Claim Your Discount Today
Take your coding game to new heights with expert help at unbeatable prices. Got a tricky project or a tight deadline? We’ve got you covered! Use code PHHBF10 at checkout and save BIG. Don’t wait—this exclusive Black Friday deal won’t last long. Secure your academic success today!
We Accept
Java is a powerful and versatile programming language, making it an excellent choice for building applications, including multiplayer games. For college assignments, especially at the graduate level, creating a multiplayer game showcases your understanding of Java's capabilities in networking, threading, and logic implementation.
In this blog, we’ll provide a step-by-step guide to help you create a basic multiplayer game using Java. We'll delve into key concepts, provide real-world code snippets, and highlight common challenges. If you're still unsure about implementing these ideas or find it challenging, remember you can always seek programming assignment help or pay someone to do my Java assignment to assist with your project.
Understanding Multiplayer Game Basics
Before diving into coding, let’s explore the fundamental architecture of a multiplayer game. A typical multiplayer game uses the following components:
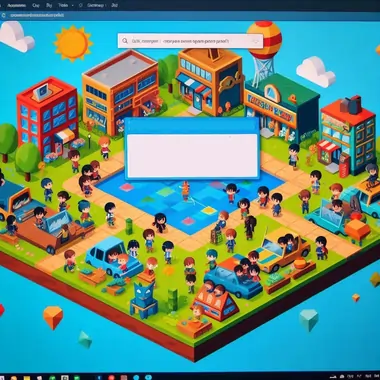
- Client-Server Model
- Server: Manages the game's logic, player connections, and data synchronization.
- Client: Represents individual players, interacting with the game through input/output.
- Networking
Networking is key in multiplayer games. In Java, the Socket and ServerSocket classes enable real-time communication between clients and the server.
- Game Logic
This governs player actions, rules, and responses, ensuring a smooth gameplay experience.
- Threading
A multiplayer game must handle multiple players simultaneously. Threads help manage concurrent connections.
Step-by-Step Guide to Build a Multiplayer Game
Let’s create a simple multiplayer number-guessing game in Java.
Step 1: Set Up Your Project
Start by creating a new Java project. You’ll need three primary classes:
- GameServer: Manages client connections and game logic.
- GameClient: Connects to the server and interacts with the game.
- Main: Starts the game.
Step 2: Implement the Server
The server is the backbone of the game. It listens for client connections, receives input, processes game logic, and sends responses to clients.
Here’s how to create the server:
import java.io.*;
import java.net.*;
import java.util.*;
public class GameServer {
private static final int PORT = 12345;
private static Set<PrintWriter> clientWriters = new HashSet<>();
public static void main(String[] args) {
System.out.println("Game Server is starting...");
try (ServerSocket serverSocket = new ServerSocket(PORT)) {
while (true) {
new ClientHandler(serverSocket.accept()).start();
}
} catch (IOException e) {
System.err.println("Server Error: " + e.getMessage());
}
}
private static class ClientHandler extends Thread {
private Socket socket;
private PrintWriter out;
private BufferedReader in;
public ClientHandler(Socket socket) {
this.socket = socket;
}
public void run() {
try {
in = new BufferedReader(new InputStreamReader(socket.getInputStream()));
out = new PrintWriter(socket.getOutputStream(), true);
synchronized (clientWriters) {
clientWriters.add(out);
}
String message;
while ((message = in.readLine()) != null) {
broadcast(message);
}
} catch (IOException e) {
System.out.println("Connection Error: " + e.getMessage());
} finally {
try {
socket.close();
} catch (IOException e) {
System.out.println("Socket Close Error: " + e.getMessage());
}
synchronized (clientWriters) {
clientWriters.remove(out);
}
}
}
private void broadcast(String message) {
synchronized (clientWriters) {
for (PrintWriter writer : clientWriters) {
writer.println(message);
}
}
}
}
}
Step 3: Build the Client
The client connects to the server, sending inputs and receiving game updates.
import java.io.*;
import java.net.*;
public class GameClient {
private static final String SERVER_ADDRESS = "localhost";
private static final int PORT = 12345;
public static void main(String[] args) {
try (Socket socket = new Socket(SERVER_ADDRESS, PORT)) {
BufferedReader in = new BufferedReader(new InputStreamReader(socket.getInputStream()));
PrintWriter out = new PrintWriter(socket.getOutputStream(), true);
System.out.println("Connected to the server!");
BufferedReader console = new BufferedReader(new InputStreamReader(System.in));
new Thread(() -> {
try {
String response;
while ((response = in.readLine()) != null) {
System.out.println("Server: " + response);
}
} catch (IOException e) {
System.err.println("Disconnected from server.");
}
}).start();
String input;
while ((input = console.readLine()) != null) {
out.println(input);
}
} catch (IOException e) {
System.err.println("Error connecting to server: " + e.getMessage());
}
}
}
Step 4: Adding Game Logic
Now, let’s add a guessing game logic to the server. Players will try to guess a random number, and the server will provide feedback.
Modify the server’s broadcast method:
private static int secretNumber = new Random().nextInt(100) + 1;
private void broadcast(String message) {
if (message.startsWith("GUESS:")) {
int guess = Integer.parseInt(message.substring(6));
if (guess == secretNumber) {
for (PrintWriter writer : clientWriters) {
writer.println("Correct! The number was " + secretNumber);
}
resetGame();
} else if (guess < secretNumber) {
for (PrintWriter writer : clientWriters) {
writer.println("Too low! Try again.");
}
} else {
for (PrintWriter writer : clientWriters) {
writer.println("Too high! Try again.");
}
}
}
}
private void resetGame() {
secretNumber = new Random().nextInt(100) + 1;
for (PrintWriter writer : clientWriters) {
writer.println("New game started! Guess a number between 1 and 100.");
}
}
Step 5: Running and Testing
- Run the Server: Start the GameServer first.
- Connect Clients: Launch multiple instances of GameClient.
- Play the Game: Clients can guess the number, and the server provides feedback.
Benefits of Creating a Multiplayer Game
- Hands-on Networking Experience: Understand how Socket programming works in Java.
- Game Development Skills: Learn to design and implement game logic.
- Collaborative Features: Add features like leaderboards, score tracking, or timers to enhance your project.
Overcoming Challenges
- Managing Threads: Ensure proper synchronization to avoid race conditions.
- Error Handling: Add comprehensive exception handling for network errors.
- Scalability: Test with multiple clients to ensure stable performance.
Final Thoughts
Creating a multiplayer game in Java is a fantastic way to enhance your programming skills and impress your professors. This blog walked you through the basics of setting up a game server, implementing client connections, and adding game logic.
Remember, if you’re short on time or find Java assignments overwhelming, you can always pay someone to do my Java assignment through reliable platforms like ProgrammingHomeworkHelp.com. With the right resources and support, you’ll be acing your assignments in no time.