Claim Your Discount Today
Ring in Christmas and New Year with a special treat from www.programminghomeworkhelp.com! Get 15% off on all programming assignments when you use the code PHHCNY15 for expert assistance. Don’t miss this festive offer—available for a limited time. Start your New Year with academic success and savings. Act now and save!
We Accept
- 1. Introduction to Hash Tables
- 1. What is a Hash Table?
- 2. Key Components of a Hash Table
- 3. Implementing a Hash Table
- 1. Basic Hash Table Implementation
- 4. Collision Resolution Techniques
- 1. Linear Probing
- 2. Quadratic Probing
- 3. Chaining
- 5. Improving Hash Table Performance
- 1. Load Factor and Resizing
- 2. Hash Function Quality
- 3. Iterators
- 1. Algorithm for Checking Equivalence
- 2. Performance Improvements
- Conclusion
Hash tables are a cornerstone of efficient data management in computer science and mastering them can significantly enhance your programming skills. This blog post delves into the intricacies of hash tables, focusing on common java programming assignments involving hash table implementations, collision resolution, performance optimization, and algorithm improvements. By the end of this guide, you’ll have a solid understanding of how to approach and excel in data structure assignments that involve hash tables.
1. Introduction to Hash Tables
1. What is a Hash Table?
A hash table, also known as a hash map, is a data structure that offers a way to store and retrieve data efficiently using key-value pairs. The primary operations of a hash table are:
- Insertion: Adding a key-value pair to the table.
- Deletion: Removing a key-value pair from the table.
- Lookup: Retrieving the value associated with a given key.
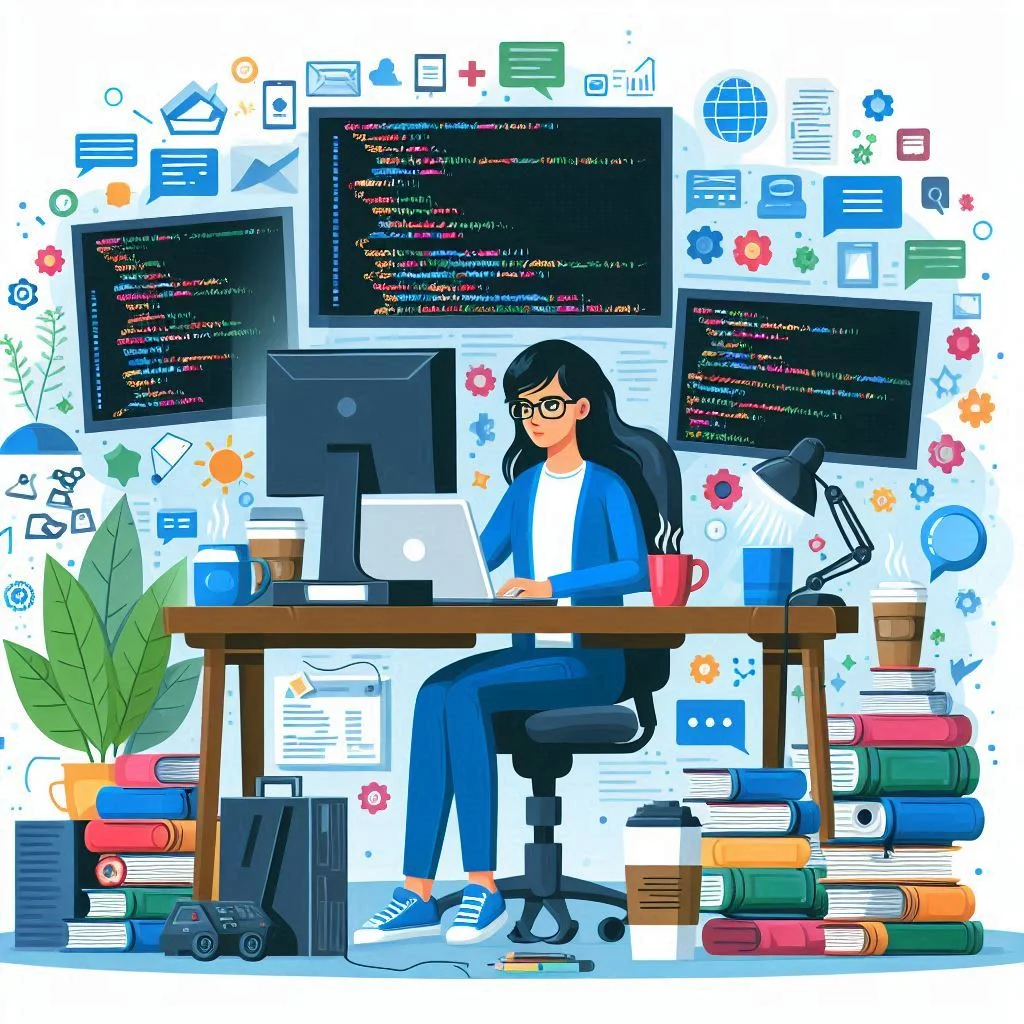
Hash tables are designed to provide average-case constant time complexity, O(1), for these operations. This efficiency is achieved through a hash function, which maps keys to indices in an underlying array.
2. Key Components of a Hash Table
- Hash Function: Converts a key into an index of an array where the value is stored. A good hash function distributes keys uniformly across the array to minimize collisions.
- Array: The underlying storage where the values are kept. Each position in the array corresponds to a potential index generated by the hash function.
- Collision Resolution: Mechanisms to handle cases where multiple keys hash to the same index. Common techniques include linear probing, quadratic probing, and chaining.
3. Implementing a Hash Table
1. Basic Hash Table Implementation
To build a basic hash table, start with a simple implementation that handles key-value pairs and basic operations. Here’s a brief outline:
1. Define the Hash Table Structure:
public class MyHashTable<K, V> {
private static final int INITIAL_CAPACITY = 16;
private static final float LOAD_FACTOR = 0.75f;
private Entry<K, V>[] table;
private int size = 0;
// Inner class to represent a key-value pair
private static class Entry<K, V> {
K key;
V value;
Entry<K, V> next;
Entry(K key, V value, Entry<K, V> next) {
this.key = key;
this.value = value;
this.next = next;
}
}
public MyHashTable() {
table = new Entry[INITIAL_CAPACITY];
}
}
2. Implement the Hash Function:
private int hash(K key) {
return (key == null) ? 0 : Math.abs(key.hashCode() % table.length);
}
3. Add Key-Value Pair:
public void put(K key, V value) {
int index = hash(key);
Entry<K, V> newEntry = new Entry<>(key, value, table[index]);
table[index] = newEntry;
size++;
if (size > table.length * LOAD_FACTOR) {
resize();
}
}
4. Retrieve Value by Key:
public V get(K key) {
int index = hash(key);
Entry<K, V> entry = table[index];
while (entry != null) {
if (entry.key.equals(key)) {
return entry.value;
}
entry = entry.next;
}
return null;
}
5. Remove Key-Value Pair:
public void remove(K key) {
int index = hash(key);
Entry<K, V> previous = null;
Entry<K, V> current = table[index];
while (current != null) {
if (current.key.equals(key)) {
if (previous == null) {
table[index] = current.next;
} else {
previous.next = current.next;
}
size--;
return;
}
previous = current;
current = current.next;
}
}
6. Resize the Hash Table:
private void resize() {
Entry<K, V>[] oldTable = table;
table = new Entry[oldTable.length * 2];
size = 0;
for (Entry<K, V> entry : oldTable) {
while (entry != null) {
put(entry.key, entry.value);
entry = entry.next;
}
}
}
4. Collision Resolution Techniques
Collision resolution is crucial for maintaining the efficiency of a hash table. Here, we explore different techniques:
1. Linear Probing
Linear probing resolves collisions by finding the next available slot in the array. While simple to implement, it can lead to clustering issues where groups of adjacent elements are filled.
Implementation:
private int probe(int index) {
while (table[index] != null) {
index = (index + 1) % table.length;
}
return index;
}
2. Quadratic Probing
Quadratic probing reduces clustering by increasing the step size quadratically. This technique distributes keys more uniformly compared to linear probing.
Implementation:
private int probe(int index, int i) {
return (index + i * i) % table.length;
}
3. Chaining
Chaining uses linked lists to handle collisions. Each array slot points to a list of entries with the same hash index. This approach avoids clustering but requires additional memory for the lists.
Implementation:
// Insert into the list at table[index]
Entry<K, V> newEntry = new Entry<>(key, value, table[index]);
table[index] = newEntry;
5. Improving Hash Table Performance
1. Load Factor and Resizing
Maintaining a proper load factor is essential for hash table performance. A higher load factor means fewer empty slots but more collisions. Implement dynamic resizing to keep the load factor within an optimal range.
Resizing Implementation:
private void resize() {
Entry<K, V>[] oldTable = table;
table = new Entry[oldTable.length * 2];
size = 0;
for (Entry<K, V> entry : oldTable) {
while (entry != null) {
put(entry.key, entry.value);
entry = entry.next;
}
}
}
2. Hash Function Quality
A good hash function distributes keys uniformly across the table. Poor hash functions can lead to excessive collisions and degrade performance. Test and refine your hash function to ensure it provides a good distribution.
Example Hash Function:
private int hash(K key) { int hash = key.hashCode(); hash = (hash ^ (hash >>> 16)) & (table.length - 1); return hash; }
3. Iterators
Implementing an iterator allows you to traverse the elements in the hash table. Ensure your iterator provides consistent and efficient access.
Iterator Implementation:
public Iterator<V> iterator() {
return new Iterator<V>() {
private int currentIndex = 0;
private Entry<K, V> currentEntry = null;
@Override
public boolean hasNext() {
if (currentEntry != null && currentEntry.next != null) {
return true;
}
while (currentIndex < table.length) {
if (table[currentIndex] != null) {
return true;
}
currentIndex++;
}
return false;
}
@Override
public V next() {
if (currentEntry == null || currentEntry.next == null) {
while (currentIndex < table.length && table[currentIndex] == null) {
currentIndex++;
}
if (currentIndex < table.length) {
currentEntry = table[currentIndex++];
}
}
V value = currentEntry.value;
currentEntry = currentEntry.next;
return value;
}
};
}
1. Algorithm for Checking Equivalence
The equalsUniqueElements method checks if two hash tables are equivalent by comparing their elements. Ensure the algorithm accurately compares the elements and handles edge cases.
Corrected Algorithm:
public boolean equalsUniqueElements(MyHashTable<K, V> other) {
if (this.size != other.size) {
return false;
}
for (Entry<K, V> entry : this.table) {
while (entry != null) {
if (!other.contains(entry.key)) {
return false;
}
entry = entry.next;
}
}
return true;
}
private boolean contains(K key) {
int index = hash(key);
Entry<K, V> entry = table[index];
while (entry != null) {
if (entry.key.equals(key)) {
return true;
}
entry = entry.next;
}
return false;
}
2. Performance Improvements
Evaluate and enhance the performance of your hash table. Aim for O(1) time complexity for insertion, removal, and lookup operations. Optimize the hash function, collision resolution technique, and resizing strategy to meet performance goals.
Conclusion
Hash tables are a powerful data structure with numerous applications. By mastering hash table implementations, collision resolution techniques, and performance optimizations, you can tackle a wide range of programming assignments and improve your problem-solving skills. Focus on understanding the core concepts, implementing efficient algorithms, and following best practices to solve programming assignments.
Continue experimenting and refining your hash table implementations to deepen your understanding and achieve optimal performance. With practice and perseverance, you'll become adept at solving complex problems involving hash tables and other data structures.
This detailed guide provides a comprehensive overview of hash tables, including implementation, collision resolution, performance improvements, and best practices. By following these principles and techniques, you’ll be well-equipped to handle programming assignments and build efficient data structures.