Claim Your Offer
New semester, new challenges—but don’t stress, we’ve got your back! Get expert programming assignment help and breeze through Python, Java, C++, and more with ease. For a limited time, enjoy 10% OFF on all programming assignments with the Spring Special Discount! Just use code SPRING10OFF at checkout! Why stress over deadlines when you can score high effortlessly? Grab this exclusive offer now and make this semester your best one yet!
We Accept
CSV (Comma Separated Values) files are ubiquitous in data handling, serving as a bridge between different software and systems by providing a simple way to store and exchange tabular data. Whether you're managing a small dataset for a class project or handling large-scale data for professional analysis, understanding how to efficiently read, write, and analyze CSV files is crucial.
In Python, the csv module simplifies working with CSV files, making data manipulation straightforward and accessible. This guide will walk you through the fundamental techniques required to master CSV data handling, addressing common problems and offering practical solutions to help you solve your Python assignments effectively.
Introduction to CSV File Operations
CSV files are favored for their simplicity and versatility. They represent data in plain text format, where each line corresponds to a row of the table, and values within the row are separated by commas (or other delimiters). This format is widely supported across various platforms and applications, making it an essential skill for anyone working with data.
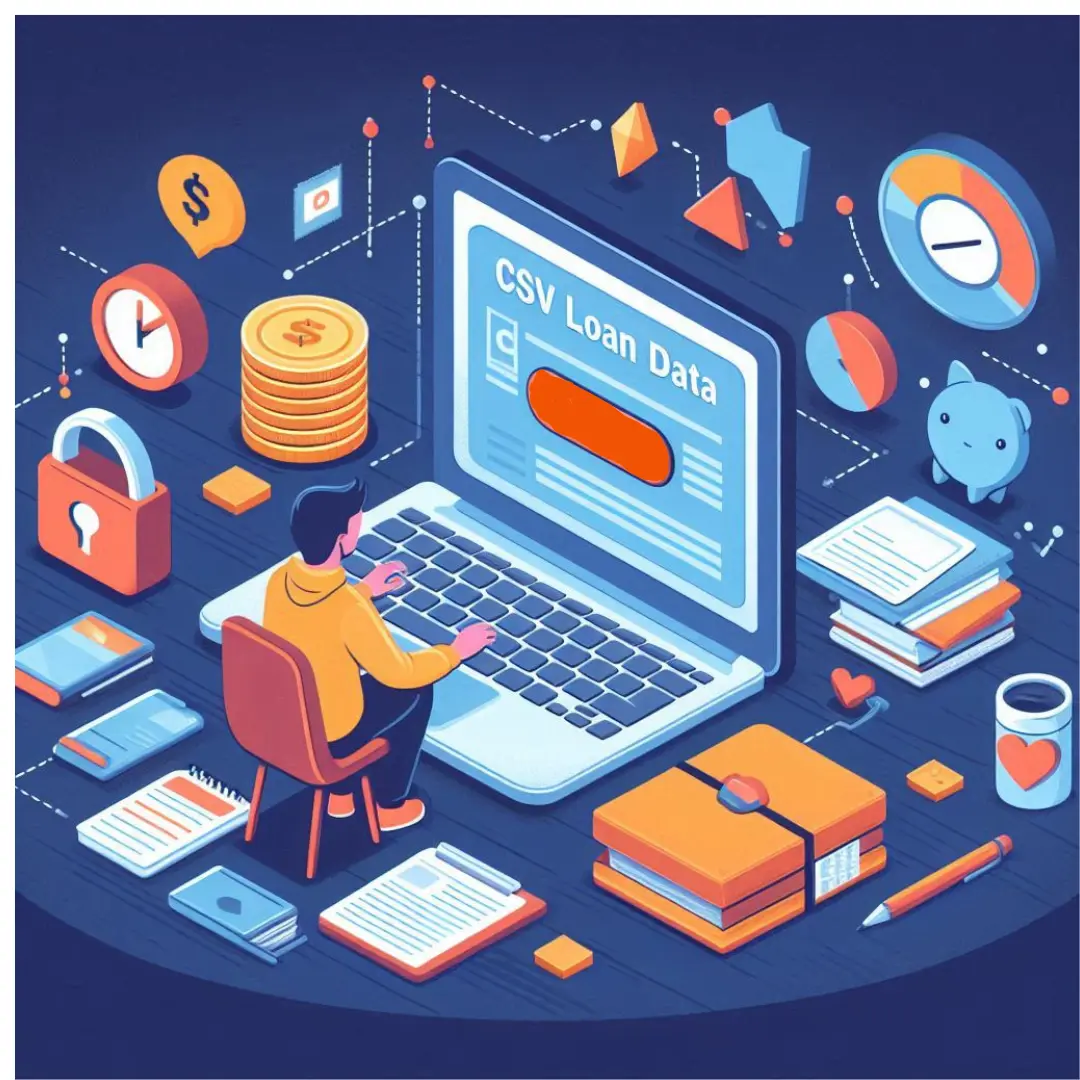
Python's csv module is a powerful tool for reading from and writing to CSV files. This module provides two primary classes: csv.reader for reading and csv.writer for writing.
Reading from a CSV File
To read data from a CSV file, follow these steps:
- Open the File: Use Python’s built-in open() function.
- Create a CSV Reader Object: Pass the file object to csv.reader().
- Iterate Through Rows: Use a loop to process each row of data.
Here’s a basic example of reading a CSV file:
import csv
def read_csv(file_path):
with open(file_path, mode='r') as file:
csv_reader = csv.reader(file)
for row in csv_reader:
print(row)
# Example usage
read_csv('novels.csv')
In this example:
- The with open(file_path, mode='r') statement opens the file in read mode and ensures it is properly closed after reading.
- csv.reader(file) creates a reader object that can iterate over lines in the CSV file.
- The loop prints each row, where each row is represented as a list of values.
Writing to a CSV File
To write data to a CSV file, follow these steps:
- Open the File: Use open() with write mode.
- Create a CSV Writer Object: Pass the file object to csv.writer().
- Write Rows: Use writerow() to write a single row or writerows() to write multiple rows.
Here’s an example of writing to a CSV file:
import csv
def write_csv(file_path, header, rows):
with open(file_path, mode='w', newline='') as file:
csv_writer = csv.writer(file)
csv_writer.writerow(header)
csv_writer.writerows(rows)
# Example usage
header = ['Novel', 'Year First Published', 'Author']
rows = [['Silas Marner', 1861, 'George Eliott'],
['Great Expectations', 1861, 'Charles Dickens'],
['The Hunger Games', 2011, 'Suzanne Collins']]
write_csv('novels.csv', header, rows)
In this example:
- mode='w' specifies write mode, creating a new file or overwriting an existing one.
- newline='' prevents extra blank lines in the output on some platforms.
- csv_writer.writerow(header) writes the header row, and csv_writer.writerows(rows) writes multiple rows.
Problem-Specific Solutions
Problem 1: Generating a Report of Books Borrowed in 2019
To solve this problem, you need to:
- Read the Book Data: Load book information from books.csv.
- Read the Loan Data: Load loan records from bookloans.csv.
- Filter Loans by Year: Convert loan dates from Excel format to Python datetime objects and filter for the year 2019.
- Compute Loan Statistics: Calculate the total number of days each book was loaned and sort the results.
Here’s a step-by-step solution:
import csv
from datetime import datetime, timedelta
# Convert Excel date to Python datetime
def excel_date_to_date(excel_date):
start_date = datetime(1899, 12, 30)
return start_date + timedelta(days=excel_date)
# Load book data
def load_books(file_path):
books = {}
with open(file_path, mode='r') as file:
csv_reader = csv.reader(file)
next(csv_reader) # Skip header
for row in csv_reader:
book_id, title, author = row
books[book_id] = {'title': title, 'author': author}
return books
# Process loan data
def process_loans(books, loans_file):
loan_summary = {}
with open(loans_file, mode='r') as file:
csv_reader = csv.reader(file)
for row in csv_reader:
book_id, _, loan_date, return_date = row
if book_id not in books:
continue
loan_date = excel_date_to_date(int(loan_date))
return_date = excel_date_to_date(int(return_date))
days_loaned = (return_date - loan_date).days
if book_id in loan_summary:
loan_summary[book_id]['total_days'] += days_loaned
loan_summary[book_id]['loan_count'] += 1
else:
loan_summary[book_id] = {
'title': books[book_id]['title'],
'author': books[book_id]['author'],
'total_days': days_loaned,
'loan_count': 1
}
return loan_summary
# Generate report
def generate_report(loan_summary):
sorted_summary = sorted(loan_summary.items(), key=lambda x: x[1]['loan_count'])
for book_id, info in sorted_summary:
print(f"Book: {info['title']}, Author: {info['author']}, Days Loaned: {info['total_days']}, Loan Count: {info['loan_count']}")
# Main function
def main():
books = load_books('books.csv')
loan_summary = process_loans(books, 'bookloans.csv')
generate_report(loan_summary)
# Execute
main()
Explanation:
- excel_date_to_date() converts Excel serial dates to Python datetime objects.
- load_books() reads the book information and stores it in a dictionary.
- process_loans() reads the loan records, computes loan statistics, and stores them in a dictionary.
- generate_report() sorts and prints the loan summary.
Problem 2: Report on Genres and Sub-Genres Borrowed
To generate reports on genres and sub-genres, follow these steps:
- Extend Book Data: Include genre information in books.csv.
- Analyze Genre Data: Track and count loans by genre and sub-genre.
Here’s the code to achieve this:
import csv
from collections import defaultdict
# Load book data with genres
def load_books_with_genres(file_path):
books = {}
with open(file_path, mode='r') as file:
csv_reader = csv.reader(file)
next(csv_reader) # Skip header
for row in csv_reader:
book_id, title, author, genre, sub_genre = row
books[book_id] = {
'title': title,
'author': author,
'genre': genre,
'sub_genre': sub_genre
}
return books
# Analyze genres and sub-genres
def analyze_genres_and_sub_genres(books, loans_file):
genre_counts = defaultdict(int)
sub_genre_counts = defaultdict(int)
with open(loans_file, mode='r') as file:
csv_reader = csv.reader(file)
for row in csv_reader:
book_id, _, loan_date, return_date = row
if book_id not in books:
continue
genre = books[book_id]['genre']
sub_genre = books[book_id]['sub_genre']
genre_counts[genre] += 1
sub_genre_counts[sub_genre] += 1
# Print genre counts
print("Genres Borrowed:")
for genre, count in sorted(genre_counts.items(), key=lambda x: x[1]):
print(f"Genre: {genre}, Count: {count}")
# Print sub-genre counts
print("\nSub-Genres Borrowed:")
for sub_genre, count in sorted(sub_genre_counts.items(), key=lambda x: x[1]):
print(f"Sub-Genre: {sub_genre}, Count: {count}")
# Main function
def main_genres_and_sub_genres():
books = load_books_with_genres('books.csv')
analyze_genres_and_sub_genres(books, 'bookloans.csv')
# Execute
main_genres_and_sub_genres()
Explanation:
- load_books_with_genres() now includes genre and sub-genre information.
- analyze_genres_and_sub_genres() tracks loan counts by genre and sub-genre, then prints the results sorted by frequency.
Problem 3: Calculating Average Loan Durations
To compute average loan durations and handle late returns, follow these steps:
- Calculate Total Days and Number of Loans.
- Calculate Late Returns and Average Late Duration.
Here’s the code to perform these calculations:
import csv
from datetime import datetime, timedelta
from collections import defaultdict
# Convert Excel date to Python datetime (same as before)
def excel_date_to_date(excel_date):
start_date = datetime(1899, 12, 30)
return start_date + timedelta(days=excel_date)
# Analyze loan durations
def analyze_loan_durations(books_file, loans_file):
loan_summary = defaultdict(lambda: {'total_days': 0, 'loan_count': 0, 'late_days': 0})
with open(loans_file, mode='r') as file:
csv_reader = csv.reader(file)
for row in csv_reader:
book_id, _, loan_date, return_date = row
loan_date = excel_date_to_date(int(loan_date))
return_date = excel_date_to_date(int(return_date))
days_loaned = (return_date - loan_date).days
late_days = max(0, days_loaned - 14)
loan_summary[book_id]['total_days'] += days_loaned
loan_summary[book_id]['loan_count'] += 1
loan_summary[book_id]['late_days'] += late_days
total_days = sum(info['total_days'] for info in loan_summary.values())
total_loans = sum(info['loan_count'] for info in loan_summary.values())
total_late_days = sum(info['late_days'] for info in loan_summary.values())
late_loans = sum(1 for info in loan_summary.values() if info['late_days'] > 0)
avg_days = total_days / total_loans
late_percentage = (late_loans / total_loans) * 100
avg_late_days = total_late_days / late_loans if late_loans > 0 else 0
# Print reports
print(f"Total Days Borrowed: {total_days}")
print(f"Total Loans: {total_loans}")
print(f"Average Loan Duration: {avg_days:.1f} days")
print(f"Percentage of Late Returns: {late_percentage:.1f}%")
print(f"Average Late Return Duration: {avg_late_days:.1f} days")
# Main function
def main_loan_durations():
analyze_loan_durations('books.csv', 'bookloans.csv')
# Execute
main_loan_durations()
Explanation:
- analyze_loan_durations() calculates total days, average loan duration, late returns percentage, and average late return duration.
Conclusion
CSV file handling and data analysis in Python is an invaluable skill for students and professionals alike. In this guide, we've explored how to efficiently work with CSV files to perform essential data operations and generate meaningful insights. Here's a summary of what we've covered:
- CSV File Operations: We started with the basics of reading from and writing to CSV files using Python's csv module. This foundational knowledge is crucial for handling tabular data in a straightforward and efficient manner.
- Generating Detailed Reports: We tackled the problem of generating a report for books borrowed in 2019. This involved reading and processing data, calculating loan statistics, and presenting the results in a clear, sorted format. This step demonstrated how to extract and organize data to gain actionable insights.
- Analyzing Genres and Sub-Genres: We extended our analysis to include genres and sub-genres, showing how to track and report on different categories of data. This part highlighted the importance of categorizing and aggregating data to understand trends and preferences.
- Calculating Average Loan Durations: Finally, we calculated various statistics related to loan durations, including average loan periods and late returns. This analysis is critical for evaluating performance metrics and understanding patterns in the data.
These exercises not only provide practical solutions to specific problems but also illustrate broader concepts in data handling and analysis. By mastering these techniques, you are better equipped to tackle complex data assignments and real-world challenges. Whether you're working on academic projects, professional tasks, or seeking assistance with programming assignments, the ability to manipulate and analyze data effectively is a powerful asset.
As you continue to work with data, remember that these skills are foundational and can be applied to a wide range of scenarios. The principles of data processing, analysis, and reporting are consistent across various types of data and formats. Keep experimenting with different datasets and problem types to deepen your understanding and enhance your proficiency.
If you encounter new challenges or have specific questions, don’t hesitate to seek help or explore additional resources. With practice and curiosity, you'll continue to grow as a skilled data analyst and programmer.