Claim Your Discount Today
Ring in Christmas and New Year with a special treat from www.programminghomeworkhelp.com! Get 15% off on all programming assignments when you use the code PHHCNY15 for expert assistance. Don’t miss this festive offer—available for a limited time. Start your New Year with academic success and savings. Act now and save!
We Accept
- Understanding the Importance of Memory Manipulation
- The Power of Direct Memory Access
- Flexibility in Memory Allocation
- Performance Optimization Opportunities
- Foundations of Memory Manipulation: Pointers and Structs
- Understanding Pointers
- Exploring Structs
- Practical Examples and Exercises
- Advanced Memory Manipulation Techniques
- Dynamic Memory Allocation
- Double Linked-Lists
- Advanced Techniques and Applications
- Best Practices for Memory Management
- Avoiding Memory Leaks
- Handling Dangling Pointers
- Practical Applications and Examples
- Conclusion
Memory manipulation stands as a foundational skill within C programming, affording developers the capacity to efficiently allocate, deallocate, and manipulate memory resources. This comprehensive guide aims to delve into the essential techniques necessary for mastering memory manipulation in C. From grasping the significance of memory manipulation to delving into advanced techniques and best practices, this blog post endeavors to equip readers with the knowledge and skills crucial for excelling in programming assignments and projects. Through a thorough exploration of memory manipulation concepts, readers will gain a deeper understanding of how to optimize memory usage, mitigate common pitfalls, and enhance program performance. Whether navigating dynamic memory allocation, understanding pointers and structs, or implementing advanced data structures, this guide seeks to provide practical insights and actionable strategies to empower developers in their C programming endeavors.
Understanding the Importance of Memory Manipulation
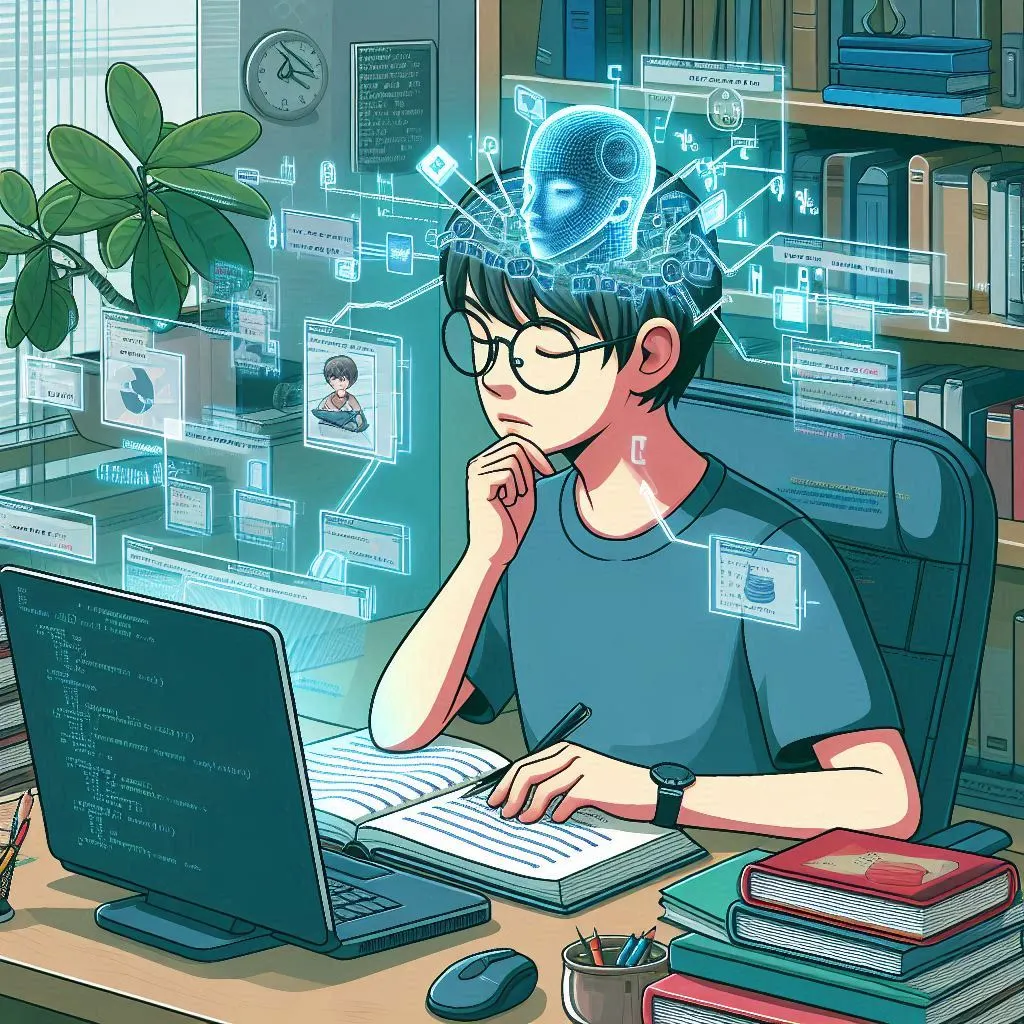
Memory manipulation is pivotal for optimizing performance and resource utilization in C programming. Unlike high-level languages such as Python or Java, C provides direct control over memory management, enabling developers to finely tune their programs for efficiency. Mastery of memory manipulation empowers programmers to dynamically allocate and deallocate memory, optimize data structures, and minimize memory overhead. This level of control is particularly valuable in scenarios where speed and resource efficiency are paramount, such as embedded systems programming and performance-critical applications. With a solid understanding of memory manipulation, developers can unlock the full potential of C programming and achieve optimal performance in their projects and assignments.
The Power of Direct Memory Access
One of the key advantages of memory manipulation in C is the ability to directly access memory addresses. This enables developers to manipulate data at the lowest level, resulting in highly efficient code and optimal performance. Direct memory access is particularly valuable in scenarios where speed and resource efficiency are paramount, such as embedded systems programming and performance-critical applications. Leveraging direct memory access empowers developers to fine-tune their programs to extract maximum efficiency from hardware resources, leading to streamlined and high-performing software solutions.
Flexibility in Memory Allocation
C provides developers with the flexibility to allocate and deallocate memory dynamically at runtime. This dynamic memory management capability allows for the creation of data structures whose size is not known at compile time, enabling more efficient memory usage and greater flexibility in program design. By dynamically allocating memory, developers can adapt their programs to changing requirements and optimize resource utilization. This flexibility is particularly advantageous in scenarios where the size of data structures may vary during program execution, enabling developers to allocate memory as needed and avoid unnecessary resource wastage.
Performance Optimization Opportunities
By mastering memory manipulation techniques, developers can optimize their C programs for maximum performance. This includes minimizing memory fragmentation, reducing memory overhead, and improving cache locality to enhance overall efficiency. With direct control over memory management, developers can fine-tune their programs to meet performance targets and deliver optimal user experiences. Leveraging performance optimization opportunities allows developers to squeeze out every bit of performance from their C programs, resulting in faster execution times and improved responsiveness.
Foundations of Memory Manipulation: Pointers and Structs
Central to memory manipulation in C are pointers and structs, core concepts empowering developers to interact with memory at a granular level. Pointers grant direct access to memory addresses, facilitating efficient data manipulation, while structs organize related data into cohesive custom data types. In unison, pointers and structs serve as the bedrock of dynamic memory allocation and data structure manipulation in C, providing developers with the tools to craft robust and scalable programs. Mastery of these fundamental concepts is paramount for navigating the intricacies of memory manipulation and fully harnessing the capabilities of C programming. By understanding pointers and structs, developers gain the ability to optimize memory usage, enhance program performance, and tackle complex programming challenges with confidence.
Understanding Pointers
Pointers are variables that store memory addresses, allowing developers to manipulate memory directly. By dereferencing pointers, developers can read from and write to specific memory locations, enabling efficient data manipulation and dynamic memory allocation. Understanding pointers is essential for mastering memory manipulation in C and unlocking the full potential of the language. With a solid grasp of pointers, developers gain the ability to optimize memory usage, implement efficient data structures, and navigate complex programming tasks with confidence.
Exploring Structs
Structs, short for structures, are user-defined data types that allow developers to group related variables together. By defining structs, developers can create complex data structures to represent real-world entities and relationships. Structs provide a way to organize and access data in memory, making them essential for building efficient and scalable C programs. Exploring structs enables developers to design modular and organized codebases, enhancing readability and maintainability while facilitating the creation of sophisticated software solutions.
Practical Examples and Exercises
To solidify understanding of pointers and structs, developers should practice writing code and solving exercises that involve their usage. This could include tasks such as implementing linked lists, trees, and other data structures using pointers and structs, as well as analyzing existing code examples to understand how memory manipulation is used in practice. Practical examples and exercises offer hands-on experience with memory manipulation concepts, reinforcing theoretical knowledge and fostering problem-solving skills essential for real-world programming challenges. By engaging in practical exercises, developers can deepen their understanding of memory manipulation techniques and build confidence in their ability to apply them effectively in their own projects.
Advanced Memory Manipulation Techniques
Advanced memory manipulation techniques build upon foundational concepts, empowering developers to efficiently tackle complex programming tasks. Techniques such as dynamic memory allocation, double linked-lists, and other advanced methods provide powerful tools for managing memory dynamically and optimizing resource usage in C programs. These techniques enable developers to create scalable and efficient solutions while addressing the challenges posed by dynamic data structures and memory management in C programming. By mastering these advanced techniques, developers can enhance the performance, scalability, and reliability of their C programs, delivering robust solutions that meet the demands of modern software development.
Dynamic Memory Allocation
Dynamic memory allocation allows developers to allocate memory at runtime, enabling the creation of data structures whose size is determined dynamically. Functions such as malloc(), calloc(), and realloc() are used to allocate memory on the heap, returning a pointer to the allocated memory block. Dynamic memory allocation is particularly useful for handling variable-sized data structures and managing memory efficiently in resource-constrained environments. By leveraging dynamic memory allocation, developers can create flexible and adaptive programs that can adjust memory usage based on runtime conditions, leading to more efficient resource utilization and enhanced program performance.
Double Linked-Lists
Double linked-lists are a type of linked list where each node contains two pointers - one pointing to the previous node and one pointing to the next node. This allows for efficient insertion and deletion operations at both ends of the list, making double linked-lists a versatile data structure for various applications. By mastering double linked-lists, developers can implement efficient and scalable data structures in their C programs. With double linked-lists, developers gain the ability to perform operations such as insertion, deletion, and traversal with ease, enabling the creation of sophisticated data structures that meet the requirements of diverse programming tasks.
Advanced Techniques and Applications
In addition to dynamic memory allocation and double linked-lists, developers can explore a variety of advanced techniques and applications for memory manipulation in C programming. These may include techniques such as memory pooling, memory mapping, and memory optimization algorithms, as well as applications such as memory-mapped I/O and shared memory concurrency. By delving into advanced techniques and applications, developers can further optimize memory usage, enhance program performance, and address complex programming challenges with efficiency and precision. Advanced memory manipulation techniques offer powerful tools for developers to create high-performance and reliable software solutions, making them essential skills for mastering C programming.
Best Practices for Memory Management
In C programming, adhering to best practices for memory management is crucial to avoid common pitfalls like memory leaks and dangling pointers. While C provides unparalleled flexibility in memory management, developers must adopt best practices to ensure the reliability and robustness of their programs. By following established guidelines and techniques, developers can minimize the risk of memory-related errors and vulnerabilities, leading to more stable and secure C programs. Effective memory management practices not only enhance the performance and efficiency of programs but also contribute to the overall quality and maintainability of the codebase. By prioritizing proper memory management techniques, developers can create software that is both efficient and reliable, meeting the demands of modern software development while reducing the likelihood of costly errors and system failures.
Avoiding Memory Leaks
Memory leaks occur when dynamically allocated memory is not properly deallocated after it is no longer needed, leading to a gradual depletion of available memory resources. To avoid memory leaks, developers should always free dynamically allocated memory using the free() function when it is no longer needed. By diligently freeing memory as soon as it is no longer needed, developers can prevent memory leaks and ensure efficient memory usage in their programs. Adopting proactive memory management practices is essential for maintaining the stability and reliability of C programs, preventing potential issues that could arise from memory leaks and optimizing overall program performance.
Handling Dangling Pointers
Dangling pointers are pointers that point to memory locations that have already been deallocated, leading to undefined behavior when dereferenced. To prevent dangling pointers, developers should set pointers to NULL after freeing the memory they point to, indicating that they no longer point to valid memory locations. By nullifying dangling pointers, developers can avoid memory-related errors and ensure the stability and reliability of their C programs. Handling dangling pointers effectively requires careful attention to memory management practices and a proactive approach to identifying and addressing potential issues before they manifest into runtime errors or system failures.
Practical Applications and Examples
To solidify understanding and mastery of memory manipulation techniques, developers should apply theoretical knowledge to real-world scenarios through practical examples and exercises. This could include writing code snippets to demonstrate memory manipulation techniques, solving programming challenges that require efficient memory usage, and analyzing existing code examples to understand how memory manipulation is used in practice. Practical applications and examples provide hands-on experience with memory manipulation concepts, allowing developers to gain insights into real-world programming scenarios and apply their knowledge effectively. By engaging in practical exercises, developers can deepen their understanding of memory manipulation techniques and build confidence in their ability to apply them in their own projects. These practical experiences help bridge the gap between theoretical knowledge and practical application, enabling developers to become proficient in memory manipulation and excel in their programming endeavors.
Conclusion
In conclusion, mastering memory manipulation in C is a journey that demands dedication, practice, and a profound comprehension of underlying principles. By embracing essential techniques, adhering to best practices, and continually refining your skills, you can harness the complete potential of C programming and thrive in your programming assignments and projects. With direct control over memory management and a comprehensive understanding of memory manipulation techniques, you'll possess the tools to confidently tackle intricate programming tasks and optimize the performance and efficiency of your C programs. As you embark on this journey, remember that persistence and a commitment to learning are key, and leverage available resources and support networks to aid you along the way. With determination and perseverance, you can navigate the complexities of memory manipulation in C and emerge as a proficient and successful programmer.