Claim Your Discount Today
Ring in Christmas and New Year with a special treat from www.programminghomeworkhelp.com! Get 15% off on all programming assignments when you use the code PHHCNY15 for expert assistance. Don’t miss this festive offer—available for a limited time. Start your New Year with academic success and savings. Act now and save!
We Accept
- Understanding the Core Concepts
- Sockets and Networking
- Client-Server Architecture
- Message Passing and Protocol Design
- Building the Chat Server
- Setting Up the Server
- Choosing a Port and Setting Up the Socket
- Handling Client Connections
- Managing Client Messages
- Developing the Chat Client
- Setting Up the Client Socket
- Receiving Messages from the Server
- Handling User Input
- Graceful Exit
- Additional Features and Considerations
- Scaling the Chat System
- Testing and Deployment
- Conclusion
In today's digital age, chat systems have become an integral part of online communication. Whether it's for social interaction, customer service, or collaboration, chat systems are ubiquitous. This blog will guide you through the process of creating a simple multi-user chat system in Python using TCP sockets. While the specific example focuses on a centralized chat server and multiple clients, the principles and steps can be applied to any similar programming assignment. If you're looking for help with Python assignments, this guide will provide you with valuable insights. Although we'll reference the concept of a multi-user chat system, the strategies discussed can be applied to various types of socket programming projects.
Understanding the Core Concepts
Before diving into the code, it’s crucial to understand the key components that make up a multi-user chat system. This understanding will help you grasp the underlying architecture and logic required to implement such a system effectively.
Sockets and Networking
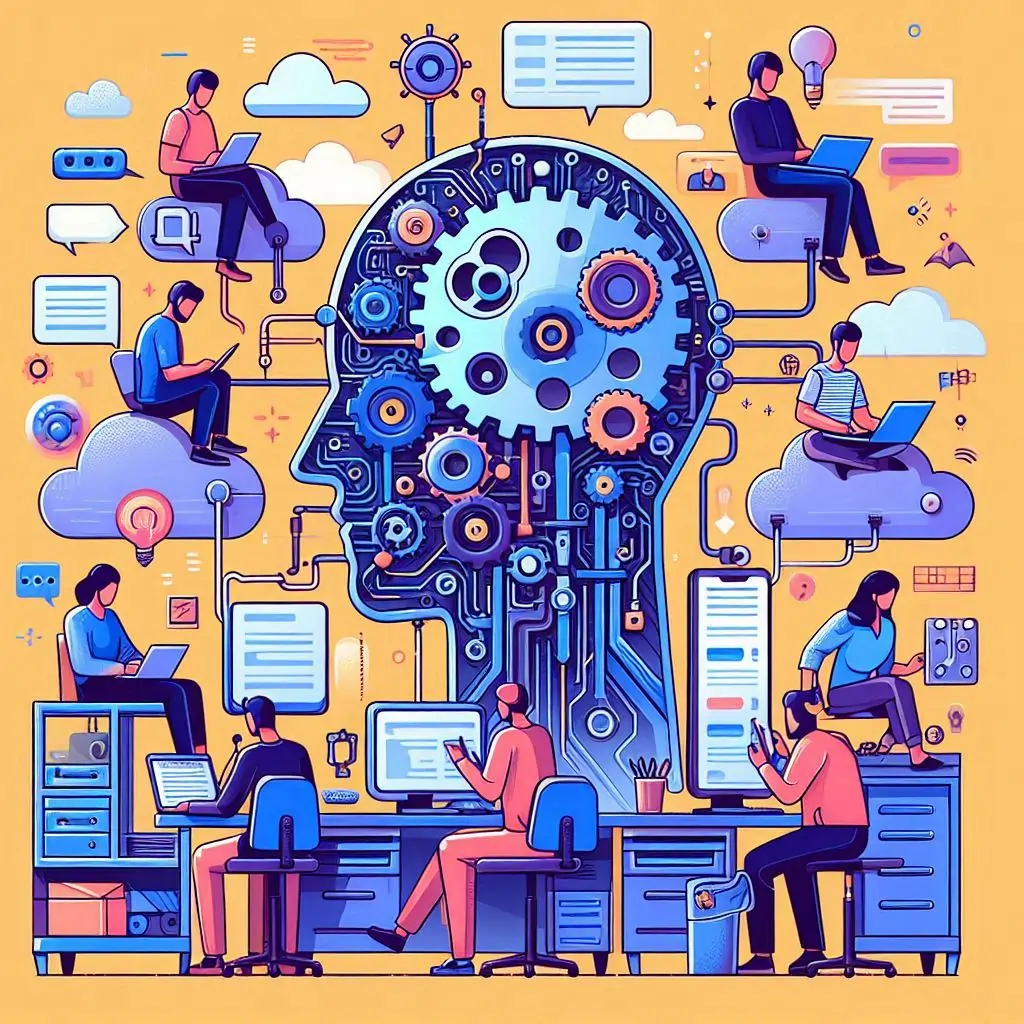
In any chat system, communication between clients and servers happens over a network. This communication is facilitated by sockets, which are endpoints for sending and receiving data. In our case, we’ll use TCP (Transmission Control Protocol) sockets, which ensure reliable, ordered, and error-checked delivery of data between the server and clients.
- TCP Sockets: These sockets establish a connection between the server and client, allowing for continuous communication. The server listens on a specific port, and clients connect to this port to exchange messages.
- IP Address and Ports: Each device on a network has a unique IP address, and each process on that device uses a port to communicate. In our chat system, the server runs on a known IP address and port, and clients connect using this information.
Client-Server Architecture
The client-server model is a network architecture where multiple clients (users) communicate with a central server. The server manages all the connections, ensuring that messages sent by one client are broadcasted to all other connected clients.
- Server: The server’s primary role is to listen for incoming connections, manage active connections, and relay messages between clients. It keeps track of who is connected and ensures that messages are delivered to the appropriate recipients.
- Client: The client is the user-facing part of the chat system. It connects to the server, allows users to send messages, and displays messages received from other clients.
Message Passing and Protocol Design
The core of a chat system lies in its ability to handle message passing effectively. This involves designing a simple protocol that both the server and clients understand.
- Message Format: Every message sent over the network should have a standard format, including the sender’s name, the message content, and a timestamp. This format ensures consistency and helps in easily identifying and displaying messages on the client side.
- Sign-In and Sign-Off: Special messages need to be handled for users signing in and out. These messages notify all connected clients about the new user or inform them when a user has left.
Building the Chat Server
The server is the backbone of our chat system. It handles multiple client connections, manages the chatroom, and ensures that messages are correctly relayed between clients.
Setting Up the Server
The first step in building our chat system is setting up the server. The server needs to listen for incoming connections on a specific port, manage connected clients, and facilitate the communication between them.
Choosing a Port and Setting Up the Socket
To begin, we choose a port for the server to listen on. This port should be known to the clients so that they can connect to the server.
import socket
def start_server():
server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server_socket.bind(('localhost', 12345)) # Use a known port
server_socket.listen()
print("Server started, waiting for connections...")
clients = []
In the code snippet above, we create a TCP socket and bind it to the local IP address (localhost) and a specific port (12345). The listen() method allows the server to start listening for incoming client connections.
Handling Client Connections
Once the server is set up, it needs to handle multiple client connections simultaneously. This can be achieved using threading, which allows the server to manage each client connection independently.
import threading
def handle_client(client_socket, client_address):
# Handle communication with the client
pass
def start_server():
# ... (previous code)
while True:
client_socket, client_address = server_socket.accept()
clients.append(client_socket)
threading.Thread(target=handle_client, args=(client_socket, client_address)).start()
In this code, the accept() method waits for a new client to connect. When a client connects, a new thread is started to handle communication with that client, allowing the server to continue accepting new connections.
Managing Client Messages
The server’s primary role is to relay messages between clients. For this, it needs to receive messages from clients, append the sender’s name and timestamp, and broadcast the message to all other clients.
import time
def handle_client(client_socket, client_address):
client_socket.send("Welcome to the chat!".encode())
clients.append(client_socket)
while True:
try:
message = client_socket.recv(1024).decode()
if message == '.':
break
broadcast_message(f"{client_address} says: {message}", client_socket)
except:
clients.remove(client_socket)
break
client_socket.close()
def broadcast_message(message, sender_socket):
for client in clients:
if client != sender_socket:
client.send(message.encode())
In this implementation, the handle_client() function manages the client’s connection, including receiving messages and broadcasting them. The broadcast_message() function sends the message to all clients except the sender, ensuring that the sender doesn’t receive their own message.
Developing the Chat Client
The client is what the end-user interacts with. It connects to the server, allows the user to send messages, and displays messages received from other clients.
Connecting to the Server
The first task for the client is to connect to the server. The client must know the server’s IP address and port number to establish this connection.
Setting Up the Client Socket
To connect to the server, the client sets up a TCP socket and uses it to initiate a connection.
def start_client(): client_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM) client_socket.connect(('localhost', 12345)) # Server's address threading.Thread(target=receive_messages, args=(client_socket,)).start() send_messages(client_socket)
In this code, the client socket connects to the server’s IP address (localhost) and port (12345). The client then starts two threads: one for receiving messages and another for sending messages.
Receiving Messages from the Server
The client needs to continuously listen for incoming messages from the server. These messages are displayed to the user in real-time.
def receive_messages(client_socket):
while True:
try:
message = client_socket.recv(1024).decode()
print(message)
except:
break
The receive_messages() function runs in a loop, continuously checking for new messages from the server. If a message is received, it’s printed on the client’s console.
Sending Messages to the Server
The client also needs to handle user input, sending each message to the server as the user types it.
Handling User Input
The client prompts the user to enter a username and messages. These messages are then sent to the server.
def send_messages(client_socket):
username = input("Enter your username: ")
client_socket.send(username.encode())
while True:
message = input()
if message == '.':
client_socket.send(message.encode())
break
client_socket.send(f"{username}: {message}".encode())
In this function, the client first prompts the user for a username, which is sent to the server. Then, in a loop, the client reads user input and sends it to the server. If the user enters a period (.), the client sends a sign-off message and breaks the loop, effectively ending the session.
Graceful Exit
When the user decides to leave the chat, the client needs to ensure that the server is informed, and the connection is closed gracefully.
def send_messages(client_socket):
# ... (previous code)
if message == '.':
client_socket.send(message.encode())
print("You have left the chat.")
client_socket.close()
break
This ensures that when the user exits the chat by typing . the client sends a sign-off message to the server, closes the connection, and exits the loop.
Additional Features and Considerations
Building a basic multi-user chat system is a great start, but there are additional features and considerations that can enhance the user experience and the robustness of your system.
Implementing User Authentication
In a more complex system, you might want to implement user authentication. This could involve requiring users to log in with a username and password before joining the chat.
- User Authentication: Implementing a login system adds a layer of security. Users can have unique accounts, and only registered users are allowed to join the chat.
- Password Management: For secure authentication, consider hashing passwords and storing them securely. Use libraries like bcrypt for hashing.
Handling Multiple Chat Rooms
Another enhancement could be the introduction of multiple chat rooms. This allows users to join specific chat rooms, each with its own set of participants and messages.
- Chat Rooms: Instead of a single chat room, you can create multiple chat rooms, each with its own set of users. Users can join and leave chat rooms as they wish.
- Room Management: The server can manage multiple rooms, routing messages to the appropriate room based on the client’s selection.
Improving Message Handling and UI
Enhancing the user interface and message handling can significantly improve the usability of your chat system.
- Message Formatting: Consider adding formatting options for messages, such as bold, italics, or colored text. This makes the chat more engaging and easier to follow.
- Graphical User Interface (GUI): Instead of relying on a command-line interface, you can build a more user-friendly and visually appealing GUI using a library like Tkinter, PyQt, or Kivy. A GUI allows users to interact with the chat system through windows, buttons, and other graphical elements, making the chat experience more intuitive.
- Message Timestamps: Adding timestamps to messages can help users keep track of when messages were sent and received. This feature is particularly useful in group chats where multiple users are interacting simultaneously.
- Notification System: Implement a notification system that alerts users when they receive a new message, especially if they are working on other tasks. This can be done using desktop notifications or visual indicators within the chat window.
Scaling the Chat System
As your chat system grows, you may need to consider scalability. This involves making sure that your system can handle an increasing number of users without performance degradation.
Handling More Clients
As the number of clients increases, the server needs to efficiently manage connections and data flow.
- Asynchronous Programming: To handle a large number of clients, consider using asynchronous programming techniques. Libraries like asyncio in Python allow for non-blocking I/O operations, enabling the server to handle multiple clients more efficiently.
- Load Balancing: If your chat system grows significantly, you might need to implement load balancing. This involves distributing the incoming client connections across multiple servers to prevent any single server from becoming overwhelmed.
- Database Integration: For a large-scale system, you may need to integrate a database to store user data, chat history, and other information. Using a database allows for more complex features, such as persistent chat history and user profiles.
Security Considerations
Security is a critical aspect of any online communication system. As you scale your chat system, it’s important to ensure that it is secure from potential threats.
- Encryption: To protect user data, implement encryption for all data transmitted between clients and the server. SSL/TLS protocols can be used to encrypt the data, ensuring that it cannot be intercepted or tampered with by malicious actors.
- Input Validation: Always validate user input to prevent common security vulnerabilities like SQL injection or cross-site scripting (XSS). This is especially important if you are storing user input in a database or displaying it on a web interface.
- Rate Limiting: Implement rate limiting to prevent abuse of the chat system, such as spamming. Rate limiting controls how frequently a user can send messages, helping to maintain a healthy chat environment.
Optimizing Performance
As your chat system handles more users, optimizing performance becomes crucial. Here are some strategies to ensure your system runs smoothly:
- Efficient Data Handling: Optimize how data is handled between the server and clients. For example, minimize the size of messages sent over the network to reduce bandwidth usage.
- Caching: Implement caching mechanisms to store frequently accessed data temporarily. This reduces the load on the server and improves response times.
- Resource Management: Monitor and manage server resources such as CPU, memory, and network bandwidth. Use tools to analyze performance and identify bottlenecks that could affect scalability.
Testing and Deployment
After building your chat system, thorough testing and proper deployment are essential to ensure that it functions correctly in a real-world environment.
Unit Testing
Unit testing involves testing individual components of your chat system to ensure that they work as expected.
- Test Cases: Write test cases for critical components such as the server’s message broadcasting function, client connection handling, and user authentication. This helps catch bugs early in the development process.
- Mocking: Use mocking techniques to simulate client-server interactions during testing. This allows you to test server functionality without requiring multiple clients to be connected simultaneously.
Load Testing
Load testing evaluates how your chat system performs under various levels of user activity.
- Simulate Users: Use load testing tools to simulate multiple users interacting with the chat system. This helps identify performance issues that may arise when the system is under heavy load.
- Measure Performance: Monitor key performance indicators such as response time, throughput, and error rates during load testing. This data can guide optimizations to improve scalability.
Deployment Strategies
Once your chat system has been thoroughly tested, it’s time to deploy it to a live environment.
- Cloud Deployment: Consider deploying your chat system on cloud platforms like AWS, Azure, or Google Cloud. These platforms offer scalable infrastructure and services that can help manage server load and provide global availability.
- Containerization: Use containerization technologies like Docker to package your chat application and its dependencies into a portable container. This simplifies deployment and ensures consistency across different environments.
- Continuous Integration/Continuous Deployment (CI/CD): Implement CI/CD pipelines to automate the testing and deployment process. This allows you to quickly and reliably deploy updates and bug fixes to your chat system.
Conclusion
Building a multi-user chat system in Python is an excellent way to explore and understand the fundamentals of network programming, client-server architecture, and real-time communication. By following this comprehensive guide, you can create a robust chat system that not only meets the requirements of a programming assignment but also scales to handle real-world use cases. From setting up a basic server-client communication framework to implementing additional features like user authentication, multiple chat rooms, and security enhancements, this guide covers the essential steps to develop a fully functional chat system. Furthermore, by considering scalability, performance optimization, and thorough testing, you can ensure that your chat system is both reliable and efficient.Whether you’re a student tackling a programming assignment or a developer looking to build a scalable chat application, this guide provides the knowledge and tools you need to succeed. With the principles and techniques outlined here, you’ll be well-equipped to handle any similar programming challenges that come your way.