Claim Your Offer
Unlock an amazing offer at www.programminghomeworkhelp.com with our latest promotion. Get an incredible 10% off on your all programming assignment, ensuring top-quality assistance at an affordable price. Our team of expert programmers is here to help you, making your academic journey smoother and more cost-effective. Don't miss this chance to improve your skills and save on your studies. Take advantage of our offer now and secure exceptional help for your programming assignments.
We Accept
- Understanding the Problem
- Key Concepts
- Graph Representation
- Grid Graph Structure
- Vertex and Edge Definitions
- Transforming the Reliability Problem
- Implementation Steps
- 1. Read and Parse Graph Input
- 2. Implement the Graph Data Structure
- 3. Implement Dijkstra’s Algorithm
- 4. Output the Results
- Example Implementation
- Conclusion
Dijkstra’s Algorithm is a fundamental algorithm in computer science used to find the shortest path from a source vertex to all other vertices in a graph with non-negative edge weights. However, in certain applications, like network reliability or probabilistic graph problems, finding the “shortest” path is not always straightforward. Instead, we might be interested in finding the most “reliable” path, where edge weights are probabilities representing the reliability of the path. This blog aims to provide you with a comprehensive guide on how to approach and solve graph-based algorithm assignments, particularly those that involve finding the most reliable path in a weighted, undirected graph using Dijkstra's algorithm.
While we will reference a specific data structure assignment that requires the implementation of Dijkstra's algorithm to find the most reliable path in a weighted, undirected grid graph, the strategies discussed here will be applicable to a broad range of similar problems.
Understanding the Problem
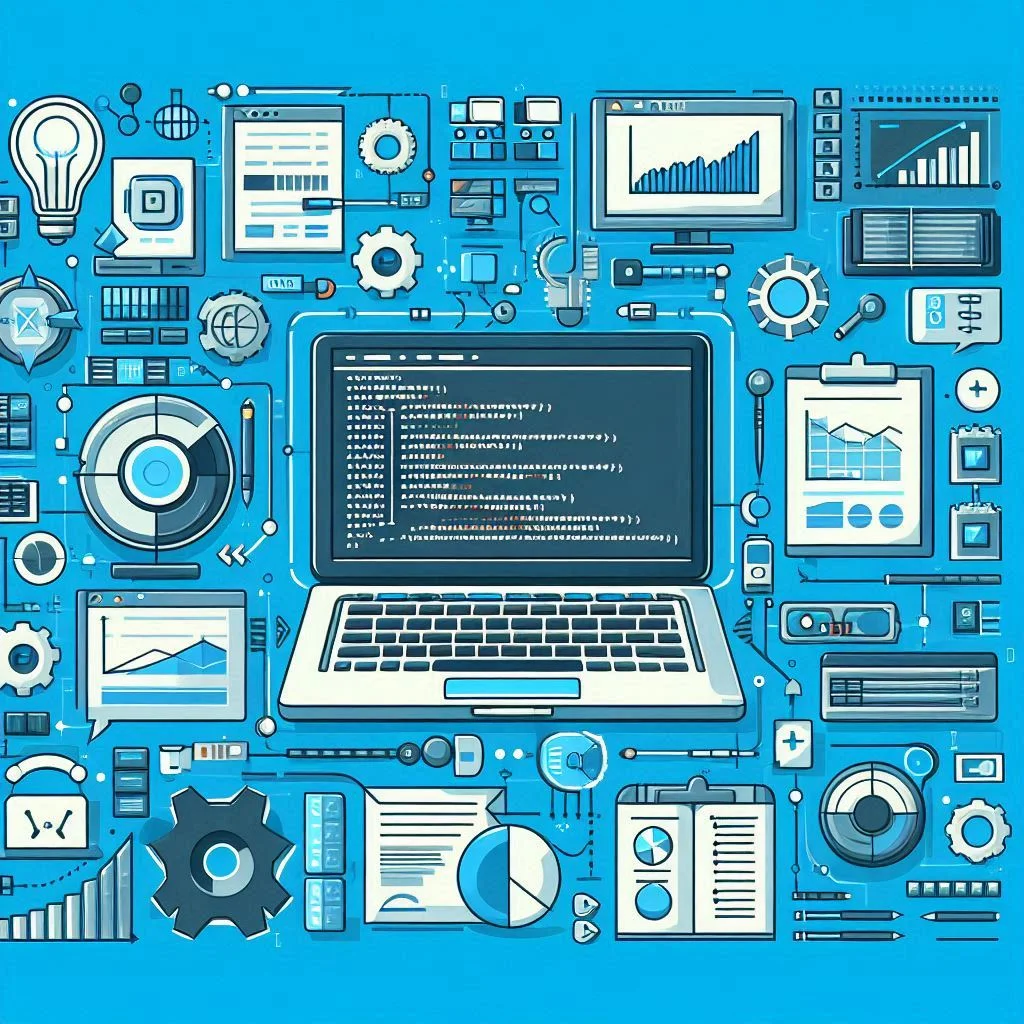
Before diving into the implementation, it's essential to understand the problem thoroughly. This problem typically involves a graph where nodes represent entities, and edges represent the relationships between them. In this specific case, the graph is undirected, meaning that the edges have no direction, and the graph is weighted, meaning that each edge has a numerical value (weight) associated with it.
Key Concepts
- Graph: A collection of vertices (nodes) connected by edges (lines).
- Undirected Graph: A graph where edges have no direction, meaning the relationship between two nodes is mutual.
- Weighted Graph: A graph where edges have numerical values representing cost, distance, or, in this case, reliability.
- Grid Graph: A special type of graph where nodes are arranged in a grid-like structure, and each node is connected to its adjacent nodes.
- Dijkstra’s Algorithm: An algorithm used to find the shortest (or in this case, most reliable) path between a source node and all other nodes in the graph.
In the context of your java assignment, the goal is to implement Dijkstra's algorithm to determine the most reliable path between a source node and a destination node in a weighted, undirected grid graph. The reliability of a path is defined as the product of the edge weights along that path.
Graph Representation
Grid Graph Structure
A grid graph is a special type of graph that has a grid-like structure, with vertices organized in a regular pattern, much like a chessboard. Each vertex is connected to its adjacent vertices in a grid pattern, forming a lattice of edges. In such a graph, edge weights are often represented as probabilities, indicating the likelihood of successful transmission between connected nodes.
Vertex and Edge Definitions
- Vertex (MyVertex Class): In the given problem, each vertex is represented by the MyVertex class. This class encapsulates properties such as the vertex label, distance from the source, and predecessor vertex. It also supports methods to get and set these properties.
- Edge (MyWeightedUndirectedGraph Interface): The MyWeightedUndirectedGraph interface defines basic operations for handling edges in an undirected, weighted graph. It includes methods for reading vertices, retrieving neighbors, and managing edge weights.
Transforming the Reliability Problem
To use Dijkstra’s Algorithm, which is designed for shortest-path problems, for finding the most reliable path, we need to transform the problem from maximizing the product of edge weights to minimizing the sum of transformed edge weights. This transformation leverages the properties of logarithms:
- Logarithmic Transformation: The key insight is to use logarithms to transform the product of probabilities into a sum. For a path with edge weights w1,w2,…,wn the reliability is given by the product w1×w2×⋯×wn . Taking the logarithm, we convert this product into a sum of logarithms:
log(w1×w2×⋯×wn)=log(w1)+log(w2)+⋯+log(wn)
Since log(w) is negative for w<1, minimizing the sum of logarithms is equivalent to maximizing the product of the probabilities.
Implementation Steps
1. Read and Parse Graph Input
The first step in implementing the algorithm is to read and parse the graph input. The input format typically consists of a number of edges followed by the edges themselves, each defined by two vertices and a weight. The read method in the MyWeightedUndirectedGraph interface is responsible for this task.
- Reading the Graph: The graph is read from standard input or a file. For each edge, the vertices and weight are read, and the weight is transformed using the logarithm function. This transformation allows us to use Dijkstra's Algorithm on the transformed weights.
2. Implement the Graph Data Structure
A concrete implementation of the MyWeightedUndirectedGraph interface needs to efficiently store and manage the graph data. This typically involves:
- Storing Vertices and Edges: Using data structures like HashMap to map vertices to their neighbors and edge weights.
- Handling Vertex Properties: Storing properties like distance from the source and predecessor for each vertex.
3. Implement Dijkstra’s Algorithm
Dijkstra’s Algorithm is used to compute the shortest path based on the transformed edge weights. The core of Dijkstra's Algorithm involves:
- Priority Queue: Using a priority queue to efficiently retrieve and update vertices with the minimum distance. The MyPriorityQueue interface defines operations for managing the priority queue.
- Relaxation: For each vertex, update the distances to its neighbors if a shorter path is found. This step uses the logarithmically transformed weights.
4. Output the Results
Once Dijkstra’s Algorithm completes, the most reliable path can be reconstructed from the predecessor information. The output should include:
- Path Reconstruction: Starting from the destination vertex, trace back through the predecessor vertices to reconstruct the path.
- Reliability Calculation: Compute the reliability of the path by exponentiating the sum of transformed weights.
Example Implementation
Here is a simplified example implementation of Dijkstra’s Algorithm applied to a grid graph for finding the most reliable path:
import java.util.*;
public class MyWeightedUndirectedGraphLab11 implements MyWeightedUndirectedGraph {
private Map<MyVertex, Map<MyVertex, Double>> adjacencyList;
public MyWeightedUndirectedGraphLab11() {
this.adjacencyList = new HashMap<>();
}
@Override
public MyVertex read(Scanner in) {
int numEdges = in.nextInt();
MyVertex source = null;
for (int i = 0; i < numEdges; i++) {
String label1 = in.next();
String label2 = in.next();
double weight = in.nextDouble();
MyVertex vtx1 = new MyVertex(label1);
MyVertex vtx2 = new MyVertex(label2);
if (source == null) source = vtx1;
addEdge(vtx1, vtx2, -Math.log(weight)); // Transform weight
}
return source;
}
@Override
public Set<MyVertex> vertexSet() {
return adjacencyList.keySet();
}
@Override
public MyVertex locate(MyVertex inVtx) {
return inVtx;
}
@Override
public Set<MyVertex> neightborsOf(MyVertex inVtx) {
return adjacencyList.getOrDefault(inVtx, Collections.emptyMap()).keySet();
}
@Override
public void addEdge(MyVertex vtx1, MyVertex vtx2, Double inWeight) {
adjacencyList.computeIfAbsent(vtx1, k -> new HashMap<>()).put(vtx2, inWeight);
adjacencyList.computeIfAbsent(vtx2, k -> new HashMap<>()).put(vtx1, inWeight);
}
@Override
public double weightOfEdge(MyVertex vtx1, MyVertex vtx2) {
return adjacencyList.getOrDefault(vtx1, Collections.emptyMap()).getOrDefault(vtx2, Double.MAX_VALUE);
}
public static void dijkstra(MyWeightedUndirectedGraph graph, MyVertex source) {
source.setDistanceFromSource(0);
source.setKnown(false);
PriorityQueue<MyVertex> pq = new PriorityQueue<>(Comparator.comparingDouble(MyVertex::getDistanceFromSource));
pq.add(source);
while (!pq.isEmpty()) {
MyVertex u = pq.poll();
u.setKnown(true);
for (MyVertex v : graph.neightborsOf(u)) {
if (!v.isKnown()) {
double newDist = u.getDistanceFromSource() + graph.weightOfEdge(u, v);
if (newDist < v.getDistanceFromSource()) {
v.setDistanceFromSource(newDist);
v.setPred(u);
pq.add(v);
}
}
}
}
}
public static void printPathFromSourceTo(MyVertex target) {
if (target == null) return;
List<String> path = new ArrayList<>();
double cost = 0.0;
while (target != null) {
path.add(target.getLabel());
if (target.getPred() != null) {
cost += Math.exp(-target.getDistanceFromSource()); // Inverse of transformed weight
}
target = target.getPred();
}
Collections.reverse(path);
System.out.println("Path=" + String.join("->", path));
System.out.println("Cost=" + cost);
}
public static void main(String[] args) throws Exception {
MyWeightedUndirectedGraph graph = new MyWeightedUndirectedGraphLab11();
Scanner sc = new Scanner(System.in);
MyVertex source = graph.read(sc);
System.out.print("Executing Dijkstra's algorithm...");
long start = System.currentTimeMillis();
dijkstra(graph, source);
long end = System.currentTimeMillis();
System.out.println("Done, time=" + (end - start));
System.out.print("Print the most reliable path from " + source + " to: ");
MyVertex destination = new MyVertex(sc.next());
printPathFromSourceTo(destination);
System.out.println("Done. Normal Termination.");
}
}
Conclusion
Dijkstra’s Algorithm is a versatile tool for finding shortest paths in graphs, but its application can be extended to more complex problems like finding the most reliable path in a grid graph. By transforming the problem into a format suitable for Dijkstra’s Algorithm, we can effectively leverage its efficiency and accuracy. This guide has detailed the transformation process, implementation steps, and provided practical code examples to help you solve your programming assignments. Remember to test thoroughly and optimize your implementation for better performance in larger graphs.