Claim Your Offer
New semester, new challenges—but don’t stress, we’ve got your back! Get expert programming assignment help and breeze through Python, Java, C++, and more with ease. For a limited time, enjoy 10% OFF on all programming assignments with the Spring Special Discount! Just use code SPRING10OFF at checkout! Why stress over deadlines when you can score high effortlessly? Grab this exclusive offer now and make this semester your best one yet!
We Accept
- Understanding Doubly Linked Lists
- What is a Doubly Linked List?
- Common Operations on Doubly Linked Lists
- Key Tips for Solving Doubly Linked List Assignments
- Understand the Requirements
- Break Down the Problem
- Optimize Traversal
- Write and Test Incrementally
- Handle Edge Cases
- Use Helper Methods
- Efficiency Matters
- Advanced Techniques and Best Practices
- Using Sentinel Nodes
- Iterators
- Avoiding Common Mistakes
- Debugging Tips
- Practice with Real Problems
- Conclusion
Doubly Linked Lists are a cornerstone of computer science and a fundamental concept in many data structure assignments. Whether you're a beginner or an advanced programmer, understanding how to implement and manipulate a Doubly Linked List is essential. This guide will walk you through the basics, provide a step-by-step implementation, and offer tips to help you tackle similar programming assignments effectively.
Understanding Doubly Linked Lists
A Doubly Linked List is a collection of nodes, each containing three components: data, a reference to the next node, and a reference to the previous node. This structure allows for efficient insertion and deletion of elements from both ends of the list.
What is a Doubly Linked List?
A Doubly Linked List differs from a Singly Linked List in that each node has two pointers:
- Next: Points to the next node in the sequence.
- Previous: Points to the previous node in the sequence.
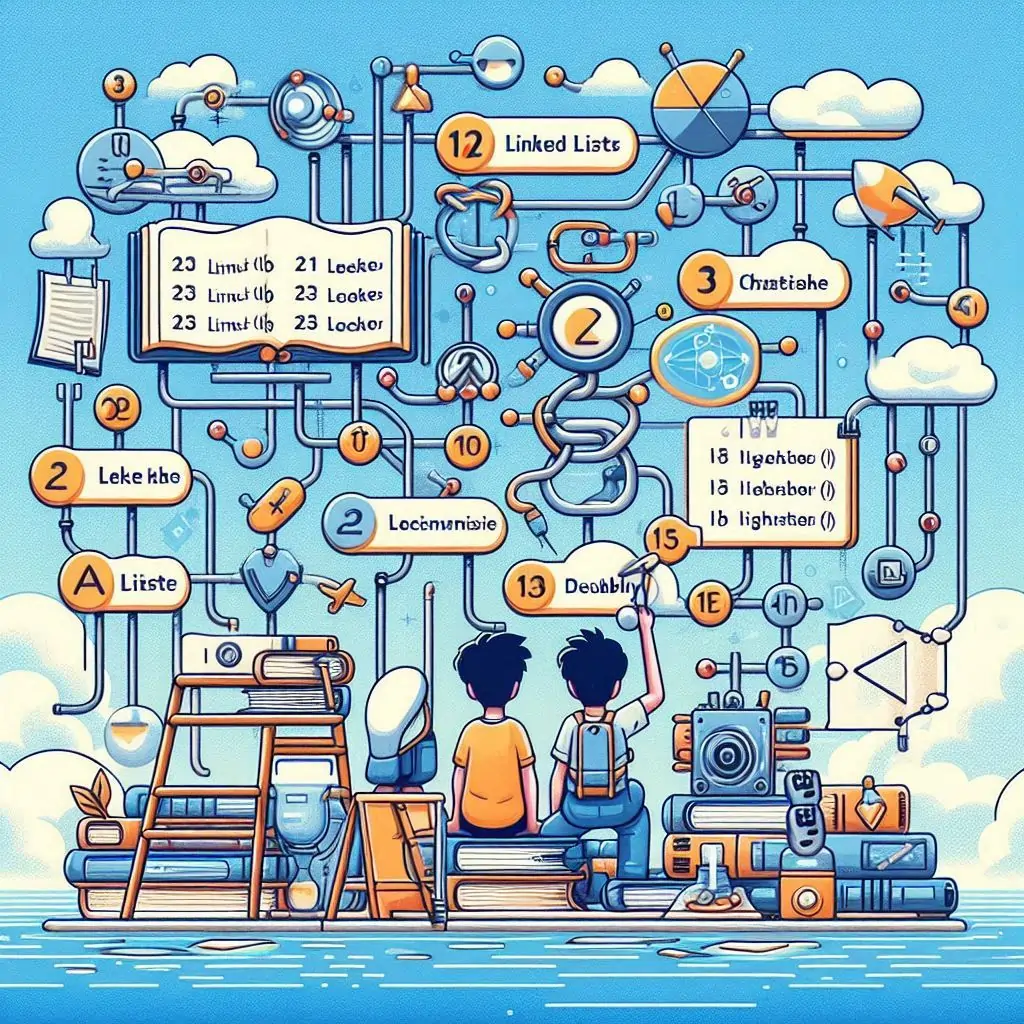
This bi-directional linking facilitates traversal from both the head and the tail, making operations like insertion and deletion more efficient.
Structure of a Doubly Linked List
In a Doubly Linked List:
- The head is the first node, and its previous reference is null.
- The tail is the last node, and its next reference is null.
- Intermediate nodes have both next and previous references pointing to their adjacent nodes.
Here’s a visual representation:
Head <-> Node1 <-> Node2 <-> ... <-> NodeN <-> Tail
Benefits of Using a Doubly Linked List
Doubly Linked Lists offer several advantages:
- Efficient Insertions and Deletions: You can insert or delete nodes at both ends in constant time.
- Bidirectional Traversal: Allows easy navigation forwards and backwards.
- Flexibility: Can be used in various applications like undo functionality in text editors, navigation in web browsers, and more.
Common Operations on Doubly Linked Lists
To master Doubly Linked Lists, you need to understand and implement several key operations:
- Adding Nodes: At the front, back, or any specified index.
- Removing Nodes: From the front, back, or any specified index.
- Traversing the List: From either end based on the required operation.
Let's dive deeper into these operations.
Adding Nodes
Adding nodes to a Doubly Linked List can be done in three ways:
- Add to Front: Insert a new node at the beginning.
- Add to Back: Append a new node at the end.
- Add at Index: Insert a new node at a specified position.
Here’s how you can implement these methods in Java:
public void addToFront(T data) {
DoublyLinkedListNode<T> newNode = new DoublyLinkedListNode<>(data);
if (isEmpty()) {
head = tail = newNode;
} else {
newNode.setNext(head);
head.setPrev(newNode);
head = newNode;
}
size++;
}
public void addToBack(T data) {
DoublyLinkedListNode<T> newNode = new DoublyLinkedListNode<>(data);
if (isEmpty()) {
head = tail = newNode;
} else {
newNode.setPrev(tail);
tail.setNext(newNode);
tail = newNode;
}
size++;
}
public void addAtIndex(int index, T data) {
if (index < 0 || index > size) throw new IndexOutOfBoundsException();
if (index == 0) {
addToFront(data);
} else if (index == size) {
addToBack(data);
} else {
DoublyLinkedListNode<T> newNode = new DoublyLinkedListNode<>(data);
DoublyLinkedListNode<T> current = head;
for (int i = 0; i < index; i++) {
current = current.getNext();
}
newNode.setNext(current);
newNode.setPrev(current.getPrev());
current.getPrev().setNext(newNode);
current.setPrev(newNode);
size++;
}
}
Removing Nodes
Similarly, nodes can be removed from a Doubly Linked List in three ways:
- Remove from Front: Delete the node at the beginning.
- Remove from Back: Delete the node at the end.
- Remove at Index: Delete the node at a specified position.
Here’s the implementation for these methods:
public T removeFromFront() {
if (isEmpty()) throw new NoSuchElementException();
T data = head.getData();
head = head.getNext();
if (head != null) {
head.setPrev(null);
} else {
tail = null;
}
size--;
return data;
}
public T removeFromBack() {
if (isEmpty()) throw new NoSuchElementException();
T data = tail.getData();
tail = tail.getPrev();
if (tail != null) {
tail.setNext(null);
} else {
head = null;
}
size--;
return data;
}
public T removeAtIndex(int index) {
if (index < 0 || index >= size) throw new IndexOutOfBoundsException();
if (index == 0) {
return removeFromFront();
} else if (index == size - 1) {
return removeFromBack();
} else {
DoublyLinkedListNode<T> current = head;
for (int i = 0; i < index; i++) {
current = current.getNext();
}
T data = current.getData();
current.getPrev().setNext(current.getNext());
current.getNext().setPrev(current.getPrev());
size--;
return data;
}
}
Traversing the List
Efficient traversal is key to optimizing your Doubly Linked List operations. Depending on the index, you may choose to start from the head or the tail to minimize the number of steps.
Here’s a basic traversal method to get a node at a specific index:
public T get(int index) {
if (index < 0 || index >= size) throw new IndexOutOfBoundsException();
DoublyLinkedListNode<T> current = (index < size / 2) ? head : tail;
if (current == head) {
for (int i = 0; i < index; i++) {
current = current.getNext();
}
} else {
for (int i = size - 1; i > index; i--) {
current = current.getPrev();
}
}
return current.getData();
}
Key Tips for Solving Doubly Linked List Assignments
When faced with an assignment involving Doubly Linked Lists, it's crucial to approach the problem methodically. Here are some tips to help you succeed.
Understand the Requirements
The first step is to thoroughly understand the assignment requirements. This involves:
- Reading the specifications: Pay close attention to the javadocs and any provided instructions.
- Identifying core functionalities: Determine the essential operations you need to implement.
Break Down the Problem
Dividing the problem into smaller, manageable parts can make it easier to tackle. Focus on implementing one feature at a time:
- Node Definition: Ensure your node class encapsulates the necessary data and references.
- List Initialization: Set up your list with head and tail references.
- Core Methods: Implement methods for adding, removing, and accessing nodes.
Optimize Traversal
Efficient traversal can significantly improve the performance of your Doubly Linked List. Depending on the index, start from the head or the tail:
- Closer to Head: Traverse from the head.
- Closer to Tail: Traverse from the tail.
This optimization minimizes the number of steps required to reach the desired node.
Write and Test Incrementally
Implementing and testing incrementally helps isolate and fix bugs early. Follow these steps:
- Write one method at a time: Focus on one operation before moving to the next.
- Test thoroughly: Use both provided tests and your own to ensure coverage of edge cases.
Handle Edge Cases
Edge cases can often cause unexpected issues. Consider scenarios like:
- Empty list: Handle operations when the list is empty.
- Single-element list: Manage cases where the list has only one node.
- Boundary indices: Ensure your methods correctly handle the first and last indices.
Use Helper Methods
Helper methods can encapsulate repetitive code, making your main methods cleaner and more maintainable:
- Encapsulation: Isolate complex logic into private methods.
- Reusability: Use these methods across multiple operations to avoid redundancy.
Efficiency Matters
Efficiency is critical, especially for frequently used operations. Aim for optimal time complexity:
- Constant Time: Operations like adding/removing from the front or back should ideally be O(1).
- Linear Time: Operations like adding/removing at an index or traversing the list may be O(n).
By focusing on these principles, you can create a robust and efficient Doubly Linked List.
Advanced Techniques and Best Practices
Beyond the basics, there are advanced techniques and best practices that can help you refine your Doubly Linked List implementation.
Using Sentinel Nodes
While the assignment specifies not to use phantom or sentinel nodes, it's worth understanding their role in more complex scenarios:
- Phantom Nodes: These are dummy nodes used to simplify edge cases at the head and tail.
- Usage: While not applicable here, in other contexts, sentinel nodes can streamline list operations by removing the need to check for null.
Iterators
Implementing an iterator can make traversal more intuitive and aligns with common Java practices:
- Iterator Interface: Implement the Iterator interface for your list.
- Traversal: This allows for enhanced for-loops and simplifies external iteration.
Here’s a simple example of an iterator for a Doubly Linked List:
public class DoublyLinkedListIterator<T> implements Iterator<T> {
private DoublyLinkedListNode<T> current;
public DoublyLinkedListIterator(DoublyLinkedListNode<T> head) {
current = head;
}
@Override
public boolean hasNext() {
return current != null;
}
@Override
public T next() {
if (!hasNext()) throw new NoSuchElementException();
T data = current.getData();
current = current.getNext();
return data;
}
}
Avoiding Common Mistakes
Avoiding common mistakes can save you time and frustration:
- Null Checks: Always check for null references when accessing node pointers.
- Boundary Conditions: Handle indices at the boundaries (0 and size-1) carefully.
- Consistency: Ensure that all list modifications maintain the integrity of the previous and next references.
Debugging Tips
Effective debugging is crucial for resolving issues:
- Print Statements: Use print statements to track the flow and state of your list.
- Debuggers: Utilize debugging tools to step through your code and inspect variables.
- Unit Tests: Write comprehensive unit tests to cover all possible scenarios and edge cases.
Practice with Real Problems
To master Doubly Linked Lists, practice with real-world problems:
- Coding Challenges: Participate in coding challenges and competitions.
- Open-Source Projects: Contribute to open-source projects that use linked lists.
By applying these advanced techniques and best practices, you can refine your skills and become proficient in working with Doubly Linked Lists.
Conclusion
Mastering Doubly Linked Lists is a crucial step in becoming a proficient programmer. By understanding their structure, implementing core operations, and applying advanced techniques, you can tackle assignments with confidence. Remember to break down problems, optimize traversal, handle edge cases, and practice regularly. With these strategies, you'll be well-equipped to handle any Doubly Linked List challenge that comes your way.