' ')
else:
print(get_direction_symbol(path, i, j), end=' ')
else:
print('-', end=' ')
print()
use print statements or a debugger to trace the function calls and identify any issues. Check the values of variables at different stages of the execution to pinpoint the problem.
Conclusion
Solving matrix pathfinding assignments requires a systematic approach and careful attention to detail. By breaking down the problem into manageable tasks, planning your approach, and methodically implementing the solution, you can effectively tackle these assignments. Remember to test thoroughly and refine your approach based on feedback and test results.
Completing your programming assignments can be challenging, but with practice and persistence, you can master them. Use this guide as a reference to help you navigate through similar assignments and build your problem-solving skills. Good luck with your programming assignments!