Claim Your Offer
Unlock an amazing offer at www.programminghomeworkhelp.com with our latest promotion. Get an incredible 10% off on your all programming assignment, ensuring top-quality assistance at an affordable price. Our team of expert programmers is here to help you, making your academic journey smoother and more cost-effective. Don't miss this chance to improve your skills and save on your studies. Take advantage of our offer now and secure exceptional help for your programming assignments.
We Accept
- Understanding the Problem
- Key Components of the System
- Essential Operations
- Setting Up the Project
- 1. Project Structure
- 2. Implementing the Red-Black Tree
- 3. Implementing the Min-Heap
- 4. Library Operations
- 5. Main Class
- 6. Compiling and Running the Project
- Conclusion
Creating a library management system is a challenging yet rewarding project that integrates fundamental programming concepts. In this blog, we will walk you through the essential steps to develop a GatorLibrary Management System using Java. This system effectively handles the management of books, patrons, and borrowing operations by leveraging sophisticated data structures like Red-Black Trees for efficient book storage and retrieval, and Binary Min-Heaps for managing book reservations. By implementing these structures, the system ensures optimal performance in handling book availability, patron borrowing requests, and reservation prioritization. Through detailed explanations and practical examples, you will gain insights into designing and implementing each component of the system, from inserting and deleting books to managing reservations and handling patron interactions. Whether you are a student looking to understand these concepts or seeking help with your Java assignment, this guide will provide you with valuable knowledge and hands-on experience in building robust library management systems.
Understanding the Problem
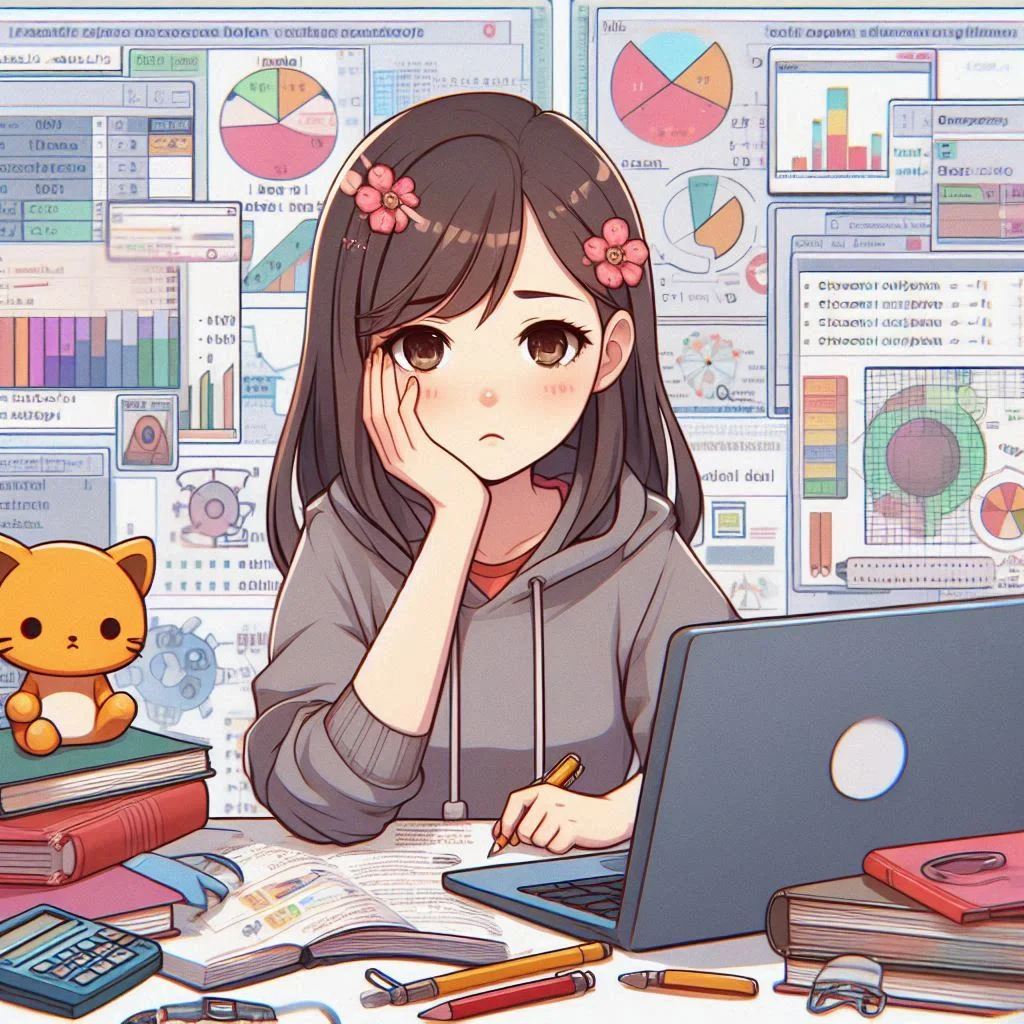
The GatorLibrary Management System needs to:
- Manage books and their details: This includes maintaining comprehensive information about each book, such as BookID, BookName, AuthorName, AvailabilityStatus, and BorrowedBy. This ensures accurate tracking of book statuses within the library's inventory.
- Track patrons who borrow books: The system must keep records of patrons who borrow books, facilitating efficient transaction management and preventing conflicts over book availability.
- Handle book reservations using a priority queue: Implementing a priority queue ensures orderly management of book reservations. Patrons can reserve books that are currently unavailable, prioritized by criteria like patron priority level and reservation timestamp.
- Use a Red-Black Tree for efficient book management: This balanced binary search tree structure is employed to optimize the storage and retrieval of books. It supports fast operations such as insertion, deletion, and search, essential for maintaining a dynamic library collection.
- Implement Binary Min-Heaps for managing book reservations: Each book maintains a Binary Min-Heap to manage reservations, ensuring efficient handling of patron requests based on priority and reservation timestamp.
These features collectively empower the GatorLibrary Management System to operate seamlessly, enhancing library efficiency and user satisfaction.
Key Components of the System
- Red-Black Tree: Central to the GatorLibrary Management System's architecture is the Red-Black Tree, a balanced binary search tree. This data structure efficiently organizes and manages the library's collection of books. Each node in the tree represents a book and holds essential attributes such as BookID, BookName, AuthorName, AvailabilityStatus, BorrowedBy, and a ReservationHeap. The Red-Black Tree ensures that operations like insertion, deletion, and search are performed in logarithmic time, maintaining optimal performance as the library's inventory evolves.
- Binary Min-Heaps: A critical component for handling book reservations, Binary Min-Heaps are utilized extensively in the system. Each book within the library maintains its own Binary Min-Heap, dedicated to managing patron reservations. These heaps prioritize reservations based on two main criteria: the patron’s priority level and the timestamp of the reservation. This prioritization ensures that when a book is unavailable for immediate borrowing, reservations are processed efficiently, granting patrons fair access to desired titles based on their priority status and the order of reservation.
These foundational components not only streamline the management of books and patron interactions but also uphold the system's efficiency and reliability. By leveraging the Red-Black Tree for structured book storage and employing Binary Min-Heaps for systematic reservation management, the GatorLibrary Management System exemplifies robust design principles aimed at enhancing user experience and operational effectiveness within a dynamic library environment.
Essential Operations
The GatorLibrary Management System facilitates several crucial operations to efficiently manage its resources and serve patrons effectively:
- PrintBook(bookID): This operation retrieves and displays detailed information about a specific book identified by its unique BookID. It ensures patrons can quickly ascertain the availability, borrower details, and reservation status of any book in the library's collection.
- PrintBooks(bookID1, bookID2): Enabling users to retrieve information about multiple books within a specified range, PrintBooks(bookID1, bookID2) ensures comprehensive access to details across a segment of the library's inventory. This operation supports efficient catalog browsing and management.
- InsertBook(bookID, bookName, authorName, availabilityStatus): Adding a new book to the library involves inserting its details into the Red-Black Tree. This operation ensures that each book is uniquely identified and properly categorized within the library's structured database, facilitating seamless management and retrieval.
- BorrowBook(patronID, bookID, patronPriority): Facilitating patron interactions, BorrowBook(patronID, bookID, patronPriority) allows users to borrow a book if available or make a reservation if the desired book is currently on loan. The system prioritizes reservations using Binary Min-Heaps, ensuring fair access based on patron priority and reservation timestamps.
- ReturnBook(patronID, bookID): When a patron returns a borrowed book, ReturnBook(patronID, bookID) updates the book's availability status and potentially assigns it to the next patron in the reservation queue. This operation optimizes resource utilization and ensures efficient circulation of library materials.
- DeleteBook(bookID): Removing a book from the library database, DeleteBook(bookID) triggers notifications to patrons on the reservation list, informing them of changes in availability. This operation maintains the integrity of the library's catalog and manages patron expectations effectively.
- FindClosestBook(targetID): Supporting quick access to books, FindClosestBook(targetID) identifies and prints details of the book closest in ID to the specified targetID. This operation enhances user convenience by facilitating rapid retrieval of relevant library resources.
- ColorFlipCount(): Essential for maintaining the integrity of the Red-Black Tree, ColorFlipCount() monitors and reports the frequency of color changes within the tree's nodes. This analytical tool aids in optimizing the performance of tree operations and ensuring the system's stability over time.
These operations collectively empower the GatorLibrary Management System to deliver efficient and user-friendly library services, enhancing both operational efficiency and patron satisfaction.
Setting Up the Project
1. Project Structure
Organizing the GatorLibrary project involves creating a structured directory layout. The GatorLibrary/ directory contains essential subdirectories and files:
- src/: This directory hosts the Java source code files, including Main.java for program entry, Library.java for library management logic, Book.java for defining book attributes, RedBlackTree.java for implementing the Red-Black Tree data structure, and MinHeap.java for managing reservations using Binary Min-Heaps.
- input/: Contains input test files (test1.txt), facilitating automated testing and validation of system functionalities.
- output/: Reserved for storing output files generated during program execution.
- Makefile: A script defining compilation rules for building the project, ensuring ease of compilation and execution across different environments.
- report.pdf: Documentation providing detailed project information, function prototypes, and explanations, essential for project submission and review.
Create a project directory with the following structure:
GatorLibrary/
│-- src/
│ │-- Main.java
│ │-- Library.java
│ │-- Book.java
│ │-- RedBlackTree.java
│ │-- MinHeap.java
│-- input/
│ │-- test1.txt
│-- output/
│-- Makefile
│-- report.pdf
2. Implementing the Red-Black Tree
In RedBlackTree.java, the Red-Black Tree implementation forms a core component of the GatorLibrary system. Each node within the tree represents a book and includes attributes such as bookID, bookName, authorName, isAvailable status, borrowedBy patron ID, and a reservationHeap managed by a Binary Min-Heap. The Node class initializes with these attributes and handles node color and pointers (left, right, parent). Methods for insertion, deletion, and balancing ensure efficient management of book records within the tree, supporting operations critical to maintaining library catalog integrity and efficient resource utilization.
public class RedBlackTree {
private class Node {
int bookID;
String bookName;
String authorName;
boolean isAvailable;
int borrowedBy;
MinHeap reservationHeap;
// Node color and pointers
boolean color; // true for red, false for black
Node left, right, parent;
// Node constructor
public Node(int bookID, String bookName, String authorName) {
this.bookID = bookID;
this.bookName = bookName;
this.authorName = authorName;
this.isAvailable = true;
this.borrowedBy = -1;
this.reservationHeap = new MinHeap();
this.color = true; // new nodes are red
}
}
private Node root;
private Node TNULL;
// Initialize the Red-Black Tree with TNULL nodes
public RedBlackTree() {
TNULL = new Node(-1, "", "");
TNULL.color = false;
TNULL.left = null;
TNULL.right = null;
root = TNULL;
}
// Implement insertion, deletion, and balancing methods
// ...
}
By structuring the project systematically and implementing robust data structures like the Red-Black Tree, the GatorLibrary project ensures scalability, performance, and maintainability. This setup facilitates comprehensive library management functionalities, from book insertion and deletion to efficient reservation handling, enhancing both operational efficiency and user experience in library operations.
3. Implementing the Min-Heap
In MinHeap.java, the Binary Min-Heap is crucial for managing reservations efficiently within the GatorLibrary system. The MinHeap class initializes with an empty list (heap) to store Reservation objects, each representing a patron's reservation details including patronID, priority, and timestamp. Methods are implemented to insert new reservations, maintain heap properties, and manage patron priorities effectively. This data structure ensures that book reservations are handled in a structured manner, prioritizing access based on patron priority levels and reservation timestamps, thereby optimizing the library's resource allocation and patron satisfaction.
import java.util.ArrayList;
import java.util.List;
public class MinHeap {
private List<Reservation> heap;
public MinHeap() {
this.heap = new ArrayList<>();
}
private class Reservation {
int patronID;
int priority;
long timestamp;
public Reservation(int patronID, int priority, long timestamp) {
this.patronID = patronID;
this.priority = priority;
this.timestamp = timestamp;
}
}
// Methods to insert, remove, and maintain heap properties
// ...
}
4. Library Operations
Within Library.java, methods are implemented to execute fundamental library operations necessary for day-to-day management:
- insertBook(int bookID, String bookName, String authorName, boolean availabilityStatus): Facilitates the addition of new books to the library's catalog using the Red-Black Tree data structure.
- printBook(int bookID): Enables retrieval and display of detailed information about a specific book identified by its unique bookID.
- borrowBook(int patronID, int bookID, int patronPriority): Implements logic for patrons to borrow books, managing reservations using the Binary Min-Heap if the desired book is currently unavailable.
- returnBook(int patronID, int bookID): Handles the process of returning borrowed books and reassigning them to the next patron in line, if applicable. These operations ensure seamless interaction between patrons and the library system, enhancing operational efficiency and user experience.
public class Library {
private RedBlackTree books;
public Library() {
books = new RedBlackTree();
}
public void insertBook(int bookID, String bookName, String authorName, boolean availabilityStatus) {
books.insert(new Book(bookID, bookName, authorName, availabilityStatus));
}
public void printBook(int bookID) {
// Search and print book details
}
public void borrowBook(int patronID, int bookID, int patronPriority) {
// Implement borrowing logic
}
public void returnBook(int patronID, int bookID) {
// Implement return logic
}
// Additional methods for other operations
// ...
}
By implementing these components and operations systematically, the GatorLibrary system is equipped to handle diverse library management tasks effectively. The structured approach to data handling and operation execution ensures reliability, scalability, and optimal performance, meeting the demands of modern library management practices efficiently.
5. Main Class
In Main.java, the main class serves as the entry point for the GatorLibrary Management System, handling command-line arguments and input/output operations seamlessly. Upon execution, an instance of the Library class is instantiated to manage the core functionalities of the library system. The main method reads input commands from a specified text file (args[0]), processes each command to execute corresponding operations like book insertion, borrowing, returning, and querying, ensuring smooth interaction between users and the system. Exception handling ensures robustness by catching and printing any potential IO errors, maintaining the reliability of the program under various runtime conditions.
import java.io.*;
public class Main {
public static void main(String[] args) {
Library library = new Library();
try (BufferedReader br = new BufferedReader(new FileReader(args[0]))) {
String line;
while ((line = br.readLine()) != null) {
// Parse and execute commands
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
6. Compiling and Running the Project
To compile and run the GatorLibrary project effectively, a Makefile is created to automate the build process:
- Makefile: The Makefile defines two main targets:
- all: Compiles the Java source files (src/*.java) and stores the compiled .class files in the bin directory using the javac command.
- run: Executes the compiled program (Main class) using the java command with the classpath set to the bin directory and passes input from input/test1.txt, facilitating automated testing and validation of system functionalities. This setup streamlines the development and testing workflow, ensuring consistent and reliable execution of the GatorLibrary Management System across different environments.
all:
javac -d bin src/*.java
run:
java -cp bin Main input/test1.txt
By implementing the Main class and setting up a Makefile for compilation and execution, developers can efficiently manage and deploy the GatorLibrary project, ensuring adherence to best practices in software development and facilitating seamless integration into operational environments.
Conclusion
Developing a GatorLibrary Management System using Java requires a solid grasp of intricate data structures and algorithms. This guide equips you with the knowledge and step-by-step instructions to construct a resilient system capable of efficiently overseeing library operations. By implementing advanced concepts like Red-Black Trees for organized book management and Binary Min-Heaps for effective reservation handling, you'll enhance your understanding of Java programming while building practical skills in software development. Whether you're a student striving to comprehend these principles or seeking assistance with a programming assignment, this resource empowers you to create functional solutions for managing books, patrons, and borrowing processes. Embrace the challenge, explore the intricacies of Java programming, and embark on a journey towards mastering library management systems. Happy coding!