Claim Your Offer
Unlock an amazing offer at www.programminghomeworkhelp.com with our latest promotion. Get an incredible 10% off on your all programming assignment, ensuring top-quality assistance at an affordable price. Our team of expert programmers is here to help you, making your academic journey smoother and more cost-effective. Don't miss this chance to improve your skills and save on your studies. Take advantage of our offer now and secure exceptional help for your programming assignments.
We Accept
- Introduction to Shell Programming
- Core Responsibilities of a Shell
- Core Concepts
- 1. Process Management
- 2. Job Control
- 3. File Redirection
- Detailed Steps to Implement a Shell Program
- 1. Setting Up the Environment
- 2. Designing the Shell Program
- 3. Executing Commands
- 4. Job Management
- Implementing Built-in Commands
- Help Command
- Quit Command
- Job Control Commands
- File Redirection
- Signal Handling
- Race Conditions
- Testing and Debugging
- Conclusion
Programming a shell from scratch is an enriching experience that hones your understanding of operating systems, process management, and job control. Building a shell program is a foundational exercise that helps in understanding process management, job control, and Unix-like system calls. This guide is tailored for students working on assignments similar to creating a simple shell program, such as the MASH (MAson SHell) project. We’ll dive into key concepts, practical tips, and strategies to successfully operating system assignment.
Introduction to Shell Programming
A shell is a command-line interface that allows users to interact with the operating system by typing commands. It processes user commands, executes programs, and manages various system tasks. There are two main types of shells:
- Interactive Shells: Used directly by users, providing command prompts and executing commands.
- Non-Interactive Shells: Often used in scripts to execute a series of commands automatically.
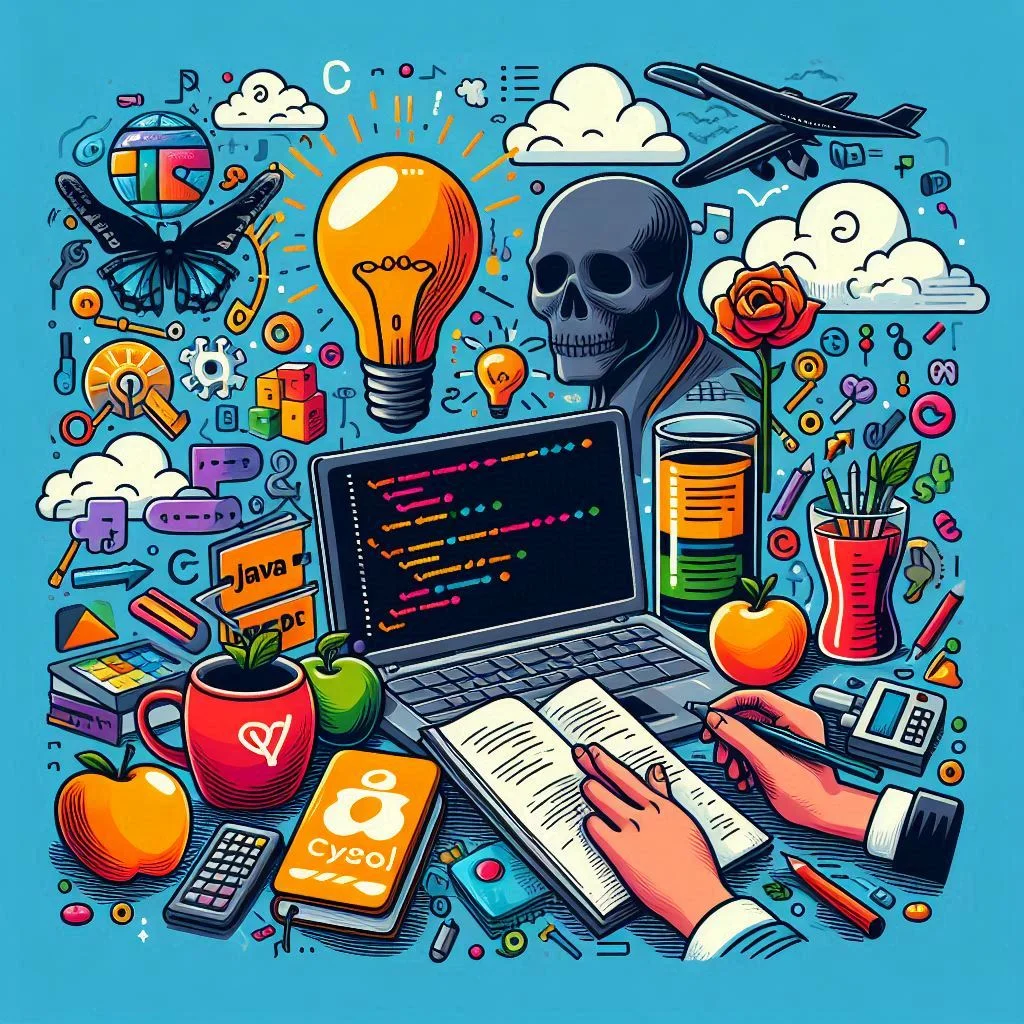
In this guide, we'll focus on creating a simple interactive shell that can handle commands, manage jobs, and perform basic file redirection.
Core Responsibilities of a Shell
- Command Parsing: The shell must read user input, parse it into commands and arguments, and understand any special characters like redirection operators.
- Command Execution: It should be able to execute built-in commands directly or start external programs.
- Job Management: The shell needs to handle foreground and background jobs, manage job statuses, and support job control commands.
- File Redirection: It should support input and output redirection to and from files.
- Signal Handling: The shell must handle signals, especially those related to job control (e.g., SIGINT for interruption).
Core Concepts
1. Process Management
In Unix-like operating systems, a process is an instance of a running program. The shell needs to manage processes effectively to execute commands and handle job control.
Forking and Executing Processes
- fork(): Creates a new process by duplicating the current process. The new process is called the child process, and the original is the parent.
pid_t pid = fork();
if (pid < 0) {
// Error handling
} else if (pid == 0) {
// Child process code
} else {
// Parent process code
}
- exec() Family: Replaces the current process image with a new process image. Functions like execl(), execv(), execle(), and execvp() are used to run new programs.
execl("/bin/ls", "ls", "-l", (char *) NULL);
2. Job Control
Job control refers to managing multiple processes or tasks. Key concepts include foreground and background jobs:
- Foreground Job: The shell waits for the process to complete before accepting new commands.
- Background Job: The shell does not wait for the process to complete and immediately returns to the prompt.
Background and Foreground Job Management
- Running a Job in the Background: Append an & to the command.
execl("/bin/sleep", "sleep", "10", (char *) NULL);
- Handling Signals: Signals like SIGINT (interrupt) and SIGTSTP (suspend) are used to manage job control.
signal(SIGINT, handle_sigint);
signal(SIGTSTP, handle_sigstp);
3. File Redirection
File redirection involves changing the standard input/output of a process to or from files.
- Redirecting Output: Use > to redirect output to a file.
freopen("output.txt", "w", stdout);
- Redirecting Input: Use < to take input from a file.
freopen("input.txt", "r", stdin);
- Appending to Files: Use >> to append output to a file.
freopen("output.txt", "a", stdout);
Detailed Steps to Implement a Shell Program
1. Setting Up the Environment
Before diving into implementation, ensure you have a development environment set up with the following tools:
- Compiler: Typically, gcc for C programs.
- Editor/IDE: Any text editor or Integrated Development Environment (IDE) of your choice.
- Unix-like System: A system like Linux or macOS, or a virtual machine running a Unix-like OS.
2. Designing the Shell Program
Parsing User Input
- Prompt Display: The shell should display a prompt to indicate readiness for user input. For example, MASH>.
- Reading Input: Use functions like fgets() to read a line of input from the user. Remember to strip the newline character at the end.
char buffer[1024];
printf("MASH> ");
if (fgets(buffer, sizeof(buffer), stdin) == NULL) {
perror("fgets");
exit(EXIT_FAILURE);
}
buffer[strcspn(buffer, "\n")] = '\0'; // Remove newline
3. Tokenizing Input: Use strtok() to split the input line into tokens based on spaces. This will help in extracting the command and its arguments.
char *token = strtok(buffer, " ");
4. Parsing Command and Arguments: Create an array to store arguments and a structure to manage additional options like file redirection.
char *argv[64];
int argc = 0;
while (token != NULL) {
argv[argc++] = token;
token = strtok(NULL, " ");
}
argv[argc] = NULL;
Define a structure for additional command options:
typedef struct cmd_aux {
char *in_file;
char *out_file;
int is_append;
int is_bg;
} Cmd_aux;
5. Handling Special Characters: Parse for special characters like & for background execution or > and < for redirection. Update the Cmd_aux structure accordingly.
Cmd_aux aux = {NULL, NULL, 0, 0};
for (int i = 0; i < argc; i++) {
if (strcmp(argv[i], "&") == 0) {
aux.is_bg = 1;
argv[i] = NULL;
} else if (strcmp(argv[i], ">") == 0) {
aux.out_file = argv[++i];
aux.is_append = 0;
} else if (strcmp(argv[i], ">>") == 0) {
aux.out_file = argv[++i];
aux.is_append = 1;
} else if (strcmp(argv[i], "<") == 0) {
aux.in_file = argv[++i];
}
}
3. Executing Commands
1. Built-in Commands: Implement built-in commands like help, quit, jobs, fg, bg, and kill. These commands should be handled directly by the shell without forking a new process.
if (strcmp(argv[0], "help") == 0) {
// Print help information
} else if (strcmp(argv[0], "quit") == 0) {
exit(EXIT_SUCCESS);
}
2. External Commands: For non-built-in commands, fork a new process using fork(). In the child process, use execv() or execl() to execute the program. The parent process should wait for the child to complete if it is a foreground job.
pid_t pid = fork();
if (pid == 0) {
// Child process
if (execv(argv[0], argv) == -1) {
perror("execv");
exit(EXIT_FAILURE);
}
} else {
// Parent process
if (!aux.is_bg) {
waitpid(pid, NULL, 0);
}
}
3. Path Resolution: If the command is not in the current directory, check /usr/bin/ as an alternative path.
char path[1024];
snprintf(path, sizeof(path), "./%s", argv[0]);
if (access(path, X_OK) != 0) {
snprintf(path, sizeof(path), "/usr/bin/%s", argv[0]);
}
4. Job Management
1. Foreground and Background Jobs: Maintain a list of jobs with their statuses. Use waitpid() to monitor and update job statuses.
typedef struct job {
int job_id;
pid_t pid;
char cmd[1024];
int is_running;
} Job;
2. Job Control Commands: Implement job control commands like jobs, fg, and bg. Update job status based on user commands.
if (strcmp(argv[0], "jobs") == 0) {
// List all background jobs
} else if (strcmp(argv[0], "fg") == 0) {
// Bring job to foreground
} else if (strcmp(argv[0], "bg") == 0) {
// Resume job in background
}
Implementing Built-in Commands
Built-in commands are executed directly by the shell without creating new processes. Implement common built-in commands such as help, quit, and job control commands (jobs, fg, bg, kill).
Help Command
Provide information about the shell and available commands:
void help() {
printf("MASH Shell Commands:\n");
printf("help - Display this help message\n");
printf("quit - Exit the shell\n");
// Additional commands
}
Quit Command
Terminate the shell:
void quit() {
exit(0);
}
Job Control Commands
- jobs: List background jobs.
void list_jobs() {
for (int i = 0; i < num_jobs; i++) {
printf("Job ID: %d, PID: %d, Command: %s, Status: %s\n", jobs[i].job_id, jobs[i].pid, jobs[i].cmd, jobs[i].is_running ? "Running" : "Stopped");
}
}
- fg: Bring a background job to the foreground.
void bring_fg(int job_id) {
// Find job with job_id and bring it to foreground
}
- bg: Resume a stopped background job.
void resume_bg(int job_id) {
// Find job with job_id and resume it
}
- kill: Send a signal to a job.
void kill_job(int job_id, int signal) {
// Find job with job_id and send the specified signal
File Redirection
1. Opening Files: Use open() to handle file redirection for input and output.
int fd;
if (aux.in_file) {
fd = open(aux.in_file, O_RDONLY);
dup2(fd, STDIN_FILENO);
close(fd);
}
if (aux.out_file) {
int flags = O_WRONLY | O_CREAT | (aux.is_append ? O_APPEND : O_TRUNC);
fd = open(aux.out_file, flags, 0600);
dup2(fd, STDOUT_FILENO);
close(fd);
}
2. Restoring Standard Streams: After executing the command, restore the standard input/output if necessary.
dup2(saved_stdin, STDIN_FILENO);
dup2(saved_stdout, STDOUT_FILENO);
3. Redirecting Output
int fd = open("output.txt", O_WRONLY | O_CREAT | O_TRUNC, 0600);
dup2(fd, STDOUT_FILENO);
close(fd);
4. Redirecting Input
int fd = open("input.txt", O_RDONLY);
dup2(fd, STDIN_FILENO);
close(fd);
5. Appending Output
int fd = open("output.txt", O_WRONLY | O_CREAT | O_APPEND, 0600);
dup2(fd, STDOUT_FILENO);
close(fd);
Signal Handling
1. Handling Signals: Set up signal handlers using sigaction() to manage signals like SIGINT and SIGTSTP.
struct sigaction sa;
sa.sa_handler = &sigint_handler;
sigemptyset(&sa.sa_mask);
sa.sa_flags = 0;
sigaction(SIGINT, &sa, NULL);
2. Signal Handlers: Define handlers to manage foreground job signals.
void sigint_handler(int signum) {
// Handle SIGINT
}
Race Conditions
1. Avoiding Race Conditions: Block signals during critical sections to prevent race conditions when updating job lists.
sigset_t new_set, old_set;
sigemptyset(&new_set);
sigaddset(&new_set, SIGCHLD);
sigprocmask(SIG_BLOCK, &new_set, &old_set);
// Critical section
sigprocmask(SIG_SETMASK, &old_set, NULL);
Testing and Debugging
- Unit Testing: Test each component of your shell program separately to ensure correctness.
- Integration Testing: Test the entire shell program with various commands and scenarios.
- Debugging: Use debugging tools like gdb and logging functions to troubleshoot issues.
Conclusion
Building a shell from scratch is a profound learning experience that deepens your understanding of Unix-like operating systems. It challenges you to engage with fundamental concepts such as process management, job control, and system calls, providing practical insights into how operating systems handle user interactions and task scheduling.
This project not only solidifies your grasp of these core concepts but also develops your problem-solving skills and attention to detail to solve your operating system assignment. By building and refining your shell, you will gain a valuable perspective on the inner workings of operating systems and prepare yourself for more advanced topics in system programming and software development.