Claim Your Discount Today
Take your coding game to new heights with expert help at unbeatable prices. Got a tricky project or a tight deadline? We’ve got you covered! Use code PHHBF10 at checkout and save BIG. Don’t wait—this exclusive Black Friday deal won’t last long. Secure your academic success today!
We Accept
- Understanding the Game Mechanics
- 1. Define the Game Board and Pieces
- 2. Actions and Rules
- 3. Game End Conditions
- Programming Strategy
- 1. Setup the Game State
- 2. Implement Movement Logic
- 3. Implement Boom Actions
- 4. Game End Conditions
- Testing and Debugging
- 1. Unit Tests
- 2. Integration Tests
- 3. Edge Cases
- Conclusion
Designing and implementing board game simulations can be an intricate yet fascinating challenge. These game design assignments often require a deep understanding of game mechanics, strategic rules, and precise coding techniques to create a functional and engaging game. This guide explores a comprehensive approach to tackling such programming tasks, focusing on a strategic board game where players manipulate pieces on a grid, execute moves, and trigger explosive chain reactions.
By examining the essential aspects of game mechanics, movement rules, and game state management, we will break down the process of coding a board game simulation. Whether you’re dealing with a classic game or a novel creation, this guide will provide you with the tools and strategies needed to successfully navigate and implement these complex computer science assignments.
Understanding the Game Mechanics
1. Define the Game Board and Pieces
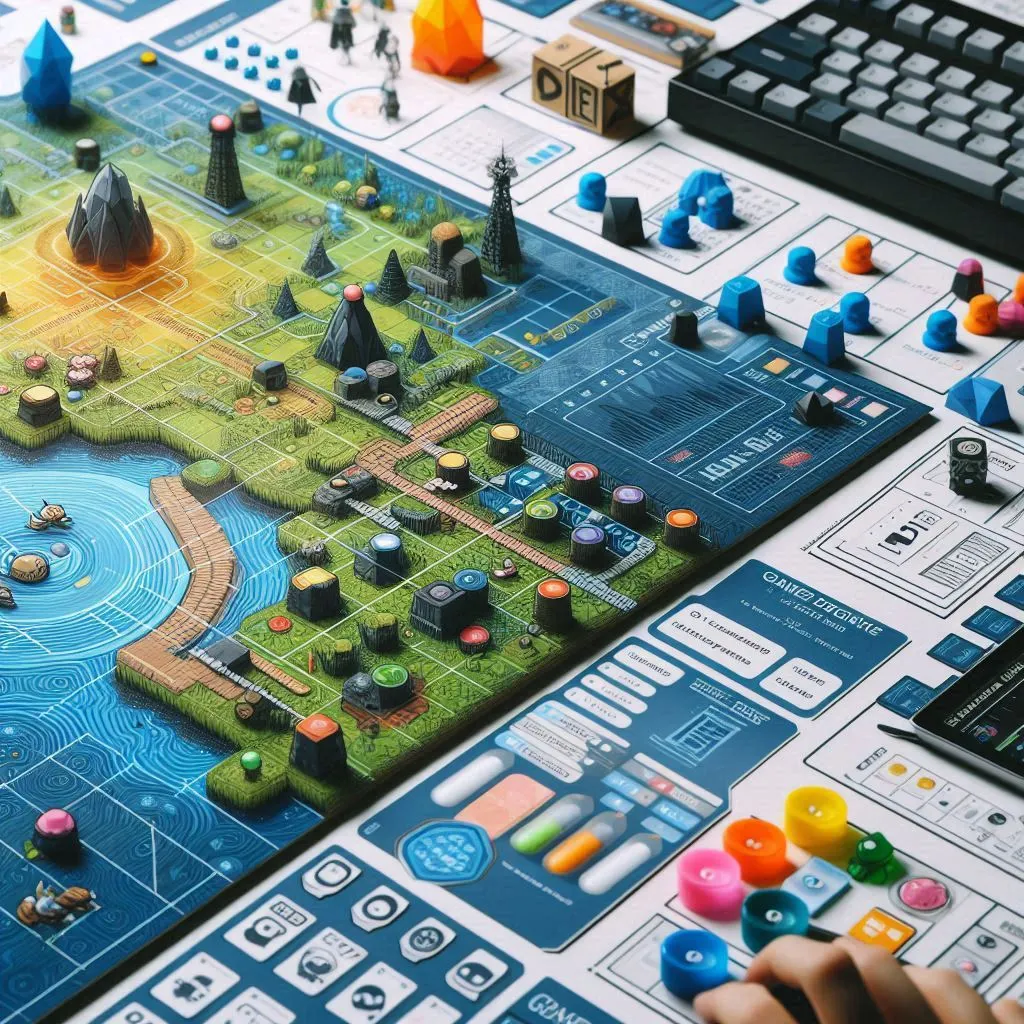
a. Board Setup
Start by defining the dimensions and initial setup of the board. For instance, an 8x8 board is a common choice for many strategy games. Here’s how you can approach this:
- Dimensions: Create a data structure to represent the board. For an 8x8 board, you can use a two-dimensional array (matrix) where each element represents a square on the board.
- Initial Configuration: Populate the board with the initial arrangement of pieces. In your example, pieces start in specific rows and columns, so you’ll need to define these positions in your initialization code.
b. Pieces and Stacks
- Piece Representation: Define classes or structures to represent the pieces and their stacks. Each piece might have attributes such as color, stack size, and position.
- Stacks: Implement logic to handle stacks of pieces. A stack can be represented by a single piece with a stack size attribute or a more complex data structure depending on the game’s requirements.
Example:
class Piece:
def __init__(self, color, stack_size=1):
self.color = color
self.stack_size = stack_size
class Board:
def __init__(self):
self.grid = [[None for _ in range(8)] for _ in range(8)]
2. Actions and Rules
a. Move Actions
- Movement Rules: Define how pieces move on the board. Consider the allowed directions (up, down, left, right) and any restrictions based on stack size and board boundaries.
- Move Function: Implement a function that takes the start position, direction, and distance as inputs and updates the board state accordingly.
Example Move Function:
def move_piece(self, start_position, direction, distance):
# Example of a move function
x, y = start_position
if direction == 'up':
new_x, new_y = x - distance, y
elif direction == 'down':
new_x, new_y = x + distance, y
elif direction == 'left':
new_x, new_y = x, y - distance
elif direction == 'right':
new_x, new_y = x, y + distance
# Check boundaries and move piece
if 0 <= new_x < 8 and 0 <= new_y < 8:
# Update board with new position
pass
b. Boom Actions
- Explosion Mechanics: Define how a boom or explosion affects the board. Typically, this involves removing a stack and triggering explosions in adjacent squares.
- Chain Reactions: Implement recursive or iterative functions to handle chain reactions resulting from a single explosion.
Example Boom Function:
Python code
def boom_action(self, position):
# Trigger explosion at the given position
x, y = position
# Remove the stack at position
# Trigger explosions in the 3x3 area
for i in range(-1, 2):
for j in range(-1, 2):
nx, ny = x + i, y + j
if 0 <= nx < 8 and 0 <= ny < 8:
# Check if there is a stack and trigger explosion
pass
3. Game End Conditions
- Win/Loss Conditions: Define the criteria for winning or losing the game. Typically, a game ends when a player has no remaining pieces.
- Draw Conditions: Implement conditions for a draw, such as a repeated board configuration or a maximum number of turns.
Example Game End Checks:
def check_win(self):
# Check if a player has won
pass
def check_draw(self):
# Check for draw conditions
pass
Programming Strategy
1. Setup the Game State
- Initialize the Board: Write code to set up the board with the initial configuration. Ensure that all pieces are placed in their correct starting positions.
- Define the Pieces: Implement the classes or structures to represent different types of pieces, including their attributes and behaviors.
Example Initialization:
def initialize_board():
board = Board()
# Place pieces in initial positions
for row in range(2):
for col in range(8):
board.grid[row][col] = Piece('White')
board.grid[7 - row][col] = Piece('Black')
return board
2. Implement Movement Logic
- Move Function: Ensure that your move function handles all possible cases, including moving over other pieces and not moving off the board.
- Boundary Checks: Implement checks to ensure that pieces do not move outside the board or into invalid positions.
Example Move Function with Boundary Checks:
def move_piece(self, start_position, direction, distance):
x, y = start_position
if direction == 'up':
new_x, new_y = x - distance, y
elif direction == 'down':
new_x, new_y = x + distance, y
elif direction == 'left':
new_x, new_y = x, y - distance
elif direction == 'right':
new_x, new_y = x, y + distance
# Check if move is within boundaries
if 0 <= new_x < 8 and 0 <= new_y < 8:
# Check if destination is valid
if self.grid[new_x][new_y] is None or self.grid[new_x][new_y].color == self.grid[x][y].color:
# Perform the move
pass
3. Implement Boom Actions
- Explosion Function: Write the function to handle the explosion of a stack. Ensure it triggers explosions in adjacent squares and handles chain reactions.
- Chain Reactions: Use recursion or iterative methods to handle multiple explosions triggered by a single action.
Example Explosion Function with Chain Reactions:
def boom_action(self, position):
x, y = position
if self.grid[x][y] is not None:
self.grid[x][y] = None # Remove the stack at position
# Trigger explosions in the 3x3 area
for i in range(-1, 2):
for j in range(-1, 2):
nx, ny = x + i, y + j
if 0 <= nx < 8 and 0 <= ny < 8:
# Check if there is a stack and trigger explosion
self.explode(nx, ny)
def explode(self, x, y):
# Implement the recursive explosion logic here
pass
4. Game End Conditions
- Win/Loss Checks: Implement functions to determine if a player has won or lost based on the remaining pieces on the board.
- Draw Checks: Implement checks to handle draw conditions, including detecting repeated board states or exceeding turn limits.
Example Win/Loss Check:
def check_win(self):
white_pieces = any(piece.color == 'White' for row in self.grid for piece in row)
black_pieces = any(piece.color == 'Black' for row in self.grid for piece in row)
if not white_pieces:
return 'Black Wins'
elif not black_pieces:
return 'White Wins'
return None
Testing and Debugging
1. Unit Tests
- Test Functions Individually: Write unit tests for each function to ensure they work as expected. For example, test movement functions with various input scenarios to validate their correctness.
- Edge Cases: Test edge cases such as moving pieces at the edges of the board, handling maximum stack sizes, and triggering explosions at the board’s corners.
Example Unit Test:
def test_move_piece():
board = initialize_board()
board.move_piece((1, 1), 'up', 2)
assert board.grid[0][1] is not None # Check new position
2. Integration Tests
- Test the Entire Game: Run integration tests to ensure that all components of the game work together seamlessly. This includes testing complete game scenarios from setup to win/loss conditions.
Example Integration Test:
def test_game_flow():
board = initialize_board()
board.move_piece((1, 1), 'up', 2)
board.boom_action((0, 1))
assert board.check_win() is None # Check if game flow is correct
3. Edge Cases
- Boundary Conditions: Test scenarios where pieces move to or from the edges of the board. Ensure that your code handles these cases without errors.
- Maximum Stack Sizes: Test cases where stacks reach maximum allowed sizes. Ensure that the game logic handles large stacks correctly.
Example Edge Case Test:
def test_max_stack_size():
board = initialize_board()
# Simulate adding tokens to a stack until the maximum size
pass
Conclusion
Tackling programming assignments for board games involves a detailed understanding of the game mechanics, implementing functions to handle game actions, and thorough testing to ensure correctness. By breaking down the problem into manageable components, such as initializing the board, implementing movement and explosion mechanics, and checking game end conditions, you can solve your python assignments methodically.
Understanding the rules of the game, developing robust functions to handle various scenarios, and testing thoroughly will help you create effective solutions for board game programming assignments. With practice and attention to detail, you’ll be well-equipped to handle similar assignments and excel in programming challenges.