Claim Your Discount Today
Kick off the fall semester with a 20% discount on all programming assignments at www.programminghomeworkhelp.com! Our experts are here to support your coding journey with top-quality assistance. Seize this seasonal offer to enhance your programming skills and achieve academic success. Act now and save!
We Accept
- Planning and Requirements Gathering
- Define the Objectives
- Gather Functional Requirements
- Identify Non-Functional Requirements
- System Design
- Database Design
- User Interface Design
- System Architecture
- Implementation
- Setting Up the Development Environment
- Developing the Modules
- Testing and Deployment
- Testing the Application
- Deploying the Application
- Maintenance and Updates
- Regular Maintenance
- Feature Updates
Creating a comprehensive restaurant management system using Java involves a meticulous approach to planning, designing, and implementing various components that work seamlessly together. This guide will cover the essential steps and considerations for building a Java application using JDBC and a SQL database. By following these guidelines, you will be able to create an efficient and functional application to automate a restaurant's daily operations. Whether you need help with Java assignment or are looking to enhance your programming skills, this guide will be a valuable resource.
Planning and Requirements Gathering
Developing a successful restaurant management system starts with thorough planning and gathering detailed requirements. This stage lays the foundation for all subsequent development efforts and ensures that the final product meets the needs of its users.
Define the Objectives
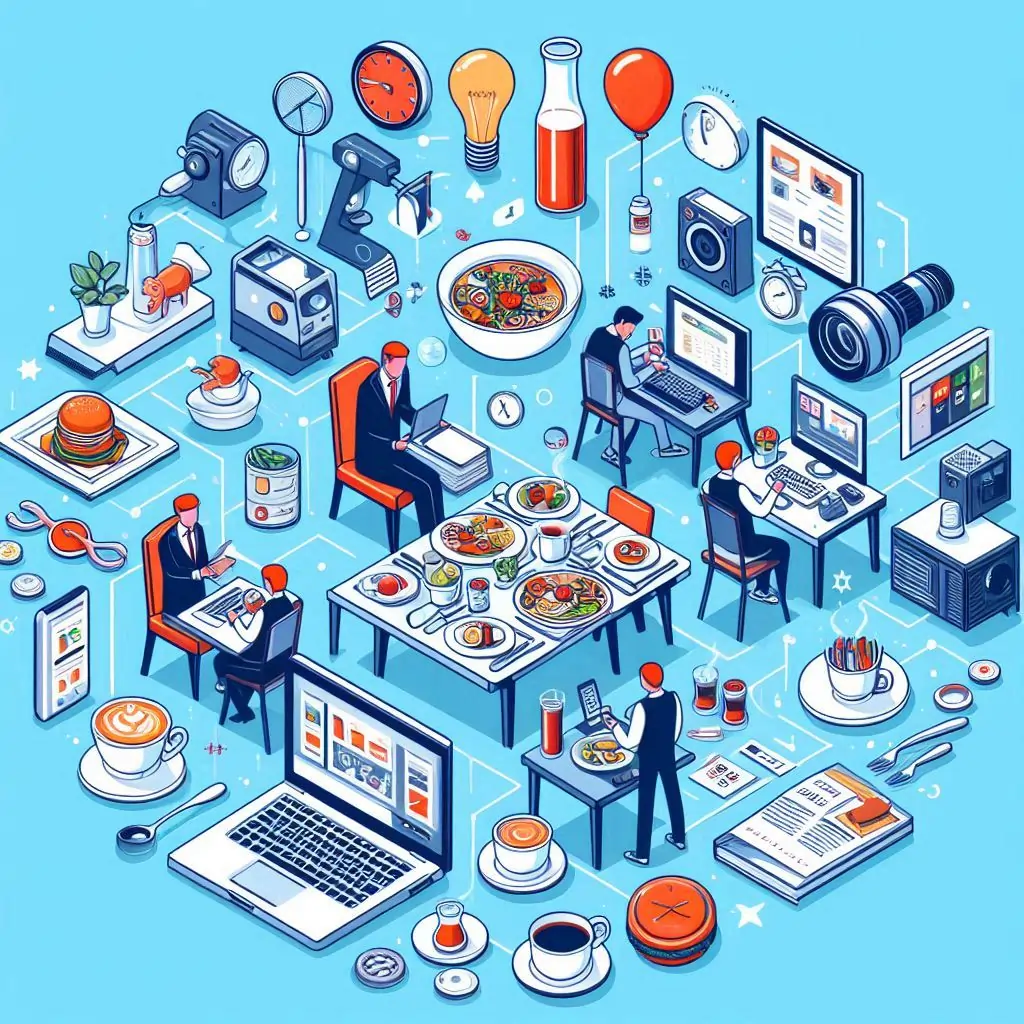
The primary step in any project is to clearly define its objectives. For a restaurant management system, the goals typically include automating and streamlining various processes to improve efficiency and customer service. Key objectives might include:
- Automating Table Management: Allowing staff to easily view and update table statuses.
- Streamlining Order Processing: Enabling efficient communication between waitstaff and kitchen staff.
- Simplifying Employee Management: Providing managers with tools to manage employee schedules, payroll, and performance.
Having clear objectives helps in setting the right direction for the project and ensures that all stakeholders are on the same page regarding what the final product should achieve.
Gather Functional Requirements
Functional requirements specify what the system should do. They are the features and capabilities that the application must possess to meet the needs of its users. For a restaurant management system, functional requirements might include:
- User Login and Authentication: Employees, including waitstaff, busboys, chefs, and managers, must log in with their credentials.
- Table Status Management: The ability to view and update the status of each table (e.g., open, occupied, dirty).
- Order Taking and Processing: Functionality for taking orders, sending them to the kitchen, and marking them as complete.
- Employee Management: Tools for managers to add, edit, and remove employees, manage schedules, and process payroll.
- Report Generation: The ability to generate reports on sales, employee performance, and other key metrics.
These functional requirements form the backbone of the application, guiding the design and implementation of various features.
Identify Non-Functional Requirements
Non-functional requirements define how the system performs its tasks rather than what tasks it performs. They are crucial for ensuring that the application is user-friendly, reliable, and efficient. Common non-functional requirements for a restaurant management system include:
- Usability: The application should have an intuitive and user-friendly interface that is easy to navigate.
- Performance: Screens should load quickly, ideally within 3 seconds, to ensure a smooth user experience.
- Security: Data must be handled securely, with proper encryption and access controls to protect sensitive information.
- Scalability: The system should be able to handle an increasing number of users and transactions as the restaurant grows.
- Reliability: The application should be robust and reliable, with minimal downtime and quick recovery from any issues.
By identifying these non-functional requirements, you can ensure that the application not only meets functional needs but also provides a high-quality user experience.
System Design
Designing the system involves creating a blueprint that outlines how the application will be structured and how different components will interact. This stage is critical for ensuring that the system is well-organized and efficient.
Database Design
A well-designed database is essential for managing the data that the application will handle. The process involves:
- Identifying Entities and Relationships: Determine the main entities (e.g., employees, tables, orders, menu items) and their relationships. For example, each order is associated with a table and a waiter, and each employee has login credentials and work hours.
- Creating ER Diagrams: Use entity-relationship diagrams to visualize the database structure. This helps in understanding how different entities are connected and how data flows through the system.
- Normalization: Ensure the database is normalized to eliminate redundancy and improve efficiency. This involves organizing the data into tables and defining relationships to minimize duplication and ensure data integrity.
A robust database design ensures that the application can efficiently store, retrieve, and manage data, which is crucial for its overall performance.
User Interface Design
The user interface (UI) is the part of the application that users interact with directly. It should be intuitive, visually appealing, and easy to use. Key considerations for UI design include:
- Table Layout: Provide a visual representation of the restaurant’s tables, showing their status (e.g., open, occupied, dirty). This helps staff quickly understand the current state of the restaurant and manage tables effectively.
- Order Screens: Design interfaces for taking orders, sending them to the kitchen, and marking them as complete. These screens should be straightforward and minimize the steps required to perform each task.
- Management Dashboard: Create a dashboard for managers to view reports, manage employees, and perform other administrative tasks. This dashboard should provide a comprehensive overview of the restaurant’s operations and key metrics.
By focusing on these key elements, you can create a user interface that enhances the overall usability of the application and makes it easy for staff to perform their tasks efficiently.
System Architecture
Choosing the right architecture for the application is crucial for ensuring that it is scalable, maintainable, and efficient. The Model-View-Controller (MVC) pattern is a popular choice for such applications because it separates concerns and makes the system easier to manage:
- Model: Manages the data and business logic of the application. It interacts with the database and ensures data integrity.
- View: Handles the presentation layer, displaying information to the user and collecting user input.
- Controller: Acts as an intermediary between the model and the view, processing user input and updating the view based on changes in the model.
Adopting the MVC pattern helps in organizing the codebase, making it easier to maintain and extend the application as new features are added or requirements change.
Implementation
The implementation phase involves turning the designs and plans into actual code. This stage requires careful attention to detail to ensure that the application functions as intended and meets all requirements.
Setting Up the Development Environment
Preparing the development environment is the first step in the implementation process. This involves:
- Installing the Java Development Kit (JDK) 11: JDK 11 provides the necessary tools to develop Java applications. It offers improved performance and security features, making it a suitable choice for building robust applications.
- Choosing an Integrated Development Environment (IDE): An IDE like Eclipse or IntelliJ IDEA provides tools for coding, debugging, and testing the application. These IDEs offer features like code completion, syntax highlighting, and integrated debugging, which can significantly enhance productivity.
- Setting Up a Database Management System (DBMS): Use MySQL for managing the database. MySQL is a popular choice for web applications due to its reliability, scalability, and robust feature set.
Setting up the development environment correctly ensures that the development process is smooth and efficient, with minimal technical issues.
Developing the Modules
Breaking down the application into smaller, manageable modules makes the development process more organized and manageable. Key modules for a restaurant management system include:
- Login Module: Implement user authentication and authorization. This involves creating login screens, validating user credentials, and ensuring secure access to the application.
- Table Management Module: Develop functionalities for viewing and updating table statuses. This includes displaying the current status of each table (e.g., open, occupied, dirty) and allowing staff to update these statuses as needed.
- Order Processing Module: Create interfaces for taking orders, sending them to the kitchen, and marking them as complete. This module should streamline the order-taking process and ensure that orders are accurately communicated to the kitchen.
- Employee Management Module: Implement functionalities for adding, editing, and removing employees, managing their schedules, and processing payroll. This module provides managers with tools to efficiently manage staff and ensure smooth operations.
- Report Generation Module: Develop tools for generating reports on sales, employee performance, and other key metrics. This module helps managers make informed decisions based on accurate data.
By focusing on these key modules, you can ensure that the application is well-organized and that each component works together seamlessly.
Testing and Deployment
Testing and deployment are critical stages in the development process, ensuring that the application is reliable and ready for use in a live environment.
Testing the Application
Thorough testing is essential for identifying and fixing any issues before the application is deployed. Key types of testing include:
- Unit Testing: Test individual components to ensure that each one functions as expected. This involves writing test cases for each module and verifying that they produce the correct outputs.
- Integration Testing: Ensure that different modules work together seamlessly. This involves testing the interactions between modules to identify and fix any issues that arise from their integration.
- User Acceptance Testing (UAT): Get feedback from potential users to ensure that the application meets their needs. This involves having real users test the application in a controlled environment and providing feedback on its usability and functionality.
By conducting thorough testing, you can ensure that the application is reliable and performs as expected in a live environment.
Deploying the Application
Deploying the application involves making it available for use in a live environment. Key steps in the deployment process include:
- Server Setup: Configure servers to host the application and database. This involves setting up the necessary hardware and software infrastructure to support the application.
- Data Migration: Transfer any existing data to the new system. This involves importing data from previous systems or databases and ensuring that it is accurately represented in the new application.
- User Training: Provide training to users on how to use the new system effectively. This involves creating user manuals, conducting training sessions, and offering ongoing support to help users get up to speed with the new application.
By following these steps, you can ensure a smooth deployment process and minimize any disruptions to restaurant operations.
Maintenance and Updates
Once the application is deployed, ongoing maintenance and updates are essential for ensuring that it continues to perform well and meets the evolving needs of the business.
Regular Maintenance
Regular maintenance is crucial for keeping the application running smoothly and addressing any issues that arise. Key maintenance tasks include:
- Bug Fixes: Address any issues or bugs that are reported by users. This involves investigating the cause of the issues and implementing fixes to resolve them.
- Performance Optimization: Continuously optimize the application for better performance. This involves monitoring the application’s performance and making adjustments to improve its speed and efficiency.
- Security Updates: Implement security updates to protect against potential threats. This involves staying up-to-date with the latest security patches and best practices to ensure that the application is secure.
By performing regular maintenance, you can ensure that the application remains reliable and continues to meet the needs of its users.
Feature Updates
Keeping the application updated with new features and improvements is essential for maintaining its relevance and value. Key considerations for feature updates include:
- Client Feedback: Incorporate feedback from users to enhance the application. This involves listening to user suggestions and making improvements based on their needs and preferences.
- Technology Upgrades: Update the application to use the latest technologies and frameworks. This involves staying current with industry trends and implementing updates to keep the application modern and efficient.
- New Features: Add new features to improve the functionality of the application. This involves identifying areas for improvement and implementing new features to enhance the user experience.
By focusing on these areas, you can ensure that the application continues to evolve and provide value to its users. Developing a Java-based restaurant management system involves careful planning, detailed design, and meticulous implementation. By following the steps outlined in this guide, you can create a comprehensive and efficient system that meets the needs of restaurant staff and managers, ultimately improving the overall dining experience for customers.