Claim Your Discount Today
Ring in Christmas and New Year with a special treat from www.programminghomeworkhelp.com! Get 15% off on all programming assignments when you use the code PHHCNY15 for expert assistance. Don’t miss this festive offer—available for a limited time. Start your New Year with academic success and savings. Act now and save!
We Accept
- Understanding the Basics
- Starting with Provided Code
- Enhancing the Basic Game
- Adding Obstacles
- Implementing a Scoring System
- Adding Enemy Movement
- Conclusion
Developing interactive console games with C++ offers an exciting opportunity to advance programming skills and unleash creative potential, while also providing valuable assistance with C++ assignment. Whether you're just starting or have programming experience, this guide equips you with foundational knowledge and essential tools to craft compelling console games. Through practical examples and step-by-step instructions, you'll learn how to leverage provided code samples to create your own interactive gaming experiences. From mastering input handling and implementing game loops to enhancing rendering techniques and refining game logic, each concept builds upon the next, empowering you to design dynamic and engaging games. Whether you aspire to create maze navigators, text-based adventures, or simple simulations, this guide provides a solid framework to explore and expand your game development skills in C++. Dive into the world of console game programming and discover the thrill of bringing your ideas to life through interactive gameplay and immersive user experiences.
Understanding the Basics
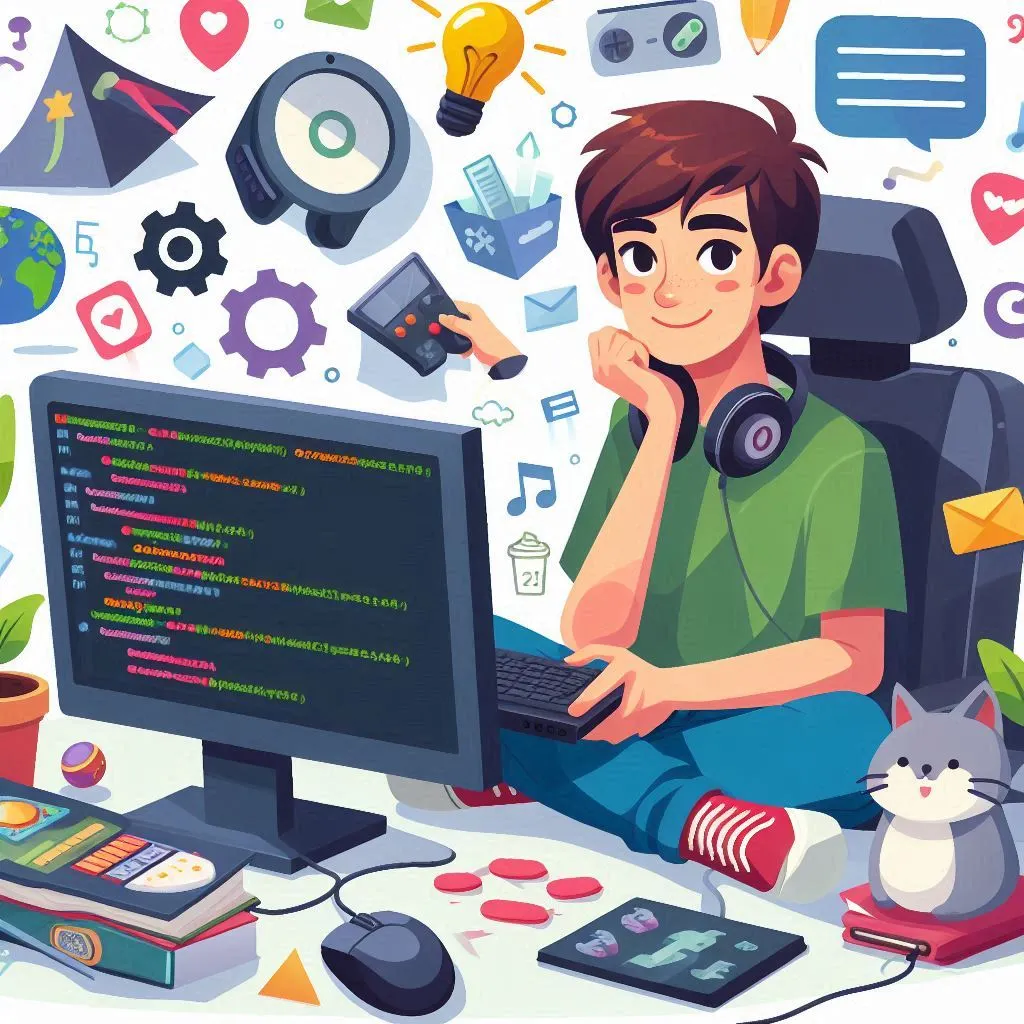
In developing interactive console games with C++, it's essential to grasp the foundational components that constitute the gameplay experience:
- Input Handling: This crucial aspect involves detecting and responding to user inputs, such as keyboard presses. Effective input handling ensures smooth interaction between the player and the game, enabling responsive gameplay mechanics.
- Game Loop: At the heart of every game is the game loop, a continuous process that updates the game state based on user inputs and internal logic. It ensures that the game responds in real-time to player actions while managing the flow of gameplay.
- Rendering: Rendering focuses on presenting the game state visually on the console screen. It involves translating the internal game data into graphical or textual representations that users can see and interact with, enhancing the immersive experience.
- Game Logic: The rules and mechanics of the game define its behavior and structure. Game logic governs how the game progresses, handles interactions between game elements, and implements win or lose conditions, providing the framework for gameplay dynamics.
Understanding these fundamental components lays a solid foundation for effectively developing interactive console games in C++, empowering developers to create engaging and enjoyable gaming experiences.
Starting with Provided Code
We begin with a simple example of code that demonstrates character movement on a console screen. The provided code initializes a console application where an "@" character navigates a 20x20 grid:
#include <iostream>
#include <Windows.h>
const HANDLE hConsole = GetStdHandle(STD_OUTPUT_HANDLE);
void ShowConsoleCursor(bool showFlag)
{
CONSOLE_CURSOR_INFO cursorInfo;
GetConsoleCursorInfo(hConsole, &cursorInfo);
cursorInfo.bVisible = showFlag;
SetConsoleCursorInfo(hConsole, &cursorInfo);
}
int main()
{
ShowConsoleCursor(false);
const int BOARD_SIZE = 20;
SetConsoleTextAttribute(hConsole, 15);
COORD pos;
pos.X = 4;
pos.Y = 10;
bool update = true;
while (true)
{
if (update)
{
system("cls");
SetConsoleCursorPosition(hConsole, pos);
std::cout << "@";
update = false;
}
if (GetAsyncKeyState(0x25) && pos.X > 0) // left
{
pos.X -= 1;
update = true;
}
else if (GetAsyncKeyState(0x27) && pos.X < BOARD_SIZE - 1) // right
{
pos.X += 1;
update = true;
}
if (GetAsyncKeyState(0x26) && pos.Y > 0) // up
{
pos.Y--;
update = true;
}
else if (GetAsyncKeyState(0x28) && pos.Y < BOARD_SIZE - 1) // down
{
pos.Y++;
update = true;
}
Sleep(40);
}
return 0;
}
This code snippet establishes a basic framework for a console game, where the "@" symbol moves within the grid in response to arrow key inputs. The ShowConsoleCursor function hides the cursor to enhance the visual experience of the game, while the SetConsoleTextAttribute function sets the text color to white. The game loop continuously updates the character's position based on user input, demonstrating fundamental concepts of input handling and console rendering in game development with C++.
Enhancing the Basic Game
Now that we have established a foundational setup for our console game, let's explore ways to enrich the gameplay experience with additional features:
- Obstacle Avoidance: Introduce obstacles scattered throughout the grid that the "@" character must navigate around. This adds an element of strategy and challenge, requiring players to plan their movements carefully to avoid collisions.
- Score System: Implement a scoring mechanism that rewards players based on their performance. Points can be awarded for each successful movement or for collecting items placed within the grid. This encourages replayability and competition among players to achieve higher scores.
- Enemy Movement: Introduce dynamic enemies that move independently within the grid, posing a threat to the player-controlled character. Enemies could pursue the player or patrol specific areas, adding a layer of suspense and requiring players to devise evasion strategies.
- Game Over Conditions: Define clear conditions that signal the end of the game, such as colliding with an enemy or obstacle, running out of health or lives, or reaching a specific time limit. Game over scenarios provide closure to gameplay sessions and motivate players to improve their skills for subsequent attempts.
By incorporating these features into our basic game framework, we can transform a simple movement exercise into a dynamic and engaging console gaming experience. These enhancements not only add complexity and depth to gameplay but also showcase the versatility and creativity achievable through C++ game development.
Adding Obstacles
Let's enhance our game by introducing obstacles into the grid. By modifying the code, we can incorporate a grid layout with obstacles represented by a designated character, such as '#'. These obstacles will obstruct the movement of our player character '@', adding a layer of challenge and strategy to the gameplay. Through this modification, we ensure that the player must navigate carefully around these obstacles to avoid collisions and progress through the game effectively.
#include <iostream>
#include <fstream>
#include <string>
#include <Windows.h>
#include <array>
#include <vector>
const HANDLE hConsole = GetStdHandle(STD_OUTPUT_HANDLE);
using namespace std;
void ShowConsoleCursor(bool showFlag)
{
CONSOLE_CURSOR_INFO cursorInfo;
GetConsoleCursorInfo(hConsole, &cursorInfo);
cursorInfo.bVisible = showFlag;
SetConsoleCursorInfo(hConsole, &cursorInfo);
}
int main()
{
ShowConsoleCursor(false);
const int BOARD_SIZE = 20;
const char OBSTACLE = '#';
char grid[BOARD_SIZE][BOARD_SIZE] = {};
// Place obstacles on the grid
for (int i = 0; i < BOARD_SIZE; ++i)
{
for (int j = 0; j < BOARD_SIZE; ++j)
{
if ((i + j) % 5 == 0) // Simple pattern for obstacles
{
grid[i][j] = OBSTACLE;
}
}
}
COORD pos;
pos.X = 4;
pos.Y = 10;
bool update = true;
while (true)
{
if (update)
{
system("cls");
for (int i = 0; i < BOARD_SIZE; ++i)
{
for (int j = 0; j < BOARD_SIZE; ++j)
{
if (i == pos.Y && j == pos.X)
{
cout << "@";
}
else
{
cout << grid[i][j];
}
}
cout << endl;
}
update = false;
}
if (GetAsyncKeyState(0x25) && pos.X > 0 && grid[pos.Y][pos.X - 1] != OBSTACLE) // left
{
pos.X -= 1;
update = true;
}
else if (GetAsyncKeyState(0x27) && pos.X < BOARD_SIZE - 1 && grid[pos.Y][pos.X + 1] != OBSTACLE) // right
{
pos.X += 1;
update = true;
}
if (GetAsyncKeyState(0x26) && pos.Y > 0 && grid[pos.Y - 1][pos.X] != OBSTACLE) // up
{
pos.Y--;
update = true;
}
else if (GetAsyncKeyState(0x28) && pos.Y < BOARD_SIZE - 1 && grid[pos.Y + 1][pos.X] != OBSTACLE) // down
{
pos.Y++;
update = true;
}
Sleep(40);
}
return 0;
}
Implementing a Scoring System
Next, we'll implement a scoring system to track the player's progress and performance within the game. The score will increment as the character moves across the grid, rewarding players for their navigation skills and strategic decisions. This addition not only enhances the competitive aspect of the game but also motivates players to improve their gameplay by aiming for higher scores with each session. Displaying the score prominently on the console screen ensures that players can easily track their achievements and engage more deeply with the gaming experience.
#include <iostream>
#include <fstream>
#include <string>
#include <Windows.h>
#include <array>
#include <vector>
const HANDLE hConsole = GetStdHandle(STD_OUTPUT_HANDLE);
using namespace std;
void ShowConsoleCursor(bool showFlag)
{
CONSOLE_CURSOR_INFO cursorInfo;
GetConsoleCursorInfo(hConsole, &cursorInfo);
cursorInfo.bVisible = showFlag;
SetConsoleCursorInfo(hConsole, &cursorInfo);
}
int main()
{
ShowConsoleCursor(false);
const int BOARD_SIZE = 20;
const char OBSTACLE = '#';
char grid[BOARD_SIZE][BOARD_SIZE] = {};
int score = 0;
for (int i = 0; i < BOARD_SIZE; ++i)
{
for (int j = 0; j < BOARD_SIZE; ++j)
{
if ((i + j) % 5 == 0)
{
grid[i][j] = OBSTACLE;
}
}
}
COORD pos;
pos.X = 4;
pos.Y = 10;
bool update = true;
while (true)
{
if (update)
{
system("cls");
cout << "Score: " << score << endl;
for (int i = 0; i < BOARD_SIZE; ++i)
{
for (int j = 0; j < BOARD_SIZE; ++j)
{
if (i == pos.Y && j == pos.X)
{
cout << "@";
}
else
{
cout << grid[i][j];
}
}
cout << endl;
}
update = false;
}
if (GetAsyncKeyState(0x25) && pos.X > 0 && grid[pos.Y][pos.X - 1] != OBSTACLE) // left
{
pos.X -= 1;
update = true;
}
else if (GetAsyncKeyState(0x27) && pos.X < BOARD_SIZE - 1 && grid[pos.Y][pos.X + 1] != OBSTACLE) // right
{
pos.X += 1;
update = true;
}
if (GetAsyncKeyState(0x26) && pos.Y > 0 && grid[pos.Y - 1][pos.X] != OBSTACLE) // up
{
pos.Y--;
update = true;
}
else if (GetAsyncKeyState(0x28) && pos.Y < BOARD_SIZE - 1 && grid[pos.Y + 1][pos.X] != OBSTACLE) // down
{
pos.Y++;
update = true;
}
score++;
Sleep(40);
}
return 0;
}
Adding Enemy Movement
To intensify the gameplay further, we'll introduce dynamic enemies that actively pursue the player character. These enemies, represented by the 'E' symbol, will move strategically within the grid, aiming to intercept and challenge the player. By incorporating enemy movement, we heighten the game's tension and require players to devise evasion tactics and quick decision-making skills. This addition transforms our basic console game into a dynamic and engaging experience, where players must balance offense and defense while navigating through obstacles and avoiding enemy encounters.
#include <iostream>
#include <fstream>
#include <string>
#include <Windows.h>
#include <array>
#include <vector>
#include <cstdlib>
#include <ctime>
const HANDLE hConsole = GetStdHandle(STD_OUTPUT_HANDLE);
using namespace std;
void ShowConsoleCursor(bool showFlag)
{
CONSOLE_CURSOR_INFO cursorInfo;
GetConsoleCursorInfo(hConsole, &cursorInfo);
cursorInfo.bVisible = showFlag;
SetConsoleCursorInfo(hConsole, &cursorInfo);
}
int main()
{
ShowConsoleCursor(false);
const int BOARD_SIZE = 20;
const char OBSTACLE = '#';
const char ENEMY = 'E';
char grid[BOARD_SIZE][BOARD_SIZE] = {};
int score = 0;
srand(static_cast<unsigned int>(time(0)));
for (int i = 0; i < BOARD_SIZE; ++i)
{
for (int j = 0; j < BOARD_SIZE; ++j)
{
if ((i + j) % 5 == 0)
{
grid[i][j] = OBSTACLE;
}
else if (rand() % 20 == 0) // Randomly place enemies
{
grid[i][j] = ENEMY;
}
}
}
COORD pos;
pos.X = 4;
pos.Y = 10;
bool update = true;
while (true)
{
if (update)
{
system("cls");
cout << "Score: " << score << endl;
for (int i = 0; i < BOARD_SIZE; ++i)
{
for (int j = 0; j < BOARD_SIZE; ++j)
{
if (i == pos.Y && j == pos.X)
{
cout << "@";
}
else
{
cout << grid[i][j];
}
}
cout << endl;
}
update = false;
}
if (GetAsyncKeyState(0x25) && pos.X > 0 && grid[pos.Y][pos.X - 1] != OBSTACLE) // left
{
pos.X -= 1;
update = true;
}
else if (GetAsyncKeyState(0x27) && pos.X < BOARD_SIZE - 1 && grid[pos.Y][pos.X + 1] != OBSTACLE) // right
{
pos.X += 1;
update = true;
}
if (GetAsyncKeyState(0x26) && pos.Y > 0 && grid[pos.Y - 1][pos.X] != OBSTACLE) // up
{
pos.Y--;
update = true;
}
else if (GetAsyncKeyState(0x28) && pos.Y < BOARD_SIZE - 1 && grid[pos.Y + 1][pos.X] != OBSTACLE) // down
{
pos.Y++;
update = true;
}
// Move enemies towards the player
for (int i = 0; i < BOARD_SIZE; ++i)
{
for (int j = 0; j < BOARD_SIZE; ++j)
{
if (grid[i][j] == ENEMY)
{
if (i < pos.Y && grid[i + 1][j] == 0)
{
grid[i][j] = 0;
grid[i + 1][j] = ENEMY;
}
else if (i > pos.Y && grid[i - 1][j] == 0)
{
grid[i][j] = 0;
grid[i - 1][j] = ENEMY;
}
else if (j < pos.X && grid[i][j + 1] == 0)
{
grid[i][j] = 0;
grid[i][j + 1] = ENEMY;
}
else if (j > pos.X && grid[i][j - 1] == 0)
{
grid[i][j] = 0;
grid[i][j - 1] = ENEMY;
}
}
}
}
// Check for game over
if (grid[pos.Y][pos.X] == ENEMY)
{
system("cls");
cout << "Game Over! Final Score: " << score << endl;
break;
}
score++;
Sleep(40);
}
return 0;
}
By implementing these enhancements—obstacles, a scoring system, and enemy movement—we elevate our console game from a simple exercise to an immersive and challenging gaming experience. These features not only add complexity and depth but also showcase the versatility of C++ in game development, allowing developers to create interactive and compelling gameplay scenarios within a console environment.
Conclusion
In conclusion, mastering the development of interactive console games with C++ opens doors to both enhancing programming skills and unleashing creative potential. Starting with fundamental setups and gradually integrating advanced features such as obstacle handling, scoring systems, and dynamic enemy behaviors enriches the gaming experience. This iterative process not only solidifies understanding of programming principles but also fosters innovation in game design. Embrace experimentation and enjoy the journey of transforming conceptual ideas into interactive realities through coding. Whether you're a novice or university student, the skills gained from crafting console games in C++ empower you to solve your programming assignment and create immersive and engaging gaming experiences. Happy coding and may your ventures into game development be rewarding and inspiring!