Claim Your Discount Today
Ring in Christmas and New Year with a special treat from www.programminghomeworkhelp.com! Get 15% off on all programming assignments when you use the code PHHCNY15 for expert assistance. Don’t miss this festive offer—available for a limited time. Start your New Year with academic success and savings. Act now and save!
We Accept
- Introduction to the Problem
- Understanding Object-Oriented Concepts
- Designing the Classes
- Base Class: Account
- Derived Class: AccountChecking
- Derived Class: AccountSaving
- Implementing the Container Class
- Main Program Logic
- Conclusion and Key Takeaways
Object-oriented programming (OOP) is essential for any aspiring software developer, especially when it comes to designing complex systems like a banking application. This blog post provides valuable assistance with computer science assignments by delving into the process of enhancing a bank account management system using C++. By introducing concepts like inheritance, polymosrphism, and efficient data handling, we'll explore how to create a versatile system that can handle multiple types of bank accounts with unique behaviors. Whether you're looking to improve your coding skills or seeking guidance on a challenging project, this guide offers a clear roadmap to successfully implement and extend a dynamic banking application.
Introduction to the Problem
The assignment requires extending a bank account system using object-oriented programming (OOP) concepts in C++. Specifically, you'll be implementing inheritance, polymorphism, and file handling by creating two derived classes, AccountChecking and AccountSaving, which inherit from a base class Account. The assignment involves overriding methods, handling transactions with specific rules, and managing account data through file operations.
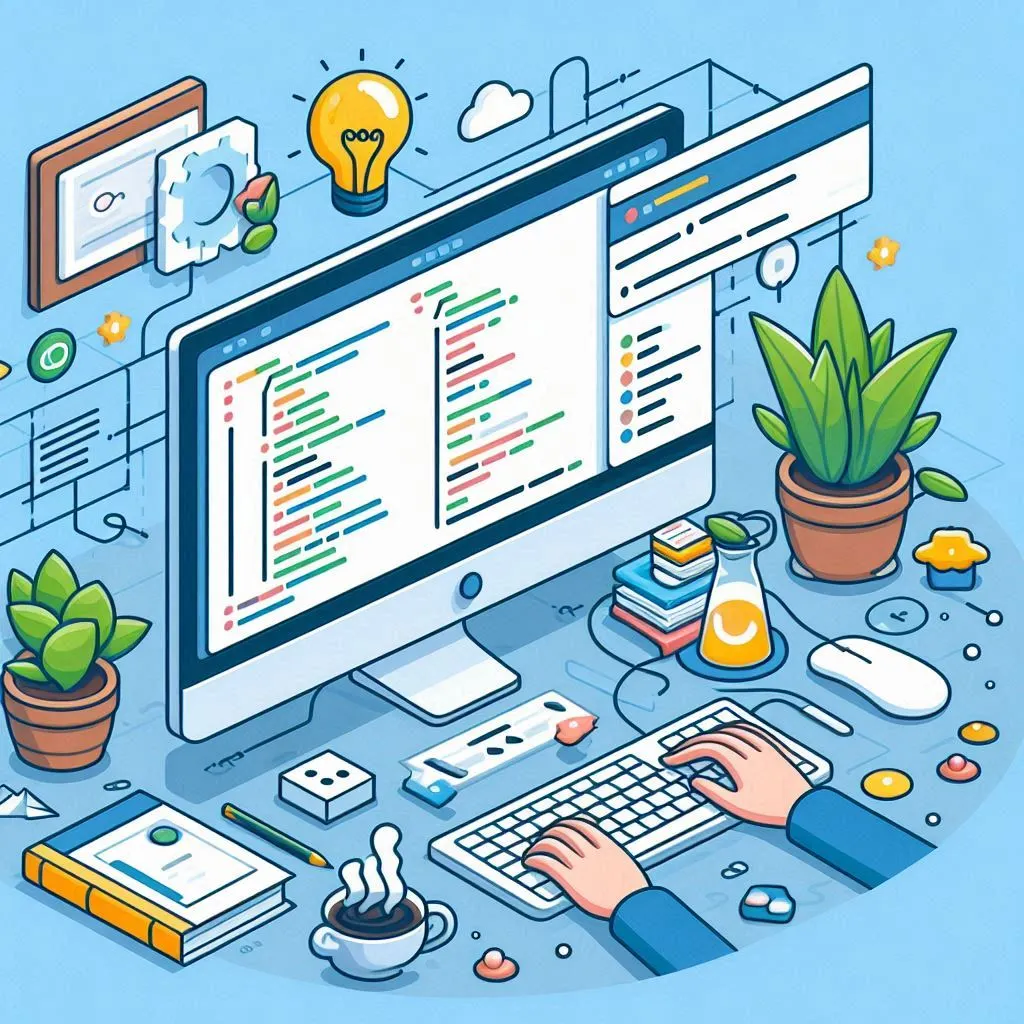
Understanding Object-Oriented Concepts
Before diving into the code, it's important to understand some fundamental OOP concepts:
- Inheritance: Allows a class (derived class) to inherit attributes and methods from another class (base class). This promotes code reuse.
- Polymorphism: Refers to the ability to process objects differently based on their data type or class. In C++, this is often achieved using virtual functions.
- Encapsulation: The bundling of data with methods that operate on that data, restricting direct access to some components.
- Abstraction: Hiding the complex implementation details and showing only the necessary features of an object.
Designing the Classes
Base Class: Account
The Account class is the base class from which AccountChecking and AccountSaving will inherit. It will contain common attributes like accountID, balance, and customerName, and methods like deposit, withdraw, and operator+=.
// Account.h
#pragma once
#include <string>
#include <iostream>
class Account {
protected:
int accountID;
float balance;
std::string customerName;
public:
Account(int id, float bal, std::string name)
: accountID(id), balance(bal), customerName(name) {}
virtual ~Account() {}
virtual void deposit(float amount) {
balance += amount;
std::cout << "Deposited " << amount << ". New balance: " << balance << std::endl;
}
virtual void withdraw(float amount) {
if (amount > balance) {
std::cerr << "Insufficient balance!" << std::endl;
} else {
balance -= amount;
std::cout << "Withdrew " << amount << ". New balance: " << balance << std::endl;
}
}
virtual Account& operator+=(Account& other) {
this->deposit(other.balance);
other.balance = 0;
return *this;
}
virtual void display() const {
std::cout << "Account ID: " << accountID << ", Name: " << customerName
<< ", Balance: " << balance << std::endl;
}
int getAccountID() const { return accountID; }
float getBalance() const { return balance; }
std::string getCustomerName() const { return customerName; }
};
Explanation:
- The Account class encapsulates common data (accountID, balance, and customerName) and provides methods to interact with these data.
- The methods deposit, withdraw, and operator+= are marked virtual, which allows them to be overridden in derived classes.
Derived Class: AccountChecking
The AccountChecking class inherits from Account and introduces a transactionFee. The deposit and withdrawal operations will consider this fee.
// AccountChecking.h
#pragma once
#include "Account.h"
class AccountChecking : public Account {
private:
float transactionFee;
public:
AccountChecking(int id, float bal, std::string name, float fee)
: Account(id, bal, name), transactionFee(fee) {}
void deposit(float amount) override {
amount -= transactionFee;
if (amount < 0) {
std::cerr << "Deposit amount is less than transaction fee!" << std::endl;
} else {
balance += amount;
std::cout << "Deposited " << amount << " after transaction fee of " << transactionFee
<< ". New balance: " << balance << std::endl;
}
}
void withdraw(float amount) override {
amount += transactionFee;
if (amount > balance) {
std::cerr << "Insufficient balance after considering transaction fee!" << std::endl;
} else {
balance -= amount;
std::cout << "Withdrew " << amount - transactionFee
<< " after transaction fee of " << transactionFee
<< ". New balance: " << balance << std::endl;
}
}
void display() const override {
Account::display();
std::cout << "Transaction Fee: " << transactionFee << std::endl;
}
};
Explanation:
- AccountChecking inherits from Account and overrides the deposit and withdraw methods to account for transactionFee.
- It ensures that the transaction fee is subtracted from the deposit amount or added to the withdrawal amount before performing the operation.
Derived Class: AccountSaving
The AccountSaving class also inherits from Account and adds a minimumBalance attribute. It restricts withdrawals or transfers that would reduce the balance below this minimum.
// AccountSaving.h
#pragma once
#include "Account.h"
class AccountSaving : public Account {
private:
float minimumBalance;
public:
AccountSaving(int id, float bal, std::string name, float minBal)
: Account(id, bal, name), minimumBalance(minBal) {}
void withdraw(float amount) override {
if (balance - amount < minimumBalance) {
std::cerr << "Cannot withdraw " << amount
<< ". Minimum balance requirement not met!" << std::endl;
} else {
balance -= amount;
std::cout << "Withdrew " << amount << ". New balance: " << balance << std::endl;
}
}
Account& operator+=(Account& other) override {
float transferAmount;
std::cout << "Enter amount to transfer from " << other.getCustomerName() << ": ";
std::cin >> transferAmount;
if (other.getBalance() >= transferAmount && (balance + transferAmount >= minimumBalance)) {
this->deposit(transferAmount);
other.withdraw(transferAmount);
} else {
std::cerr << "Transfer failed. Check balance and minimum balance requirements." << std::endl;
}
return *this;
}
void display() const override {
Account::display();
std::cout << "Minimum Balance: " << minimumBalance << std::endl;
}
};
Explanation:
- AccountSaving adds the minimumBalance attribute and enforces it during withdrawals or transfers.
- The operator+= is overridden to handle the transfer operation, ensuring that the balance never drops below the minimumBalance.
Implementing the Container Class
The Accounts class will manage multiple Account objects using a container like std::vector. It will support loading and storing accounts from a file.
// Accounts.h
#pragma once
#include <vector>
#include <fstream>
#include <iostream>
#include "AccountChecking.h"
#include "AccountSaving.h"
class Accounts {
private:
std::vector<Account*> accounts;
public:
~Accounts() {
for (auto account : accounts) {
delete account;
}
}
void addAccount(Account* account) {
accounts.push_back(account);
}
void loadAccounts(const std::string& filename) {
std::ifstream infile(filename);
if (!infile) {
std::cerr << "Failed to open file!" << std::endl;
return;
}
int numAccounts;
infile >> numAccounts;
for (int i = 0; i < numAccounts; ++i) {
int id, type;
float balance, feeOrMinBalance;
std::string name;
infile >> id >> type >> feeOrMinBalance >> balance;
infile.ignore(); // Ignore the newline
std::getline(infile, name);
if (type == 1) { // Checking account
accounts.push_back(new AccountChecking(id, balance, name, feeOrMinBalance));
} else if (type == 2) { // Saving account
accounts.push_back(new AccountSaving(id, balance, name, feeOrMinBalance));
}
}
infile.close();
}
void storeAccounts(const std::string& filename) const {
std::ofstream outfile(filename);
if (!outfile) {
std::cerr << "Failed to open file!" << std::endl;
return;
}
outfile << accounts.size() << std::endl;
for (auto account : accounts) {
auto checkingAccount = dynamic_cast<AccountChecking*>(account);
auto savingAccount = dynamic_cast<AccountSaving*>(account);
if (checkingAccount) {
outfile << checkingAccount->getAccountID() << " 1 " << checkingAccount->getBalance()
<< " " << checkingAccount->getCustomerName() << std::endl;
} else if (savingAccount) {
outfile << savingAccount->getAccountID() << " 2 " << savingAccount->getBalance()
<< " " << savingAccount->getCustomerName() << std::endl;
}
}
outfile.close();
}
void displayAccounts() const {
for (auto account : accounts) {
account->display();
}
}
};
Explanation:
- The Accounts class manages a collection of Account* objects, allowing polymorphic behavior.
- The loadAccounts method reads account data from a file and dynamically creates objects of AccountChecking or AccountSaving.
- The storeAccounts method writes the account data back to a file, ensuring the type and specific data are preserved.
Main Program Logic
The AccountSystem class will handle user interactions, allowing them to add accounts, perform transactions, and manage account data.
// AccountSystem.h
#pragma once
#include "Accounts.h"
class AccountSystem {
private:
Accounts accounts;
public:
void run() {
int choice;
while (true) {
std::cout << "1. Add Account\n2. Display Accounts\n3. Load Accounts\n4. Store Accounts\n5. Exit\n";
std::cout << "Enter choice: ";
std::cin >> choice;
if (choice == 1) {
addAccount();
} else if (choice == 2) {
accounts.displayAccounts();
} else if (choice == 3) {
accounts.loadAccounts("accounts.dat");
} else if (choice == 4) {
accounts.storeAccounts("accounts.dat");
} else if (choice == 5) {
break;
} else {
std::cerr << "Invalid choice!" << std::endl;
}
}
}
void addAccount() {
int id, type;
float balance, feeOrMinBalance;
std::string name;
std::cout << "Enter Account ID: ";
std::cin >> id;
std::cout << "Enter Account Type (1 for Checking, 2 for Saving): ";
std::cin >> type;
std::cout << "Enter Customer Name: ";
std::cin.ignore(); // To handle newline character from previous input
std::getline(std::cin, name);
std::cout << "Enter Initial Balance: ";
std::cin >> balance;
if (type == 1) {
std::cout << "Enter Transaction Fee: ";
std::cin >> feeOrMinBalance;
accounts.addAccount(new AccountChecking(id, balance, name, feeOrMinBalance));
} else if (type == 2) {
std::cout << "Enter Minimum Balance: ";
std::cin >> feeOrMinBalance;
accounts.addAccount(new AccountSaving(id, balance, name, feeOrMinBalance));
} else {
std::cerr << "Invalid account type!" << std::endl;
}
}
};
Explanation:
- The AccountSystem class is the main interface that users interact with. It allows them to add new accounts, display existing accounts, and load or store account data from/to files.
- The run method provides a simple menu for users to choose actions. The addAccount method collects user input to create and add a new account to the system.
Conclusion and Key Takeaways
This comprehensive example demonstrates how to approach a C++ programming assignment involving inheritance, polymorphism, and file handling. Here's a recap of the key steps:
- Understanding the Problem: Break down the problem statement to identify requirements and design considerations.
- Designing the Classes: Plan your class hierarchy with a base class and derived classes, ensuring appropriate use of inheritance.
- Implementing Polymorphism: Use virtual functions to enable dynamic method binding, allowing derived classes to customize base class behavior.
- Managing Data: Implement file handling to load and store account information, ensuring the persistence of data across program sessions.
- User Interaction: Create a simple interface to interact with the system, allowing users to manage accounts effectively.
By following these steps, you can solve your programming assignment with confidence and produce well-structured, maintainable code. Tackling similar programming assignments will become easier as you apply these strategies effectively.