Claim Your Discount Today
Kick off the fall semester with a 20% discount on all programming assignments at www.programminghomeworkhelp.com! Our experts are here to support your coding journey with top-quality assistance. Seize this seasonal offer to enhance your programming skills and achieve academic success. Act now and save!
We Accept
- 1. Understanding the Problem Statement
- Key Tasks:
- 2. Breaking Down the Problem
- a. Define Calendar Components
- b. Create Functions
- 3. Planning the Solution
- a. Data Structures
- b. Function Design
- 4. Implementing the Solution
- a. Define the Calendar Structure
- b. Implement the Main Program Loop
- 5. Testing the Solution
- a. Basic Valid Inputs
- b. Edge Cases
- c. Invalid Inputs
- d. Gala Month Testing
- e. Boundary Cases
- Testing Procedure:
- 6. Error Handling and User Interaction
- Example of Error Handling:
- Conclusion
Creating a custom calendar system is a fascinating python programming assignments that requires a good understanding of how to handle user input, validate it, and generate formatted outputs based on specific rules. In this guide, we’ll explore how to tackle such a problem step-by-step, using the example of a fictional Plutonian calendar system. This guide will cover everything from understanding the problem to implementing and testing the solution in Python.
1. Understanding the Problem Statement
The problem involves designing a calendar system for an imaginary planet where:
- Days of the Week: There are 15 distinct days in a week, each named after a chemical element.
- Months: Each month can have between 42 and 95 days.
- Special Case – Gala Month: Certain months (Gala months) have unique rules where some weekdays are skipped during the second and third weeks.
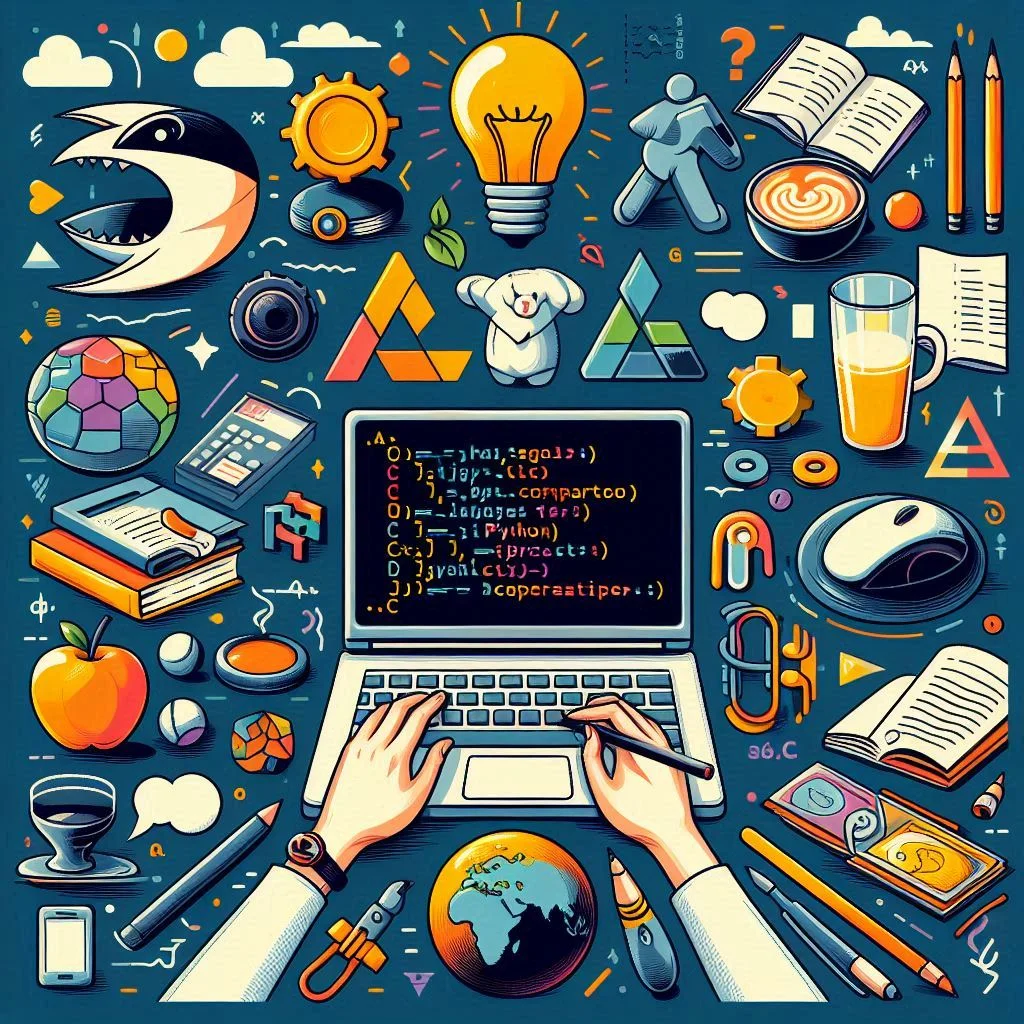
Key Tasks:
- Input Handling: Gather information from the user about the start day of the month, the number of days in the month, and whether it is a Gala month.
- Calendar Generation: Create a calendar that displays the days and skips specific days if it's a Gala month.
- Validation: Ensure that all inputs are valid and handle errors gracefully.
2. Breaking Down the Problem
To effectively solve the programming assignment, we need to break it down into smaller, manageable parts.
a. Define Calendar Components
- Days of the Week: Represent these days in a list for easy indexing.
- Month Length: Manage days from 42 to 95.
- Gala Month Rules: Skip specific days during certain weeks.
b. Create Functions
- Input Validation Functions: Ensure inputs are valid before proceeding.
- Calendar Display Functions: Format and display the calendar correctly based on input.
- Main Function: Manage user interactions and call other functions as needed.
3. Planning the Solution
a. Data Structures
- Days of the Week List: Use a list or dictionary to map days to their indices. This helps in calculating which day corresponds to which index in a month.
- Month Length: Use integer values to represent the number of days in the month. This will affect how the calendar is displayed.
- Gala Month Rules: Implement a mechanism to skip specific days in the second and third weeks.
b. Function Design
1. Validation Functions:
- Check Day Validity: Ensure the starting day is one of the predefined days.
- Check Day Count Validity: Validate that the number of days falls within the allowed range (42 to 95).
- Check Gala Month Input: Confirm that the input is either "yes" or "no".
2. Calendar Display Function:
- Format Output: Print days in a grid-like format, taking care of special rules if it's a Gala month.
- Handle Gala Month: Skip specified days during the second and third weeks.
3. Main Function:
- User Interaction: Collect and validate user inputs.
- Loop for Multiple Calendars: Allow users to generate multiple calendars until they choose to stop.
4. Implementing the Solution
Let’s dive into a detailed Python implementation.
a. Define the Calendar Structure
First, define the days of the week and create functions for validation and calendar generation.
def get_day_index(day_name):
days = ["Hydrogenday", "Heliumday", "Lithiumday", "Berylliumday", "Boronday",
"Carbonday", "Nitrogenday", "Oxygenday", "Fluorineday", "Neonday",
"Sodiumday", "Magnesiumday", "Aluminumday", "Siliconday", "Phosphorusday"]
if day_name not in days:
raise ValueError("Invalid day name")
return days.index(day_name)
def is_valid_day_count(count):
return 42 <= count <= 95
def is_valid_gala(gala):
return gala.lower() in {"yes", "no"}
def display_calendar(start_day, num_days, gala):
days = ["Hydrogenday", "Heliumday", "Lithiumday", "Berylliumday", "Boronday",
"Carbonday", "Nitrogenday", "Oxygenday", "Fluorineday", "Neonday",
"Sodiumday", "Magnesiumday", "Aluminumday", "Siliconday", "Phosphorusday"]
start_index = get_day_index(start_day)
week_days = len(days)
# Print header
print(' '.join(days))
# Print days
day_number = 1
for i in range(num_days):
if i % week_days == 0 and i > 0:
print() # New line for each week
if gala and (i // week_days + 1) in {2, 3}: # Skip days in Gala months
if days[i % week_days] in {"Heliumday", "Berylliumday", "Carbonday",
"Oxygenday", "Neonday", "Magnesiumday",
"Siliconday"}:
continue
print(f"{day_number:2}", end=' ')
day_number += 1
print()
b. Implement the Main Program Loop
Create the main function to handle user inputs, validate them, and display the calendar.
def main():
while True:
start_day = input("Enter the starting day of the month: ").strip()
try:
start_index = get_day_index(start_day)
except ValueError:
print("Invalid starting day, try again.")
continue
try:
num_days = int(input("Enter the number of days in the month: "))
if not is_valid_day_count(num_days):
print("Invalid number of days, should be between 42 and 95, try again.")
continue
except ValueError:
print("Invalid number of days, should be a whole number, try again.")
continue
gala = input("Is this a Gala month? (yes/no): ").strip().lower()
if not is_valid_gala(gala):
print("Invalid response, should be yes or no, try again.")
continue
display_calendar(start_day, num_days, gala == "yes")
another = input("Create another calendar? (yes/no): ").strip().lower()
if another == "no":
print("Finishing Plutonian Calendar Program.")
break
if __name__ == "__main__":
main()
5. Testing the Solution
Testing is a crucial step to ensure the functionality and reliability of your program. Here’s how you can test the custom calendar system:
a. Basic Valid Inputs
- Start Day: "Boronday"
- Number of Days: 63
- Gala Month: "no"
Test the program with these inputs to verify that it generates a correct calendar.
b. Edge Cases
- Start Day: "Hydrogenday"
- Number of Days: 42 (minimum)
- Gala Month: "yes"
Ensure that the program handles the minimum number of days and correctly processes a Gala month.
c. Invalid Inputs
- Invalid Day Name: "Weirdday"
- Invalid Number of Days: 101
- Invalid Gala Month Input: "maybe"
Test how the program responds to invalid inputs and ensures that it prompts the user for correct data.
d. Gala Month Testing
- Start Day: "Carbonday"
- Number of Days: 72
- Gala Month: "yes"
Verify that the program correctly skips specific days during the Gala month’s second and third weeks.
e. Boundary Cases
- Start Day: "Phosphorusday"
- Number of Days: 95 (maximum)
- Gala Month: "no"
Test the maximum number of days to ensure the program handles the upper limits correctly.
Testing Procedure:
- Run the program with various inputs to ensure all cases are handled correctly.
- Check outputs to verify that the calendar is displayed as expected, including correct handling of Gala months.
- Verify error messages to ensure they guide users to correct input.
6. Error Handling and User Interaction
Proper error handling improves the user experience. Ensure your program:
- Validates Inputs: Provide meaningful error messages when inputs are incorrect.
- Guides Users: Prompt users for re-entry if their inputs are invalid.
- Ends Gracefully: Confirm when the user decides to finish, and provide a friendly exit message.
Example of Error Handling:
def get_day_index(day_name):
days = ["Hydrogenday", "Heliumday", "Lithiumday", "Berylliumday", "Boronday",
"Carbonday", "Nitrogenday", "Oxygenday", "Fluorineday", "Neonday",
"Sodiumday", "Magnesiumday", "Aluminumday", "Siliconday", "Phosphorusday"]
if day_name not in days:
raise ValueError("Invalid day name. Please enter one of the following: " + ", ".join(days))
return days.index(day_name)
Conclusion
Creating a custom calendar system is a challenging yet rewarding task that enhances your programming skills and problem-solving abilities. By understanding the problem, breaking it down into manageable components, planning a solution, and implementing it carefully, you can build a robust and functional calendar system.
In this guide, we’ve covered everything from understanding the problem statement to implementing the solution in Python and testing it thoroughly. By following these steps, you’ll be well-equipped to solve programming assignment and create effective, custom solutions.
Feel free to explore and modify the code to suit different calendar systems or additional requirements.