Claim Your Discount Today
Ring in Christmas and New Year with a special treat from www.programminghomeworkhelp.com! Get 15% off on all programming assignments when you use the code PHHCNY15 for expert assistance. Don’t miss this festive offer—available for a limited time. Start your New Year with academic success and savings. Act now and save!
We Accept
- 1. Understanding Business Requirements
- Business Requirements Overview:
- 2. Analyzing Existing Files
- 1. HTML/CSS Files
- 2. JavaScript Files
- 3. Server-Side Code
- 4. Database Schema
- 3. Designing the Contact Us Page
- 1. Form Structure
- 2. Client-Side Form Validation
- 4. Handling Form Submission on the Server
- 1. Server-Side Script Example (PHP)
- 2. Generating Unique Entry IDs
- 5. Creating the View Entries Page
- 1. Example (PHP)
- 6. Testing Thoroughly
- 1. Functional Testing
- 2. Edge Cases
- 3. User Experience
- Conclusion
The Contact Us page is a critical component of any website, providing users with a direct channel to communicate with the business or organization. Whether it's for inquiries, support, feedback, or any other purpose, a well-designed Contact Us page can enhance user engagement and improve customer satisfaction.
This Web development assignment aims to walk you through the process of developing a Contact Us page from start to finish. It covers understanding business requirements, analyzing existing code, designing the user interface, implementing server-side logic, and ensuring robust validation and data handling. By following this comprehensive approach, you'll be able to create a functional, user-friendly, and secure Contact Us page that meets the needs of both the business and its users. By breaking down the process into manageable steps, you will gain a clearer understanding of how to approach similar assignments in your programming journey.
1. Understanding Business Requirements
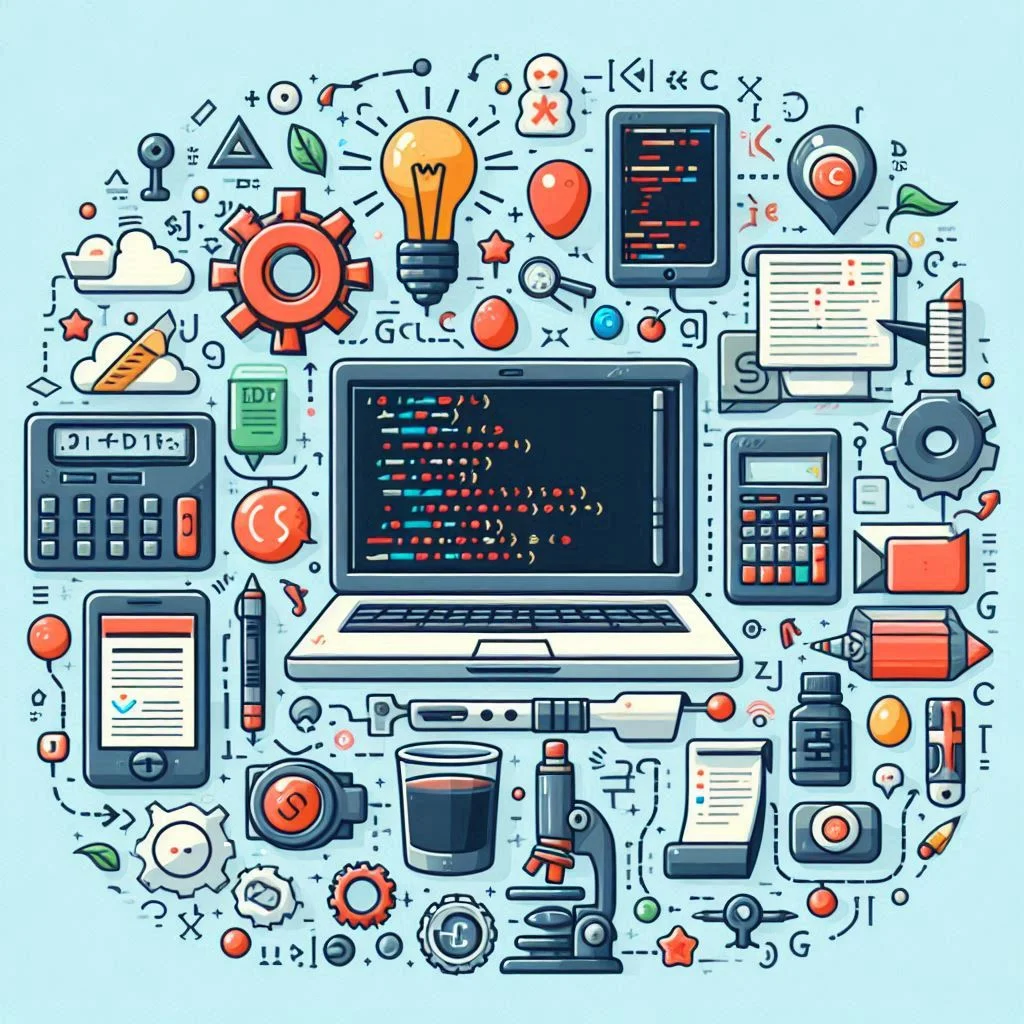
Before diving into the technical aspects, it's crucial to understand the business requirements clearly. In our example scenario, the company Widgets R-US wants to modernize its contact process by capturing user feedback in a database rather than through email The first step in any programming task is to thoroughly understand the business requirements. Let’s break down the requirements provided for the Contact Us page:
Business Requirements Overview:
1. Create a Contact Us Page: This page should include specific fields for user input.
2. Field Specifications:
- Reason for Contact: Dropdown list with sorted options.
- Email Address: Must be validated to contain a valid email format.
- First Name and Last Name: Both are required fields.
- Phone Number: Optional field, if applicable.
- Message: Required field with a maximum length of 200 characters.
3. Submission Handling:
- Confirmation: Users should receive a confirmation message and a unique entry ID.
- Entry ID: Should be a 6-digit number starting with 111111.
- View Entries Page: A separate page to display all submitted entries.
Understanding these requirements is essential as they form the blueprint of your implementation strategy.
2. Analyzing Existing Files
Once you have a grasp of the requirements, the next step is to review the files you’ve inherited. This might include HTML, CSS, JavaScript, server-side code, and database schemas. Here’s how you can analyze these files:
1. HTML/CSS Files
- HTML: Look for the structure of the Contact Us form. Check if it already contains placeholders or form elements that you need to build upon or modify.
- CSS: Review the styles applied to the form elements. Ensure they meet the design specifications required.
2. JavaScript Files
- Client-Side Validation: Check if there are any existing scripts that validate form inputs. Make sure they align with the validation rules specified in the requirements.
- Event Handling: Review how form submissions are handled. Ensure that data is being collected and processed correctly.
3. Server-Side Code
- Languages: Depending on the language (PHP, Python, Ruby, Node.js, etc.), review how data is processed, validated, and stored.
- Data Storage: Check how data is being inserted into the database and if the entry ID generation logic is already in place.
4. Database Schema
- Tables: Review the structure of the database tables to ensure they have fields for all the data you need to capture (e.g., email, name, message).
- Schema Design: Ensure that the schema supports the data relationships and integrity constraints required by the business logic.
3. Designing the Contact Us Page
With the analysis complete, you can now design the Contact Us page. This involves creating a user-friendly interface with the required fields.
1. Form Structure
Here’s an example of how you might structure the HTML for the Contact Us form:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Contact Us</title>
<style>
body {
font-family: Arial, sans-serif;
margin: 20px;
padding: 0;
}
form {
max-width: 600px;
margin: auto;
}
label {
display: block;
margin: 10px 0 5px;
}
input, select, textarea {
width: 100%;
padding: 10px;
margin-bottom: 10px;
box-sizing: border-box;
}
button {
padding: 10px 20px;
background-color: #007BFF;
color: white;
border: none;
cursor: pointer;
}
button:hover {
background-color: #0056b3;
}
</style>
</head>
<body>
<h1>Contact Us</h1>
<form id="contactForm" method="post" action="submit.php">
<label for="reason">Reason for Contact:</label>
<select id="reason" name="reason" required>
<option value="General Inquiry">General Inquiry</option>
<option value="Support">Support</option>
<option value="Feedback">Feedback</option>
</select>
<label for="email">E-Mail Address:</label>
<input type="email" id="email" name="email" required>
<label for="firstName">First Name:</label>
<input type="text" id="firstName" name="firstName" required>
<label for="lastName">Last Name:</label>
<input type="text" id="lastName" name="lastName" required>
<label for="phone">Phone Number:</label>
<input type="text" id="phone" name="phone">
<label for="message">Message:</label>
<textarea id="message" name="message" required maxlength="200"></textarea>
<button type="submit">Submit</button>
</form>
</body>
</html>
Explanation:
- HTML Structure: The form includes labels, input fields, a drop-down list, and a submit button.
- Form Attributes: The method="post" attribute ensures that form data is sent via HTTP POST, and the action="submit.php" attribute specifies the server-side script that will handle the form submission.
- CSS Styling: Basic styling is applied to improve the visual appeal and user experience.
2. Client-Side Form Validation
JavaScript can be used to add client-side validation to enhance user experience:
document.getElementById('contactForm').addEventListener('submit', function(event) {
const email = document.getElementById('email').value;
const emailPattern = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;
if (!emailPattern.test(email)) {
alert('Please enter a valid email address.');
event.preventDefault();
}
});
Explanation:
Validation Logic: The JavaScript code attaches an event listener to the form's submit event. It checks if the entered email matches the required pattern. If not, it prevents the form from submitting and alerts the user.
4. Handling Form Submission on the Server
On the server-side, you need to handle form submissions, validate data, generate a unique entry ID, and store the data in the database.
1. Server-Side Script Example (PHP)
Here’s a simple example using PHP for handling form submissions:
<?php
if ($_SERVER['REQUEST_METHOD'] === 'POST') {
// Database connection
$pdo = new PDO('mysql:host=localhost;dbname=widgets', 'user', 'password');
// Generate unique entry ID
$entryId = '111111' . str_pad(mt_rand(0, 999999), 6, '0', STR_PAD_LEFT);
// Prepare data for insertion
$reason = $_POST['reason'];
$email = $_POST['email'];
$firstName = $_POST['firstName'];
$lastName = $_POST['lastName'];
$phone = $_POST['phone'];
$message = $_POST['message'];
// Validate data (basic example)
if (!filter_var($email, FILTER_VALIDATE_EMAIL)) {
echo "Invalid email format";
exit;
}
// Insert data into database
$stmt = $pdo->prepare('INSERT INTO contacts (entry_id, reason, email, first_name, last_name, phone, message) VALUES (?, ?, ?, ?, ?, ?, ?)');
$stmt->execute([$entryId, $reason, $email, $firstName, $lastName, $phone, $message]);
// Show confirmation message
echo "Thank you! Your request has been received. Your entry ID is $entryId. <a href='view_entries.php?id=$entryId'>View your entries</a>";
}
?>
2. Generating Unique Entry IDs
To generate a unique entry ID, combine a fixed prefix with a random 6-digit number. This ensures IDs are unique and traceable.
5. Creating the View Entries Page
The view entries page allows users to see their submitted entries. It should display data in a readable format.
1. Example (PHP)
Here’s a basic PHP script to display the user’s entries:
<?php
if (isset($_GET['id'])) {
$entryId = $_GET['id'];
// Database connection
$pdo = new PDO('mysql:host=localhost;dbname=widgets', 'user', 'password');
// Fetch data from the database
$stmt = $pdo->prepare('SELECT * FROM contacts WHERE entry_id = ?');
$stmt->execute([$entryId]);
$entry = $stmt->fetch();
if ($entry) {
echo "<h1>Your Entry Details</h1>";
echo "Entry ID: " . htmlspecialchars($entry['entry_id']) . "<br>";
echo "Reason: " . htmlspecialchars($entry['reason']) . "<br>";
echo "Email: " . htmlspecialchars($entry['email']) . "<br>";
echo "First Name: " . htmlspecialchars($entry['first_name']) . "<br>";
echo "Last Name: " . htmlspecialchars($entry['last_name']) . "<br>";
echo "Phone: " . htmlspecialchars($entry['phone']) . "<br>";
echo "Message: " . htmlspecialchars($entry['message']);
} else {
echo "No entry found with ID $entryId.";
}
} else {
echo "No ID specified.";
}
?>
6. Testing Thoroughly
Testing is crucial to ensure everything works as expected. Here’s how to approach testing:
1. Functional Testing
- Form Submission: Test with valid and invalid inputs to ensure proper validation.
- Database Insertion: Verify that data is correctly stored in the database.
- Confirmation Message: Ensure users receive the correct confirmation message and entry ID.
- View Entries Page: Check if the correct data is displayed on the view entries page.
2. Edge Cases
- Test with boundary values (e.g., message length exactly 200 characters).
- Check how the system handles invalid email formats or incomplete forms.
3. User Experience
- Ensure the form is user-friendly and visually appealing.
- Check for responsiveness on different devices and screen sizes.
Conclusion
Completing programming assignments, especially those involving inherited code or partially completed projects, requires a systematic approach. By understanding business requirements, analyzing existing files, designing and implementing features, handling form submissions, and creating supportive pages, you can successfully complete your assignment.
Remember to test thoroughly and document your work to ensure quality and maintainability. By following these guidelines, you’ll be well-equipped to tackle similar assignments and excel in your programming endeavors.