Claim Your Offer
Unlock an amazing offer at www.programminghomeworkhelp.com with our latest promotion. Get an incredible 10% off on your all programming assignment, ensuring top-quality assistance at an affordable price. Our team of expert programmers is here to help you, making your academic journey smoother and more cost-effective. Don't miss this chance to improve your skills and save on your studies. Take advantage of our offer now and secure exceptional help for your programming assignments.
We Accept
- Understanding Memory Pool Basics
- Part 1: Implementing the Memory Pool
- Overview of Required Functions
- Sample Implementation
- Summary
- Part 2: Sample Application
- 1. Create a Memory Pool:
- 2. Allocate and Write Data to Each Block:
- 3. Attempt to Allocate an Additional Block:
- 4. Display the Contents of Each Block:
- 5. Free All Blocks and Destroy the Memory Pool:
- Conclusion
In many programming scenarios, managing memory efficiently is crucial for optimizing performance and resource utilization. Inefficient memory management can lead to performance bottlenecks, increased overhead, and even system crashes. One highly effective technique for improving memory management is by implementing a memory pool system. This approach involves pre-allocating a block of memory and then managing it in a structured manner, which can significantly enhance both allocation speed and overall system efficiency.
A memory pool allows for efficient allocation and deallocation by keeping track of memory blocks in a predefined pool, rather than constantly requesting and releasing memory from the operating system. This method can reduce fragmentation, minimize the time spent on allocation, and avoid the overhead associated with frequent memory operations. It is particularly useful in systems with limited resources or in real-time applications where performance consistency is critical.
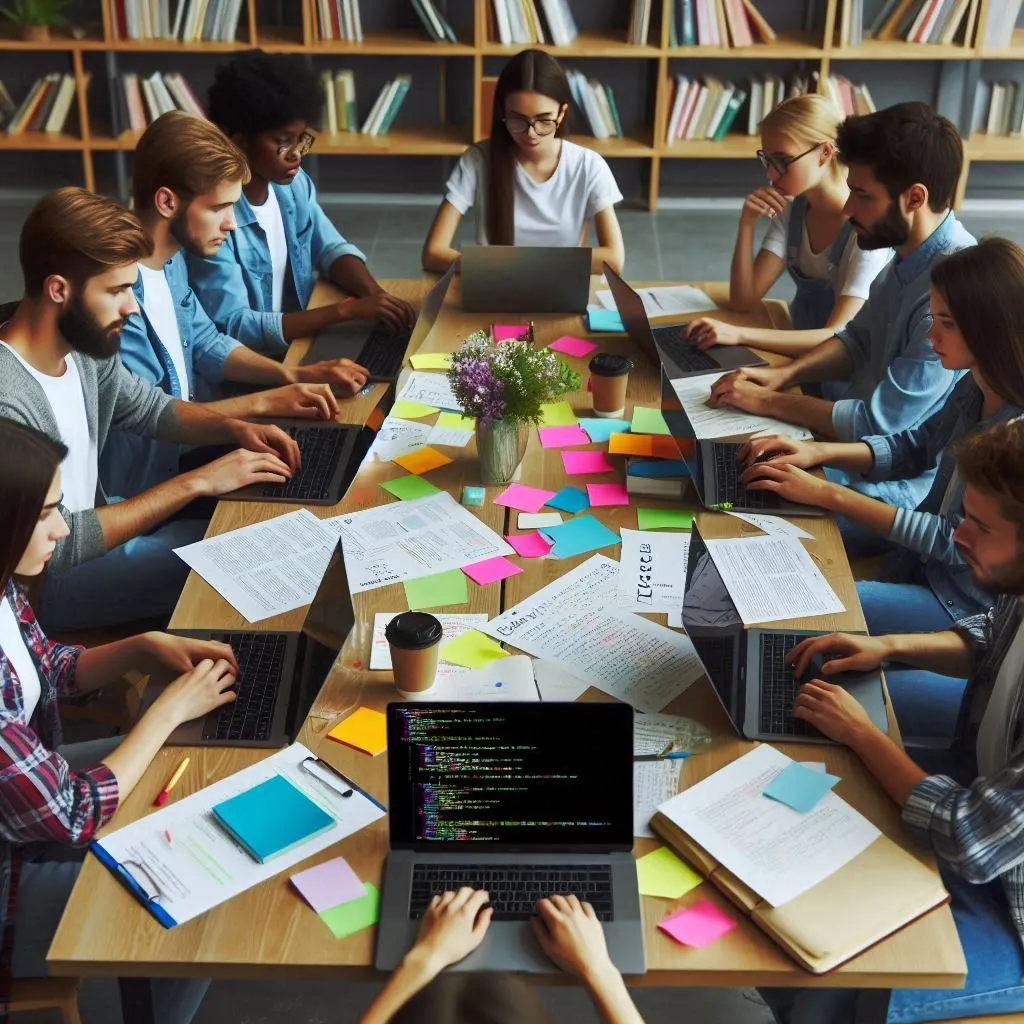
In this blog, we will provide a comprehensive yet concise overview of how to create and utilize a memory pool system in C. We will walk through the essential components and implementation details, closely mirroring the principles found in assignments of this nature. This guide is designed to be applicable to a wide range of similar assignments, offering insights into how to efficiently manage memory for various programming challenges. By delving into the fundamental concepts and practical applications of a memory pool, you will gain a deeper understanding of memory management techniques and enhance your ability to tackle similar programming tasks with confidence. This knowledge will not only improve your efficiency in handling memory-related assignments but also contribute to better performance and resource utilization in your software projects. For those seeking additional support, our C assignment help services are available to further assist with complex programming tasks.
Understanding Memory Pool Basics
A memory pool is a sophisticated technique for managing memory allocation and deallocation in a more controlled and efficient manner compared to traditional memory allocation functions like malloc and free. Unlike these standard functions, which operate directly with the operating system’s heap and can lead to fragmented memory and unpredictable performance, a memory pool pre-allocates a fixed block of memory and then manages it in a structured way.
This system involves setting aside a specific amount of memory from which allocations are made. Within this pool, memory blocks are managed according to predefined sizes and statuses, reducing the need for frequent interactions with the system's memory manager. This pre-allocation minimizes the overhead associated with repeated allocation and deallocation requests, thus improving performance and reducing latency.
Memory pools are particularly advantageous in environments where performance is critical, such as embedded systems, real-time applications, and high-performance computing scenarios. In these contexts, predictable and efficient memory management is essential to meet stringent timing requirements and resource constraints. By using a memory pool, you can achieve more consistent and reliable performance, avoid fragmentation, and manage memory usage more effectively.
Overall, a memory pool system provides a robust solution for optimizing memory management by offering more control over how memory is allocated and freed, leading to improved efficiency and performance in demanding applications. For students and developers looking to master these concepts, our programming assignment help services can provide the necessary guidance to implement such systems effectively.
Part 1: Implementing the Memory Pool
In this section, we will implement a memory pool with a set of specific API functions to manage memory blocks efficiently. A memory pool provides a controlled way to allocate and deallocate memory, optimizing performance and reducing fragmentation. Below is an overview of the required functions and a sample implementation.
Overview of Required Functions
- MP_Create(int blocksize, int num_blocks): Initializes a memory pool with the specified number of blocks and block size. Returns a pointer to the created memory pool or NULL if initialization fails.
- MP_AllocBlock(): Allocates a block from the memory pool and returns the index of the allocated block. If no blocks are available, it returns -1.
- MP_FreeBlock(int block_idx): Frees a block by its index and clears its contents. Returns 1 on success or 0 if the block index is invalid or already free.
- MP_GetBlockData(int block_idx): Retrieves a pointer to the user data portion of the specified block. Returns NULL if the block is free or the index is invalid.
- **MP_Destroy(mp_t mp): Frees all memory associated with the memory pool and sets the pool pointer to NULL. Returns 1 on successful destruction or 0 if the pointer is invalid.
Sample Implementation
Here’s a basic implementation of the memory pool functions in C:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_BLOCKS 100
#define BLOCK_SIZE 1024
typedef struct {
char *blocks;
int *block_status;
int block_size;
int num_blocks;
} mp_t;
/**
* Creates a memory pool with the specified block size and number of blocks.
* @param blocksize The size of each block in bytes.
* @param num_blocks The number of blocks in the pool.
* @return A pointer to the created memory pool, or NULL if creation fails.
*/
mp_t *MP_Create(int blocksize, int num_blocks) {
// Validate parameters
if (blocksize > BLOCK_SIZE || num_blocks > MAX_BLOCKS) return NULL;
// Allocate memory for the memory pool structure
mp_t *pool = malloc(sizeof(mp_t));
if (!pool) return NULL;
// Allocate memory for the blocks
pool->blocks = malloc(blocksize * num_blocks);
if (!pool->blocks) {
free(pool);
return NULL;
}
// Allocate memory for block status tracking
pool->block_status = calloc(num_blocks, sizeof(int));
if (!pool->block_status) {
free(pool->blocks);
free(pool);
return NULL;
}
pool->block_size = blocksize;
pool->num_blocks = num_blocks;
return pool;
}
/**
* Allocates a free block from the memory pool.
* @param pool A pointer to the memory pool.
* @return The index of the allocated block, or -1 if no blocks are available.
*/
int MP_AllocBlock(mp_t *pool) {
for (int i = 0; i < pool->num_blocks; i++) {
if (pool->block_status[i] == 0) {
pool->block_status[i] = 1;
return i;
}
}
return -1;
}
/**
* Frees a block and clears its contents.
* @param pool A pointer to the memory pool.
* @param block_idx The index of the block to free.
* @return 1 if successful, 0 if the block index is invalid or the block is already free.
*/
int MP_FreeBlock(mp_t *pool, int block_idx) {
if (block_idx < 0 || block_idx >= pool->num_blocks || pool->block_status[block_idx] == 0)
return 0;
pool->block_status[block_idx] = 0;
memset(pool->blocks + (block_idx * pool->block_size), 0, pool->block_size);
return 1;
}
/**
* Retrieves a pointer to the user data portion of a block.
* @param pool A pointer to the memory pool.
* @param block_idx The index of the block.
* @return A pointer to the block's data, or NULL if the block is free or the index is invalid.
*/
void *MP_GetBlockData(mp_t *pool, int block_idx) {
if (block_idx < 0 || block_idx >= pool->num_blocks || pool->block_status[block_idx] == 0)
return NULL;
return pool->blocks + (block_idx * pool->block_size);
}
/**
* Frees all memory associated with the memory pool.
* @param mp A pointer to the pointer of the memory pool.
* @return 1 if successful, 0 if the pointer is invalid.
*/
int MP_Destroy(mp_t **mp) {
if (!mp || !*mp) return 0;
free((*mp)->blocks);
free((*mp)->block_status);
free(*mp);
*mp = NULL;
return 1;
}
Summary
The implementation provided demonstrates the core functions needed to manage a memory pool efficiently. By using these functions, you can allocate and deallocate memory blocks with better control and less overhead compared to standard memory management techniques. This approach is especially useful in scenarios where performance and predictability are critical.
Part 2: Sample Application
To illustrate the functionality of the memory pool, we present a sample application that leverages the memory pool functions we’ve implemented. This application demonstrates how to:
1. Create a Memory Pool:
We initialize a memory pool with a block size of 100 bytes and allocate 10 blocks. This step sets up the environment for managing memory efficiently.
2. Allocate and Write Data to Each Block:
The application allocates each of the 10 blocks using the MP_AllocBlock function. For each allocated block, it writes the block’s index value into its data segment. This helps in verifying that the allocation process is functioning correctly and that each block is accessible for storing data.
3. Attempt to Allocate an Additional Block:
After all blocks are allocated, the application tries to allocate one more block to demonstrate the pool's capacity limit. It’s expected that this allocation should fail, confirming that the pool is indeed full.
4. Display the Contents of Each Block:
The application retrieves and prints the data stored in each block using MP_GetBlockData. This step verifies that the data was correctly written into each block and is retrievable. It helps in ensuring that data integrity is maintained throughout the allocation process.
5. Free All Blocks and Destroy the Memory Pool:
Once all operations are complete, the application frees each block using MP_FreeBlock and then destroys the memory pool with MP_Destroy. This final step cleans up all allocated resources, ensuring no memory leaks occur and the pool is properly deallocated.
Here is the complete code for the sample application:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// Define the memory pool functions (Assumed to be provided in the same file or included)
mp_t *MP_Create(int blocksize, int num_blocks);
int MP_AllocBlock(mp_t *pool);
int MP_FreeBlock(mp_t *pool, int block_idx);
void *MP_GetBlockData(mp_t *pool, int block_idx);
int MP_Destroy(mp_t **mp);
int main() {
// Create a memory pool with block size of 100 bytes and 10 blocks
mp_t *pool = MP_Create(100, 10);
if (!pool) {
printf("Failed to create memory pool\n");
return 1;
}
// Allocate and write data to each block
for (int i = 0; i < 10; i++) {
int idx = MP_AllocBlock(pool);
if (idx != -1) {
*(unsigned int *)MP_GetBlockData(pool, idx) = (unsigned int)idx;
printf("Block %d allocated and written with value %x\n", idx, idx);
} else {
printf("Failed to allocate block\n");
}
}
// Attempt to allocate an additional block to show that the pool is full
int failed_idx = MP_AllocBlock(pool);
if (failed_idx == -1) {
printf("Block allocation failed as expected\n");
}
// Display the contents of each block
for (int i = 0; i < 10; i++) {
int *data = (int *)MP_GetBlockData(pool, i);
if (data) {
printf("Block %d contains value %x\n", i, *data);
}
}
// Free all blocks
for (int i = 0; i < 10; i++) {
MP_FreeBlock(pool, i);
}
// Destroy the memory pool
MP_Destroy(&pool);
return 0;
}
Explanation
1. Creating the Memory Pool:
The MP_Create function is used to set up the memory pool with the specified block size and number of blocks. This function ensures that all necessary memory allocations are performed and returns a pointer to the newly created memory pool. If the creation fails, an error message is displayed.
2. Allocating and Writing Data:
The application loops through and allocates each block using MP_AllocBlock. Each allocated block is then filled with its index value. This process verifies that the allocation function is working as intended and that each block is accessible for writing data.
3. Handling Full Pool:
After successfully filling all blocks, the application attempts to allocate an additional block. This operation is expected to fail, demonstrating the pool’s capacity limits. The application correctly handles this situation by acknowledging the failed allocation.
4. Displaying Block Contents:
The contents of each block are retrieved and displayed using MP_GetBlockData. This step ensures that the data written to the blocks is correctly stored and retrievable, verifying the integrity of the memory pool's data management.
5. Freeing and Destroying the Pool:
Finally, the application frees all allocated blocks and destroys the memory pool. This ensures that all allocated memory is properly deallocated, preventing memory leaks and ensuring that resources are released.
This sample application serves as a practical example of how to utilize memory pool functions to manage memory efficiently. It demonstrates allocation, data management, and cleanup procedures, providing a clear understanding of how to apply these concepts in real-world scenarios.
Conclusion
This blog provided a comprehensive and practical approach to implementing a memory pool system in C, focusing on the fundamental operations necessary for efficient memory management. We detailed the essential functions needed to create a memory pool, allocate and free blocks, retrieve block data, and destroy the pool, offering a foundational framework for managing memory in a controlled and efficient manner.
Understanding and applying these principles enables students to handle a wide range of assignments involving memory management with greater ease and precision. The ability to implement a memory pool effectively is crucial in various programming scenarios, from system-level programming to performance-critical applications, where efficient memory usage and reduction of fragmentation are key.
By mastering the implementation of memory pools, students not only enhance their technical skills but also gain insights into optimizing resource management in software development. This knowledge will serve as a valuable asset in both academic assignments and real-world projects, ensuring that you can approach memory management challenges with confidence and competence.
As you apply these concepts to similar assignments, you'll be better prepared to design robust and efficient memory management solutions, contributing to improved software performance and reliability.