Claim Your Discount Today
Kick off the fall semester with a 20% discount on all programming assignments at www.programminghomeworkhelp.com! Our experts are here to support your coding journey with top-quality assistance. Seize this seasonal offer to enhance your programming skills and achieve academic success. Act now and save!
We Accept
- Understanding the Assignment Requirements
- Analyzing the Problem
- Defining Class Responsibilities
- Creating Class Diagrams
- Writing Pseudocode
- Implementing the Classes
- Implementing the Account Class
- Implementing the Accounts Class
- Implementing the AccountSystem Class
- Testing and Debugging
- Testing Individual Components
- Debugging Techniques
- Conclusion
Programming assignments involving complex class designs, such as a bank account system, are an excellent way to strengthen your understanding of object-oriented programming and dynamic memory management in C++. These assignments challenge you to create well-structured, efficient, and maintainable code. In this guide, we'll explore a systematic approach to tackling such assignments, covering everything from initial planning to final implementation and testing. By following these steps, you’ll enhance your skills in solving C++ assignments and develop robust, high-quality code.
Understanding the Assignment Requirements
Before you start coding, it's crucial to fully grasp the requirements of your assignment. This understanding will guide your design decisions and ensure that your implementation meets the specified criteria.
Analyzing the Problem
The first step in any programming assignment is to analyze the problem statement carefully. In the context of a bank account system, you might be tasked with implementing several classes, each with specific responsibilities and interactions. Here's how you can break down the requirements:
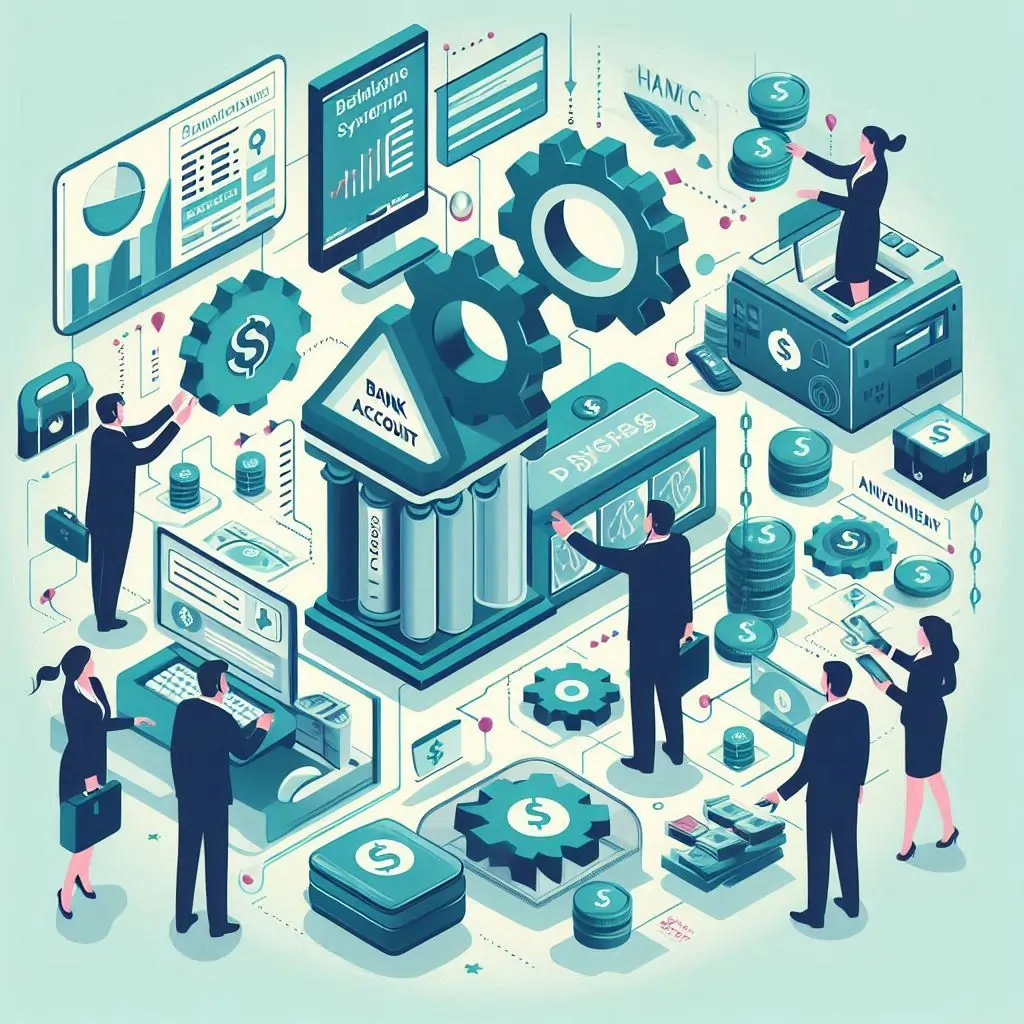
- Account Class: This class typically manages the details and operations of an individual bank account. You'll need to implement methods for depositing, withdrawing, and transferring funds, as well as constructors, destructors, and operator overloads.
- Accounts Class: This container class holds multiple Account objects. Your task will be to manage a dynamic array of accounts, providing functionality to add, find, and access accounts without using STL containers.
- AccountSystem Class: This class acts as an interface for users to interact with the bank account system. It should support operations like adding accounts, performing transactions, and printing account details.
Defining Class Responsibilities
Clearly defining the responsibilities of each class is crucial for a successful implementation. This involves deciding which data members and methods belong to each class and how they interact with each other.
Account Class
The Account class is responsible for managing individual account data and operations. Key considerations include:
- Data Members: Define private members for account ID, customer name, and balance. You might also include pointers for dynamic memory management.
- Methods: Implement methods for depositing and withdrawing funds, accessing private data, and overloading operators to support complex operations like fund transfers.
Accounts Class
The Accounts class serves as a container for managing multiple Account objects. Important aspects include:
- Data Members: Use a dynamic array to store accounts, along with variables to track the array's size and maximum capacity.
- Methods: Provide methods for adding accounts, finding accounts by ID, and accessing accounts by index. Avoid using STL containers to meet assignment constraints.
AccountSystem Class
The AccountSystem class provides a user-friendly interface for interacting with the account system. Key responsibilities include:
- Methods: Implement operations for adding new accounts, depositing, withdrawing, transferring funds, and printing account details.
Creating Class Diagrams
Visualizing your design through class diagrams helps ensure clarity and coherence. These diagrams illustrate the relationships between classes and highlight key attributes and methods.
Overall System Diagram
Start with a high-level diagram showing the relationships between the Account, Accounts, and AccountSystem classes. Use arrows to indicate dependencies and interactions.
Detailed Class Diagrams
Create individual diagrams for each class, detailing attributes and methods. This level of detail helps guide your implementation and ensures you capture all necessary functionality.
Writing Pseudocode
Pseudocode is a powerful tool for planning your implementation. By writing out the logic and flow of your program in plain language, you can clarify your ideas and identify potential issues before you start coding.
Account Class Pseudocode
Consider writing pseudocode for the key methods in the Account class, excluding simple getters and setters. For example:
Deposit Method Pseudocode:
Method Deposit(amount)
If amount < 0
Print "Invalid deposit amount"
Else
Increase balance by amount
Print "Deposit successful: new balance is", balance
Accounts Class Pseudocode
For the Accounts class, focus on methods for managing the dynamic array of accounts:
Add Account Method Pseudocode:
Method AddAccount(newAccount)
If size >= maximum size
Print "Cannot add more accounts"
Else
Add newAccount to array
Increase size by 1
Print "Account added successfully"
AccountSystem Class Pseudocode
The AccountSystem class handles user interactions and system operations. Plan out the logic for key methods:
Add Account Operation Pseudocode:
Method AddAccount()
Prompt user for account details
If account ID exists
Print "Account ID already exists"
Else
Create new Account object
Add account to Accounts container
Print "Account created successfully"
Implementing the Classes
With a solid plan in place, you can begin coding your classes. This section provides tips and best practices for implementing each component of the system.
Implementing the Account Class
The Account class forms the backbone of the bank account system. Pay close attention to its implementation to ensure accuracy and efficiency.
Data Members and Constructors
- Private Data Members: Define members for account ID, customer name, and balance. Use pointers for dynamic memory if necessary.
- Constructors: Implement a default constructor, copy constructor, and destructor to manage resource allocation and deallocation.
Public Methods
- Deposit and Withdraw: Implement these methods to modify the account balance, ensuring that inputs are validated and transactions are logged.
- Operator Overloading: Overload operators, such as +=, to enable intuitive operations like transferring funds between accounts.
Implementing the Accounts Class
The Accounts class requires careful management of dynamic memory, as it handles a collection of Account objects.
Data Members and Constructors
- Dynamic Array: Use a pointer to a dynamic array for storing accounts. Track the current size and maximum capacity with integer variables.
- Constructors: Implement a constructor to allocate memory for the array and a destructor to deallocate it, preventing memory leaks.
Public Methods
- Add Account: Implement a method to add new accounts to the array, checking that there is sufficient space before insertion.
- Find and Access Accounts: Provide methods to locate accounts by ID and access accounts by index, returning references where appropriate.
Implementing the AccountSystem Class
The AccountSystem class is the interface between users and the account system. It should handle user inputs and execute corresponding operations.
User Interaction and Menu System
- Display Menu: Implement a loop to repeatedly display a menu of options, allowing users to perform transactions or exit the system.
- Validate Input: Ensure that user inputs are valid, displaying error messages for invalid menu selections or transaction parameters.
System Operations
- Add, Deposit, Withdraw, Transfer: Implement these operations by calling methods on the Accounts container, ensuring that each action is validated and logged.
- Print Accounts: Provide a method to display all accounts in a formatted manner, including details like account ID, name, and balance.
Testing and Debugging
Thorough testing and debugging are crucial for ensuring that your program functions as expected and meets all requirements.
Testing Individual Components
Testing each class and method independently helps isolate issues and verify that each component behaves correctly.
Account Class Testing
- Deposit and Withdraw: Test these methods with valid and invalid inputs, checking that balances are updated and errors are handled gracefully.
- Operator Overloading: Verify that overloaded operators perform the expected operations, especially in complex scenarios like fund transfers.
Accounts Class Testing
- Add Account: Test the addition of accounts to the dynamic array, ensuring that capacity limits are respected and accounts are stored correctly.
- Find and Access Accounts: Verify that accounts can be found and accessed by ID and index, handling edge cases like non-existent accounts.
AccountSystem Class Testing
- User Operations: Test each user operation through the menu system, checking that inputs are validated, and transactions are processed correctly.
- Menu Navigation: Ensure that the menu system handles invalid selections and exits gracefully.
Debugging Techniques
- Use Debuggers: Utilize debugging tools to step through your code and identify logical errors or unexpected behavior.
- Add Logging: Insert print statements or logging calls to trace the flow of your program and identify where issues occur.
- Use Assertions: Add assert statements to check that assumptions hold at runtime, helping catch unexpected conditions early.
Conclusion
Successfully completing your programming assignment, like designing a bank account system, requires a methodical approach and attention to detail. By understanding the requirements, planning your implementation, and thoroughly testing your code, you can develop robust applications that meet specifications and demonstrate your proficiency in C++ programming. Remember, practice and perseverance are key to mastering these concepts, so continue to challenge yourself with new projects and learning opportunities.