Claim Your Offer
New semester, new challenges—but don’t stress, we’ve got your back! Get expert programming assignment help and breeze through Python, Java, C++, and more with ease. For a limited time, enjoy 10% OFF on all programming assignments with the Spring Special Discount! Just use code SPRING10OFF at checkout! Why stress over deadlines when you can score high effortlessly? Grab this exclusive offer now and make this semester your best one yet!
We Accept
- Harnessing Multithreaded Parallelism with Modern Languages and Compilers
- Language Support for Multithreading
- Compiler Optimizations for Multithreaded Code
- Static Analysis Tools
- Leveraging Functional Programming for Implicit Parallelism
- Higher-Order Functions
- Lazy Evaluation (Non-Strictness)
- Polymorphism
- Explicit Parallel Programming and Managing Concurrency
- Thread Management
- Handling Nondeterminism
- Understanding Theoretical Foundations: Lambda Calculus and Beyond
- Lambda Calculus
- Term Rewriting Systems
- Operational Semantics
- Optimizing Multithreaded Code for Modern Architectures
- Static Analysis for Parallel Code
- Compiler Optimizations
- Targeting Specific Architectures
- Utilizing Static Analysis and Compiler Optimizations
- Tools for Static Analysis
- Techniques for Compiler Optimization
- Best Practices for Using Static Analysis and Compiler Optimizations
- Conclusion
In today’s digital age, the rapid advancement of technology and the increasing complexity of computational tasks have necessitated the use of multithreaded parallelism. This approach allows for the simultaneous execution of multiple threads or processes, significantly improving the efficiency and performance of software applications. For students, particularly those involved in programming assignments that require high-performance computing, understanding and applying the principles of clean code in the context of multithreaded parallelism is essential. Clean code is not just about aesthetics; it enhances readability, maintainability, and, most importantly, the overall performance of the software. This comprehensive guide explores the significance of clean code in multithreaded parallelism, focusing on languages and compilers, functional programming paradigms, explicit parallel programming techniques, and compiler optimizations. To solve your multithread assignment and complete your programming assignment, applying these principles effectively can make a significant difference in both the quality and efficiency of your code.
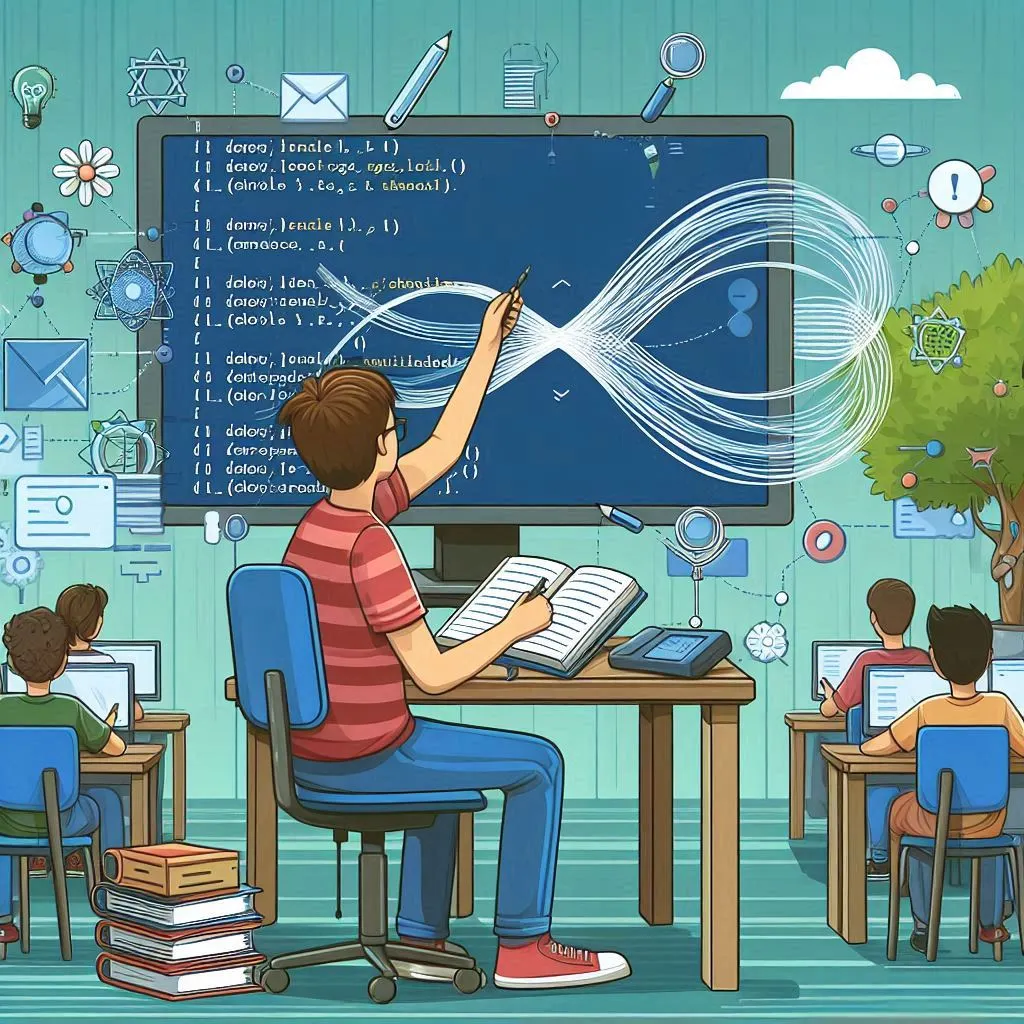
Harnessing Multithreaded Parallelism with Modern Languages and Compilers
Modern programming languages and compilers have evolved to support and optimize multithreaded parallelism. These advancements have made it easier for developers to write code that can effectively utilize multiple processors simultaneously, thereby enhancing the performance of their applications. This section delves into the roles of languages and compilers in exploiting multithreaded parallelism.
Language Support for Multithreading
Modern programming languages like C++, Java, and Python come with built-in support for multithreading. These languages provide constructs and libraries that enable developers to create and manage multiple threads effortlessly.
- C++: The C++ Standard Library includes the <thread> header, which offers a straightforward way to create and manage threads. Additionally, C++11 introduced several features that make multithreading easier, such as the std::async function for asynchronous execution and the std::mutex class for thread synchronization.
- Java: Java's concurrency framework, part of the java.util.concurrent package, provides robust support for multithreading. The Thread class, along with high-level concurrency utilities like ExecutorService and Future, simplifies the development of multithreaded applications.
- Python: Although Python's Global Interpreter Lock (GIL) can be a limitation for CPU-bound multithreaded programs, the threading and concurrent.futures modules offer easy-to-use abstractions for managing threads and asynchronous tasks. For CPU-bound tasks, Python developers often turn to multiprocessing or use C extensions to bypass the GIL.
Compiler Optimizations for Multithreaded Code
Compilers play a crucial role in optimizing multithreaded code to ensure efficient execution on modern hardware. These optimizations include automatic parallelization, vectorization, and other techniques that enhance the performance of multithreaded applications.
- Automatic Parallelization: Some compilers can automatically parallelize loops and other constructs, reducing the need for manual intervention by the programmer. This feature analyzes the code to identify parallelizable sections and transforms them to run concurrently.
- Vectorization: Vectorization is the process of converting scalar operations to vector operations, which can be executed simultaneously by modern CPUs. Compilers like GCC and Intel's ICC provide vectorization options that can significantly boost performance.
- Memory Management: Effective memory management is crucial for multithreaded applications. Compilers optimize memory access patterns to minimize cache misses and memory contention, ensuring smooth execution of concurrent threads.
Static Analysis Tools
Static analysis tools are invaluable for identifying potential issues in multithreaded code without executing it. These tools analyze the code to detect race conditions, deadlocks, and other concurrency-related problems.
- Race Conditions: Tools like ThreadSanitizer and Helgrind can detect race conditions, which occur when multiple threads access shared data concurrently without proper synchronization.
- Deadlocks: Static analysis can identify potential deadlocks by analyzing lock acquisition patterns. Tools like FindBugs for Java and Clang Static Analyzer for C++ help in detecting these issues early in the development process.
- Concurrency Bugs: Concurrency bugs, such as atomicity violations and order violations, can be challenging to diagnose. Static analysis tools provide insights into these issues, enabling developers to write cleaner and more reliable multithreaded code.
Leveraging Functional Programming for Implicit Parallelism
Functional programming paradigms offer powerful abstractions for implicit parallelism, allowing developers to write parallel code without explicitly managing threads. This section explores the key features of functional programming that facilitate clean and efficient parallel code.
Higher-Order Functions
Higher-order functions are functions that can take other functions as arguments or return them as results. They are a fundamental concept in functional programming and play a crucial role in enabling implicit parallelism.
- Map and Reduce: Functions like map and reduce are common higher-order functions in functional programming. The map function applies a given function to each element of a list, and reduce combines the results. These functions can be easily parallelized, as each operation is independent of the others.
- Function Composition: Higher-order functions enable function composition, where smaller functions are combined to form more complex operations. This modularity simplifies parallelization and enhances code readability.
Lazy Evaluation (Non-Strictness)
Lazy evaluation, also known as non-strict evaluation, is a feature of functional programming where expressions are not evaluated until their values are needed. This approach optimizes resource usage and facilitates parallel execution.
- Deferred Computation: By deferring computation, lazy evaluation allows the system to manage execution order more efficiently. This can lead to significant performance improvements in parallel applications.
- Memory Efficiency: Lazy evaluation can reduce memory usage by avoiding the creation of intermediate data structures. This is particularly beneficial in parallel programs, where memory contention can be a bottleneck.
Polymorphism
Polymorphism in functional programming allows functions to operate on different data types, enhancing code flexibility and reusability. This feature supports the creation of generic functions that can be parallelized easily.
- Generic Functions: Polymorphic functions can be applied to a wide range of data types, making them highly reusable. This reduces code duplication and promotes clean coding practices.
- Type Inference: Many functional programming languages support type inference, where the compiler automatically deduces the types of expressions. This simplifies the code and makes it more readable, which is crucial for maintaining parallel applications.
Explicit Parallel Programming and Managing Concurrency
While implicit parallelism in functional programming simplifies the development process, explicit parallel programming provides fine-grained control over parallel execution. This section discusses the techniques and challenges associated with explicit parallel programming.
Thread Management
Explicit parallel programming involves directly managing threads, including their creation, synchronization, and termination. This approach provides greater control but requires careful handling to avoid concurrency issues.
- Thread Creation and Synchronization: In explicit parallel programming, developers are responsible for creating and managing threads. This includes using synchronization mechanisms like mutexes and locks to ensure data consistency. Languages like C++ and Java provide built-in support for these constructs.
- Thread Pools: Thread pools are a common technique to manage multiple threads efficiently. A thread pool maintains a pool of worker threads that can be reused, reducing the overhead of thread creation and destruction.
Handling Nondeterminism
Nondeterminism in parallel programming refers to the unpredictable order of thread execution, which can lead to race conditions and other concurrency issues. Managing nondeterminism is crucial for writing clean and reliable parallel code.
- Race Conditions: Race conditions occur when multiple threads access shared data concurrently without proper synchronization. These issues can lead to inconsistent results and are often challenging to debug.
- Deadlocks: Deadlocks arise when two or more threads are waiting for each other to release resources, resulting in a standstill. Detecting and preventing deadlocks requires careful design and testing.
Understanding Theoretical Foundations: Lambda Calculus and Beyond
Theoretical foundations like the lambda calculus provide a formal framework for understanding functional programming and parallelism. This section explores the significance of lambda calculus and its variants in parallel programming.
Lambda Calculus
Lambda calculus is a formal system for expressing computation based on function abstraction and application. It serves as the theoretical foundation for many functional programming languages.
- Function Abstraction: Lambda calculus allows the representation of functions and their applications in a concise and formal manner. This abstraction simplifies reasoning about parallel computations.
- Variable Binding and Scope: Understanding variable binding and scope in lambda calculus helps in grasping the concepts of closures and higher-order functions, which are essential for parallel programming.
Term Rewriting Systems
Term rewriting systems involve transforming expressions based on predefined rules. These systems provide a formal method for modeling and analyzing parallel computations.
- Rewrite Rules: Rewrite rules define how expressions can be transformed. In parallel programming, these rules can model the concurrent execution of operations.
- Confluence and Termination: Analyzing the confluence and termination properties of term rewriting systems ensures that parallel computations are both correct and efficient.
Operational Semantics
Operational semantics describe how programs execute step by step, providing a framework for reasoning about program behavior. This formalism is crucial for analyzing the correctness and performance of parallel programs.
- Small-Step Semantics: Small-step semantics describe the execution of programs in small, incremental steps. This approach is useful for understanding the fine-grained behavior of parallel programs.
- Big-Step Semantics: Big-step semantics provide a higher-level view of program execution, focusing on the overall result rather than individual steps. This perspective helps in reasoning about the correctness of parallel algorithms.
Optimizing Multithreaded Code for Modern Architectures
Compiling multithreaded code for symmetric multiprocessors (SMP) and clusters involves optimizing the code to run efficiently on multiple processors or nodes. This section explores the techniques and tools used to achieve these optimizations.
Static Analysis for Parallel Code
Static analysis tools analyze code without executing it to identify potential issues and optimization opportunities. These tools are essential for developing efficient multithreaded applications.
- Detecting Concurrency Issues: Static analysis tools can identify race conditions, dead locks, and other concurrency issues early in the development process. This preemptive identification allows developers to address problems before they manifest in runtime errors, leading to cleaner and more reliable parallel code.
- Performance Analysis: Static analysis can also highlight performance bottlenecks by analyzing code paths and resource utilization. Tools like Intel VTune and the Clang Static Analyzer provide insights into how different parts of the code perform, allowing developers to optimize critical sections for better parallel execution.
Compiler Optimizations
Compiler optimizations are crucial for enhancing the performance of multithreaded applications. These optimizations transform the source code to run more efficiently on target architectures, such as symmetric multiprocessors and clusters.
- Loop Transformations: Techniques like loop unrolling and loop fusion help optimize repetitive computations by minimizing overhead and improving cache performance. These transformations enable more effective parallelization of loops.
- Automatic Parallelization: Some compilers can automatically parallelize code sections, particularly loops, by analyzing data dependencies and determining safe parallel execution paths. This reduces the programmer's burden and ensures that parallelism is correctly implemented.
- Memory Access Optimization: Optimizing memory access patterns is vital for multithreaded performance. Techniques such as data locality improvement and cache-friendly data structures minimize cache misses and memory contention, enhancing the efficiency of parallel applications.
Targeting Specific Architectures
Optimizing code for specific architectures, such as symmetric multiprocessors (SMP) and clusters, involves tailoring the compilation process to leverage the unique features of these systems.
- Symmetric Multiprocessors (SMP): SMP systems have multiple processors sharing a single memory space. Compiler optimizations for SMP focus on efficient thread synchronization and minimizing contention for shared resources. Techniques such as lock elision and hardware transactional memory are employed to optimize synchronization.
- Clusters: Clusters consist of multiple nodes, each with its own memory, connected by a network. Optimizing code for clusters involves minimizing communication overhead and efficiently distributing computations across nodes. Compilers use techniques like message passing interface (MPI) optimizations and data distribution strategies to achieve this.
Utilizing Static Analysis and Compiler Optimizations
Static analysis and compiler optimizations work hand in hand to produce efficient and reliable multithreaded code. This section delves into the practical aspects of using these tools and techniques.
Tools for Static Analysis
Static analysis tools provide automated checks and insights that are invaluable for developing multithreaded applications. Some widely used tools include:
- ThreadSanitizer: An open-source tool that detects data races in C/C++ programs. It helps identify and fix race conditions that can lead to unpredictable behavior in multithreaded applications.
- Helgrind: Part of the Valgrind suite, Helgrind is a tool for detecting data races and other threading issues in programs. It provides detailed reports that help developers understand and resolve concurrency problems.
- FindBugs: A static analysis tool for Java that identifies potential bugs, including concurrency issues like deadlocks and atomicity violations. It integrates with development environments to provide continuous feedback during the coding process.
Techniques for Compiler Optimization
Compiler optimizations involve a range of techniques aimed at improving the performance and efficiency of multithreaded code. Key techniques include:
- Inlining: The compiler replaces a function call with the actual function code. This reduces function call overhead and can lead to better optimization opportunities within the inlined code.
- Dead Code Elimination: The compiler removes code that does not affect the program's output. This streamlines the code and can reduce the computational load, particularly in complex multithreaded applications.
- Parallel Loop Transformations: Compilers analyze loops to identify opportunities for parallel execution. Techniques like loop splitting, tiling, and parallel pipelining are used to maximize parallelism while maintaining data dependencies.
Best Practices for Using Static Analysis and Compiler Optimizations
To maximize the benefits of static analysis and compiler optimizations, developers should follow these best practices:
- Regular Analysis: Integrate static analysis into the development workflow to continuously identify and address issues. This proactive approach ensures that potential problems are caught early.
- Optimization Flags: Use compiler optimization flags (e.g., -O2, -O3 in GCC) to enable various optimization levels. Experiment with different flags to find the optimal balance between performance and code complexity.
- Profile-Guided Optimization (PGO): Use profiling tools to collect runtime data and guide the optimization process. PGO allows the compiler to make more informed decisions based on actual execution patterns.
- Benchmarking and Testing: Regularly benchmark and test the optimized code to ensure that performance improvements do not introduce new issues. Use testing frameworks and performance analysis tools to validate the results.
Conclusion
Writing clean code in the context of multithreaded parallelism is a critical skill for students and developers tackling complex programming assignments. By leveraging the features of modern programming languages, understanding functional programming paradigms, and utilizing advanced compiler optimizations, students can create efficient, maintainable, and reliable parallel applications. Mastering these best practices not only enhances code quality but also prepares students for the demands of modern software development, where parallelism is increasingly prevalent.