Claim Your Discount Today
Ring in Christmas and New Year with a special treat from www.programminghomeworkhelp.com! Get 15% off on all programming assignments when you use the code PHHCNY15 for expert assistance. Don’t miss this festive offer—available for a limited time. Start your New Year with academic success and savings. Act now and save!
We Accept
- Introduction to WebGL
- Key Features of WebGL:
- Understanding the Problem Requirement
- 1. Create a 3D Scene:
- 2. Code Requirements:
- 3. Deliverables:
- Setting Up Development Environment
- Recommended Tools:
- Creating WebGL Project
- 1. HTML Setup
- 2. JavaScript Initialization
- Building the Rendering Pipeline
- 1. Shaders
- 2. Buffers and Attributes
- Adding Lighting and Textures
- Lighting:
- Example Lighting Setup:
- Implementing Interactive Controls
- Example: Toggle Lighting
- JavaScript for Control:
- Using Frame Buffers
- Creating a Framebuffer:
- Testing and Debugging
- Example Test Plan:
- Documentation:
- Best Practices and Optimization
- Code Organization:
- Performance Optimization:
- Debugging Tips:
- Conclusion
Creating a 3D animated scene using WebGL is a complex but rewarding project that combines the power of JavaScript with the precision of computer graphics. WebGL assignments can be complex and multifaceted, requiring a strong grasp of 3D graphics, animation, and interactive elements. In this detailed guide, we'll walk you through the entire process, from understanding WebGL fundamentals to implementing advanced features like lighting, textures, and interactive controls. By the end, you'll have a comprehensive understanding of how to build and optimize a 3D animated scene, complete programming assignment with best practices and troubleshooting tips.
Introduction to WebGL
WebGL (Web Graphics Library) is a JavaScript API that allows you to render interactive 3D graphics directly within any compatible web browser. It provides a way to perform hardware-accelerated graphics rendering using the HTML5 <canvas> element. Unlike traditional graphics APIs, WebGL operates within the web environment, making it highly accessible for developers familiar with JavaScript.
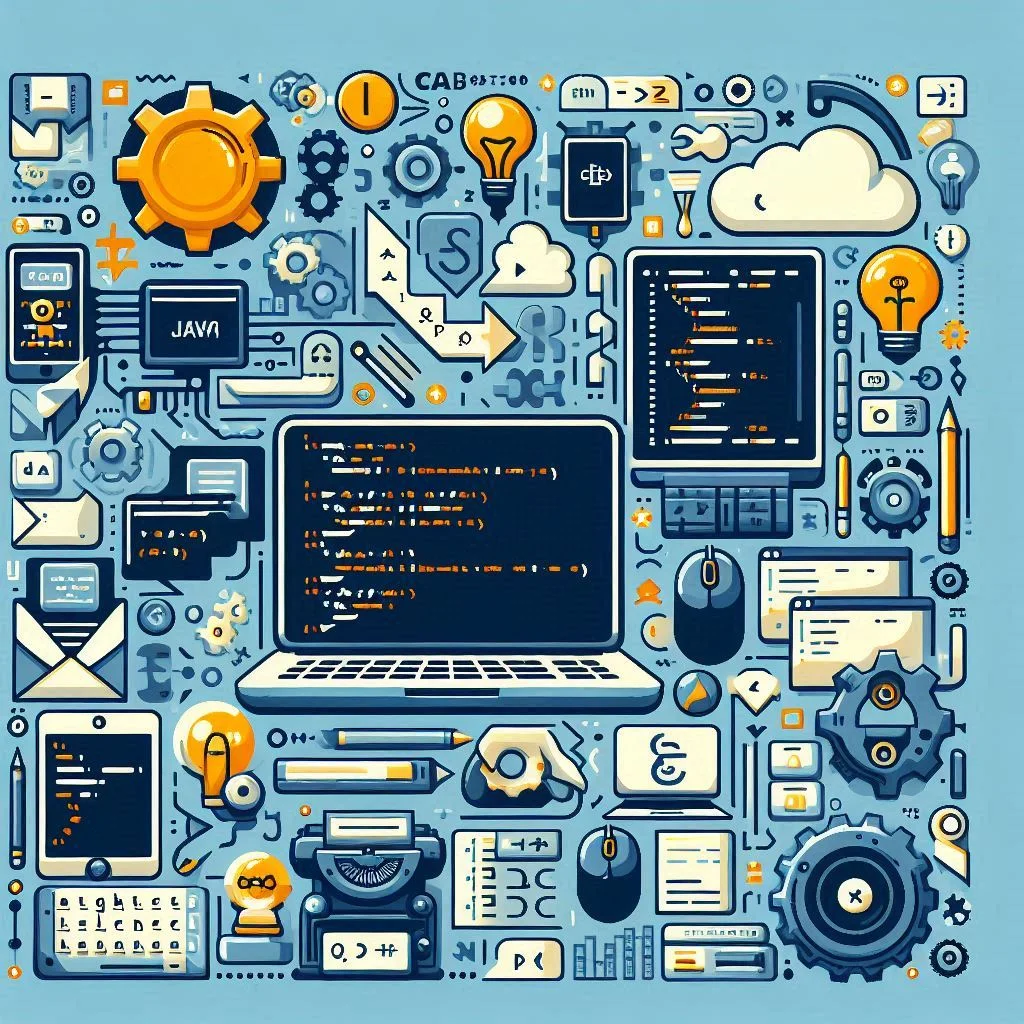
Key Features of WebGL:
- Integration with HTML5 Canvas: WebGL operates on an HTML5 <canvas> element, providing a drawing surface for rendering graphics.
- Shaders: WebGL uses GLSL (OpenGL Shading Language) to write shaders, which are small programs that run on the GPU and control the rendering pipeline.
- Hardware Acceleration: WebGL leverages the GPU to accelerate rendering, making it suitable for complex graphics and animations.
Understanding the Problem Requirement
Create a unique 3D animated scene using WebGL, incorporating various graphical elements and interactive components.
Before diving into coding, it’s crucial to break down the assignment requirements and understand each component:
1. Create a 3D Scene:
- Size: The scene must have a minimum resolution of 640x480 pixels.
- Objects: At least 10 different 3D objects need to be included.
- Lighting: Multiple lighting effects should be applied to various materials.
- Textures: Multiple textures need to be used on the objects.
- Widgets: Implement interactive elements like radio buttons or sliders to control components of the animation.
- Frame Buffers: Use frame buffers to manage rendering efficiently.
2. Code Requirements:
- WebGL: The application must be developed using WebGL.
- JavaScript Style: Follow the Google JavaScript style guide.
- Test Plan: Document a test plan with a matrix listing methods tested, testing procedures, and results.
3. Deliverables:
- Code: All JavaScript source code adhering to the Google JavaScript style guide.
- Documentation: A well-organized document with screen captures, descriptions, a test plan, and APA-style references.
Setting Up Development Environment
Before diving into coding, it's essential to set up your development environment. You'll need a modern web browser with WebGL support and a code editor for writing your HTML and JavaScript files.
Recommended Tools:
Code Editor: Use an editor like Visual Studio Code, Sublime Text, or Atom for writing and managing your code.
Web Browser: Ensure you use a browser that supports WebGL, such as Google Chrome, Mozilla Firefox, or Microsoft Edge.
Creating WebGL Project
1. HTML Setup
Start by creating a basic HTML structure that includes a <canvas> element. This canvas will serve as the rendering surface for your 3D scene.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>3D Animated Scene with WebGL</title>
<style>
body { margin: 0; }
canvas { width: 100%; height: 100%; display: block; }
</style>
</head>
<body>
<canvas id="glcanvas" width="640" height="480"></canvas>
<script src="your_script.js"></script>
</body>
</html>
2. JavaScript Initialization
Create a JavaScript file (your_script.js) where you'll write the code to initialize WebGL and render your scene.
const canvas = document.getElementById('glcanvas');
const gl = canvas.getContext('webgl');
if (!gl) {
alert('WebGL not supported. Please use a compatible browser.');
}
Building the Rendering Pipeline
WebGL rendering involves several key components: shaders, buffers, and attributes. Here’s how to set up these components:
1. Shaders
Shaders are small programs that run on the GPU and handle the rendering of your graphics. You'll need a vertex shader and a fragment shader.
Vertex Shader:
The vertex shader processes each vertex of your 3D objects. It typically handles transformations and passes data to the fragment shader.
<code ignore--minify class="code-view">attribute vec4 a_position;
attribute vec4 a_color;
varying vec4 v_color;
void main(void) {
gl_Position = a_position;
v_color = a_color;
}
</code>
Fragment Shader:
The fragment shader determines the color of each pixel. It uses the data passed from the vertex shader to apply textures and lighting effects.
precision mediump float;
varying vec4 v_color;
void main(void) {
gl_FragColor = v_color;
}
Compiling and Linking Shaders:
In your JavaScript file, compile and link the shaders into a WebGL program.
function createShader(gl, source, type) {
const shader = gl.createShader(type);
gl.shaderSource(shader, source);
gl.compileShader(shader);
if (!gl.getShaderParameter(shader, gl.COMPILE_STATUS)) {
console.error(gl.getShaderInfoLog(shader));
gl.deleteShader(shader);
return null;
}
return shader;
}
const vertexShaderSource = `...`; // Your vertex shader code
const fragmentShaderSource = `...`; // Your fragment shader code
const vertexShader = createShader(gl, vertexShaderSource, gl.VERTEX_SHADER);
const fragmentShader = createShader(gl, fragmentShaderSource, gl.FRAGMENT_SHADER);
const program = gl.createProgram();
gl.attachShader(program, vertexShader);
gl.attachShader(program, fragmentShader);
gl.linkProgram(program);
if (!gl.getProgramParameter(program, gl.LINK_STATUS)) {
console.error(gl.getProgramInfoLog(program));
}
gl.useProgram(program);
2. Buffers and Attributes
Buffers hold the vertex data for your 3D objects. Set up vertex buffers and attributes to describe the shape and color of your objects.
const vertices = new Float32Array([
// Vertex positions and colors for your objects
]);
const vertexBuffer = gl.createBuffer();
gl.bindBuffer(gl.ARRAY_BUFFER, vertexBuffer);
gl.bufferData(gl.ARRAY_BUFFER, vertices, gl.STATIC_DRAW);
const positionLocation = gl.getAttribLocation(program, 'a_position');
const colorLocation = gl.getAttribLocation(program, 'a_color');
gl.enableVertexAttribArray(positionLocation);
gl.vertexAttribPointer(positionLocation, 3, gl.FLOAT, false, 24, 0);
gl.enableVertexAttribArray(colorLocation);
gl.vertexAttribPointer(colorLocation, 3, gl.FLOAT, false, 24, 12);
Adding Lighting and Textures
Lighting:
Lighting effects add realism to your scene. You can implement multiple light sources and different types of lighting (e.g., ambient, diffuse, specular).
Example Lighting Setup:
Modify your shaders to include lighting calculations. Here's an example of a simple Phong lighting model:
Vertex Shader:
<code ignore--minify class="code-view">attribute vec4 a_position;
attribute vec4 a_color;
uniform mat4 u_modelViewMatrix;
uniform mat4 u_projectionMatrix;
varying vec4 v_color;
void main(void) {
gl_Position = u_projectionMatrix * u_modelViewMatrix * a_position;
v_color = a_color;
}
</code>
Fragment Shader:
precision mediump float;
varying vec4 v_color;
void main(void) {
// Simple lighting calculation
vec3 lightDirection = normalize(vec3(1.0, 1.0, 1.0));
float diffuse = max(dot(normalize(v_color.xyz), lightDirection), 0.0);
gl_FragColor = vec4(diffuse * v_color.rgb, v_color.a);
}
Textures:
Textures add detail to your objects. Load textures and apply them in your fragment shader.
const texture = gl.createTexture();
const image = new Image();
image.src = 'path_to_texture_image.png';
image.onload = () => {
gl.bindTexture(gl.TEXTURE_2D, texture);
gl.texImage2D(gl.TEXTURE_2D, 0, gl.RGBA, gl.RGBA, gl.UNSIGNED_BYTE, image);
gl.generateMipmap(gl.TEXTURE_2D);
};
Fragment Shader with Texture:
precision mediump float;
uniform sampler2D u_texture;
varying vec2 v_texCoord;
void main(void) {
gl_FragColor = texture2D(u_texture, v_texCoord);
}
Implementing Interactive Controls
Interactive controls such as sliders and radio buttons enhance the user experience by allowing users to modify the scene in real time.
Example: Toggle Lighting
<label>
<input type="checkbox" id="toggleLighting"> Toggle Lighting
</label>
JavaScript for Control:
document.getElementById('toggleLighting').addEventListener('change', (event) => {
// Toggle lighting effect based on checkbox state
const lightingEnabled = event.target.checked;
// Update your scene based on the lightingEnabled flag
});
Using Frame Buffers
Frame buffers are used for off-screen rendering and complex scene management. They allow you to render to textures or perform post-processing effects.
Creating a Framebuffer:
const framebuffer = gl.createFramebuffer();
gl.bindFramebuffer(gl.FRAMEBUFFER, framebuffer);
Setting Up Render Targets:
const texture = gl.createTexture();
gl.bindTexture(gl.TEXTURE_2D, texture);
gl.texImage2D(gl.TEXTURE_2D, 0, gl.RGBA, canvas.width, canvas.height, 0, gl.RGBA, gl.UNSIGNED_BYTE, null);
gl.texParameteri(gl.TEXTURE_2D, gl.TEXTURE_MIN_FILTER, gl.LINEAR);
gl.texParameteri(gl.TEXTURE_2D, gl.TEXTURE_MAG_FILTER, gl.LINEAR);
gl.framebufferTexture2D(gl.FRAMEBUFFER, gl.COLOR_ATTACHMENT0, gl.TEXTURE_2D, texture, 0);
Testing and Debugging
Testing is crucial to ensure your scene works correctly across different browsers and devices. Develop a test plan that includes various scenarios to verify functionality.
Example Test Plan:
- Functional Testing: Verify that each feature (e.g., lighting, textures, controls) works as expected.
- Performance Testing: Check the performance and responsiveness of your scene.
- Cross-Browser Testing: Ensure compatibility with different web browsers.
Documentation:
Document your process, including screen captures, test results, and code snippets. Use a well-organized format with headings, captions, and a title page.
Best Practices and Optimization
Code Organization:
- Keep your code modular by separating shader code, buffer setup, and rendering logic.
- Follow coding standards and style guides, such as the Google JavaScript Style Guide.
Performance Optimization:
- Minimize state changes and avoid redundant calculations.
- Use efficient data structures and algorithms for managing your 3D scene.
Debugging Tips:
- Use WebGL debugging tools and browser developer tools to diagnose issues.
- Check for common errors, such as invalid shader programs or incorrect buffer setups.
Conclusion
Completing a WebGL assignment involves a blend of understanding 3D graphics, coding, and documentation. By following this detailed guide, you can navigate the complexities of WebGL effectively and produce a well-structured, functional 3D scene. Remember to approach each task methodically, from setting up your environment to documenting your work. With careful planning and execution, you’ll be able to tackle any WebGL programming assignment with confidence.