Claim Your Discount Today
Take your coding game to new heights with expert help at unbeatable prices. Got a tricky project or a tight deadline? We’ve got you covered! Use code PHHBF10 at checkout and save BIG. Don’t wait—this exclusive Black Friday deal won’t last long. Secure your academic success today!
We Accept
In today’s interconnected world, peer-to-peer (P2P) systems have revolutionized how files are shared, eliminating reliance on centralized servers. Developing a P2P file-sharing system in Java is an ideal project for college students learning about network programming, multithreading, and client-server architectures.
This blog provides a complete guide to building such a system, with practical examples and Java code. Whether you're tackling this project alone or need programming assignment help, this step-by-step tutorial will be your ultimate resource. Additionally, if you’re pressed for time and wondering, "Can I pay someone to do my Java assignment?", the answer is yes—many professional platforms are ready to assist.
What Is a Peer-to-Peer File Sharing System?
A peer-to-peer (P2P) file-sharing system allows users to share files directly, without needing a central server. Each peer acts as both a client and a server, enabling decentralized communication.
This system has real-world applications in software like BitTorrent, where large files are shared efficiently between users. For university assignments, implementing a simplified P2P system can demonstrate your grasp of network protocols, Java sockets, and multithreading.
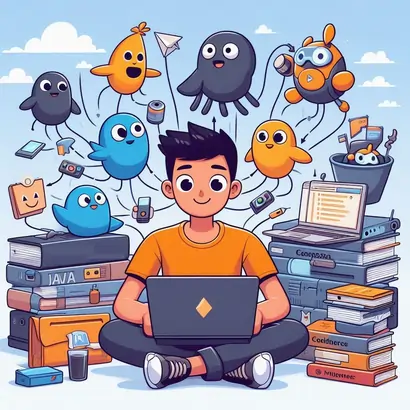
Core Features of Our P2P File Sharing System
Our P2P file-sharing system includes the following functionalities:
- Peer Registration: Peers register their IP addresses and the files they want to share with a centralized tracker.
- File Discovery: A peer can request a file, and the tracker identifies peers that host the requested file.
- File Transfer: Peers establish a direct connection to share files without involving the tracker further.
- Multithreaded Operations: The system supports simultaneous connections for efficient file sharing.
Step-by-Step Implementation
To keep things manageable, we will break the system into three main components:
- Tracker: Centralized service for file registration and peer discovery.
- Peer: A participant that registers files, shares files, and requests files.
- File Requester: Handles the process of finding and downloading files from other peers.
- Building the Tracker
The tracker maintains a registry of available files and their hosting peers. Here’s the implementation:
import java.io.*; import java.net.*; import java.util.*; public class Tracker { private static Map<string, list<string="">> fileRegistry = new HashMap<>(); public static void main(String[] args) throws IOException { ServerSocket serverSocket = new ServerSocket(5000); System.out.println("Tracker is up and running..."); while (true) { Socket clientSocket = serverSocket.accept(); new Thread(new PeerHandler(clientSocket)).start(); } } static class PeerHandler implements Runnable { private Socket socket; public PeerHandler(Socket socket) { this.socket = socket; } @Override public void run() { try { BufferedReader input = new BufferedReader(new InputStreamReader(socket.getInputStream())); PrintWriter output = new PrintWriter(socket.getOutputStream(), true); String command = input.readLine(); if (command.startsWith("REGISTER")) { String[] parts = command.split(" "); String fileName = parts[1]; String peerAddress = parts[2]; fileRegistry.putIfAbsent(fileName, new ArrayList<>()); fileRegistry.get(fileName).add(peerAddress); output.println("File registered successfully."); } else if (command.startsWith("REQUEST")) { String[] parts = command.split(" "); String fileName = parts[1]; if (fileRegistry.containsKey(fileName)) { output.println(String.join(",", fileRegistry.get(fileName))); } else { output.println("File not found."); } } socket.close(); } catch (IOException e) { e.printStackTrace(); } } } }
This tracker allows peers to register files and query for available files hosted by other peers.
- Creating the Peer
Each peer registers its files with the tracker and listens for file requests. Here's the peer implementation:
import java.io.*; import java.net.*; public class Peer { public static void main(String[] args) throws IOException { BufferedReader console = new BufferedReader(new InputStreamReader(System.in)); System.out.println("Enter Tracker IP:"); String trackerIP = console.readLine(); System.out.println("Enter Tracker Port:"); int trackerPort = Integer.parseInt(console.readLine()); System.out.println("Enter file name to register:"); String fileName = console.readLine(); registerFile(trackerIP, trackerPort, fileName); startFileServer(fileName); } private static void registerFile(String trackerIP, int trackerPort, String fileName) throws IOException { Socket socket = new Socket(trackerIP, trackerPort); PrintWriter out = new PrintWriter(socket.getOutputStream(), true); out.println("REGISTER " + fileName + " " + InetAddress.getLocalHost().getHostAddress()); BufferedReader in = new BufferedReader(new InputStreamReader(socket.getInputStream())); System.out.println(in.readLine()); socket.close(); } private static void startFileServer(String fileName) throws IOException { ServerSocket serverSocket = new ServerSocket(6000); System.out.println("Peer server started on port 6000..."); while (true) { Socket clientSocket = serverSocket.accept(); new Thread(() -> handleRequest(clientSocket, fileName)).start(); } } private static void handleRequest(Socket clientSocket, String fileName) { try { BufferedReader in = new BufferedReader(new InputStreamReader(clientSocket.getInputStream())); PrintWriter out = new PrintWriter(clientSocket.getOutputStream(), true); String request = in.readLine(); if (request.equals("GET " + fileName)) { out.println("Transferring file..."); // Simulate file transfer Thread.sleep(2000); out.println("File transfer complete."); } else { out.println("File not available."); } clientSocket.close(); } catch (Exception e) { e.printStackTrace(); } } }
This peer registers its file and listens for incoming requests to share the file.
- Requesting Files
A peer requesting a file first queries the tracker for hosting peers and then connects to one of them to download the file.
import java.io.*; import java.net.*; public class FileRequester { public static void main(String[] args) throws IOException { BufferedReader console = new BufferedReader(new InputStreamReader(System.in)); System.out.println("Enter Tracker IP:"); String trackerIP = console.readLine(); System.out.println("Enter Tracker Port:"); int trackerPort = Integer.parseInt(console.readLine()); System.out.println("Enter file name to request:"); String fileName = console.readLine(); Socket trackerSocket = new Socket(trackerIP, trackerPort); PrintWriter out = new PrintWriter(trackerSocket.getOutputStream(), true); out.println("REQUEST " + fileName); BufferedReader in = new BufferedReader(new InputStreamReader(trackerSocket.getInputStream())); String peers = in.readLine(); trackerSocket.close(); if (peers.equals("File not found.")) { System.out.println("File not available."); } else { String[] peerList = peers.split(","); for (String peer : peerList) { if (requestFileFromPeer(peer, fileName)) { break; } } } } private static boolean requestFileFromPeer(String peer, String fileName) { try { Socket socket = new Socket(peer, 6000); PrintWriter out = new PrintWriter(socket.getOutputStream(), true); BufferedReader in = new BufferedReader(new InputStreamReader(socket.getInputStream())); out.println("GET " + fileName); System.out.println(in.readLine()); System.out.println(in.readLine()); socket.close(); return true; } catch (IOException e) { System.out.println("Failed to connect to peer: " + peer); return false; } } }
Conclusion
Creating a P2P file-sharing system using Java is a rewarding way to enhance your programming skills. This project covers essential concepts like socket programming, multithreading, and client-server communication. If you’re facing challenges with similar assignments, consider reaching out for programming assignment help. Whether you want guidance or are thinking, "I need to pay someone to do my Java assignment," professional services can make a significant difference in mastering these concepts. Visit www.programminghomeworkhelp.com for expert assistance and resources tailored to your academic needs. By following this guide, you’re one step closer to building robust and efficient P2P systems, an invaluable skill for modern software development!