Claim Your Discount Today
Take your coding game to new heights with expert help at unbeatable prices. Got a tricky project or a tight deadline? We’ve got you covered! Use code PHHBF10 at checkout and save BIG. Don’t wait—this exclusive Black Friday deal won’t last long. Secure your academic success today!
We Accept
- Introduction to Data Structures and Queues
- Problem Breakdown
- Part 1: Implementing the Queue
- Understanding the Queue Structure
- Defining the Structures
- Implementing Queue Functions
- Part 2: Solving the Josephus Problem
- Understanding the Josephus Problem
- Implementing the Josephus Function
- Testing the Implementation
- Example of running a Josephus test:
- Conclusion and Reflections
- Key Takeaways:
- Additional Considerations
Understanding the core principles of data structures is essential for developing efficient, high-performing software. Among these, queues play a pivotal role in managing data flow in a variety of applications, from operating systems to complex algorithms. The ability to effectively implement and manipulate queues not only enhances your problem-solving toolkit but also deepens your comprehension of how data is organized and processed. In this discussion, we’ll explore the intricacies of queue implementation and apply these concepts to solve data structure assignments, illustrating how mastering such fundamental structures can lead to powerful and elegant solutions in programming.
Introduction to Data Structures and Queues
Data structures are fundamental concepts in computer science that allow efficient data organization and management. Among these, queues are a basic and widely-used structure. A queue is a linear data structure that follows the First-In-First-Out (FIFO) principle. This means that the first element added to the queue will be the first one to be removed.
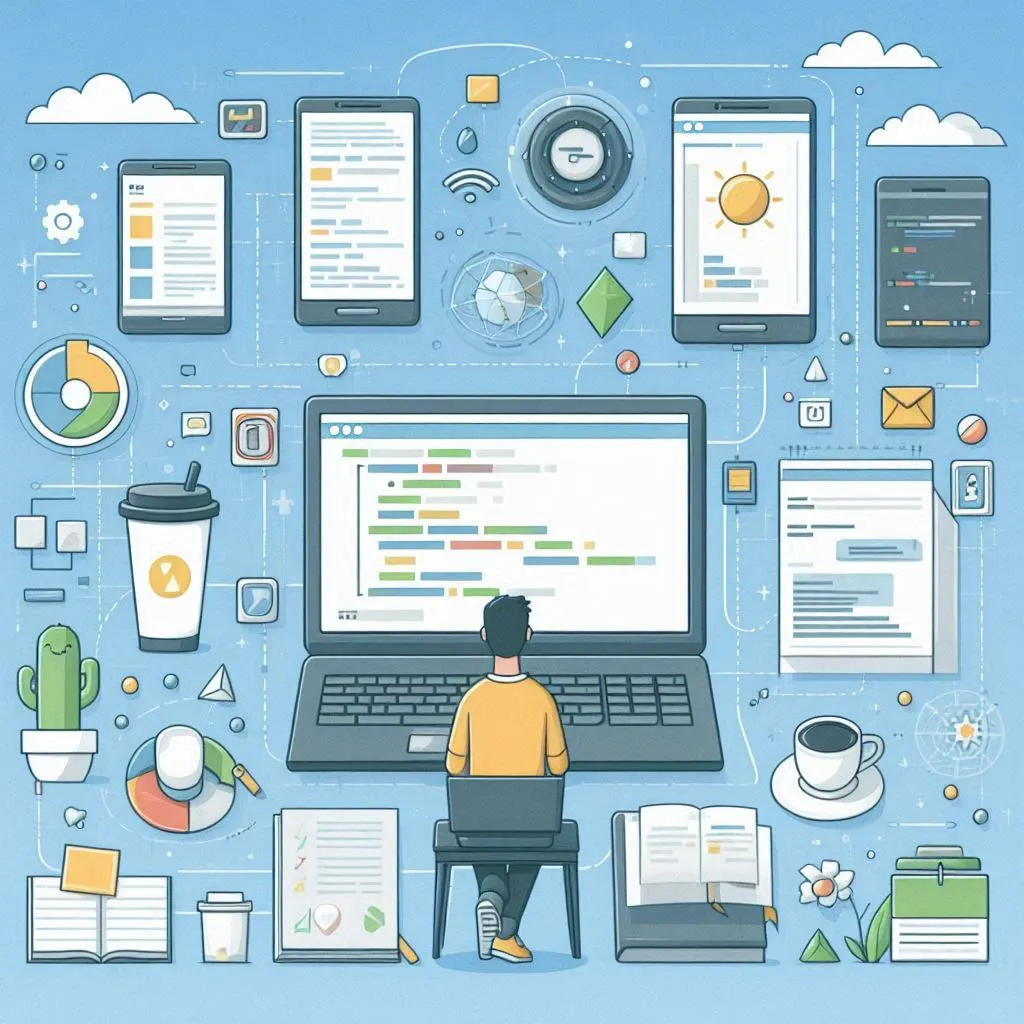
Queues are often used in scenarios like managing tasks in a CPU, handling requests in a server, or simulating real-world queues, such as lines at a checkout counter.
Problem Breakdown
This assignment requires you to:
- Implement a Queue: Develop a queue using a linked list.
- Solve the Josephus Problem: Use the queue to solve this classic algorithmic problem.
You are provided with:
- main.c: A test file that you must not modify.
- queue.h: A header file containing function prototypes and data structure definitions.
- queue.c: The file you will create and implement.
Part 1: Implementing the Queue
Understanding the Queue Structure
A queue is a linear data structure where elements are added to the rear and removed from the front. The operations you typically need to implement for a queue include:
- newQueue: Creates a new queue.
- enqueue: Adds an element to the rear.
- dequeue: Removes an element from the front.
- front: Returns the front element without removing it.
- isEmpty: Checks if the queue is empty.
In a linked list-based implementation, each element (node) in the queue contains data and a pointer to the next node. The queue itself keeps track of the front and rear nodes.
Defining the Structures
Start by defining the necessary structures in queue.c:
#include "queue.h"
#include <stdlib.h>
// Node structure
typedef struct Node {
int data;
struct Node* next;
} Node;
// Queue structure
typedef struct Queue {
Node* front;
Node* rear;
} Queue;
- Node Structure: Represents each element in the queue.
- Queue Structure: Maintains pointers to the front and rear of the queue.
Implementing Queue Functions
1. Creating a New Queue:
Queue* newQueue() {
Queue* q = (Queue*)malloc(sizeof(Queue));
q->front = q->rear = NULL;
return q;
}
Explanation: This function initializes a new queue with both front and rear set to NULL, indicating that the queue is empty.
2. Enqueue Operation:
void enqueue(Queue* q, int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = NULL;
if (q->rear == NULL) {
q->front = q->rear = newNode;
return;
}
q->rear->next = newNode;
q->rear = newNode;
}
Explanation: The enqueue function adds a new node to the rear of the queue. If the queue is empty (rear == NULL), both front and rear point to the new node. Otherwise, the new node is added to the end, and the rear pointer is updated.
3. Dequeue Operation:
int dequeue(Queue* q) {
if (q->front == NULL) {
return -1; // Queue is empty
}
Node* temp = q->front;
int value = temp->data;
q->front = q->front->next;
if (q->front == NULL) {
q->rear = NULL;
}
free(temp);
return value;
}
Explanation: The dequeue function removes the node at the front of the queue and returns its value. If the queue becomes empty after the operation, both front and rear are set to NULL.
4. Front Operation:
int front(Queue* q) {
if (q->front == NULL) {
return -1; // Queue is empty
}
return q->front->data;
}
Explanation: The front function returns the value of the front node without removing it from the queue.
5. IsEmpty Operation:
int isEmpty(Queue* q) {
return (q->front == NULL);
}
Explanation: This function checks if the queue is empty by verifying if the front pointer is NULL.
Part 2: Solving the Josephus Problem
Understanding the Josephus Problem
The Josephus problem is a theoretical problem related to a certain elimination game. People stand in a circle and every Mth person is eliminated until only one person remains. The task is to determine the order of elimination and identify the last person standing.
For example, if N = 7 and M = 3, people would be eliminated in the following order: 2, 5, 1, 6, 4, 0, leaving person 3 as the survivor.
Implementing the Josephus Function
The Josephus problem can be solved using a queue, where each person is enqueued, and the Mth person is dequeued until only one remains.
void josephus(int n, int m) {
Queue* q = newQueue();
// Enqueue all people (0 to N-1)
for (int i = 0; i < n; i++) {
enqueue(q, i);
}
printf("Order Eliminated:\n");
while (!isEmpty(q)) {
for (int i = 0; i < m - 1; i++) {
enqueue(q, dequeue(q)); // Rotate the queue by M-1
}
printf("%d ", dequeue(q)); // Eliminate the Mth person
}
printf("\n");
}
Explanation:
- First, all people (from 0 to N-1) are enqueued.
- In each iteration, the queue is rotated by dequeuing and re-enqueueing M-1 people.
- The Mth person is then dequeued and eliminated.
- This continues until only one person remains, who is the last one to be eliminated.
Testing the Implementation
To verify the correctness of your implementation, run the provided main.c test cases. The main.c file will test all functions, including edge cases such as dequeuing from an empty queue.
Example of running a Josephus test:
Bash code
./main
Select Option from list.
- 0.) Run All Tests
- 1.) Test New Queue
- 2.) Test Enqueue
- 3.) Test Front
- 4.) Test isEmpty
- 5.) Test Dequeue
- 6.) Run Randomized Tests
- 7.) Josephus Puzzle
Enter Number of test to run: 7
Enter Number of People (N): 7
Enter Person to Eliminate (M): 3
Order Eliminated: 2 5 1 6 4 0 3
Conclusion and Reflections
The assignment required the implementation of a queue using a linked list and solving the Josephus problem using this queue. Through this process, you've gained hands-on experience with basic data structures, memory management in C, and applying these concepts to complete your programming assignment.
Key Takeaways:
- Understanding Linked Lists: Implementing a queue using linked lists helps solidify your understanding of dynamic data structures.
- Algorithmic Thinking: The Josephus problem illustrates how data structures can be applied to solve complex problems.
- Memory Management: Proper handling of dynamic memory allocation and deallocation in C is crucial to avoid memory leaks and segmentation faults.
This systematic approach can be applied to various data structure and algorithm problems, providing a solid foundation for more advanced topics in computer science.
Additional Considerations
If you want to extend your understanding further:
- Time Complexity: Analyze the time complexity of each operation. For example, enqueue and dequeue operations in a linked list-based queue have O(1) complexity.
- Optimizations: Consider ways to optimize your implementation, perhaps by minimizing the number of operations in the Josephus function.
- Memory Efficiency: Explore how different data structure implementations (arrays vs. linked lists) affect memory usage.
By continuing to practice and exploring these concepts in-depth through C programming assignments, you'll build a strong foundation in data structures and algorithms, preparing you for more challenging programming problems in the future.