Claim Your Discount Today
Ring in Christmas and New Year with a special treat from www.programminghomeworkhelp.com! Get 15% off on all programming assignments when you use the code PHHCNY15 for expert assistance. Don’t miss this festive offer—available for a limited time. Start your New Year with academic success and savings. Act now and save!
We Accept
- Understanding the Project Scenario
- Key Entities and Attributes
- Requirements Breakdown
- Database Design
- 1. Schema Design
- 2. Relationships
- Implementing Procedures
- 1. Adding Records
- 2. Managing Properties
- Implementing Triggers
- 1. Updating Sales
- 2. Logging Price Changes
- Testing Procedures and Triggers
- 1. Testing Procedures
- 2. Testing Triggers
- Optimization and Security
- 1. Indexing
- 2. Constraints
- 3. Security
- Advanced Considerations
- 1. Data Normalization
- 2. Transaction Management
- 3. Performance Tuning
- Conclusion
Data has become the backbone of every industry, and real estate is no exception. A Real Estate Database Management System (REDMS) is a powerful tool that aids real estate professionals in managing vast amounts of data efficiently. From listing properties to tracking customer interactions, a robust REDMS can significantly enhance productivity, streamline operations, and ultimately drive business growth.
These assignments often require designing databases, writing stored procedures, and implementing triggers to ensure data integrity and functionality. This guide will walk you through the process of addressing such assignments, using a real estate management system as an example. By understanding the core concepts and strategies, you will be better prepared to solve your database assignment.
Understanding the Project Scenario
In the context of a real estate firm, you are tasked with creating a database system to manage various aspects of the business. This involves handling entities such as firms, properties, agents, and rooms, each with its specific attributes and relationships.
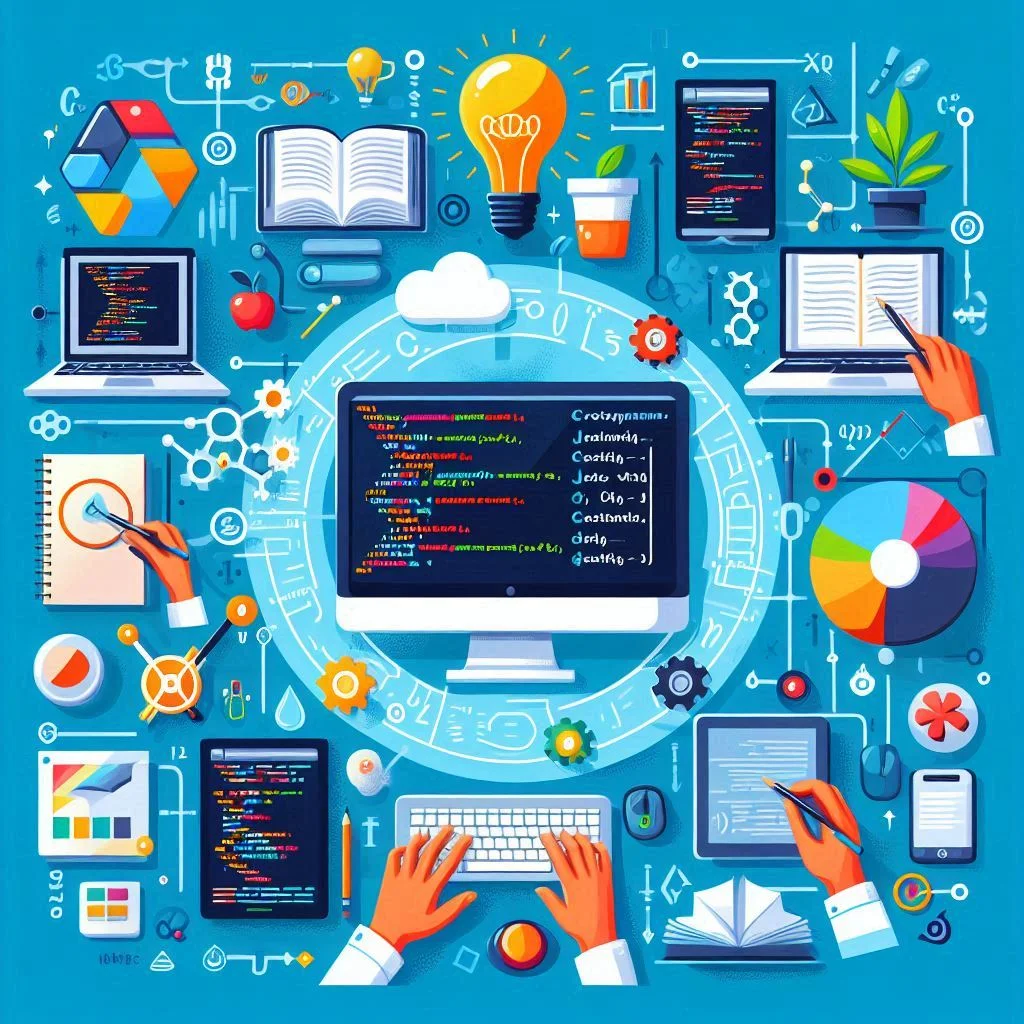
Key Entities and Attributes
1. Real Estate Firms:
- Attributes: FirmID (Primary Key), Name, Address, Phone Number, Type (Residential or Commercial).
- Relationships: A firm can have many properties and agents.
2. Properties:
- Attributes: PropertyID (Primary Key), FirmID (Foreign Key), Address, Price, Size, Status (Sold or Available), Construction Date, Type (Apartment, Condo, Single Family).
- Relationships: A property is listed by one firm and can have multiple rooms. It may be assigned to one or no agent.
3. Rooms:
- Attributes: RoomID (Primary Key), PropertyID (Foreign Key), Room Type (Bathroom, Bedroom, Living Room), Size, Flooring Type (Carpet, Wood, Tile).
- Relationships: A room belongs to one property.
4. Agents:
- Attributes: AgentID (Primary Key), FirmID (Foreign Key), First Name, Last Name (Unique), Address, Salary, Total Sales.
- Relationships: An agent works for one firm and may be assigned to multiple properties.
Requirements Breakdown
1. Adding Records:
- Procedures to add new firms, properties, rooms, and agents.
- Ensure no duplicates and validate data.
2. Managing Properties:
- Procedures for updating property status, price, and querying properties based on state.
3. Triggers:
- Automatic actions to update agent sales when a property is sold.
- Logging changes in property prices.
Database Design
1. Schema Design
Creating a robust schema involves defining tables, their attributes, and relationships. Here’s a detailed look at each table:
Firms Table
The Firms table will store information about each real estate firm.
Attributes:
- FirmID: A unique identifier for each firm.
- Name: The name of the firm.
- Address: The firm's address.
- PhoneNumber: The firm's contact number.
- Type: Indicates whether the firm is Residential or Commercial.
SQL Definition:
CREATE TABLE Firms (
FirmID INT AUTO_INCREMENT PRIMARY KEY,
Name VARCHAR(100) NOT NULL UNIQUE,
Address VARCHAR(255),
PhoneNumber VARCHAR(20),
Type ENUM('Residential', 'Commercial') NOT NULL
);
Properties Table
The Properties table will contain details about each property listed by the firm.
Attributes:
- PropertyID: A unique identifier for each property.
- FirmID: A foreign key linking to the Firms table.
- Address: The property’s address.
- Price: The asking price of the property.
- Size: Total size of the property in square feet.
- Status: Current status (Sold or Available).
- ConstructionDate: Date when the property was constructed.
- Type: Type of property (Apartment, Condo, Single Family).
SQL Definition:
CREATE TABLE Properties (
PropertyID INT AUTO_INCREMENT PRIMARY KEY,
FirmID INT,
Address VARCHAR(255),
Price DECIMAL(10,2),
Size INT,
Status ENUM('Sold', 'Available') DEFAULT 'Available',
ConstructionDate DATE,
Type ENUM('Apartment', 'Condo', 'Single Family'),
FOREIGN KEY (FirmID) REFERENCES Firms(FirmID)
);
Rooms Table
The Rooms table will detail each room within a property.
Attributes:
- RoomID: A unique identifier for each room.
- PropertyID: A foreign key linking to the Properties table.
- RoomType: Type of room (Bathroom, Bedroom, Living Room).
- Size: Size of the room in square feet.
- FlooringType: Type of flooring (Carpet, Wood, Tile).
SQL Definition:
CREATE TABLE Rooms (
RoomID INT AUTO_INCREMENT PRIMARY KEY,
PropertyID INT,
RoomType ENUM('Bathroom', 'Bedroom', 'Living Room'),
Size INT,
FlooringType ENUM('Carpet', 'Wood', 'Tile'),
FOREIGN KEY (PropertyID) REFERENCES Properties(PropertyID)
);
Agents Table
The Agents table will store information about each agent working for a firm.
Attributes:
- AgentID: A unique identifier for each agent.
- FirmID: A foreign key linking to the Firms table.
- FirstName: Agent’s first name.
- LastName: Agent’s last name (must be unique).
- Address: Agent’s address.
- Salary: Agent’s salary.
- TotalSales: Total amount of sales made by the agent.
SQL Definition:
CREATE TABLE Agents (
AgentID INT AUTO_INCREMENT PRIMARY KEY,
FirmID INT,
FirstName VARCHAR(50),
LastName VARCHAR(50) UNIQUE,
Address VARCHAR(255),
Salary DECIMAL(10,2),
TotalSales DECIMAL(10,2) DEFAULT 0,
FOREIGN KEY (FirmID) REFERENCES Firms(FirmID)
);
2. Relationships
Define the relationships between tables to maintain data integrity:
One-to-Many:
- A firm can list many properties.
- A property can have many rooms.
- An agent can be assigned to multiple properties.
Many-to-One:
- Each property belongs to one firm.
- Each room belongs to one property.
Implementing Procedures
1. Adding Records
Add a New Firm
To add a new firm, you need a procedure that ensures there are no duplicate entries and that the data is valid.
CREATE PROCEDURE AddFirm (
IN firmName VARCHAR(100),
IN firmAddress VARCHAR(255),
IN firmPhoneNumber VARCHAR(20),
IN firmType ENUM('Residential', 'Commercial')
)
BEGIN
IF NOT EXISTS (SELECT * FROM Firms WHERE Name = firmName) THEN
INSERT INTO Firms (Name, Address, PhoneNumber, Type)
VALUES (firmName, firmAddress, firmPhoneNumber, firmType);
SELECT 'Firm added successfully' AS Message;
ELSE
SELECT 'Firm already exists' AS Message;
END IF;
END;
Add a New Property
When adding a property, ensure the price is within the specified range and the status is set to "Available" by default.
CREATE PROCEDURE AddProperty (
IN firmID INT,
IN propertyAddress VARCHAR(255),
IN propertyPrice DECIMAL(10,2),
IN propertySize INT,
IN propertyType ENUM('Apartment', 'Condo', 'Single Family')
)
BEGIN
IF propertyPrice BETWEEN 1000 AND 3000000 THEN
INSERT INTO Properties (FirmID, Address, Price, Size, Status, ConstructionDate, Type)
VALUES (firmID, propertyAddress, propertyPrice, propertySize, 'Available', CURDATE(), propertyType);
SELECT 'Property added successfully' AS Message;
ELSE
SELECT 'Price must be between $1,000 and $3,000,000' AS Message;
END IF;
END;
Add a Room
Add a room to a property by ensuring valid room and flooring types.
CREATE PROCEDURE AddRoom (
IN propertyID INT,
IN roomType ENUM('Bathroom', 'Bedroom', 'Living Room'),
IN roomSize INT,
IN flooringType ENUM('Carpet', 'Wood', 'Tile')
)
BEGIN
INSERT INTO Rooms (PropertyID, RoomType, Size, FlooringType)
VALUES (propertyID, roomType, roomSize, flooringType);
SELECT 'Room added successfully' AS Message;
END;
Add an Agent
Ensure that the agent’s last name is unique and the agent is associated with a valid firm.
CREATE PROCEDURE AddAgent (
IN firmID INT,
IN firstName VARCHAR(50),
IN lastName VARCHAR(50),
IN address VARCHAR(255),
IN salary DECIMAL(10,2)
)
BEGIN
IF NOT EXISTS (SELECT * FROM Agents WHERE LastName = lastName) THEN
INSERT INTO Agents (FirmID, FirstName, LastName, Address, Salary, TotalSales)
VALUES (firmID, firstName, lastName, address, salary, 0);
SELECT 'Agent added successfully' AS Message;
ELSE
SELECT 'Agent with this last name already exists' AS Message;
END IF;
END;
2. Managing Properties
List Properties by State
To list properties located in a specific state, use a procedure that searches for properties based on the state in their address.
CREATE PROCEDURE ListPropertiesByState (
IN stateName VARCHAR(100)
)
BEGIN
SELECT * FROM Properties WHERE Address LIKE CONCAT('%', stateName, '%');
IF ROW_COUNT() = 0 THEN
SELECT 'No properties found in this state' AS Message;
END IF;
END;
Change Property Status
This procedure changes a property’s status to "Sold". To update the agent’s total sales, you will also use a trigger.
CREATE PROCEDURE ChangePropertyStatus (
IN propertyID INT
)
BEGIN
UPDATE Properties
SET Status = 'Sold'
WHERE PropertyID = propertyID;
END;
Update Property Price
Modify the price of an available property. This procedure needs to ensure that only properties with the status "Available" can have their prices updated.
CREATE PROCEDURE UpdatePropertyPrice (
IN propertyID INT,
IN newPrice DECIMAL(10,2)
)
BEGIN
UPDATE Properties
SET Price = newPrice
WHERE PropertyID = propertyID AND Status = 'Available';
END;
Calculate Total Sales for a Firm
Retrieve the total sales amount for all agents working under a specific firm.
CREATE PROCEDURE GetFirmSales (
IN firmID INT
)
BEGIN
SELECT SUM(TotalSales) AS TotalSales
FROM Agents
WHERE FirmID = firmID;
END;
Implementing Triggers
1. Updating Sales
Trigger for Sales Update
This trigger updates the total sales of an agent when a property is sold. It is activated after a property’s status is updated to "Sold".
CREATE TRIGGER UpdateSales AFTER UPDATE ON Properties
FOR EACH ROW
BEGIN
IF OLD.Status = 'Available' AND NEW.Status = 'Sold' THEN
UPDATE Agents
SET TotalSales = TotalSales + NEW.Price
WHERE AgentID = (SELECT AgentID FROM Properties WHERE PropertyID = NEW.PropertyID);
END IF;
END;
2. Logging Price Changes
Trigger for Logging Price Changes
This trigger logs changes to property prices, recording the old and new prices, the date of the change, and the user who made the change.
CREATE TRIGGER LogPriceChange AFTER UPDATE ON Properties
FOR EACH ROW
BEGIN
IF OLD.Price <> NEW.Price THEN
INSERT INTO PriceChangeLog (PropertyID, OldPrice, NewPrice, ChangeDate, User)
VALUES (OLD.PropertyID, OLD.Price, NEW.Price, NOW(), USER());
END IF;
END;
PriceChangeLog Table Definition:
To store the log data, you will need a PriceChangeLog table:
CREATE TABLE PriceChangeLog (
LogID INT AUTO_INCREMENT PRIMARY KEY,
PropertyID INT,
OldPrice DECIMAL(10,2),
NewPrice DECIMAL(10,2),
ChangeDate DATETIME,
User VARCHAR(50),
FOREIGN KEY (PropertyID) REFERENCES Properties(PropertyID)
);
Testing Procedures and Triggers
1. Testing Procedures
To ensure that procedures are functioning correctly, perform the following tests:
- Add Firm: Insert a new firm and verify that it is added without duplication.
- Add Property: Insert properties within the valid price range and check default status.
- Add Room: Add rooms with valid types and ensure they are correctly associated with properties.
- Add Agent: Insert agents and verify unique last names and correct firm association.
2. Testing Triggers
- Sales Update: Change a property’s status to "Sold" and verify that the agent’s total sales are updated accordingly.
- Price Logging: Modify a property’s price and check that changes are logged accurately with the correct details.
Optimization and Security
1. Indexing
Create indexes to improve query performance:
- Indexes on Foreign Keys: Enhance performance for join operations.
- Indexes on Frequently Queried Fields: For example, property addresses and agent names.
2. Constraints
Ensure that constraints are properly enforced:
- Primary Keys: Maintain uniqueness of records.
- Foreign Keys: Ensure referential integrity between tables.
- Check Constraints: Validate data ranges and types.
3. Security
Consider security best practices:
- SQL Injection Prevention: Use parameterized queries to prevent SQL injection attacks.
- Access Control: Restrict database access to authorized users only.
Advanced Considerations
1. Data Normalization
Ensure that the database design adheres to normalization principles to avoid redundancy and ensure data integrity. This involves organizing tables and columns to minimize duplication and dependency.
2. Transaction Management
Implement transactions to ensure that database operations are completed successfully. Transactions help maintain data integrity by allowing rollback in case of errors.
3. Performance Tuning
Monitor and optimize database performance by analyzing query execution plans and adjusting indexes and queries as needed. Consider using database profiling tools to identify and address performance bottlenecks.
Conclusion
Successfully completing your programming assignment requires a comprehensive understanding of database design, procedures, and triggers. By following the guidelines outlined in this detailed guide, you can systematically approach and implement the requirements for a real estate database or similar assignments.
Understanding these concepts not only helps in academic assignments but also prepares you for real-world database management and programming tasks. Mastery of these skills will enable you to design efficient, reliable, and scalable database solutions, which are crucial in various professional fields.