Claim Your Offer
New semester, new challenges—but don’t stress, we’ve got your back! Get expert programming assignment help and breeze through Python, Java, C++, and more with ease. For a limited time, enjoy 10% OFF on all programming assignments with the Spring Special Discount! Just use code SPRING10OFF at checkout! Why stress over deadlines when you can score high effortlessly? Grab this exclusive offer now and make this semester your best one yet!
We Accept
- Understanding the Basics of Binary Search Trees and Hash Table
- Binary Search Trees (BSTs)
- Key Operations in BSTs:
- Hash Tables
- Key Concepts in Hash Tables:
- Closed-Address Hashing with BSTs
- Designing the Data Structure
- Hash Table Design:
- Binary Search Tree Design:
- Implementing Core Functions
- Testing and Debugging
- Basic Tests:
- Performance Testing:
- Optimization and Refinement
- Conclusion
When faced with the challenge of creating custom data structures, the task often involves combining different algorithms and concepts to optimize performance and functionality. One such intriguing challenge is designing a dictionary that integrates binary search trees (BSTs) with closed-address hashing. This type of data structures and algorithms assignment not only tests your ability to implement fundamental data structures but also challenges you to understand and apply advanced techniques for efficient data retrieval and management.
In this guide, we'll explore the process of developing a dictionary that leverages both BSTs and hashing. We'll break down the key concepts, walk through the design and implementation steps, and provide insights into testing and validating your solution. By the end of this guide, you'll have a thorough understanding of how to tackle similar programming assignments and the skills needed to build robust data structures.
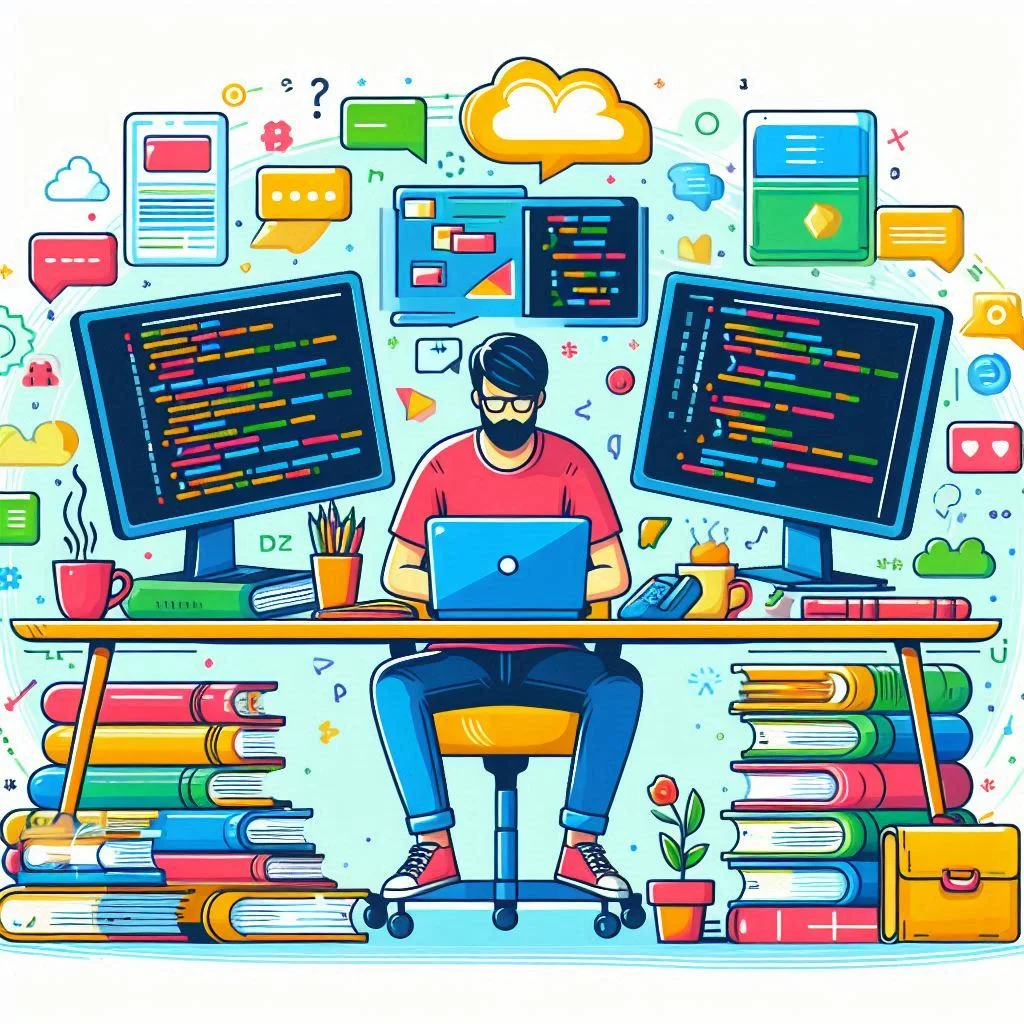
Understanding the Basics of Binary Search Trees and Hash Table
Binary Search Trees (BSTs)
A binary search tree (BST) is a type of binary tree in which each node has at most two children, referred to as the left and right children. The key property of a BST is that for any given node:
- All nodes in the left subtree have keys less than the node’s key.
- All nodes in the right subtree have keys greater than the node’s key.
This property allows for efficient search, insertion, and deletion operations, typically with an average time complexity of O(log n), where n is the number of nodes in the tree.
Key Operations in BSTs:
- Search: Traverse the tree from the root, comparing keys and moving left or right based on the comparisons.
- Insert: Similar to search, but if the target node is not found, a new node is inserted at the appropriate position.
- Delete: Remove a node while maintaining the BST property. This operation is more complex and involves handling three cases: deleting a leaf node, deleting a node with one child, and deleting a node with two children.
Hash Tables
A hash table is a data structure that maps keys to values for efficient data retrieval. It uses a hash function to compute an index into an array of buckets or slots, where the corresponding value is stored.
Key Concepts in Hash Tables:
- Hash Function: Converts a key into an index in the array. A good hash function minimizes collisions (when two keys hash to the same index).
- Collisions: Occur when multiple keys hash to the same index. Handling collisions can be done using methods like chaining (linked lists) or open addressing (probing).
Closed-Address Hashing with BSTs
In closed-address hashing, each slot in the hash table contains a data structure to handle collisions. Instead of using a linked list, we use a BST. This approach combines the benefits of hashing (efficient index computation) with the benefits of BSTs (efficient key-based operations).
Designing the Data Structure
For this assignment, the primary data structure involves a hash table where each slot contains a BST. This structure leverages the efficiency of hash tables for average-case time complexity and the sorted order and search efficiency of BSTs for worst-case scenarios.
Hash Table Design:
- Table Structure: Use a list to represent the hash table.
- Capacity: The capacity of the hash table is set during initialization and typically should be a prime number to minimize collisions.
- Hash Function: A good hash function is critical. It should distribute keys uniformly across the hash table to avoid clustering.
Binary Search Tree Design:
- Tree Nodes: Implement a TreeNode class to represent nodes in the BST. Each node should have attributes for the key, value, left child, and right child.
- BST Operations: Implement standard BST operations such as insertion, deletion, and search. To ensure efficiency, consider using self-balancing trees like AVL trees or Red-Black trees.
Implementing Core Functions
BST Operations:
1. TreeNode Class:
class TreeNode:
def __init__(self, key, value):
self.key = key
self.value = value
self.left = None
self.right = None
2. BST Insertion:
def bst_insert(root, key, value):
if root is None:
return TreeNode(key, value)
if key < root.key:
root.left = bst_insert(root.left, key, value)
elif key > root.key:
root.right = bst_insert(root.right, key, value)
else: # key == root.key
root.value = value # Update the value if key already exists
return root
3. BST Search:
def bst_search(root, key):
if root is None or root.key == key:
return root
if key < root.key:
return bst_search(root.left, key)
return bst_search(root.right, key)
4. BST Deletion:
def bst_delete(root, key):
if root is None:
return root
if key < root.key:
root.left = bst_delete(root.left, key)
elif key > root.key:
root.right = bst_delete(root.right, key)
else:
if root.left is None:
return root.right
elif root.right is None:
return root.left
temp = minValueNode(root.right)
root.key = temp.key
root.value = temp.value
root.right = bst_delete(root.right, temp.key)
return root
def minValueNode(node):
current = node
while current.left is not None:
current = current.left
return current
Hash Table Operations:
1. Hash Function:
def hash_function(key, capacity):
return hash(key) % capacity
2. Class Initialization (__init__):
class ClosedAddrUsingBSTDict:
def __init__(self, capacity=8):
self._capacity = capacity
self._table = [None] * capacity
self._size = 0
3. Set Item (__setitem__):
def __setitem__(self, key, value):
index = hash_function(key, self._capacity)
if self._table[index] is None:
self._table[index] = None
self._table[index] = bst_insert(self._table[index], key, value)
self._size += 1
4. Get Item (__getitem__):
def __getitem__(self, key):
index = hash_function(key, self._capacity)
node = bst_search(self._table[index], key)
if node is None:
return None
return node.value
5. Delete Item (__delitem__):
def __delitem__(self, key):
index = hash_function(key, self._capacity)
if self._table[index] is not None:
self._table[index] = bst_delete(self._table[index], key)
self._size -= 1
6. Containment Check (__contains__):
def __contains__(self, key): index = hash_function(key, self._capacity) return bst_search(self._table[index], key) is not None
7. Length Calculation (__len__):
def __len__(self): return self._size
8. String Representation (__str__):
def __str__(self): items = [] for bst in self._table: if bst: self._inorder(bst, items) return '{' + ', '.join(f'{k}: {v}' for k, v in items) + '}' def _inorder(self, node, items): if node: self._inorder(node.left, items) items.append((node.key, node.value)) self._inorder(node.right, items)
9. Iteration (__iter__):
def __iter__(self):
for bst in self._table:
if bst:
for key in self._inorder_keys(bst):
yield key
def _inorder_keys(self, node):
if node:
yield from self._inorder_keys(node.left)
yield node.key
yield from self._inorder_keys(node.right)
Testing and Debugging
Testing is critical to ensure that your implementation works correctly. Start with simple test cases and gradually move to more complex ones.
Basic Tests:
1. Initialization:
d = ClosedAddrUsingBSTDict()
assert len(d) == 0
print("Initialization test passed.")
2. Insertion and Retrieval:
d["apple"] = 1
assert d["apple"] == 1
assert len(d) == 1
print("Insertion and retrieval test passed.")
3. Containment Check:
assert "apple" in d
assert "banana" not in d
print("Containment check test passed.")
4. Deletion:
del d["apple"]
assert "apple" not in d
assert len(d) == 0
print("Deletion test passed.")
Advanced Tests:
1. Collision Handling:
d = ClosedAddrUsingBSTDict(capacity=4)
keys = ["apple", "banana", "grape", "cherry"]
values = [1, 2, 3, 4]
for k, v in zip(keys, values):
d[k] = v
assert all(d[k] == v for k, v in zip(keys, values))
print("Collision handling test passed.")
2. Large Dataset:
import random
d = ClosedAddrUsingBSTDict()
for i in range(1000):
d[f"key{i}"] = i
assert len(d) == 1000
for i in range(1000):
assert d[f"key{i}"] == i
print("Large dataset test passed.")
Performance Testing:
- Timing Comparisons: Use a timing program to compare the performance of your implementation with other dictionary types. Measure the time taken for insertions, deletions, and searches.
Example Timing Program:
import time
def time_operations(d, n=1000):
start = time.time()
for i in range(n):
d[f"key{i}"] = i
insertion_time = time.time() - start
start = time.time()
for i in range(n):
_ = d[f"key{i}"]
search_time = time.time() - start
start = time.time()
for i in range(n):
del d[f"key{i}"]
deletion_time = time.time() - start
return insertion_time, search_time, deletion_time
# Test with your implementation
d = ClosedAddrUsingBSTDict()
insertion_time, search_time, deletion_time = time_operations(d)
print(f"ClosedAddrUsingBSTDict - Insert: {insertion_time}s, Search: {search_time}s, Delete: {deletion_time}s")
Optimization and Refinement
After testing, focus on optimizing your code for efficiency:
- Self-Balancing BSTs: Consider using AVL trees or Red-Black trees to maintain balanced BSTs, which ensure O(log n) time complexity for insertions, deletions, and searches.
- Efficient Memory Usage: Ensure that your data structure uses memory efficiently, particularly for large datasets. Avoid unnecessary data duplication and manage memory allocation carefully.
- Dynamic Resizing: Implement dynamic resizing for the hash table to maintain a low load factor. Resize the table when the number of elements exceeds a certain threshold to avoid excessive collisions.
Example of Dynamic Resizing:
class ClosedAddrUsingBSTDict:
def __init__(self, capacity=8):
self._capacity = capacity
self._table = [None] * capacity
self._size = 0
def _resize(self):
new_capacity = self._capacity * 2
new_table = [None] * new_capacity
for bst in self._table:
if bst:
self._transfer_bst(bst, new_table, new_capacity)
self._table = new_table
self._capacity = new_capacity
def _transfer_bst(self, node, new_table, new_capacity):
if node:
self._transfer_bst(node.left, new_table, new_capacity)
self._transfer_bst(node.right, new_table, new_capacity)
index = hash_function(node.key, new_capacity)
if new_table[index] is None:
new_table[index] = None
new_table[index] = bst_insert(new_table[index], node.key, node.value)
def __setitem__(self, key, value):
if self._size > self._capacity * 0.75:
self._resize()
index = hash_function(key, self._capacity)
if self._table[index] is None:
self._table[index] = None
self._table[index] = bst_insert(self._table[index], key, value)
self._size += 1
Conclusion
Approaching complex programming assignments requires a systematic and methodical strategy. By breaking down the problem, designing appropriate data structures, implementing core functions, and thoroughly testing and optimizing your code, you can tackle any similar python assignment effectively. This comprehensive guide should serve as a valuable resource for mastering such tasks, paving the way for success in your computer science courses and beyond.
For additional resources and support on programming assignments, consider visiting Programming Homework Help. With practice and persistence, you'll become proficient in handling complex data structures and algorithms, enabling you to excel in your academic and professional pursuits.